Difference between revisions of "CSC111 JES Page on Sound Processing"
(→Reference) |
(→Reference) |
||
Line 10: | Line 10: | ||
<br /> | <br /> | ||
+ | <br /> | ||
+ | =Videos= | ||
+ | <br /> | ||
+ | * <center><videoflash>s9GBf8y0lY0</videoflash> | ||
+ | </center> | ||
+ | <br />Physics of sound: A metal plate is connected to an oscillator and frequency is increased... | ||
+ | * <center><videoflash>cK2-6cgqgYA</videoflash></center> | ||
+ | <br />The source of waves] (1933). You may skip after the first 6 minutes. | ||
+ | * ''Morning Edition'' 's Report on [http://www.npr.org/templates/story/story.php?storyId=102234687 Guito Monks] | ||
+ | |||
+ | ** Playing with [[Media:thisisatest.wav | ThisIsATest.wav]] | ||
+ | [[Image:SoundMediaTool.png | right | 300px ]] | ||
+ | ** Sound Tool | ||
+ | ** Increasing the amplitude of the signal (doubling) | ||
+ | ** Noise issue | ||
+ | ** Decreasing the amplitude of the signal. | ||
+ | ** Reducing the noise | ||
+ | ** Saturating/clipping the noise | ||
+ | ** Copying sound clip | ||
+ | ** Creating an echo | ||
+ | <br /> | ||
=Reference= | =Reference= | ||
<br /> | <br /> |
Revision as of 21:49, 23 February 2014
--D. Thiebaut (talk) 20:12, 23 February 2014 (EST)
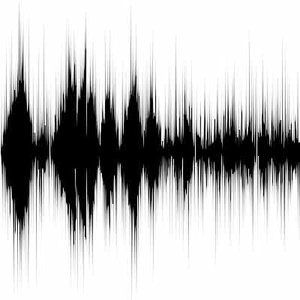
Contents
Download Page
Videos
Physics of sound: A metal plate is connected to an oscillator and frequency is increased...
The source of waves] (1933). You may skip after the first 6 minutes.
- Morning Edition 's Report on Guito Monks
- Playing with ThisIsATest.wav
- Sound Tool
- Increasing the amplitude of the signal (doubling)
- Noise issue
- Decreasing the amplitude of the signal.
- Reducing the noise
- Saturating/clipping the noise
- Copying sound clip
- Creating an echo
Reference
- JES's reference page from Depauw University
- Lab 7 CSC111 2010
- Lab 6 CSC231 2010
- [http://cs.smith.edu/classwiki/index.php/CSC111_Lab_7
Installation
Sound Files
- Get some sample wav files:
- Note: There's more at http://buggerluggs.tripod.com/ie/wav-dir184.htm and http://www.duke.edu/~rfb/palace/wavs/dratcomp.wav. If you want to download more to your account, simply find the URL of a wav file, for example http://www.duke.edu/~rfb/palace/wavs/energize.wav, and download it to your computer.
If you want to install JES on your personal Windows computer
If you want to install JES on your Windows laptop or computer, you can get Jes from the Google code web site. Grab the 3.2.1 version for Windows and install it on your Windows machine.
If you want to install JES on your personal Mac
Jes for the Mac is available on Google code. Get Jes 3.2.1 for the Mac, open the zip file, and move the JES 3.2.1.app to your Applications folder.
Example 1: Playing Sound Files
- In the JES program area, type in the following main() function:
file = pickAFile()
sound = makeSound( file )
blockingPlay( sound )
- Save the file as sound1.py
- Click on Load Program
- In the interactive area, type
- When prompted for a sound file, select thisisatest.wav in the Sound Files folder.
- Make sure the speakers are on, but not too loud and listen to the sentence being played.
- Try one or two other sound files.
Part 3: Altering a sound file
- You will reduce the intensity of the sound by dividing all the samples by half
- Copy/Paste the program below in JES
- Read it to make sure you understand it.
