Difference between revisions of "CSC111 Homework 7 2015b"
Line 9: | Line 9: | ||
=Problem #1= | =Problem #1= | ||
<br /> | <br /> | ||
− | [[RockPaperScissors.png| right|150px]] | + | [[Image:RockPaperScissors.png| right|150px]] |
Write a program called hw7_1.py that prompts the user for the name of a text file containing the plays selected by two users playing a game of [https://en.wikipedia.org/wiki/Rock-paper-scissors Rock-Scissors-Paper]. | Write a program called hw7_1.py that prompts the user for the name of a text file containing the plays selected by two users playing a game of [https://en.wikipedia.org/wiki/Rock-paper-scissors Rock-Scissors-Paper]. | ||
Revision as of 19:12, 21 October 2015
--D. Thiebaut (talk) 22:07, 20 October 2015 (EDT)
<showafterdate after="20151022 17:00" before="20151231 00:00">
This homework is due on Thursday 10/29/2015 at 11:55 p.m..
Contents
Problem #1
Write a program called hw7_1.py that prompts the user for the name of a text file containing the plays selected by two users playing a game of Rock-Scissors-Paper.
make sure your program contains a main() function that is called at the end of your program and that runs the whole program.
Here is an example of the possible contents of the text file:
RR RS SS PS PR PP PR SS
Each line must contain 2 letters, without any spaces between them. There are only 2 letters per line. Each letter is guaranteed to be R, P, or S. Nothing else will be found in the file. There might be space before or after the 2 letters, though, and the letters can be upper- or lower-case.
Your program will read the file and output only 1 line containing 1 number. This number will either be 1, 2, or 0. 1 will indicate that Player 1 (the first letter on the line) wins, 2 will indicate that Player 2 (the second letter) wins, and 0 will indicate a tie.
In the example above, we have the following: 4 ties, Player 1 wins 3 times, and Player 2 wins once. The output of your program should be
1
since Player 1 has won.
Submit your program to Moodle, Section HW 8 PB 1. You will be able to evaluate this program yourself.
Problem 2
Same setup as Problem 1. Your program should be called hw7_2.py and should contain a main() function. This time your program will stop processing the information in a text file (which is also called plays.txt) as soon as the difference in points between Player1 and Player2 is 3.
For example, assume the file contains:
RS RS RS SR SR SR SR SR SR SR SR SS
Your program will output 1 because the first 3 lines correspond to 3 plays when Player 1 wins. After your program has processed the letters from the 3rd line of the file, the counter associated with Player 1 will be 3 and that of Player 2 will be 0. The difference is 3, and your program will stop, indicating that Player 1 has won. You see that the file contains additional lines that would make Player 2 wins in the end, but we are only interested in the case where the difference between Player 1 and Player 2 reaches 3 points.
You can assume that the file will always contain enough lines to create a difference of 3 points between the two players.
Once again, make sure your program only outputs one of three possible numbers: 1, 2, or 0.
Submit your program to Moodle, Section HW 8 PB 2. You will be able to evaluate your program.
Problem 3
Your program will be called hw7_3.py and should contain a main() function.
It is similar to the solution for Problem 2, but this time the text file may contain invalid lines. An invalid line is a line that does not contain 2 letters only, that are each, either R, P, or S, upper or lower case.
Examples of invalid lines:
RRR R S 12 R:S R-S
Examples of valid lines:
RR RS rs SS sP
Your program will stop when the difference in points between Player 1 and Player 2 is 3. There will always be enough lines with the right plays in the text file to make the difference in points reach 3.
Submit your program on Moodle in the HW 8 PB 3 section. Evaluation will turned off for this program. (Remember, we're slowly removing your training wheels!)
Problem 4
Write a program called hw7_4.py, that contains a main() function. It should be similar to the program for Problem 3, but you need to modify the rules of the game to include a new "figure": the Hole. The hole is made by putting one's hand in the shape of a cylinder.
