Difference between revisions of "CSC111 Lab 2 2014"
(→Challenge #3: and ATM that delivers bills and coins) |
(→Challenge #6: Programmable ATM Machine) |
||
(23 intermediate revisions by the same user not shown) | |||
Line 2: | Line 2: | ||
---- | ---- | ||
<br /> | <br /> | ||
+ | |||
=Teller Machine Program= | =Teller Machine Program= | ||
[[Image:ATM.jpg|right|200px]] | [[Image:ATM.jpg|right|200px]] | ||
Line 51: | Line 52: | ||
<br /> | <br /> | ||
<source lang="python"> | <source lang="python"> | ||
− | no20 = amount // | + | no20 = amount // 20 |
− | leftover = amount % | + | leftover = amount % 20 |
</source> | </source> | ||
<br /> | <br /> | ||
Line 79: | Line 80: | ||
* Run your program and make sure it sill outputs the correct result. | * Run your program and make sure it sill outputs the correct result. | ||
<br /> | <br /> | ||
+ | |||
==Flexibility and Adaptability== | ==Flexibility and Adaptability== | ||
<br /> | <br /> | ||
Line 124: | Line 126: | ||
<br /> | <br /> | ||
− | =Functions: abs(), int(), str()= | + | =Python Functions: abs(), int(), str(), len()= |
<br /> | <br /> | ||
Python supports many functions. A very good way to understand how they work is to try them in the '''Console''' window of Idle, i.e. the window where programs output their result. | Python supports many functions. A very good way to understand how they work is to try them in the '''Console''' window of Idle, i.e. the window where programs output their result. | ||
Line 159: | Line 161: | ||
>>> amount = 1234 | >>> amount = 1234 | ||
>>> 1234 // 10 | >>> 1234 // 10 | ||
+ | |||
+ | >>> 1234 // 100 | ||
+ | |||
+ | >>> 1234 % 100 | ||
+ | |||
+ | >>> 1234.0 % 100 | ||
+ | |||
+ | >>> int( 1234.0 ) % 100 | ||
>>> 'a' * '5' | >>> 'a' * '5' | ||
Line 177: | Line 187: | ||
>>> print( str( 5 ) + "apples" ) | >>> print( str( 5 ) + "apples" ) | ||
+ | |||
+ | >>> dog = "Pluto" | ||
+ | >>> len( dog ) | ||
+ | |||
+ | >>> dog = "+ " + dog + " +" | ||
+ | >>> dog | ||
+ | |||
+ | >>> len( dog ) | ||
+ | |||
+ | >>> "-" * len( dog ) | ||
</pre></code> | </pre></code> | ||
Line 208: | Line 228: | ||
|- | |- | ||
| | | | ||
− | ==Challenge #3: | + | ==Challenge #3: an ATM that delivers bills and coins== |
|} | |} | ||
[[Image:QuestionMark3.jpg|right|120px]] | [[Image:QuestionMark3.jpg|right|120px]] | ||
Line 223: | Line 243: | ||
0 nickel(s) | 0 nickel(s) | ||
2 penny or pennies | 2 penny or pennies | ||
+ | |||
+ | <br /> | ||
+ | <br /> | ||
+ | |||
+ | =The '''input()''' Function= | ||
+ | <br /> | ||
+ | Try the following statements in the console window. Again, some of these might generate errors. It is important for you to understand why these errors occur. | ||
+ | |||
+ | <br /> | ||
+ | <code><pre> | ||
+ | >>> firstName = input( "Enter your first name: " ) | ||
+ | >>> firstName | ||
+ | |||
+ | >>> lastName = input( "Enter your last name: " ) | ||
+ | >>> lastName | ||
+ | |||
+ | >>> print( firstName, lastName ) | ||
+ | |||
+ | >>> x = input( "enter a number: " ) | ||
+ | >>> x | ||
+ | |||
+ | >>> x + 5 | ||
+ | |||
+ | >>> x = input( "Enter a number: " ) | ||
+ | >>> x | ||
+ | |||
+ | >>> x = int( answer ) | ||
+ | >>> x | ||
+ | |||
+ | >>> y = int( "314.56789" ) | ||
+ | >>> y | ||
+ | </pre></code> | ||
+ | <br /> | ||
+ | |||
+ | <br /> | ||
+ | {| style="width:100%; background:silver" | ||
+ | |- | ||
+ | | | ||
+ | ==Challenge #4: Gathering student information== | ||
+ | |} | ||
+ | [[Image:QuestionMark4.jpg|right|120px]] | ||
+ | <br /> | ||
+ | Write a python program that asks the user for her name, box number, 99-Id number, and phone number, and that outputs it back on the screen nicely formatted. | ||
+ | |||
+ | Example (the information entered by the user is in boldface): | ||
