Difference between revisions of "CSC111 Homework 5 2011"
(→Grading) |
(→Inspiration) |
||
(27 intermediate revisions by the same user not shown) | |||
Line 1: | Line 1: | ||
--[[User:Thiebaut|D. Thiebaut]] 09:05, 18 October 2011 (EDT) | --[[User:Thiebaut|D. Thiebaut]] 09:05, 18 October 2011 (EDT) | ||
---- | ---- | ||
− | [[ | + | [[Image:hw5solClooney.png|right|300px]] |
__TOC__ | __TOC__ | ||
<br /> | <br /> | ||
<br /> | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <bluebox> | ||
+ | This assignment is due on Tuesday evening, '''Oct. 25th, at midnight'''. You can work on it in pairs, or individually. Make sure you submit 3 files when you are done with this assignment. | ||
+ | </bluebox> | ||
+ | <br /> | ||
+ | |||
=Automatic Picture Editor= | =Automatic Picture Editor= | ||
Line 17: | Line 26: | ||
* The picture '''must''' have a '''gif''' extension, otherwise the graphics.py library will not be able to load it. Very likely the picture you will have of yourself will have a '''jpg''', '''jpeg''', or '''png''' extension. That's ok, you can transform it to a '''gif''' extension by uploading it to this Web site and downloading the '''gif''' version: [http://www.coolutils.com/online/image-converter/ www.coolutils.com] | * The picture '''must''' have a '''gif''' extension, otherwise the graphics.py library will not be able to load it. Very likely the picture you will have of yourself will have a '''jpg''', '''jpeg''', or '''png''' extension. That's ok, you can transform it to a '''gif''' extension by uploading it to this Web site and downloading the '''gif''' version: [http://www.coolutils.com/online/image-converter/ www.coolutils.com] | ||
* Once you have the picture, put it in the same directory (folder) where your python program will reside. | * Once you have the picture, put it in the same directory (folder) where your python program will reside. | ||
+ | * Make sure the '''graphics.py''' library is there as well. | ||
* Find out the width and height of your image (use Google to help you figure out how to do this with Windows or Mac OSX, or use a simple [http://maven.smith.edu/~111a/imagesize.htm utility] I have put together for this purpose). | * Find out the width and height of your image (use Google to help you figure out how to do this with Windows or Mac OSX, or use a simple [http://maven.smith.edu/~111a/imagesize.htm utility] I have put together for this purpose). | ||
* Then create a short '''python module''', called '''userInfo.py'''. Make sure you spell it exactly as shown, as your program wil be tested with a '''userInfo.py''' module of my own. | * Then create a short '''python module''', called '''userInfo.py'''. Make sure you spell it exactly as shown, as your program wil be tested with a '''userInfo.py''' module of my own. | ||
Line 32: | Line 42: | ||
</source> | </source> | ||
<br /> | <br /> | ||
− | :Of course, you will have to replace ''alexandra | + | :Of course, you will have to replace ''alexandra'' in the file name and caption to what you picked for your file name and your caption. |
* You are now ready to write '''hw5.py''', a python program that will import everything from the userInfo.py module, and use it to conduct its transformation. | * You are now ready to write '''hw5.py''', a python program that will import everything from the userInfo.py module, and use it to conduct its transformation. | ||
* Here's a debugging version of '''hw5.py''' that will help you figure out how things will work. | * Here's a debugging version of '''hw5.py''' that will help you figure out how things will work. | ||
Line 59: | Line 69: | ||
* You are now ready to start programming | * You are now ready to start programming | ||
− | * Here is a list of the different transformations your program will have to perform | + | * Here is a list of the different transformations your program will have to perform: |
+ | *# The demo program I used to pixelate Clooney is not very robust. It will crash if the size of the square ('''sq''') does not divide the width or height exactly. You will have to fix this so that your program can work with any value of width and height. | ||
*# Your program will put a border around the picture. The border should be at least 5 pixels wide, and be drawn using 2 different colors (at least). | *# Your program will put a border around the picture. The border should be at least 5 pixels wide, and be drawn using 2 different colors (at least). | ||
*# Your program should use some pixelation operation (similar to what we did with Clooney) to modify the face of the person in the image (but not the border!). You can use one of the methods we demonstrated in class, or come up with your own. | *# Your program should use some pixelation operation (similar to what we did with Clooney) to modify the face of the person in the image (but not the border!). You can use one of the methods we demonstrated in class, or come up with your own. | ||