The new rules with the hole are as follows:
- R H: Hole wins, as Rock falls in Hole
- H H: Tie
- S H: Hole wins, as Scissors fall in Hole
- H P: Paper wins, as paper covers Hole
You will have noticed that the new rules are unfair. Holes wins more often than it loses. That's ok for this game.
The text file, still called plays.txt, will now contain text lines, some valid, some invalid, where the valid lines contain only 2 characters, upper or lower case, each either 'R', 'P', 'S', or 'H'.
Make your program stop when
- the difference in points between Player 1 and Player 2 is 3, or
- or when the text file has been exhausted, and there are no more lines to read. In this case, the difference between the two players' points could be 0, 1, 2 or 3.
The output of your program is different from the previous programs. Your program should now output 2 numbers, on the same line, separated by a space. The first number is 1 if Player 1 wins, 2 if Player 2 wins, and 0 if it's a tie. The second number is the number of plays they had to go through to get a difference of 3 points. For example:
1 10
would indicate that Player 1 wins after 10 rounds.
0 1000
would indicate that the players played for 1000 rounds, and finished with a tie. Because they finish with a difference in point that is less than 3, 1000 must be the number of valid lines in the text file.
Submit your program on Moodle, in the HW 8 PB 4 section. You will not be able to evaluate this program. Make sure you test it thoroughly.
Here are special cases your program should be able to handle.
- the text file is empty or contains only invalid lines. In this case the output should be 0 0
- the text file contains 3 valid lines, and Player 1 (conversely Player 2) wins on all three lines. The output in this case should be 1 3.
- the text file contains 10000 lines of the form RR. The output should be 0 10000.
Problem 5
- Write a graphics program that produces a 600x600 window similar to this one.
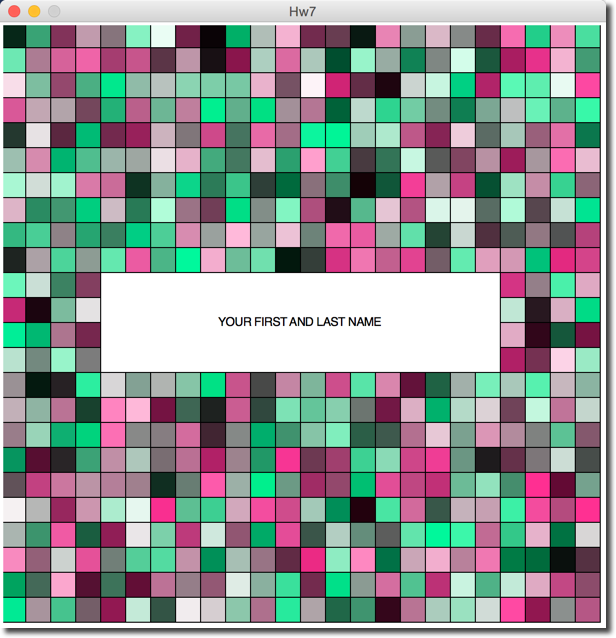
- The squares should be 25x25 pixels in width and height. Their color should be random.
- Your name should appear in a white box centered in the middle of the window.
- Store your program in a file called hw7_5.py and submit it in the HW7 PB 5 PROGRAM section. You won't be able to evaluate this program, as Moodle can't automatically grade graphics program. Your program will be graded by hand.
- Submit a screen capture of the window generated by your program. Submit it in the HW7 PB5 IMAGE section. Note: Programs submitted without a screen capture will automatically be given a grade of 0.