+ | |||
+ | your first name? '''Allie''' | ||
+ | your last name? '''Gator''' | ||
+ | your box number? '''1234''' | ||
+ | your phone number? '''413 456 7890''' | ||
+ | your Smith Id? '''990123456''' | ||
+ | |||
+ | |||
+ | Name: Allie Gator (990123456) | ||
+ | box #: 1234 | ||
+ | phone: 413 456 7890 | ||
+ | |||
+ | <br /> | ||
+ | <br /> | ||
+ | {| style="width:100%; background:silver" | ||
+ | |- | ||
+ | | | ||
+ | |||
+ | ==Challenge #5: (Challenging) Printing the student information it in a box== | ||
+ | |} | ||
+ | [[Image:QuestionMark5.jpg|right|120px]] | ||
+ | <br /> | ||
+ | Same idea as with the previous challenge but after inputing the information from the user, the program outputs it in a box! | ||
+ | |||
+ | Example: | ||
+ | |||
+ | <center>[[Image:Lab2_2014_boxedOutput.png]]</center> | ||
+ | <br /> | ||
+ | |||
+ | |||
+ | <br /> | ||
+ | <br /> | ||
+ | {| style="width:100%; background:silver" | ||
+ | |- | ||
+ | | | ||
+ | ==Challenge #6: Programmable ATM Machine== | ||
+ | |} | ||
+ | [[Image:QuestionMark6.jpg|right|120px]] | ||
+ | <br /> | ||
+ | Modify your last ATM program so that it prompts the user for an amount of money to withdraw, and then it breaks it down into number of bills of different denominations. | ||
+ | <br /> | ||
+ | Here is an example with the user input in boldface: | ||
+ | |||
+ | |||
+ | Please enter the amount you want to withdraw: '''231.50''' | ||
+ | Please lift you keyboard and find: | ||
+ | ... | ||
+ | |||
+ | |||
+ | <br /> | ||
+ | <br /> | ||
+ | {| style="width:100%; background:silver" | ||
+ | |- | ||
+ | | | ||
+ | |||
+ | ==Challenge #7: Seconds to Days, Hours, Minutes, Second converter== | ||
+ | |} | ||
+ | [[Image:QuestionMark8.jpg|right|120px]] | ||
+ | <br /> | ||
+ | Write a program that prompts the user for a time expressed as a '''number of seconds'''. The program then outputs the equivalent number of days, hours, minutes, and seconds. | ||
+ | |||
+ | Make the program output the result in a box, similar to the one shown below (the user input is in boldface): | ||
+ | |||
+ | Please enter a time expressed in seconds: '''3662''' | ||
+ | |||
+ | |||
+ | +--------------------------------------------+ | ||
+ | | 0 day(s) 1 hour(s) 1 minute(s) 2 second(s) | | ||
+ | +--------------------------------------------+ | ||
+ | |||
+ | <br /> | ||
+ | |||
+ | =Submission= | ||
+ | <br /> | ||
+ | * Store the last program that you were able to run without errors in a file called '''lab2.py'''. | ||
+ | |||
+ | * Write down your name at the top of the program, in a comment, and add your 2-letter Id (the list of 2-letter Ids is available [[CSC111_2-Letter_Ids_2014| here]]). Follow this example | ||
+ | |||
+ | # lab2.py | ||
+ | # Mickey Mouse (aa) | ||
+ | # Solution program for Challenge 3 | ||
+ | |||
+ | * If you worked on this lab with a partner, add the two names in the program header. | ||
+ | |||
+ | # lab2.py | ||
+ | # Mickey Mouse (aa) | ||
+ | # Donald Duck (cc) | ||
+ | # Solution program for Challenge 7 | ||
+ | |||
+ | * Submit your program [http://cs.smith.edu/~thiebaut/111b/submitL2.php here]. | ||
+ | |||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | You are now ready for [[CSC111 Homework 2 2014| Homework #2]]! | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | [[Category:CSC111]][[Category:Labs]][[Category:Python]] |
Latest revision as of 15:28, 6 February 2014
--D. Thiebaut (talk) 14:06, 4 February 2014 (EST)
Contents
Teller Machine Program
This section has to do with a program that takes some integer (without a decimal part) amount of dollars and figures out how to break it down into the least number of 20-, 10-, 5-, and 1-bills.
