Difference between revisions of "Simple Computer Simulator Instruction-Set"
(→Number-in-Memory Instructions) |
(→Instructions that use the Index Register to Access Data) |
||
(29 intermediate revisions by the same user not shown) | |||
Line 6: | Line 6: | ||
<bluebox> | <bluebox> | ||
This page documents all the instructions that are supported by the Simple Computer Simulator shown below. You can click on the image to go to the Javascript simulator. This simulator is used in the CSC103 How Computers Work course at Smith College. | This page documents all the instructions that are supported by the Simple Computer Simulator shown below. You can click on the image to go to the Javascript simulator. This simulator is used in the CSC103 How Computers Work course at Smith College. | ||
+ | <br /> | ||
+ | The instructions are presented in functional groups, rather than in logical ones, so that simple programs can be created with just the first 3 groups, allowing more programming sophistication as subsequent groups are explored. | ||
</bluebox> | </bluebox> | ||
<br /> | <br /> | ||
<center>[[Image:SimpleComputerSimulatorFace.png|600px|link=http://cs.smith.edu/dftwiki/media/simulator/]]<br />[http://cs.smith.edu/dftwiki/media/simulator/ Simple Computer Simulator]</center> | <center>[[Image:SimpleComputerSimulatorFace.png|600px|link=http://cs.smith.edu/dftwiki/media/simulator/]]<br />[http://cs.smith.edu/dftwiki/media/simulator/ Simple Computer Simulator]</center> | ||
<br /> | <br /> | ||
− | =Instructions Using a Number= | + | =Table Format= |
+ | <br /> | ||
+ | All the instructions supported by our Simple Computer Simulator are presented in tables below. The format of the table is explained below. | ||
+ | ;The Instruction | ||
+ | : The first column contains the instruction and the type of operand it supports. Some instructions have no operands (for example '''TXA''', '''TAX''', or '''HALT'''), some have a number, as in '''LOAD 3''', some a number in brackets, as in '''STORE [10]''', and some still have something different, as in '''LOAD [X]'''. | ||
+ | ; The Decimal Code | ||
+ | : All instructions are stored in memory in binary. Binary contains only 0s and 1s. Therefore anything in memory is a number. We use words to represent instructions, for example '''LOAD''' or '''STORE''', simply because it is much easier for us to remember words than numbers. But actually the language we are using is a code. Each instruction is associated with a number. This is the number shown in this column. We show it in decimal as it is the easiest system for humans to comprehend. <br />Assume that our program contains the instruction '''LOAD 7''', and that it is located at Address 0. When this instruction is translated (programmers say ''assembled'') into numbers so that it can be stored in memory, we see from the second table below that its decimal code is 4, and its binary code is 00000100. The memory will contain 2 numbers representing the instruction, a 4 at Address 0, and a 7 (00000111 in binary) at Address 1. The first number, at Address 0, is the '''code''', the second number, at Address 1, is the '''data'''.<br />Illustration of how the simulator will show '''LOAD 7''' in memory. | ||
+ | <br /> | ||
+ | <center>[[Image:SCS_Load7_decimal.png|300px]] [[Image:SCS_Load7_binary.png|300px]]</center> | ||
+ | <br /> | ||
+ | ; The Binary Code | ||
+ | : This number is simply the binary representation of the previous number. | ||
+ | ;Description | ||
+ | : Just a few words to help you understand what the instruction actually does, and how it uses its operands, if any. | ||
+ | <br /> | ||
+ | |||
+ | =An Instruction to end a Program = | ||
+ | <br /> | ||
+ | Every program must stop execution at some point. The way to do this is to have a special instruction that stops the execution. In our case, this instruction is '''HALT'''. In the simulator, the HALT forces animation to stop and prevents the ''Step'' button to operate. In real computers, there is an instruction similar to HALT that actually forces operating system to take over the computer, and remove the program from memory, making the space it was occupying available for other programs the user may want or need to load. | ||
+ | <br /> | ||
+ | {| class="wikitable" width="100%" | ||
+ | ! width="20%" | Instruction | ||
+ | ! width="15%" |Code<br />(decimal) | ||
+ | ! width="15%" |Code<br />( binary) | ||
+ | ! width="50%" |Description | ||
+ | |- | ||
+ | | | ||
+ | HALT | ||
+ | | | ||
+ | 127 | ||
+ | | | ||
+ | 01111111 | ||
+ | | | ||
+ | * This instruction forces the processor to stop when the program ends. A program should always have a HALT instruction. While it is most often found at the end of a program, it can also be found inside the program when sophisticated loops are used. | ||
+ | |} | ||
+ | <br /> | ||
+ | |||
+ | =Instructions Using the Accumulator and a Number= | ||