# select a sound file
file = pickAFile()
sound = makeSound( file )
# play it
blockingPlay( sound )
# lower its intensity by half
for i in range( 1, getLength( sound ) ):
value = getSampleValueAt( sound, i ) # get the value of the i-th sample
setSampleValueAt( sound, i, value / 2 ) # set the sample to half its original value
# play new waveform back
blockingPlay( sound )
Part 4: Reversing a Sound File
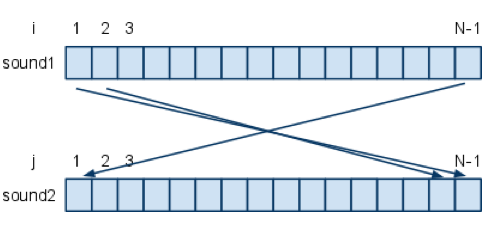
- The goal is to create 2 sound objects, sound1 and sound2 from the same file, and take the value of the sample at Index 1 in sound1 and to store it at Index N-1 in sound2. Identically for the sample at Index 2 which will go to Index N-2 in sound2.
- Complete the table below and write down the relationship existing between i and j:
i | j | i+j |
1 | ||
2 | ||
3 | ||
... | ||
N-2 | ||
N-1 |
- From the table above extract the equation that yields j as a function of i
- Write your for-loop to reverse sound1 into sound2
N = ... for i in range( 1, N ): j = ... sample = getSampleValueAt( sound1, i ) setSampleValueAt( sound2, j, sample )
- Test your program by making it play sound1 first, then sound2.
Part 9: ECHO Echo echo
Same as Part 8, but now you will make 2 copies of your sound object, attenuate the first one by 0.5, the second one by 0.25, and delay each by 5000 from each other, and create a reverberation effect.
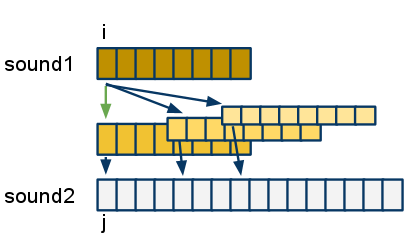
JES Sound Functions
This is just reference material taken from Depaw U. that you may want to play with.
A sound is treated as a collection of sample values, much as a picture is a collection of pixels. Here are the functions on sounds:
makeSound(file) | Return a sound loaded from a WAV, AU, or AIFF file |
makeEmptySound(sec) | Return a blank sound (all samples 0) of length sec seconds |
writeSoundTo(s, file) | Save s in the given file; uses the same format as when loaded (makeEmptySound creates a sound in WAV format) |
openSoundTool(s) | Open the sound exploration window for s |
play(s) | Play sound s in the background |
playAtRate(s, r) | Play s at speed r--1.0 is normal, 2.0 is twice as fast, etc. |
playInRange(s, b, e) | Play s from sample number b to e |
playAtRateInRange(s, r, b, e) | Play s from b to e at speed r |
blockingPlay(s) | Play s and wait for it to finish (also, blockingPlayInRange and blockingPlayAtRateInRange) |
getSamples(s) | Return the collection of samples in s (not quite a list, but indexable and usable in a for loop) |
getLength(s) | Return the number of samples in s |
getSamplingRate(s) | Return the number of samples per second in s |
There are also some useful methods on sound and sample objects:
s.getSampleValue(i) | Return the (left channel, if stereo) value of the ith sample in s (the first sample is at index 1) |
s.getLeftSampleValue(i) | Return the left channel value at index i |
s.getRightSampleValue(i) | Return the right channel value at index i |
s.setSampleValue(i, v) | Set the (left) value of sample i to v; the range of sample values is -32,768 to +32,767 |
s.setLeftSampleValue(i, v) | Same |
s.setRightSampleValue(i, v) | Guess what? |
s.getSampleObjectAt(i) | Extracts the sample object at index i, without creating a whole collection of samples |
samp.value | Return the value of sample samp; change the value by assigning to this |
samp.left | Return or change the left channel value of samp |
samp.right | Return or change the right channel value of samp |
Finally, JES provides a simple function to play a MIDI note:
playNote(n, dur, vol) | plays note number n (middle C is 60, C-sharp is 61, etc.; the range is 0-127) for dur milliseconds at volume vol (maximum is 127). |
The function pauses execution until the note is finished. For much more complete support of MIDI music, see the next section.