Difference between revisions of "Tutorial: Simple Drobox-Based Server for iPad/iPhone"
(→Source Code) |
(→Source Code) |
||
Line 63: | Line 63: | ||
</source> | </source> | ||
<br /> | <br /> | ||
− | The script is self explanatory. | + | The script is self explanatory. Make sure to replace the paths of '''inputDir''', '''outputDir''' and '''log''' with valid paths on your system. |
<br /> | <br /> | ||
Revision as of 13:36, 20 May 2014
--D. Thiebaut (talk) 12:12, 20 May 2014 (EDT)
The purpose of this tutorial is to setup a simple server to process photographs taken on a mobile device. Two devices must be linked by a shared Dropbox folder: a Mac running iOS and working as the server, and a mobile device on which the Dropbox app is installed. Whenever a new photo is deposited in the shared Dropbox folder on the mobile device, the image is replicated in the Mac's local Dropbox folder, and an iOS launchd process is automatically called to process the image and generate some new version of it. The resulting new version is automatically replicated in the Dropbox folder on the mobile device. Et voilà!
This type of setup can easily be modified to work in cases where programming in php/javascript is not possible or easy. The advantage of the method presented here is that the launchd script is run with the same privileges as the user, and has access to the the whole set of executable and file systems available to the logged in user.
Overview
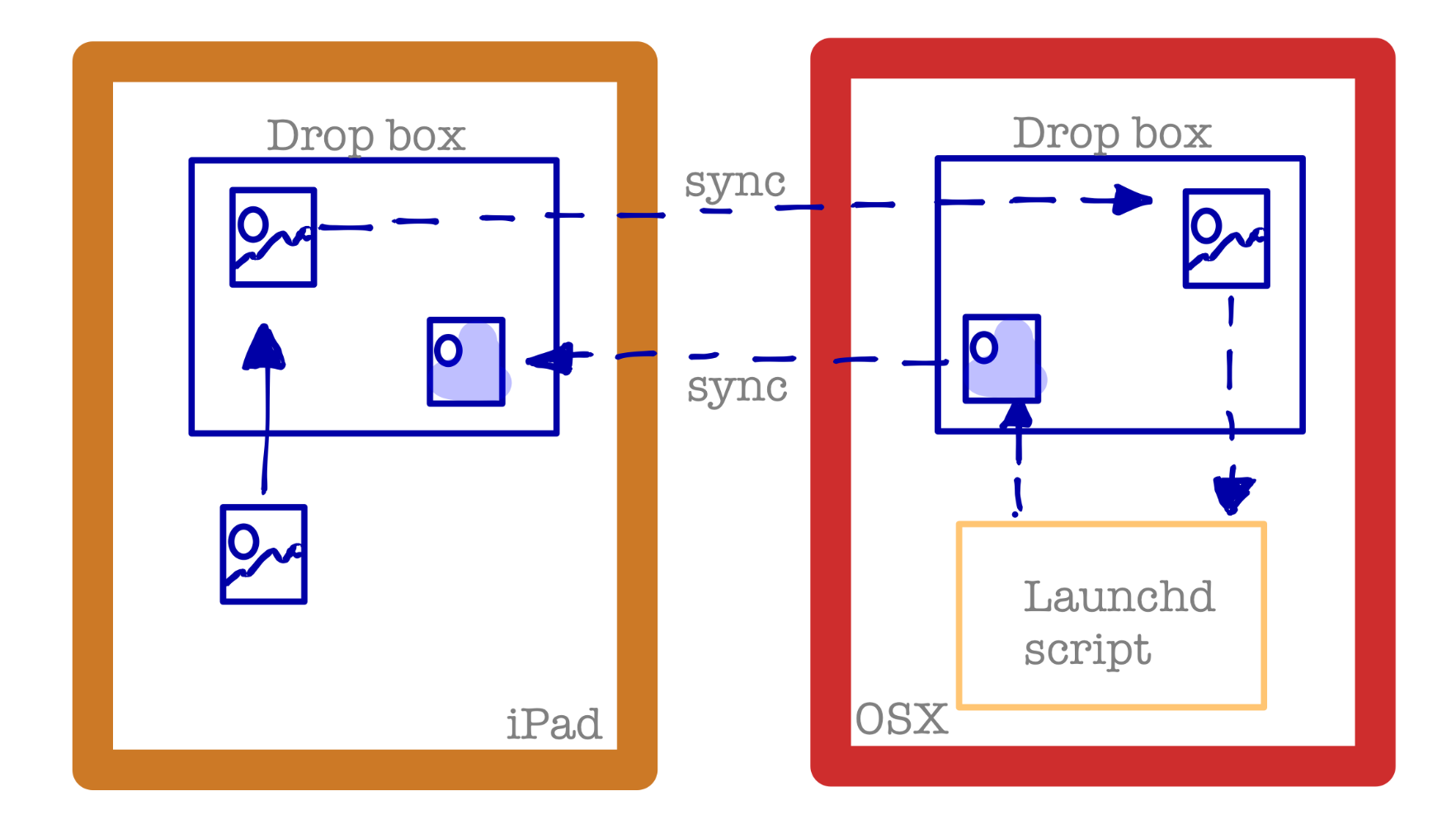
This is the scenario we want to implement
- we put a picture in a a Dropbox folder on our mobile device. Here we assume that the mobile device is an iPad but the idea would work on any mobile device where a Dropbox app is running.
- Dropbox automatically syncs the picture onto a Dropbox folder on an OSX computer. We assume that the same user is logged in the two devices, the iPad and the OSX computer, so that the two Dropbox folders are automatically synchronized.
- Once the file appears in the OSX computer, a launchd script discovers it and processes it in some way. For this tutorial we will simply generate a black and white version of it. This new image is automatically synchronized and replicated in the iPad's Dropbox folder.
Step 1: Create a Bash Script to Process the Image
The first step is create a regular bash script that checks for the presence of a file in a given Dropbox folder, say inputDir, and if found, creates a monochrome version that is stored in a different Dropbox folder, say outputDir. The original file is removed from inputDir to prevent an endless processing of the file.
Source Code
Here's a the script, below:
#! /bin/bash
# makeMonochrome.sh
# D. Thiebaut
# checks the Dropbox folder's iPad directory inputDir
# for an image file. When found, transforms it to
# black and white and saves the resulting version in
# outputDir
inputDir=/whereEverYourDropboxFolderIsLocated/Dropbox/inputDir
outputDir=/whereEverYourDropboxFolderIsLocated/Dropbox/outputDir
log=/WhereEverYouWantToSaveTheLog/log.txt
# if there's a file in the inputDir folder, process it, then remove it
if ls ${inputDir}/* &> /dev/null; then
date > $log
file=`ls -1 ${inputDir}/* | head -1`
basename=${file##*/}
newFile=${outputDir}/BW_${basename}
echo "file = $file" >> $log
echo "newFile = $newFile" >> $log
echo "convert $file -monochrome $newFile" >> $log
# transform to black and white
convert $file -monochrome $newFile
# remove the file from the inputDir
rm $file
echo "makeMonochrome.sh done!" >> $log
date >> $log
fi
The script is self explanatory. Make sure to replace the paths of inputDir, outputDir and log with valid paths on your system.
Testing
Make the script executable:
chmod +x makeMonochrome.sh
Then put a jpg or png file in the inputDir folder. Run the makeMonochrome.sh script from the command line. Verify that the result is a new file prefixed with BW_ in the outputDir folder of Dropbox.