<br /> | <br /> | ||
These instructions operate with a single number (we refer to them as ''constants'') that is either loaded into, added, or subtracted from the accumulator register. | These instructions operate with a single number (we refer to them as ''constants'') that is either loaded into, added, or subtracted from the accumulator register. | ||
Line 29: | Line 68: | ||
| | | | ||
* This instruction adds the ''number'' to the one already in the accumulator. For example, if the accumulator register already contains 10, and the processor executes '''ADD ''3'' ''' the result is that the accumulator will contain ''13'' after the instruction. | * This instruction adds the ''number'' to the one already in the accumulator. For example, if the accumulator register already contains 10, and the processor executes '''ADD ''3'' ''' the result is that the accumulator will contain ''13'' after the instruction. | ||
− | + | ||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
|- | |- | ||
| | | | ||
Line 80: | Line 111: | ||
|} | |} | ||
<br /> | <br /> | ||
− | = | + | |
+ | = Instructions Using the Accumulator and Memory= | ||
<br /> | <br /> | ||
These instructions are followed by a number in brackets. This number refers to the location, or ''address'' in memory where the actual operand is located. So, if the number following the instruction is [''100''], it means that the instruction will use whatever number is stored at 100. A simple analogy might help here. Think of the difference between these two statements: | These instructions are followed by a number in brackets. This number refers to the location, or ''address'' in memory where the actual operand is located. So, if the number following the instruction is [''100''], it means that the instruction will use whatever number is stored at 100. A simple analogy might help here. Think of the difference between these two statements: | ||
Line 108: | Line 140: | ||
| | | | ||
* This instruction is similar to '''ADD ''number,'' ''' except that now the number to add to the accumulator is located in the memory at the address specified in the instruction. For example, if the memory cell at Address ''20'' contains ''4'', and if the accumulator contains ''10'', then the instruction '''ADD [20]''' adds 4 to 10, resulting in 14, which becomes the new contents of the accumulator. | * This instruction is similar to '''ADD ''number,'' ''' except that now the number to add to the accumulator is located in the memory at the address specified in the instruction. For example, if the memory cell at Address ''20'' contains ''4'', and if the accumulator contains ''10'', then the instruction '''ADD [20]''' adds 4 to 10, resulting in 14, which becomes the new contents of the accumulator. | ||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
|- | |- | ||
| | | | ||
Line 167: | Line 190: | ||
<br /> | <br /> | ||
− | == | + | =Instructions Manipulating the Index Register= |
+ | <br /> | ||
+ | The Index, '''IX''' in the simulator, is a register in the processor that contains numbers, just as the Accumulator does. However, the numbers in the index represent addresses of cells containing numbers. The Index is useful is situations where we have several numbers in consecutive memory locations, and we want to perform the same operation on each one, say add 1 to each of the numbers. In this case we store in the Index the address of the first number, say, 100, and operate on that number ''through the Index''. We'll need new instructions for that, but for right now we just want to see how we can load numbers in the index. | ||
<br /> | <br /> | ||
{| class="wikitable" width="100%" | {| class="wikitable" width="100%" | ||
Line 174: | Line 199: | ||
! width="15%" |Code<br />( binary) | ! width="15%" |Code<br />( binary) | ||
! width="50%" |Description | ! width="50%" |Description | ||
+ | |||
+ | <!-- ============================================================= --> | ||
|- | |- | ||
| | | | ||
− | ADDX | + | ADDX ''number'' |
| | | | ||
28 | 28 | ||
Line 182: | Line 209: | ||
00011100 | 00011100 | ||
| | | | ||
− | * | + | * This instruction adds a number to the contents of the Index register. For example, if the Index register contains the number 10, then '''ADDX ''1'' ''' will add 1 to 10, and the Index will contain 11 after the instruction is executed. |
|- | |- | ||
| | | | ||
− | + | ADDX [''address''] | |
| | | | ||
30 | 30 | ||
Line 191: | Line 218: | ||
00011110 | 00011110 | ||
| | | | ||
− | * | + | * This instruction is similar to the one above, except that the value that is added to the contents of IX comes from a memory cell whose address is given in the instruction. For example, if IX contains 10, and the memory cell at Address ''20'' contains 5, then '''ADDX [''20'']''' will result in replacing the contents of IX with 10+5, or 15. |
+ | |||
|- | |- | ||
| | | | ||