− | *# Your program will display the caption inside the border, centered relative to the width of the image, and either at the top, or at the bottom of the frame. | + | *# Your program will display the caption inside the border, centered relative to the width of the image, and either at the top, or at the bottom of the frame. The text of the caption is the contents of the '''caption''' variable in the '''userInfo''' module. |
*# Your program will perform some RGB transformation of the image (but not of the frame or the caption). Use your imagination for the type of RGB transformation. The last lab shows you several options. | *# Your program will perform some RGB transformation of the image (but not of the frame or the caption). Use your imagination for the type of RGB transformation. The last lab shows you several options. | ||
− | * In addition, you will have to make sure that every transformation your program carries out on the image uses only the variables '''width''' and '''height''' that are defined in '''userInfo.py''', because your program will be tested not only with the gif file you will upload, but also with an other gif file that will be defined in a different '''userInfo.py'''. In other words, I will have a gif file different from yours, say '''111test.gif''', and a different '''userInfo.py''' module that will contain the width and height of 111test.gif, with a caption such as '''111a test caption''', and I will put both of them | + | *# The order in which your program will carry out the operations is not necessarily the same as the order in which they are listed here! |
+ | * In addition, you will have to make sure that every transformation your program carries out on the image uses only the variables '''width''' and '''height''' that are defined in '''userInfo.py''', because your program will be tested not only with the gif file you will upload, but also with an other gif file that will be defined in a different '''userInfo.py'''. In other words, I will have a gif file different from yours, say '''111test.gif''', and a different '''userInfo.py''' module that will contain the width and height of 111test.gif, with a caption such as '''111a test caption''', and I will put both of them in the same folder with your program, and I will launch your program. If your program is written correctly it will put the right border, right caption, and will apply the right transformations to the test image. | ||
− | :You can test your program by creating a second gif file of different width and height as your first image, and a new userInfo.py file, and see if your program works '''without having you to change it''' | + | :You can test your program by creating a second gif file of different width and height as your first image, and a new userInfo.py file, and see if your program works '''without having you to change it''' for the new image. |
+ | |||
+ | ==An Example== | ||
+ | * Below is an example of what your program ''could'' do. For this example I have pixelated the image using squares while transforming the colors to black and white, added two borders, and placed the caption "''George Clooney''" in the bottom border. Feel free to use different transformations. For your information, you can transform an image to black and white by setting the RGB values to the average of their values, i.e. R = G = B = (R+G+B)//3. (''Note: the size of the graphics window for the image below was 600 by 400.'') | ||
+ | |||
+ | <br /> | ||
+ | |||
+ | <center>[[Image:hw5solClooney.png]]</center> | ||
+ | |||
+ | <br /> | ||
+ | |||
+ | * The userInfo.py file for this output is | ||
+ | |||
+ | <source lang="python"> | ||
+ | # userInfo.py | ||
+ | # module containing information about a gif file | ||
+ | # to be process by hw5.py | ||
+ | filename = "Clooney.gif" | ||
+ | caption = "George Clooney" | ||
+ | width = 600 | ||
+ | height = 400 | ||
+ | </source> | ||
==Submission== | ==Submission== | ||
Line 75: | Line 108: | ||
** and your '''gif image file'''. | ** and your '''gif image file'''. | ||
− | * If you forget any one of these files, your program will crash when I run it, and your grade will be at most a | + | * If you forget any one of these files, your program will crash when I run it, and your grade will be at most a C-. |
* Use the online submission form to submit your files: [http://cs.smith.edu/~111a/submit5.htm submit5] | * Use the online submission form to submit your files: [http://cs.smith.edu/~111a/submit5.htm submit5] | ||
Line 86: | Line 119: | ||
<br /> | <br /> | ||
<br /> | <br /> | ||
+ | |||
+ | =Inspiration= | ||
+ | (Section added on 10/23/11) | ||