- Hints: you may want to play with this simple program before you start:
for i in range( 0, 5 ): for j in range( 0, 5 ): print( i, j )
</showafterdate>
<showafterdate after="20151030 00:00" before="20151231 00:00">
Solution Programs
Problem 1
# hw7_1.py Rock Paper Scissors
# D. Thiebaut
# Solution program for Homework #8
# This program reads plays between two players from a text file.
# Each line in the text file contains 2 letters that are either R, P, or S.
# The first letter indicates the play by the first player, and the second
# letter the play by the second player.
# The program computes the winner of all the plays stored in the text
# file and outputs 1 if it's Player 1 (the first letter), 2 if it's Player 2 (the
# second letter, or 0 in case of a tie.
#
PLAYER1WINS= 1
PLAYER2WINS= 2
TIE = 0
FILENAME = "plays.txt"
# pickALetter: prompts the user for a letter, and doe not return
# until it is valid.
# playRound: plays a round between PLAYER1 and PLAYER2.
# returns the winner of the round (PLAYER1 or PLAYER2)
def playRound( player1, player2 ):
if player1==player2:
#print( "It's a tie!" )
return TIE
if ( player1=="P" and player2=="S" ) \
or ( player1=="R" and player2=="P" ) \
or ( player1=="S" and player2=="R" ):
#print( "I win this round!" )
return PLAYER2
#print( "You win this round!" )
return PLAYER1
# playRound: plays a round between PLAYER2 and PLAYER1. PLAYER1
# picks a letter, PLAYER2 picks a letter at random.
# displays outcome of the game. Returns winner
def playRound( p1, p2 ):
# checks for ties
if p1 == p2:
return TIE
# look at conditions where PLAYER2 wins
if (p1=="P" and p2=="S") \
or (p1=="R" and p2=="P") \
or (p1=="S" and p2=="R"):
return PLAYER2WINS
# PLAYER1 must have won
return PLAYER1WINS
# readFile: reads the contents of the file and returns it
# as a list of lines.
def readFile():
list = []
file = open( FILENAME, "r" )
lines = file.readlines()
file.close()
# remove the "\n" at the end of each line
# and make sure all characters are upper case
plays = []
for line in lines:
plays.append( line.strip().upper() )
return plays
# main: plays several rounds of the rock-paper-scissors game
# until one player gets 3 more points than the other. Then
# displays the winner.
def main():
# counters for winning rounds
player1Count = 0
player2Count = 0
# read the text file with all the plays
plays = readFile()
for play in plays:
player1 = play[0]
player2 = play[1]
winner = playRound( player1, player2 )
if winner == PLAYER1WINS:
player1Count += 1
if winner == PLAYER2WINS:
player2Count += 1
# print 1 if player1 wins, 2 if player2 wins,
# or 0 if tie
if player1Count > player2Count:
print( 1 )
elif player1Count < player2Count:
print( 2 )
else:
print( 0 )
main()
Problem 2
# hw7_2.py Rock Paper Scissors
# D. Thiebaut
# Solution program for Homework #8 Problem 2.
# This program reads plays between two players from a text file.
# Each line in the text file contains 2 letters that are either R, P, or S.
# The first letter indicates the play by the first player, and the second
# letter the play by the second player.
# The program computes the winner who is the player to get 3 points
# over the score of the other one. The plays are taken from the same
# text file as hw7_1.py.
# The program outputs 1 if Player 1 (the first letter) wins, 2 if it's Player 2 (the
# second letter, or 0 in case of a tie (but that shouldn't happen).
#
PLAYER1WINS= 1
PLAYER2WINS= 2
TIE = 0
FILENAME = "plays.txt"
# pickALetter: prompts the user for a letter, and doe not return
# until it is valid.
# playRound: plays a round between PLAYER1 and PLAYER2.
# returns the winner of the round (PLAYER1 or PLAYER2)
def playRound( player1, player2 ):
if player1==player2:
#print( "It's a tie!" )
return TIE
if ( player1=="P" and player2=="S" ) \
or ( player1=="R" and player2=="P" ) \
or ( player1=="S" and player2=="R" ):
#print( "I win this round!" )
return PLAYER2
#print( "You win this round!" )