− | + | LOADX ''number'' | |
| | | | ||
− | + | 8 | |
| | | | ||
− | + | 00001000 | |
| | | | ||
− | * | + | * This instruction sets the contents of the Index to the number. For example, '''LOADX ''10'' ''' will result in the number 10 appearing inside the Index register. |
|- | |- | ||
| | | | ||
− | + | STOREX [''address''] | |
| | | | ||
− | + | 22 | |
| | | | ||
− | + | 00010110 | |
| | | | ||
− | * | + | * This instruction makes a copy of the contents of the Index register and saves it in a cell whose address is the one specified in the instruction. If IX contains 10, then the instruction '''STOREX [''20'']''' will copy the number 10 in the memory cell at Address 20. This does not affect the contents of IX. It does not get change by the copy operation. |
− | |||
|- | |- | ||
| | | | ||
− | + | SUBX ''number'' | |
| | | | ||
− | + | 36 | |
| | | | ||
− | + | 00100100 | |
| | | | ||
− | * | + | *This instruction subtracts a number from the contents of the Index register. For example, if the Index register contains the number 10, then '''SUBX ''1'' ''' will subtract 1 from 10, and the Index will contain 9 after the instruction is executed. |
+ | |- | ||
|- | |- | ||
| | | | ||
− | + | SUBX [''address''] | |
| | | | ||
− | + | 38 | |
| | | | ||
− | + | 00100110 | |
| | | | ||
− | * | + | * Similarly to the way ADDX [''address''], this instruction takes the quantity found in memory at the location specified by ''address'' and subtracts it from the contents of the IX register. |
+ | |} | ||
+ | |||
+ | <br /> | ||
+ | |||
+ | =The Jump instruction= | ||
+ | <br /> | ||
+ | The jump instruction forces the processor to continue executing instructions at the address specified in the instruction. For example, '''JUMP ''30'' ''' forces the processor to go to Address ''30'' and take the instruction it finds there as the new instruction to execute. It will then continue on with the executions that sequentially follow the one at Address 30. | ||
+ | |||
+ | {| class="wikitable" width="100%" | ||
+ | ! width="20%" | Instruction | ||
+ | ! width="15%" |Code<br />(decimal) | ||
+ | ! width="15%" |Code<br />( binary) | ||
+ | ! width="50%" |Description | ||
|- | |- | ||
| | | | ||
− | + | JUMP ''address'' | |
| | | | ||
− | + | 64 | |
| | | | ||
− | + | 01000000 | |
| | | | ||
− | * | + | * Forces the processor to continue execution at the location specified: ''address''. |
+ | |} | ||
+ | <br /> | ||
+ | = Compare and Jump-If Instructions = | ||
+ | <br /> | ||
+ | The compare and jump-If instructions operate together. We always want to use them together to test conditions and execute one sequence of instruction or another. When programming, when we want to test something we need to create two paths for the execution of the program. One path will be taken if the test is true (say, is the contents of the accumulator less than 10), and another path if it is false. | ||
+ | |||
+ | For example, imagine that we do not know if the number in the Accumulator contains a number greater than 100. If so, we'll want to stop the program, otherwise we'll want to continue with some more computation | ||
+ | |||
+ | ... | ||
+ | 10: COMP 100 | ||
+ | 12: JLT 16 | ||
+ | 14: HALT | ||
+ | 16: ''some more computation'' | ||
+ | ... | ||
+ | |||
+ | The instruction at Address 10 compares the contents of the accumulator to 100. Some information about this comparison is kept inside the processor. The next instruction, at Address 12, is '''JLT 16'''. Its behavior is to force the processor to ''jump'' to Address 16 '''if, and only if,''' the result of the previous comparison is true, i.e. the accumulator is less than 100. If the accumulator is actually less than 100, the processor will jump to Address 16 and execute some more instructions. Otherwise, if the accumulator contains a number equal to or greater than 100, then it simply does not jump. And since a processor always execute instuctions in sequence, it moves on to the next instruction which is '''HALT''' and which forces it to stop. | ||
+ | <br /> | ||
+ | {| class="wikitable" width="100%" | ||
+ | ! width="20%" | Instruction | ||
+ | ! width="15%" |Code<br />(decimal) | ||
+ | ! width="15%" |Code<br />( binary) | ||
+ | ! width="50%" |Description | ||
|- | |- | ||
| | | | ||
− | + | COMP ''number'' | |
+ | | | ||
+ | 84 | ||
| | | | ||
− | + | 01010100 | |
| | | | ||
− | + | * This instruction compares the ''number'' to the one already in the accumulator. For example, if the accumulator register already contains 10, and the processor executes '''COMP ''3'' ''' the result is the comparison of ''10'' to ''3''. ''10'' is greater, and is not equal to ''3''. This will prevent a '''JLT''' (jump if less than) to jump to its target, and will prevent a '''JEQ''' instruction from jumping. On the other hand, if the accumulator had contained ''2'', then a '''COMP ''2'' ''' would have allowed a subsequent '''JEQ''' instruction to jump to its target. | |