+ | * Here are some portraits that involve some computer alteration. Some of them are more complex than what we can do with this assignment, but the idea here is to give you some inspiration! :-) | ||
+ | [[Image:CSCPixelationPortrait0.png|175px]] | ||
+ | [[Image:CSCPixelationPortrait1.jpg|175px]] | ||
+ | <!--[[Image:CSCPixelationPortrait2.jpg|175px]]--> | ||
+ | [[Image:CSCPixelationPortrait4.jpg|175px]] | ||
+ | [[Image:CSCPixelationPortrait5.jpg|175px]] | ||
+ | [[Image:CSCPixelationPortrait6.jpg|175px]] | ||
+ | [[Image:CSCPixelationPortrait7.jpg|175px]] | ||
+ | [[Image:CSCPixelationPortrait8.jpg|175px]] | ||
+ | [[Image:CSCPixelationPortrait3.jpg|175px]] | ||
+ | [[Image:CSCPixelationPortrait9.jpg|175px]] | ||
+ | [[Image:CSCPixelationPortrait10.jpg|175px]] | ||
+ | [[Image:CSCPixelationPortrait11.jpg|175px]] | ||
+ | [[Image:CSCPixelationPortrait12.jpg|175px]] | ||
+ | [[Image:CSCPixelationPortrait13.jpg|175px]] | ||
+ | |||
+ | <onlydft> | ||
+ | Code for the image size reporting php program: | ||
+ | |||
+ | ===uploadImage.php=== | ||
+ | <source lang="php"> | ||
+ | |||
+ | <?php | ||
+ | // uploadImage.php | ||
+ | // D. Thiebaut | ||
+ | |||
+ | $theFileName = $_FILES['uploaded']['name']; | ||
+ | |||
+ | $target = "uploads2"; | ||
+ | $target = $target . "/images"; | ||
+ | mkdir( $target, 0777 ); | ||
+ | $target = $target . "/" . basename( $_FILES['uploaded']['name']) ; | ||
+ | |||
+ | |||
+ | if (move_uploaded_file($_FILES['uploaded']['tmp_name'], $target)) { | ||
+ | |||
+ | list($width, $height, $type, $attr) = getimagesize( $target ); | ||
+ | |||
+ | echo "<html><body><h2>Image Properties</h2><P><P><P><ul>"; | ||
+ | echo "<LI>Image name $theFileName"; | ||
+ | echo "<LI>Image width $width"; | ||
+ | echo "<BR>"; | ||
+ | echo "<LI>Image height $height"; | ||
+ | echo "<BR>"; | ||
+ | echo "</UL><P><P>"; | ||
+ | echo "<img src=\"$target\" width=\"300\"><P>"; | ||
+ | } | ||
+ | else { | ||
+ | echo "<html><body><h2>Error...</h2>"; | ||
+ | } | ||
+ | |||
+ | echo "</body></html>"; | ||
+ | |||
+ | ?> | ||
+ | |||
+ | </source> | ||
+ | |||
+ | ===imagesize.htm=== | ||
+ | |||
+ | <source lang="php"> | ||
+ | <html> | ||
+ | <head> | ||
+ | <title>111a Image-Size Utility</title> | ||
+ | </head> | ||
+ | <body> | ||
+ | <h2>Show Image Geometry</h2> | ||
+ | |||
+ | <h3>Utility for Homework 5</h3> | ||
+ | <form enctype="multipart/form-data" action="uploadImage.php" method="POST"> | ||
+ | <P> | ||
+ | <input type="hidden" name="hw" value="hw5" /> | ||
+ | <!--P>Which homework assignment (i.e. hw1, hw2...)? <input type="text" name="hw" /--> | ||
+ | |||
+ | <P> | ||
+ | <P> | ||
+ | Please pick an image file for which you want the geometry: <input name="uploaded" type="file" /><br /> | ||
+ | <P> | ||
+ | <P> | ||
+ | <input type="submit" value="Upload" /> | ||
+ | </form> | ||
+ | </body> | ||
+ | </html> | ||
+ | </source> | ||
+ | |||
+ | </onlydft> | ||
[[Category:CSC111]][[Category:Python]][[Category:Homework]] | [[Category:CSC111]][[Category:Python]][[Category:Homework]] |
Latest revision as of 12:38, 23 October 2011
--D. Thiebaut 09:05, 18 October 2011 (EDT)
Contents
This assignment is due on Tuesday evening, Oct. 25th, at midnight. You can work on it in pairs, or individually. Make sure you submit 3 files when you are done with this assignment.
Automatic Picture Editor
Overview
- Your assignment is to write a graphics program that takes a picture of you and modify it by apply several different transformations, in fashion similar to what we did to poor George Clooney (see program).
- The challenging part is that your program should work for pictures of different geometry, and with different captions (more on that later).
Preparation
- It will be easier if you do not use beowulf for this assignment. Use a computer in the lab, or your own computer to perform the work on this assignment.
- Select a picture of yourself that is in the area of 300 by 200 pixels (if possible). Don't select something that is 1000 by 1000 or your program will take forever to process it and you will waste a lot of time debugging.
- The picture must have a gif extension, otherwise the graphics.py library will not be able to load it. Very likely the picture you will have of yourself will have a jpg, jpeg, or png extension. That's ok, you can transform it to a gif extension by uploading it to this Web site and downloading the gif version: www.coolutils.com
- Once you have the picture, put it in the same directory (folder) where your python program will reside.
- Make sure the graphics.py library is there as well.
- Find out the width and height of your image (use Google to help you figure out how to do this with Windows or Mac OSX, or use a simple utility I have put together for this purpose).
- Then create a short python module, called userInfo.py. Make sure you spell it exactly as shown, as your program wil be tested with a userInfo.py module of my own.
