Difference between revisions of "CSC103 Homework 3 2013"
(→Preparation: Step 2) |
(→Preparation: Step 2) |
||
Line 80: | Line 80: | ||
sto 10 ; store AC back at Location 10 | sto 10 ; store AC back at Location 10 | ||
sub-c 20 ; subtract 20 from AC | sub-c 20 ; subtract 20 from AC | ||
− | jmz done | + | jmz done ; if AC is zero, jump to Label done |
− | jmp 0 | + | jmp 0 ; jump to Address 0 |
− | done: hlt | + | done: hlt ; stop |
@10 | @10 |
Revision as of 13:53, 25 September 2013
--D. Thiebaut (talk) 08:34, 24 September 2013 (EDT)
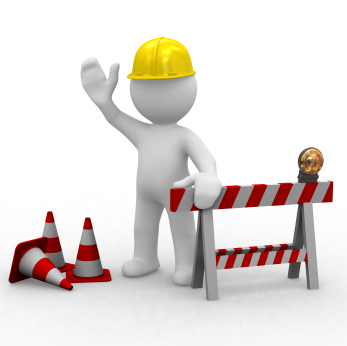
Contents
Problem 1
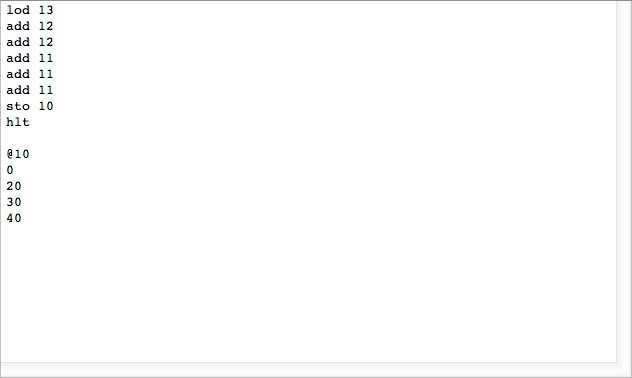
The program above is very cryptic. Your assignment is to add comments to the program and give names to the
different variables to make it more easily understandable. A comment is just some documentation following a semi-colon.
Use the program below as an example of what you should emulate. Do not put my name on your listing, but yours instead.
; sum of 2 integers
; D. Thiebaut CSC103
; The program takes 2 variables stored in memory at
; Address 10 (and up) computes their sum and stores
; it in a 3rd variable.
@0 ;code section starts at address 0
start: lod var1 ;AC <- 3
add var2 ;AC <- 3+5 = 8
sto sum ;sum <- 8
hlt
@10 ;data section starts at Address 1
var1: 3
var2: 5
sum: 0
Make sure that you add comments to the right of each instruction indicating what the instructions do. Make sure you
indicate as well what the final result of the computation is.
Problem 2: Mystery Program
I have created a program with the simulator, translated it, and it has been loaded into memory. But instead of showing it to you as instructions, I am showing it you as numbers...
Your job is to reconstruct my original program, fully documented, as for Problem 1.
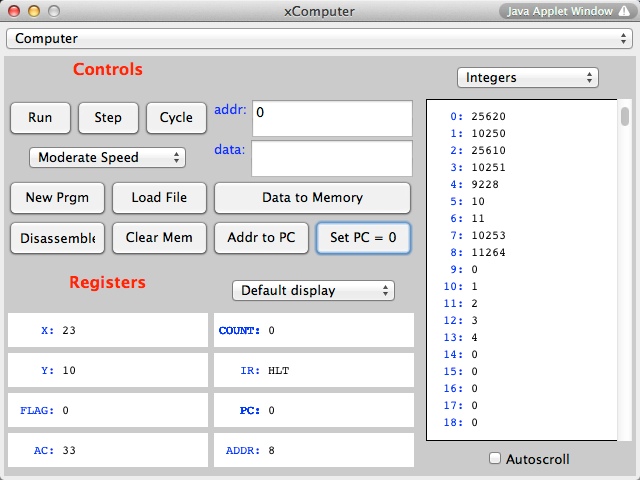
Challenging Problem
Preparation: Step 1
First load this program in the simulator and single step it. Click on cycle slowly and verify that whatever happens with the AC register makes sense:
@0
lod 10
add-c 1
sto 10
jmp 0
@10
5
You will notice that the program has an infinite loop and that it keeps on doing whatever it is doing... forever.
Let's make it stop after a while. That's Step 2.
Preparation: Step 2
I have modified the program and added a few instructions:
@0
lod 10 ; AC <-- contents of Memory Location 10
add-c 1 ; AC <-- AC + 1
sto 10 ; store AC back at Location 10
sub-c 20 ; subtract 20 from AC
jmz done ; if AC is zero, jump to Label done
jmp 0 ; jump to Address 0
done: hlt ; stop
@10
0 ; we use Memory Location 10 as a counter
The program below computes the sum of some numbers. You have to figure out what it does.
@0
lod counter
add sum
sto sum
lod counter
add-c 1
sto counter
sub max
jmz done
jmp 0
done: hlt
@10
counter: 0
max: 6
sum: 0
The tricky part of the program is with the instruction JMZ done. JMZ is a jump instruction. It is similar to