+ | |- | ||
| | | | ||
− | * | + | COMP [''address''] |
− | + | | | |
+ | 86 | ||
+ | | | ||
+ | 01010110 | ||
+ | | | ||
+ | * This instruction is similar to '''COMP ''number,'' ''' except that now the number which is compared to the accumulator is located in the memory at the address specified in the instruction. For example, if the memory cell at Address ''20'' contains ''4'', and if the accumulator contains ''10'', then the instruction '''COMP [20]''' compares 10 to 4. | ||
|- | |- | ||
| | | | ||
− | + | COMPX ''number'' | |
| | | | ||
− | + | 92 | |
| | | | ||
− | + | 01011100 | |
| | | | ||
− | * | + | * This instruction is similar to '''COMP ''number'' ''', except that the comparison is performed between IX and the ''number''. |
|- | |- | ||
| | | | ||
− | + | COMPX [''address''] | |
| | | | ||
− | + | 94 | |
| | | | ||
− | + | 01011110 | |
| | | | ||
− | * | + | * This instruction is similar to '''COMP [''address''] ''', except that the comparison is performed between IX and the number stored at the given ''address'' in memory. |
+ | |||
|- | |- | ||
| | | | ||
− | JEQ | + | JEQ ''address'' |
| | | | ||
68 | 68 | ||
Line 277: | Line 349: | ||
01000100 | 01000100 | ||
| | | | ||
− | * | + | * If the result of the previous comparison is that the two quantities were equal, then this instruction makes the processor ''jump'' to the address specified. If the result of the comparison is that the two quantities were different, the processor moves on to the next instruction in memory. |
|- | |- | ||
| | | | ||
− | JLT | + | JLT ''address'' |
| | | | ||
72 | 72 | ||
Line 286: | Line 358: | ||
01001000 | 01001000 | ||
| | | | ||
− | * | + | * If the result of the previous comparison is that the contents of the Accumulator is less than the quantity it is compared to, then this instruction makes the processor ''jump'' to the address specified. If the result of the comparison is that the value in the Accumulator is equal to, or greather than the second quantity, then the processor moves on to the next instruction in memory. |
+ | |||
+ | |} | ||
+ | <br /> | ||
+ | |||
+ | =Register-Exchange Instructions= | ||
+ | <br /> | ||
+ | These instructions allow the programmer to copy the contents of the Accumulator in the Index register, and vice versa. | ||
+ | |||
+ | {| class="wikitable" width="100%" | ||
+ | ! width="20%" | Instruction | ||
+ | ! width="15%" |Code<br />(decimal) | ||
+ | ! width="15%" |Code<br />( binary) | ||
+ | ! width="50%" |Description | ||
+ | |- | ||
|- | |- | ||
| | | | ||
− | + | TAX | |
| | | | ||
− | + | 79 | |
| | | | ||
− | + | 01001111 | |
| | | | ||
− | * | + | * Transfer Accumulator into Index. The contents of the Index register is erased and the value contained in the Accumulator is copied there. |
− | |||
|- | |- | ||
| | | | ||
− | + | TXA | |
| | | | ||
− | + | 83 | |
| | | | ||
− | + | 01010011 | |
| | | | ||
− | * | + | * Transfer Index to Accumulator. The contents of the Accumulator register is erased and the value contained in the Index is copied there. |
+ | |} | ||
+ | |||
+ | <br /> | ||
+ | |||
+ | |||
+ | |||
+ | <br /> | ||
+ | |||
+ | =Other Instructions= | ||
+ | <br /> | ||
+ | The instructions in this section are extra; they are instructions that are logical to have, but they are not necessary for writing programs for solving problems. They might provide for simpler solution, but we would have been able to write code with that would have performed just the same with the instructions presented above. None-the-less, you may be interested in exploring assembly language with the simulator some more, in which case you'll find that your ability to program will be fairly improved with the additions of the instructions below. | ||
+ | |||
+ | Some of the instructions use a new type of operand: "[X]". The instruction '''ADD [X]''' for example, adds a number to the contents of the Accumulator, but this number is found in memory at the address contained in the Index register. For example, if the Accumulator contains 10, and the Index register IX contains 30, and if at Address 30 we have the number 5, then '''ADD [X]''' will fetch 5 from address 30 that it gets from IX, add it to 10 that is in the Accumulator. The result of the addition is 30 + 10, or 40, and this number gets stored back in the Accumulator. | ||
+ | |||
+ | <br /> | ||
+ | {| class="wikitable" width="100%" | ||
+ | ! width="20%" | Instruction | ||
+ | ! width="15%" |Code<br />(decimal) | ||
+ | ! width="15%" |Code<br />( binary) | ||
+ | ! width="50%" |Description | ||
|- | |- | ||
| | | | ||
− | + | ADDX [X] | |
| | | | ||
− | + | 29 | |
| | | | ||
− | + | 00011101 | |
| | | | ||
− | * | + | * Fetches the number in memory whose address is currently in the Index register. This number is then added to the Index register. |
|- | |- | ||
| | | | ||
− | + | ADDX ''number'' | |
