Difference between revisions of "CSC212 Lab 7b 2014"
(→Create a new Java File) |
(→Create a new Java File) |
||
Line 94: | Line 94: | ||
<br /> | <br /> | ||
:* '''Finish''' | :* '''Finish''' | ||
+ | <br /> | ||
+ | <center>[[Image:CSC212_Ecplise5.png|500px]]</center> | ||
+ | <br /> |
Revision as of 20:30, 8 October 2014
--D. Thiebaut (talk) 20:56, 8 October 2014 (EDT)
This section of the lab assumes that you have installed Eclipse on your system. If not, then use one of the Linux Mint machines in the lab. For the purpose of discovering Eclipse and its amazing features, we will develop a program using the top-down approach.
Contents
Your Lab Assignment
Write a program that reads a collection of lines from the standard input (either from the keyboard or redirected from a file). The lines contain a list of file names along with their size, expressed in Kilobytes. Here's an example of the type of input your program will receive:
./ArtOfAssembly 10012 ./ArtOfAssembly/CH01 276 ./ArtOfAssembly/CH02 300 ./ArtOfAssembly/CH03 480 ./ArtOfAssembly/CH04 272 ./ArtOfAssembly/CH05 328 ./ArtOfAssembly/CH06 620 ./ArtOfAssembly/CH07 148 ./ArtOfAssembly/CH08 748 ./ArtOfAssembly/CH09 436 ./ArtOfAssembly/CH10 248 ./ArtOfAssembly/CH11 436 ./ArtOfAssembly/CH12 348 ./ArtOfAssembly/CH13 524 ./ArtOfAssembly/CH14 500 ./ArtOfAssembly/CH14/org_gifs 72 ./ArtOfAssembly/CH15 380 ./ArtOfAssembly/CH16 772 ./ArtOfAssembly/CH16/chars 32 ./ArtOfAssembly/CH17 248 ./ArtOfAssembly/CH18 304 ./ArtOfAssembly/CH19 612 ./ArtOfAssembly/CH20 348 ./ArtOfAssembly/CH21 200 ./ArtOfAssembly/CH22 196 ./ArtOfAssembly/CH23 60 ./ArtOfAssembly/CH24 352 ./ArtOfAssembly/CH25 256 ./ArtOfAssembly/chars 16 ./ArtOfAssembly/fwd 68