return PLAYER1
# playRound: plays a round between PLAYER2 and PLAYER1. PLAYER1
# picks a letter, PLAYER2 picks a letter at random.
# displays outcome of the game. Returns winner
def playRound( p1, p2 ):
# checks for ties
if p1 == p2:
return TIE
# look at conditions where PLAYER2 wins
if (p1=="P" and p2=="S") \
or (p1=="R" and p2=="P") \
or (p1=="S" and p2=="R"):
return PLAYER2WINS
# PLAYER1 must have won
return PLAYER1WINS
# readFile: reads the contents of the file and returns it
# as a list of lines.
def readFile():
list = []
file = open( FILENAME, "r" )
lines = file.readlines()
file.close()
# remove the "\n" at the end of each line
# and make sure all characters are upper case
plays = []
for line in lines:
plays.append( line.strip().upper() )
return plays
# main: plays several rounds of the rock-paper-scissors game
# until one player gets 3 more points than the other. Then
# displays the winner.
def main():
# counters for winning rounds
player1Count = 0
player2Count = 0
# read the text file with all the plays
plays = readFile()
for play in plays:
player1 = play[0]
player2 = play[1]
winner = playRound( player1, player2 )
if winner == PLAYER1WINS:
player1Count += 1
if winner == PLAYER2WINS:
player2Count += 1
# stop if one player gets 3 points over the other's score.
if abs( player1Count - player2Count ) >= 3:
break
# print 1 if player1 wins, 2 if player2 wins,
# or 0 if tie
if player1Count > player2Count:
print( 1 )
elif player1Count < player2Count:
print( 2 )
else:
print( 0 )
main()
Problem 3
# hw7_3.py Rock Paper Scissors
# D. Thiebaut
# Solution program for Homework #8 Problem 3.
# This program reads plays between two players from a text file which
# may contain invalid lines.
# Each line in the text file contains 2 letters that are either R, P, or S.
# The first letter indicates the play by the first player, and the second
# letter the play by the second player.
# The program computes the winner of all the plays stored in the text
# file and outputs 1 if it's Player 1 (the first letter), 2 if it's Player 2 (the
# second letter, or 0 in case of a tie.
#
PLAYER1WINS= 1
PLAYER2WINS= 2
TIE = 0
FILENAME = "plays.txt"
# pickALetter: prompts the user for a letter, and doe not return
# until it is valid.
# playRound: plays a round between PLAYER1 and PLAYER2.
# returns the winner of the round (PLAYER1 or PLAYER2)
def playRound( player1, player2 ):
if player1==player2:
#print( "It's a tie!" )
return TIE
if ( player1=="P" and player2=="S" ) \
or ( player1=="R" and player2=="P" ) \
or ( player1=="S" and player2=="R" ):
#print( "I win this round!" )
return PLAYER2
#print( "You win this round!" )
return PLAYER1
# playRound: plays a round between PLAYER2 and PLAYER1. PLAYER1
# picks a letter, PLAYER2 picks a letter at random.
# displays outcome of the game. Returns winner
def playRound( p1, p2 ):
# checks for ties
if p1 == p2:
return TIE
# look at conditions where PLAYER2 wins
if (p1=="P" and p2=="S") \
or (p1=="R" and p2=="P") \
or (p1=="S" and p2=="R"):
return PLAYER2WINS
# PLAYER1 must have won
return PLAYER1WINS
# readFile: reads the contents of the file and returns it
# as a list of lines.
def readFile():
list = []
file = open( FILENAME, "r" )
lines = file.readlines()
file.close()
# remove the "\n" at the end of each line
# and make sure all characters are upper case
plays = []
for line in lines:
# strip the line and make it uppercase
line = line.strip().upper()
# reject lines that do not have 2 characters
if len( line ) != 2:
continue
# test both characters and reject if not R, P, or S.
for i in range( 2 ):
if not line[i] in ["R", "P", "S" ]:
continue
# otherwise the line is good
plays.append( line )