| | | | ||
− | + | 25 | |
| | | | ||
− | + | 00011001 | |
| | | | ||
− | * | + | * Adds ''number'' to the contents of the Index register. |
+ | |||
|- | |- | ||
| | | | ||
− | + | COMPX [X] | |
| | | | ||
− | + | 93 | |
| | | | ||
− | + | 01011101 | |
| | | | ||
− | * | + | * Compares the Index register to the value stored in memory at an address which is currently stored in the same Index register. |
|- | |- | ||
| | | | ||
− | + | DIVX ''number'' | |
| | | | ||
− | + | 45 | |
| | | | ||
− | + | 00101101 | |
− | | | + | | |
− | * | + | * Divides the contents of the Index register by ''number'' and keeps only the integer part (without decimals). This result replaces the old contents of the Index register. |
+ | |||
+ | |||
+ | |||
+ | |||
|- | |- | ||
| | | | ||
− | + | LOADX [''address''] | |
| | | | ||
− | + | 10 | |
| | | | ||
− | + | 00001010 | |
| | | | ||
− | * | + | * Fetches the data stored in memory at the location specified by ''address'' and copies it into the Index register. |
|- | |- | ||
| | | | ||
− | + | LOADX [X] | |
| | | | ||
− | + | 9 | |
| | | | ||
− | + | 00001001 | |
| | | | ||
− | * | + | * Fetches the number stored in memory at the address that is currently stored in the Index register, and copies this number in the Index register. |
+ | |||
|- | |- | ||
| | | | ||
− | + | LOADX ''number'' | |
| | | | ||
− | + | 5 | |
| | | | ||
− | + | 00000101 | |
| | | | ||
− | * | + | * Replaces the current contents of the Index register by ''number''. |
+ | |||
|- | |- | ||
| | | | ||
− | + | MULX ''number'' | |
| | | | ||
− | + | 41 | |
| | | | ||
− | + | 00101001 | |
| | | | ||
− | * | + | * Takes the contents of the Index register and multiplies it by ''number''. The result is then stored back in the Index register, replacing its original contents. |
+ | |||
|- | |- | ||
| | | | ||
− | + | STOREX [X] | |
| | | | ||
− | + | 21 | |
| | | | ||
− | + | 00010101 | |
| | | | ||
− | * | + | * Stores the contents of the Index register in memory at an address which is also the contents of the Index register. For example, if the Index register contains the value 40, then '''STOREX [X]''' will store the number 40 at Address 40 in memory. |
+ | |||
|- | |- | ||
| | | | ||
− | + | STOREX [''address''] | |
| | | | ||
− | + | 17 | |
| | | | ||
− | + | 00010001 | |
| | | | ||
− | * | + | * Copies the contents of the Index register in memory at a location specified by ''address''. |
+ | |||
+ | |||
+ | |||
|- | |- | ||
| | | | ||
− | + | SUBX [X] | |
| | | | ||
37 | 37 | ||
Line 409: | Line 526: | ||
00100101 | 00100101 | ||
| | | | ||
− | * | + | * Fetches the value stored in memory at the address specified in the Index register and subtracts that number from the Index Register. |
|- | |- | ||
| | | | ||
− | + | SUBX ''number'' | |
| | | | ||
33 | 33 | ||
Line 419: | Line 536: | ||
00100001 | 00100001 | ||
| | | | ||
− | * | + | * Subtracts ''number'' from the value stored in the Index register. Replaces the contents of the Index register with the result of the subtraction. |
+ | |||
+ | |- | ||
+ | |} | ||
+ | <br /> | ||
+ | |||
+ | =Miscellaneous Instructions= | ||
+ | <br /> | ||
+ | Most processor supports an instruction that doesn't do anything. It is often used for padding programs. | ||
+ | <br /> | ||
+ | {| class="wikitable" width="100%" | ||
+ | ! width="20%" | Instruction | ||
+ | ! width="15%" |Code<br />(decimal) | ||
+ | ! width="15%" |Code<br />( binary) | ||
+ | ! width="50%" |Description | ||
|- | |- | ||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
|- | |- | ||
| | | | ||
− | + | NOP | |
| | | | ||
− | + | 0 | |
| | | | ||
− | + | 00000000 | |
| | | | ||
− | * | + | * This instruction simply makes the processor go to the next instruction. No register is modified except the Program Counter (PC). |
− | |||
|} | |} | ||
+ | |||
+ | <br /> | ||
+ | <br /><br /><br /><br /><br /><br /> | ||
+ | <br /><br /><br /><br /><br /><br /><br /> | ||
+ | [[Category:CSC103]] |
Latest revision as of 11:14, 31 August 2014
--D. Thiebaut (talk) 16:57, 26 August 2014 (EDT)
Contents
- 1 Table Format
- 2 An Instruction to end a Program
- 3 Instructions Using the Accumulator and a Number
- 4 Instructions Using the Accumulator and Memory
- 5 Instructions Manipulating the Index Register
- 6 The Jump instruction
- 7 Compare and Jump-If Instructions
- 8 Register-Exchange Instructions
- 9 Other Instructions
- 10 Miscellaneous Instructions
This page documents all the instructions that are supported by the Simple Computer Simulator shown below. You can click on the image to go to the Javascript simulator. This simulator is used in the CSC103 How Computers Work course at Smith College.
The instructions are presented in functional groups, rather than in logical ones, so that simple programs can be created with just the first 3 groups, allowing more programming sophistication as subsequent groups are explored.