Pseudo-Code
On a piece of paper, figure out an algorithm for taking the amount of money to withdraw from the ATM, or teller machine, and compute the number of different bills. Write it down in pseudocode, i.e. a mixture of English and Python.
- You need to start with some amount
- You compute first the number of $20 bills,
- then the number of $10 bills,
- ...
- down to the number of $1 bills.
Comments
- Use Idle and open a new window.
- In a new window, put down your pseudo code in several lines and put a #-sign in front of each line, so that they become Python comments.
- Try to run the program and verify that no errors are detected. There shouldn't be any Python code in your program, only comments. Idle will be able to tell you if it sees anything else that is not a comment!
Adding Code
- Now that you have the structure for your program, add enough Python code to make your code display
- The total amount
- The number of $20-bills
- When you get the right number output, add some more python to get the program to print the amount of $10-bills.
- Finish up the program
- Verify that your program works. Below is a typical output you should try to emulate:
Amount to withdraw = 97 Please lift keyboard and find: 4 $20-bill(s) 1 $10-bill(s) 1 $5-bill(s) 2 $1-bill(s)
Using Constants
- You probably have statements like these in your program
no20 = amount // 20
leftover = amount % 20
- Instead of using the literals 20, 10, 5, and 1 in the program, we can use variables to hold them. It adds to the complexity a tiny bit, but makes the program more useful. You'll see how.
- Modify your program and add these variables near the top of the program:
DENOM1 = 20
DENOM2 = 10
DENOM3 = 5
DENOM4 = 1
- and where you compute the number of $20-bills, replace 20 by DENOM1. You should get something like this:
noBills = amount // DENOM1
leftover = amount % DENOM1
print( noBills, "$", DENOM1, "bill(s)" )
- Run your program. Make sure it still outputs the correct result after this modification. If not, fix the error(s)!
- Replace 10, 5, and 1 by DENOM2, DENOM3, DENOM4 in the remaining part of your program.
- Run your program and make sure it sill outputs the correct result.
Flexibility and Adaptability
Imagine that your program will be used in an area where the bills do not come in 20, 10, 5 or 1 denominations, but in 100, 50, 10, and 1.
Figure out a way to make the least amount of change to your program so that it now outputs the correct break down for any amount, but in $100-, $50-, $10- and $1-bills.
Make sure your program works! Below is the output of the program if the amount is set to $97:
Amount to withdraw = $ 97 Please lift keyboard and find: 0 $ 100 bill(s) 1 $ 50 bill(s) 4 $ 10 bill(s) 7 $ 1 bill(s)
Challenge #1: A Change Machine |
- Using a similar approach, write a program that, given some number of pennies, will output the correct number of quarters, dimes, nickels, and pennies. You should initialize the amount of pennies with an integer, like this:
pennies = 84
- Here is an example of what your program should output for 84 pennies:
Amount to withdraw = 84 penny or pennies Please lift keyboard and find: 3 quarter(s) 0 dime(s) 1 nickel(s) 4 penny or pennies
Python Functions: abs(), int(), str(), len()
Python supports many functions. A very good way to understand how they work is to try them in the Console window of Idle, i.e. the window where programs output their result.
Below is a series of statements you should type in the console, and as you see how Python interprets these statements, this should help you figure out how the functions work.