The last line indicates that the file fwd in the directory ArtOfAssembly is 68 KBytes in size.
The Assignment is to keep track of all the files that are larger than 100 KBytes and store them in a list for later processing (for example because we may want to compress them).
Your program will print the list of the large files (>100K) at the end of the program.
Top Level
Think for a minute about what your program needs to do:
1. get the input, one line at a time, 2. extract the size from the line. 3. if the size is larger than 100, add the file and its size to a list 4. once the input has been completely read, output the list of large files
Launch Eclipse
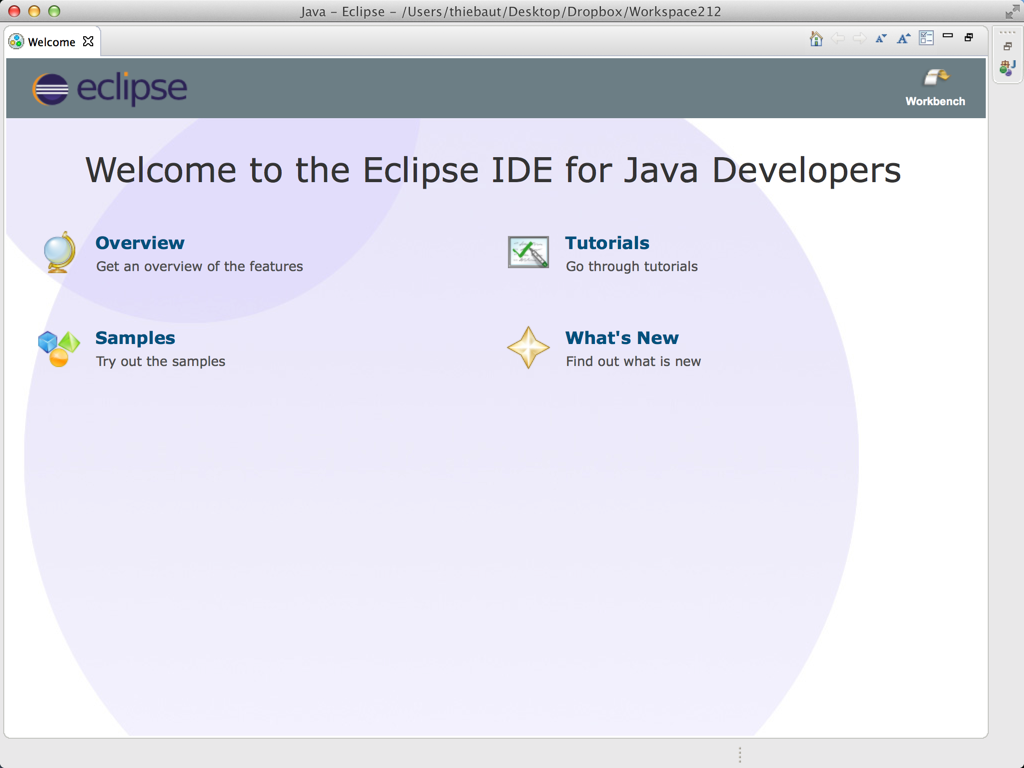
- Close the Welcome Window
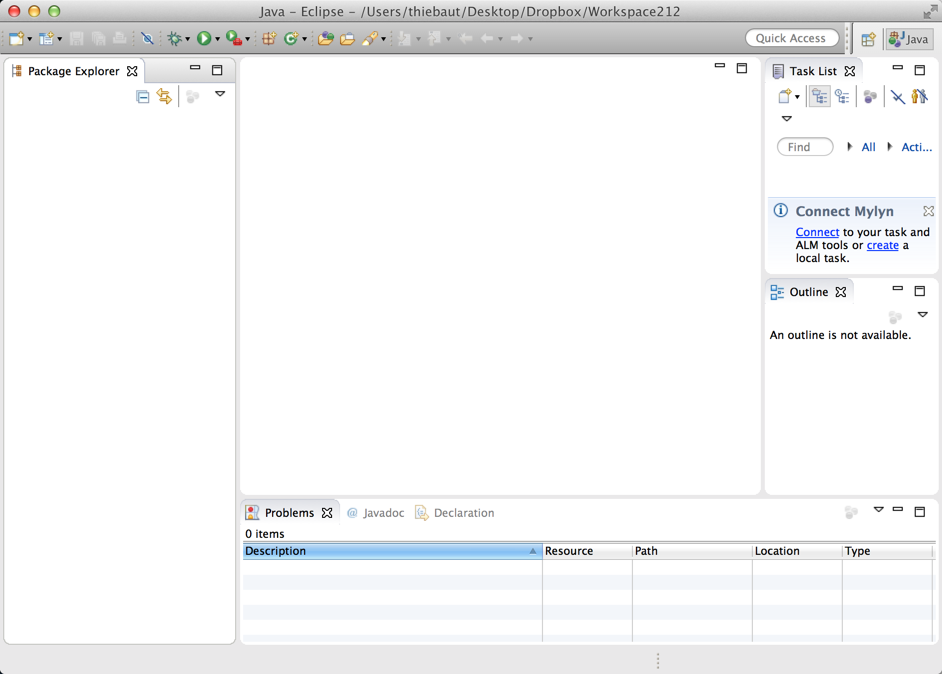
Create A Project
- Create a new Project. Eclipse organizes Java programs into project. Let's create a project called CSC212 where you'll store your Java file(s). You should have to do this only once this semester (unless you want to organize your files by, say, homework or lab sections).
- File
- New
- Java Project
- CSC212
- Finish
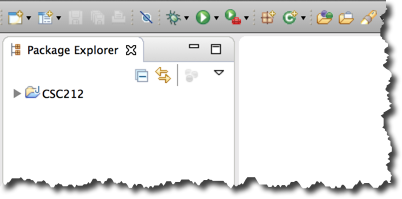
Create a new Java File
- Select CSC212
- Right-Click or Control-Click on it, depending on your type of machine, and pick
- File
- New
- Class
- Lab7a (don't add the .java extension)
- Click on public static void main( String[] args )
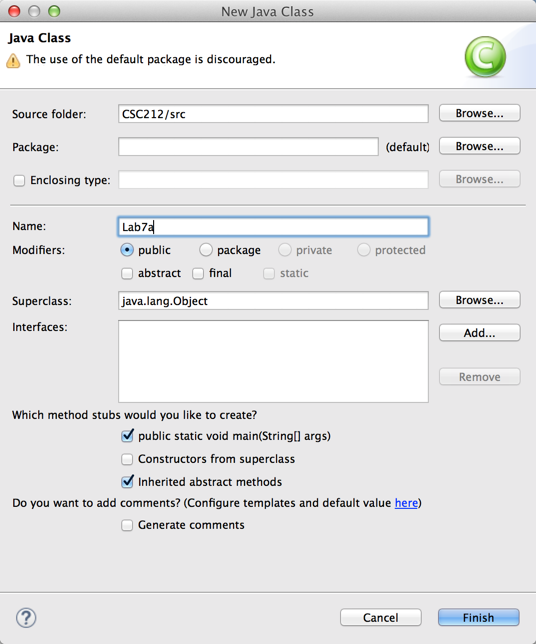
- Finish
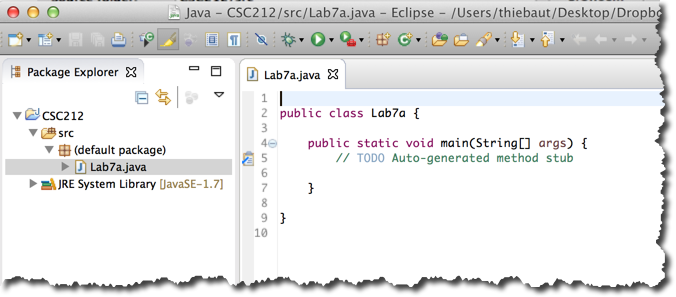