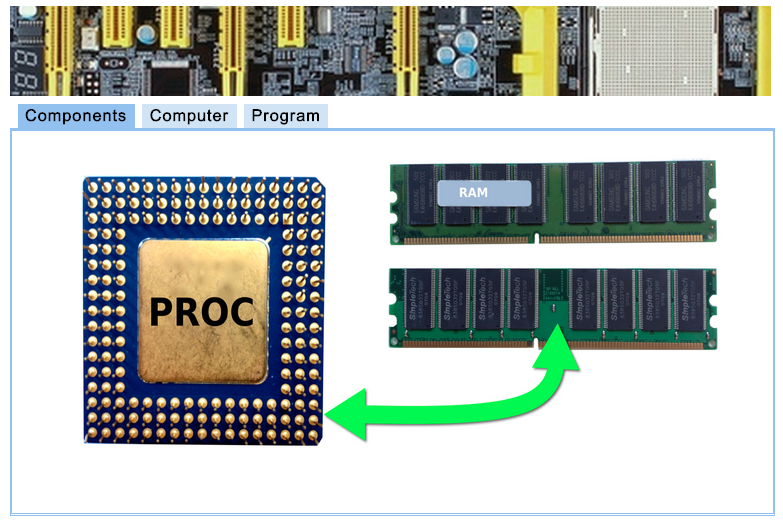
Simple Computer Simulator
Table Format
All the instructions supported by our Simple Computer Simulator are presented in tables below. The format of the table is explained below.
- The Instruction
- The first column contains the instruction and the type of operand it supports. Some instructions have no operands (for example TXA, TAX, or HALT), some have a number, as in LOAD 3, some a number in brackets, as in STORE [10], and some still have something different, as in LOAD [X].
- The Decimal Code
- All instructions are stored in memory in binary. Binary contains only 0s and 1s. Therefore anything in memory is a number. We use words to represent instructions, for example LOAD or STORE, simply because it is much easier for us to remember words than numbers. But actually the language we are using is a code. Each instruction is associated with a number. This is the number shown in this column. We show it in decimal as it is the easiest system for humans to comprehend.
Assume that our program contains the instruction LOAD 7, and that it is located at Address 0. When this instruction is translated (programmers say assembled) into numbers so that it can be stored in memory, we see from the second table below that its decimal code is 4, and its binary code is 00000100. The memory will contain 2 numbers representing the instruction, a 4 at Address 0, and a 7 (00000111 in binary) at Address 1. The first number, at Address 0, is the code, the second number, at Address 1, is the data.
Illustration of how the simulator will show LOAD 7 in memory.
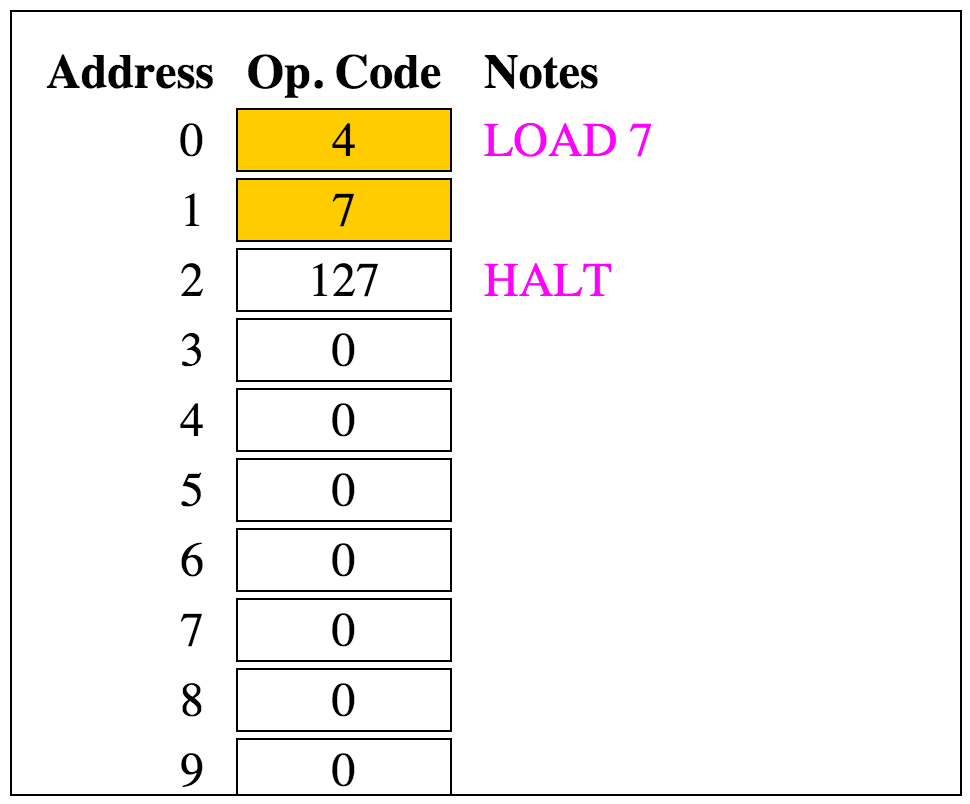
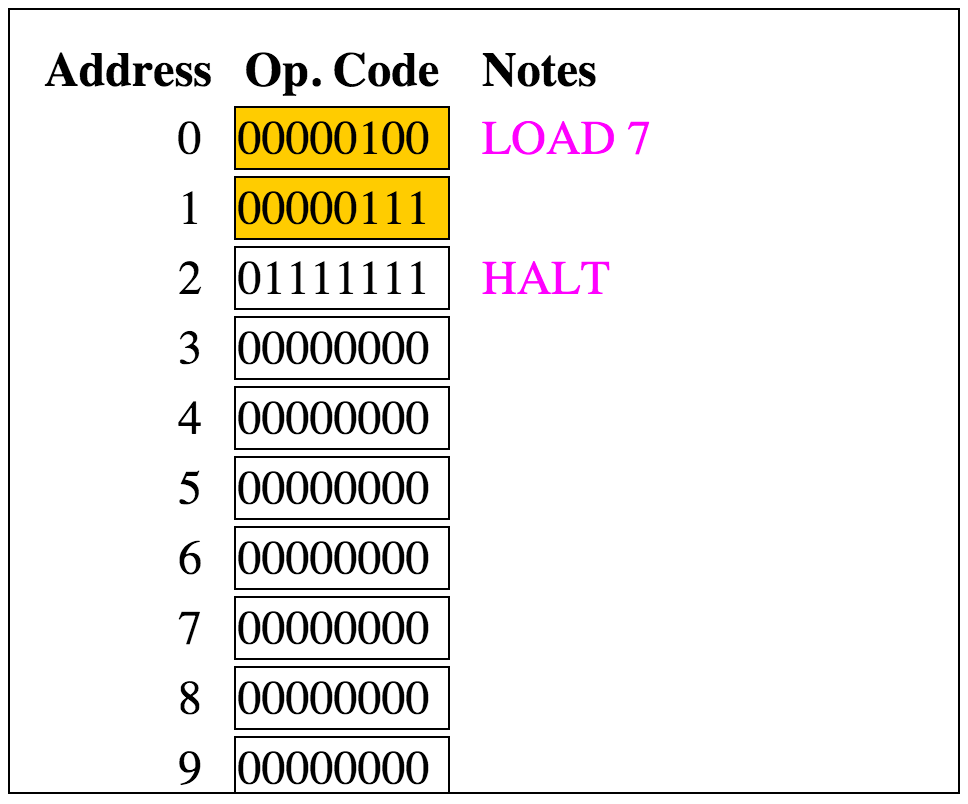
- The Binary Code
- This number is simply the binary representation of the previous number.
- Description
- Just a few words to help you understand what the instruction actually does, and how it uses its operands, if any.
An Instruction to end a Program