>>> a = 10.34
>>> a
>>> int( a )
>>> a = -10.34
>>> abs( a )
>>> abs( a * 100 )
>>> str( 10.34 )
>>> 'hello' + 10.34
>>> 'hello' + str( 10.34 )
>>> amount = 123.45
>>> int( amount )
>>> int( amount * 10 )
>>> int( amount / 10 )
>>> amount = 1234
>>> 1234 // 10
>>> 1234 // 100
>>> 1234 % 100
>>> 1234.0 % 100
>>> int( 1234.0 ) % 100
>>> 'a' * '5'
>>> 'a' * 5
>>> '3' * 5
>>> 3 * 5
>>> str( 3 ) + str( 5 )
>>> str( 3 ) * 5
>>> print( 5, "apples" )
>>> print( 5 + "apples" )
>>> print( str( 5 ) + "apples" )
>>> dog = "Pluto"
>>> len( dog )
>>> dog = "+ " + dog + " +"
>>> dog
>>> len( dog )
>>> "-" * len( dog )
Challenge #2: Cleaning Up the Output |
Using what you just learned, modify your ATM program (the one that outputs the number of bills), and make the output look neat.
In particular, change the output that looks like this:
4 $ 20 bill(s) 1 $ 10 bill(s) 1 $ 5 bill(s) 2 $ 1 bill(s)
and make it look like this:
4 $20 bill(s) 1 $10 bill(s) 1 $5 bill(s) 2 $1 bill(s)
Challenge #3: an ATM that delivers bills and coins |
Combine the two program sections, the one that delivers bills and the one that delivers coins and create a program that would allow an amount of the form 123.47 to be withdrawn from the machine. In this case the output of your program should be:
6 $20 bill(s) 0 $10 bill(s) 0 $5 bill(s) 3 $1 bill(s) 1 quarter(s) 2 dime(s) 0 nickel(s) 2 penny or pennies
The input() Function
Try the following statements in the console window. Again, some of these might generate errors. It is important for you to understand why these errors occur.
>>> firstName = input( "Enter your first name: " )
>>> firstName
>>> lastName = input( "Enter your last name: " )
>>> lastName
>>> print( firstName, lastName )
>>> x = input( "enter a number: " )
>>> x
>>> x + 5
>>> x = input( "Enter a number: " )
>>> x
>>> x = int( answer )
>>> x
>>> y = int( "314.56789" )
>>> y
Challenge #4: Gathering student information |
Write a python program that asks the user for her name, box number, 99-Id number, and phone number, and that outputs it back on the screen nicely formatted.
Example (the information entered by the user is in boldface):
your first name? Allie your last name? Gator your box number? 1234 your phone number? 413 456 7890 your Smith Id? 990123456 Name: Allie Gator (990123456) box #: 1234 phone: 413 456 7890
Challenge #5: (Challenging) Printing the student information it in a box |
Same idea as with the previous challenge but after inputing the information from the user, the program outputs it in a box!
Example:
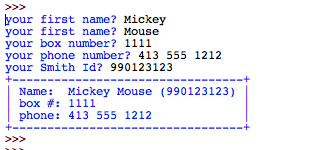
Challenge #6: Programmable ATM Machine |
Modify your last ATM program so that it prompts the user for an amount of money to withdraw, and then it breaks it down into number of bills of different denominations.
Here is an example with the user input in boldface:
Please enter the amount you want to withdraw: 231.50 Please lift you keyboard and find: ...
Challenge #7: Seconds to Days, Hours, Minutes, Second converter |
Write a program that prompts the user for a time expressed as a number of seconds. The program then outputs the equivalent number of days, hours, minutes, and seconds.
Make the program output the result in a box, similar to the one shown below (the user input is in boldface):
Please enter a time expressed in seconds: 3662 +--------------------------------------------+ | 0 day(s) 1 hour(s) 1 minute(s) 2 second(s) | +--------------------------------------------+
Submission
- Store the last program that you were able to run without errors in a file called lab2.py.
- Write down your name at the top of the program, in a comment, and add your 2-letter Id (the list of 2-letter Ids is available here). Follow this example
# lab2.py # Mickey Mouse (aa) # Solution program for Challenge 3
- If you worked on this lab with a partner, add the two names in the program header.
# lab2.py # Mickey Mouse (aa) # Donald Duck (cc) # Solution program for Challenge 7
- Submit your program here.
You are now ready for Homework #2!