# return the good lines
return plays
# main: plays several rounds of the rock-paper-scissors game
# until one player gets 3 more points than the other. Then
# displays the winner.
def main():
# counters for winning rounds
player1Count = 0
player2Count = 0
# read the text file with all the plays
plays = readFile()
for play in plays:
player1 = play[0]
player2 = play[1]
winner = playRound( player1, player2 )
if winner == PLAYER1WINS:
player1Count += 1
if winner == PLAYER2WINS:
player2Count += 1
# stop if one player gets 3 points over the other's score.
if abs( player1Count - player2Count ) >= 3:
break
# print 1 if player1 wins, 2 if player2 wins,
# or 0 if tie
if player1Count > player2Count:
print( 1 )
elif player1Count < player2Count:
print( 2 )
else:
print( 0 )
main()
Problem 4
# hw7_4.py Rock Paper Scissors
# D. Thiebaut
# Solution program for Homework #8 Problem 3.
# This program reads plays between two players from a text file which
# may contain invalid lines.
# Each line in the text file contains 2 letters that are either R, P, H, or S.
# The first letter indicates the play by the first player, and the second
# letter the play by the second player.
# The rules are the rules of Rock-Paper-Scissors, augmented with the
# following conditions:
#
# R H: Hole wins, as Rock falls in Hole
# H H: Tie
# S H: Hole wins, as Scissors fall in Hole
# H P: Paper wins, as paper covers Hole
#
# The program computes the winner of all the plays stored in the text
# file and outputs 1 if it's Player 1 (the first letter), 2 if it's Player 2 (the
# second letter, or 0 in case of a tie.
#
PLAYER1WINS= 1
PLAYER2WINS= 2
TIE = 0
FILENAME = "plays.txt"
# pickALetter: prompts the user for a letter, and doe not return
# until it is valid.
# playRound: plays a round between PLAYER1 and PLAYER2.
# returns the winner of the round (PLAYER1 or PLAYER2)
def playRound( player1, player2 ):
if player1==player2:
#print( "It's a tie!" )
return TIE
if ( player1=="P" and player2=="S" ) \
or ( player1=="R" and player2=="P" ) \
or ( player1=="S" and player2=="R" ) \
or ( player1=="R" and player2=="H" ) \
or ( player1=="S" and player2=="H" ):
#print( "I win this round!" )
return PLAYER2WINS
#print( "You win this round!" )
return PLAYER1WINS
# readFile: reads the contents of the file and returns it
# as a list of lines.
def readFile():
list = []
file = open( FILENAME, "r" )
lines = file.readlines()
file.close()
# remove the "\n" at the end of each line
# and make sure all characters are upper case
plays = []
for line in lines:
# strip the line and make it uppercase
line = line.strip().upper()
# reject lines that do not have 2 characters
if len( line ) != 2:
continue
# test both characters and reject if not R, P, or S.
for i in range( 2 ):
if not line[i] in ["R", "P", "S", "H" ]:
continue
# otherwise the line is good
plays.append( line )
# return the good lines
return plays
# main: plays several rounds of the rock-paper-scissors game
# until one player gets 3 more points than the other. Then
# displays the winner.
def main():
# counters for winning rounds
player1Count = 0
player2Count = 0
# read the text file with all the plays
plays = readFile()
#print( plays )
# keep track of number of plays
count = 0
for play in plays:
count += 1
player1 = play[0]
player2 = play[1]
winner = playRound( player1, player2 )
if winner == PLAYER1WINS:
player1Count += 1
if winner == PLAYER2WINS:
player2Count += 1
#print( player1, player2, player1Count, player2Count, count )
# stop if one player gets 3 points over the other's score.
if abs( player1Count - player2Count ) >= 3:
break
# print 1 if player1 wins, 2 if player2 wins,
# or 0 if tie
if player1Count > player2Count:
print( 1, count )
elif player1Count < player2Count:
print( 2, count )
else:
print( 0, count )
main()
</showafterdate>