Every program must stop execution at some point. The way to do this is to have a special instruction that stops the execution. In our case, this instruction is HALT. In the simulator, the HALT forces animation to stop and prevents the Step button to operate. In real computers, there is an instruction similar to HALT that actually forces operating system to take over the computer, and remove the program from memory, making the space it was occupying available for other programs the user may want or need to load.
Instruction | Code (decimal) |
Code ( binary) |
Description |
---|---|---|---|
HALT |
127 |
01111111 |
|
Instructions Using the Accumulator and a Number
These instructions operate with a single number (we refer to them as constants) that is either loaded into, added, or subtracted from the accumulator register.
Instruction | Code (decimal) |
Code ( binary) |
Description |
---|---|---|---|
ADD number |
24 |
00011000 |
|
DIV number |
44 |
00101100 |
|
LOAD number |
4 |
00000100 |
|
MUL number |
40 |
00101000 |
|
SUB number |
32 |
00100000 |
|
Instructions Using the Accumulator and Memory
These instructions are followed by a number in brackets. This number refers to the location, or address in memory where the actual operand is located. So, if the number following the instruction is [100], it means that the instruction will use whatever number is stored at 100. A simple analogy might help here. Think of the difference between these two statements:
- "Please read the book The Little Prince."
and the statement
- "Please read the book from the library, with Call Number PQ2637.A274 P4613 2000".
Both statements refer to reading the same book. The first one refers to the book directly. The instructions in the section above operate similarly with their operands. The second statement refers to the book indirectly, by giving you its address in the library. The instructions in this section perform the same way. By putting brackets around the numbers that follow the instructions, we indicate that the numbers used are not the actual numbers we want to combine with the accumulator, but the address of the cells where we will find the numbers of interest.
This may still be a bit obscure, but read on the description for each instruction and this will hopefully become a bit clearer.
