Difference between revisions of "CSC270 Exercises on Assembly Language"
(→Pause) |
(→Exercise 7) |
||
(6 intermediate revisions by the same user not shown) | |||
Line 56: | Line 56: | ||
<br /> | <br /> | ||
<br /> | <br /> | ||
+ | ==Exercise 6== | ||
+ | |||
+ | * Back to Exercise 4. Go through each instruction and identify the different addressing modes you used for each instruction. | ||
+ | |||
+ | ==Exercise 7== | ||
+ | |||
+ | * Analyze the program below and make it shorter and/or faster. Your program should compute the same result as the original program. | ||
+ | |||
<br /> | <br /> | ||
+ | <source lang="asm"> | ||
+ | |||
+ | org 0 | ||
+ | jmp start | ||
+ | |||
+ | a db 0 | ||
+ | b db 0 | ||
+ | c db 0 | ||
+ | |||
+ | start: ldaa a | ||
+ | inca | ||
+ | staa a | ||
+ | ldaa b | ||
+ | inca | ||
+ | inca | ||
+ | staa b | ||
+ | ldaa c | ||
+ | inca | ||
+ | inca | ||
+ | inca | ||
+ | staa c | ||
+ | jmp start | ||
+ | |||
+ | </source> | ||
<br /> | <br /> | ||
+ | |||
<br /> | <br /> | ||
<br /> | <br /> | ||
Line 65: | Line 98: | ||
<br /> | <br /> | ||
<br /> | <br /> | ||
+ | <br /> | ||
+ | <onlydft> | ||
+ | <code><pre> | ||
+ | a db 0 ; | ||
+ | b db 0 | ||
+ | c db 0 | ||
+ | |||
+ | start: ldaa #1 ; 86 01 2 cycles | ||
+ | staa a ; 97 00 3 cycles | ||
+ | inca ; 4C 2 cycles | ||
+ | staa b ; 97 01 3 cycles | ||
+ | inca ; 4C 2 cycles | ||
+ | staa c ; 97 02 3 cycles | ||
+ | jmp start ; 7E 00 03 3 cycles | ||
+ | ;; ----- ---------- | ||
+ | ;; 13 bytes 18 cycles | ||
+ | |||
+ | |||
+ | |||
+ | |||
+ | </pre></code> | ||
+ | </onlydft> | ||
[[Category:CSC270]][[Category:6811]][[Category:6800]][[Category:Exercises]] | [[Category:CSC270]][[Category:6811]][[Category:6800]][[Category:Exercises]] |
Latest revision as of 09:26, 2 April 2012
--D. Thiebaut 16:20, 27 March 2012 (EDT)
Contents
Learning Assembly Through Exercises
Exercise 1
- Write a program that uses 3 byte variables, a, b, and c. The variable are initialized before the program starts with the values 2, 5, and 0, respectively.
- The program will add up the contents of the variable a to that of b and store the result into c. Only c will change.
Exercise 2
- Assemble the program of Exercise 1
- How many bytes does it contain?
- How fast will it go from beginning to end, assuming each 6811 cycle is 1 µsecond?
Exercise 3
- Same as Exercise 1, but for the Pentium.
- Assuming that the Pentium runs at a clock speed of 3 GHz, how many times could the program run on the Pentium while the same program run on the 1MHz 6811?
Exercise 4
- We want to compute Y = a + b - c + 3, where Y, a, b, and c are byte variables.
- Write the program that performs this operation.
- Assemble the program
- Count the total number of cycles it will take to run, and the total number of bytes it will use up in RAM.
Exercise 5
- Write a program that computes the first 5 terms of the fibonacci sequence, assuming that your program starts with 5 variables that all zero.
- Assemble it.
- How many cycles?
- How many bytes?
Pause
- Let's learn about direct addressing mode
- The excerpt below is taken from Introduction to 6811 Programming by Fred G. Martin.
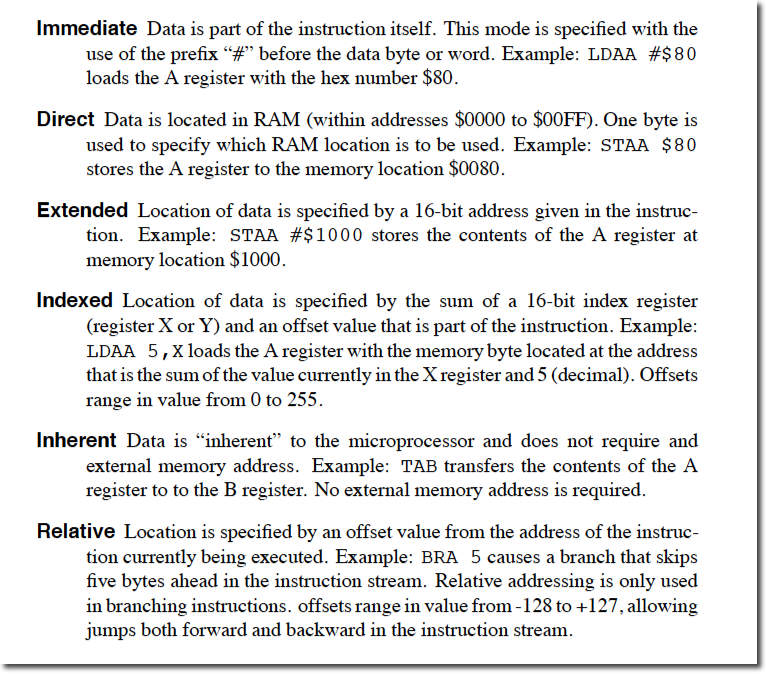
Exercise 6
- Back to Exercise 4. Go through each instruction and identify the different addressing modes you used for each instruction.
Exercise 7
- Analyze the program below and make it shorter and/or faster. Your program should compute the same result as the original program.
org 0
jmp start
a db 0
b db 0
c db 0
start: ldaa a
inca
staa a
ldaa b
inca
inca
staa b
ldaa c
inca
inca
inca
staa c
jmp start