Difference between revisions of "CSC111 Lab 9 2015"
(→preparation) |
|||
Line 41: | Line 41: | ||
* Modify your function and add code that will '''catch the exception''': | * Modify your function and add code that will '''catch the exception''': | ||
<br /> | <br /> | ||
− | ::<source lang="python" highlight=" | + | ::<source lang="python" highlight="9-14"> |
− | # | + | # betterGetInput: returns an integer larger |
− | # than 0. | + | # than 0. Catches Value errors |
− | def | + | def betterGetInput(): |
− | + | ||
− | while | + | # repeat forever... |
− | + | while True: | |
− | + | ||
− | + | # try to get an int | |
− | + | try: | |
− | + | x = int( input( "Enter an integer greater than 0: " ) ) | |
+ | except ValueError: | ||
+ | # the user must have entered something other than an int | ||
+ | print( "Invalid entry. Not an integer. Try again!" ) | ||
+ | continue | ||
+ | |||
+ | # There was no errors. See if the number is negative | ||
+ | if x <= 0: | ||
+ | print( "You entered a negative number. Try again!" ) | ||
+ | else: | ||
+ | return x | ||
</source> | </source> | ||
<!-- /showafterdate --> | <!-- /showafterdate --> |
Revision as of 06:31, 29 March 2015
--D. Thiebaut (talk) 07:14, 29 March 2015 (EDT)
Exceptions
preparation
- Create a new program called lab9_1.py, and copy this code to the new Idle window.
# lab9_1.py # Your name here # getInput: returns an integer larger # than 0. Expected to be robust def getInput(): while True: x = int( input( "Enter an integer greater than 0: " ) ) if x <= 0: print( "Invalid entry. Try again!" ) else: return x def main(): num = getInput() print( "You have entered", num ) main()
- Test it with numbers such as -3, -10, 0, 5. Verify that the input function works well when you enter numbers.
- Test your program again, and this time enter expressions such as "6.3", or "hello" (without the quotes).
- Make a note of the Error reported by Python:
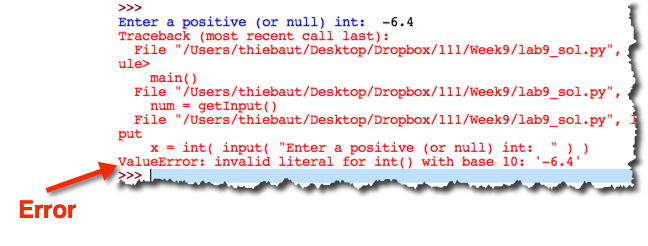
- Modify your function and add code that will catch the exception:
# betterGetInput: returns an integer larger # than 0. Catches Value errors def betterGetInput(): # repeat forever... while True: # try to get an int try: x = int( input( "Enter an integer greater than 0: " ) ) except ValueError: # the user must have entered something other than an int print( "Invalid entry. Not an integer. Try again!" ) continue # There was no errors. See if the number is negative if x <= 0: print( "You entered a negative number. Try again!" ) else: return x