Instruction | Code (decimal) |
Code ( binary) |
Description |
---|---|---|---|
ADD [address] |
26 |
00011010 |
|
DIV [address] |
46 |
00101110 |
|
LOAD [address] |
6 |
00000110 |
|
MUL [address] |
42 |
00101010 |
|
STORE [address] |
18 |
00010010 |
|
SUB [address] |
34 |
00100010 |
|
Instructions Manipulating the Index Register
The Index, IX in the simulator, is a register in the processor that contains numbers, just as the Accumulator does. However, the numbers in the index represent addresses of cells containing numbers. The Index is useful is situations where we have several numbers in consecutive memory locations, and we want to perform the same operation on each one, say add 1 to each of the numbers. In this case we store in the Index the address of the first number, say, 100, and operate on that number through the Index. We'll need new instructions for that, but for right now we just want to see how we can load numbers in the index.
Instruction | Code (decimal) |
Code ( binary) |
Description |
---|---|---|---|
ADDX number |
28 |
00011100 |
|
ADDX [address] |
30 |
00011110 |
|
LOADX number |
8 |
00001000 |
|
STOREX [address] |
22 |
00010110 |
|
SUBX number |
36 |
00100100 |
|
SUBX [address] |
38 |
00100110 |
|
The Jump instruction
The jump instruction forces the processor to continue executing instructions at the address specified in the instruction. For example, JUMP 30 forces the processor to go to Address 30 and take the instruction it finds there as the new instruction to execute. It will then continue on with the executions that sequentially follow the one at Address 30.
Instruction | Code (decimal) |
Code ( binary) |
Description |
---|---|---|---|
JUMP address |
64 |
01000000 |
|
Compare and Jump-If Instructions
The compare and jump-If instructions operate together. We always want to use them together to test conditions and execute one sequence of instruction or another. When programming, when we want to test something we need to create two paths for the execution of the program. One path will be taken if the test is true (say, is the contents of the accumulator less than 10), and another path if it is false.
For example, imagine that we do not know if the number in the Accumulator contains a number greater than 100. If so, we'll want to stop the program, otherwise we'll want to continue with some more computation
... 10: COMP 100 12: JLT 16 14: HALT 16: some more computation ...
The instruction at Address 10 compares the contents of the accumulator to 100. Some information about this comparison is kept inside the processor. The next instruction, at Address 12, is JLT 16. Its behavior is to force the processor to jump to Address 16 if, and only if, the result of the previous comparison is true, i.e. the accumulator is less than 100. If the accumulator is actually less than 100, the processor will jump to Address 16 and execute some more instructions. Otherwise, if the accumulator contains a number equal to or greater than 100, then it simply does not jump. And since a processor always execute instuctions in sequence, it moves on to the next instruction which is HALT and which forces it to stop.
Instruction | Code (decimal) |
Code ( binary) |
Description |
---|---|---|---|
COMP number |
84 |
01010100 |
|
COMP [address] |
86 |
01010110 |
|
COMPX number |
92 |
01011100 |
|
COMPX [address] |
94 |
01011110 |
|
JEQ address |
68 |
01000100 |
|
JLT address |
72 |
01001000 |
|
Register-Exchange Instructions
These instructions allow the programmer to copy the contents of the Accumulator in the Index register, and vice versa.
Instruction | Code (decimal) |
Code ( binary) |
Description |
---|---|---|---|
TAX |
79 |
01001111 |
|
TXA |
83 |
01010011 |
|
Other Instructions
The instructions in this section are extra; they are instructions that are logical to have, but they are not necessary for writing programs for solving problems. They might provide for simpler solution, but we would have been able to write code with that would have performed just the same with the instructions presented above. None-the-less, you may be interested in exploring assembly language with the simulator some more, in which case you'll find that your ability to program will be fairly improved with the additions of the instructions below.
Some of the instructions use a new type of operand: "[X]". The instruction ADD [X] for example, adds a number to the contents of the Accumulator, but this number is found in memory at the address contained in the Index register. For example, if the Accumulator contains 10, and the Index register IX contains 30, and if at Address 30 we have the number 5, then ADD [X] will fetch 5 from address 30 that it gets from IX, add it to 10 that is in the Accumulator. The result of the addition is 30 + 10, or 40, and this number gets stored back in the Accumulator.
Instruction | Code (decimal) |
Code ( binary) |
Description |
---|---|---|---|
ADDX [X] |
29 |
00011101 |
|
ADDX number |
25 |
00011001 |
|
COMPX [X] |
93 |
01011101 |
|
DIVX number |
45 |
00101101 |
|
LOADX [address] |
10 |
00001010 |
|
LOADX [X] |
9 |
00001001 |
|
LOADX number |
5 |
00000101 |
|
MULX number |
41 |
00101001 |
|
STOREX [X] |
21 |
00010101 |
|
STOREX [address] |
17 |
00010001 |
|
SUBX [X] |
37 |
00100101 |
|
SUBX number |
33 |
00100001 |
|
Miscellaneous Instructions
Most processor supports an instruction that doesn't do anything. It is often used for padding programs.
Instruction | Code (decimal) |
Code ( binary) |
Description |
---|---|---|---|
NOP |
0 |
00000000 |
|