# userInfo.py
# module containing information about a gif file
# to be process by hw5.py
filename = "alexandra.gif"
caption = "Alexandra"
width = 400
height = 300
- Of course, you will have to replace alexandra in the file name and caption to what you picked for your file name and your caption.
- You are now ready to write hw5.py, a python program that will import everything from the userInfo.py module, and use it to conduct its transformation.
- Here's a debugging version of hw5.py that will help you figure out how things will work.
# hw5.py
# your name
from userInfo import *
from graphics import *
def main():
print( "Gif file: ", filename ) # this will print alexandra.gif
print( "width of gif file: ", width ) # this will print 400
print( "height of gif file: ", height ) # this will print 300
print( "User name: ", caption ) # this will print Alexandra
main()
Your assignment
- You are now ready to start programming
- Here is a list of the different transformations your program will have to perform:
- The demo program I used to pixelate Clooney is not very robust. It will crash if the size of the square (sq) does not divide the width or height exactly. You will have to fix this so that your program can work with any value of width and height.
- Your program will put a border around the picture. The border should be at least 5 pixels wide, and be drawn using 2 different colors (at least).
- Your program should use some pixelation operation (similar to what we did with Clooney) to modify the face of the person in the image (but not the border!). You can use one of the methods we demonstrated in class, or come up with your own.
- Your program will display the caption inside the border, centered relative to the width of the image, and either at the top, or at the bottom of the frame. The text of the caption is the contents of the caption variable in the userInfo module.
- Your program will perform some RGB transformation of the image (but not of the frame or the caption). Use your imagination for the type of RGB transformation. The last lab shows you several options.
- The order in which your program will carry out the operations is not necessarily the same as the order in which they are listed here!
- In addition, you will have to make sure that every transformation your program carries out on the image uses only the variables width and height that are defined in userInfo.py, because your program will be tested not only with the gif file you will upload, but also with an other gif file that will be defined in a different userInfo.py. In other words, I will have a gif file different from yours, say 111test.gif, and a different userInfo.py module that will contain the width and height of 111test.gif, with a caption such as 111a test caption, and I will put both of them in the same folder with your program, and I will launch your program. If your program is written correctly it will put the right border, right caption, and will apply the right transformations to the test image.
- You can test your program by creating a second gif file of different width and height as your first image, and a new userInfo.py file, and see if your program works without having you to change it for the new image.
An Example
- Below is an example of what your program could do. For this example I have pixelated the image using squares while transforming the colors to black and white, added two borders, and placed the caption "George Clooney" in the bottom border. Feel free to use different transformations. For your information, you can transform an image to black and white by setting the RGB values to the average of their values, i.e. R = G = B = (R+G+B)//3. (Note: the size of the graphics window for the image below was 600 by 400.)
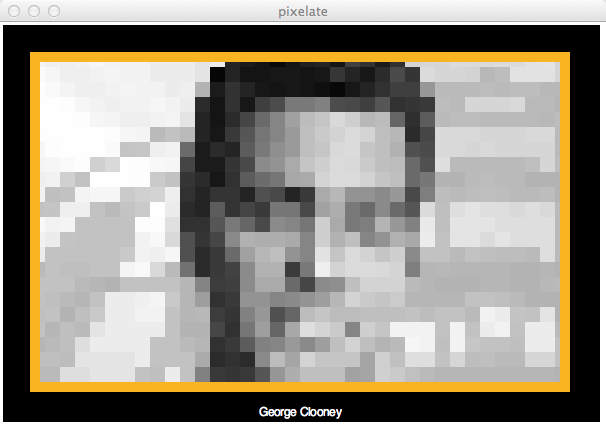
- The userInfo.py file for this output is
# userInfo.py
# module containing information about a gif file
# to be process by hw5.py
filename = "Clooney.gif"
caption = "George Clooney"
width = 600
height = 400
Submission
- You have to submit three files:
- hw5.py,
- userInfo.py,
- and your gif image file.
- If you forget any one of these files, your program will crash when I run it, and your grade will be at most a C-.
- Use the online submission form to submit your files: submit5
Grading
- Up to 2/3 extra points will be given to imaginative designs (both for the graphics, and for the code).
- Up to a point will be removed for missing or incomplete documentation. Look at the posted solutions for previous Homework assignments to see examples of good documentation.
- A program that works on the user-supplied image, but not on the instructor supplied image will be worth at most B.
- A program that crashes when it runs will be worth at most C-.
Inspiration
(Section added on 10/23/11)
- Here are some portraits that involve some computer alteration. Some of them are more complex than what we can do with this assignment, but the idea here is to give you some inspiration! :-)