Difference between revisions of "CSC270 Homework 5"
(New page: This homework assignment is due on 3/11/09, at 7:00 pm. =Problem #1= Implement a GYR-light sequencer that is located on Elm St to allow students to cross. The setup is the following....) |
(→Problem #2) |
||
(3 intermediate revisions by the same user not shown) | |||
Line 8: | Line 8: | ||
The setup is the following. | The setup is the following. | ||
− | On both sides of the street we have a switch that can be ON or OFF. When the two switches are OFF, the lights go GREEN, YELLOW, RED, GREEN, YELLOW, RED, etc at a regular frequency, one time unit for each state. | + | * On both sides of the street we have a switch that can be ON or OFF. When the two switches are both ON, or both OFF, the lights go GREEN, YELLOW, RED, GREEN, YELLOW, RED, etc at a regular frequency, one time unit for each state. |
− | When a student wants to cross the street, she flips one of the switches | + | * When a student wants to cross the street, she flips one of the switches. So now we have one switch ON, one switch OFF. As soon as the lights reach the RED state, the sequencer keeps outputting RED, and stays that way as long as the switches are different. |
− | The student crosses the street, safe that the traffic will stop at the red light. | + | * The student crosses the street, safe in the knowledge that the traffic will stop at the red light. |
− | When she reaches the other side of the street, she flips the other switch | + | * When she reaches the other side of the street, she flips the other switch, so now both switches are the same. The sequencer resumes its GREEN, YELLOW, RED cycle. |
− | In summary: when both switches are the same, the sequencer cycles through all 3 colors. When the switches are different, the sequencer stays in red as | + | In summary: when both switches are the same, the sequencer cycles through all 3 colors. When the switches are different, the sequencer continues whatever it was doing until it reaches red, and then stays in red as long as the switches remain different. When the switches are both the same again, the sequencer moves on to Green and the cycle continues. |
Implement this circuit with D flipflops. | Implement this circuit with D flipflops. | ||
Line 26: | Line 26: | ||
Note: there are several ways to simulate the 2 switches. One way is to define ahead of time what their values will be at each step of the simulation. | Note: there are several ways to simulate the 2 switches. One way is to define ahead of time what their values will be at each step of the simulation. | ||
− | For example, | + | For example, below is a python program simulating the D-flipflop and XOR gate of a Lab 4: |
+ | <br /> | ||
+ | <center>[[Image:CSC270 controllableOscillator.png]]</center> | ||
+ | <br /> | ||
+ | |||
+ | <code><pre> | ||
+ | Q = 0 | ||
+ | cmdValues = [0,0,0,0,0,0,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0] | ||
+ | |||
+ | for cmd in cmdValues: | ||
+ | |||
+ | D = Q ^ cmd | ||
+ | |||
+ | print "cmd: %d Q: %d" % ( cmd, Q ) | ||
+ | |||
+ | # tick! | ||
+ | Q = D | ||
+ | |||
+ | </pre></code> | ||
+ | |||
+ | =Problem #3= | ||
+ | |||
+ | Implement a 3-bit counter that counts down with JK flip-flops. By counting down I mean the output goes 111, 110, 101, 100, 011, 010, 001, 000, 111, etc. | ||
+ | |||
+ | =Problem #4= | ||
+ | |||
+ | Submit the python code that verifies that your answer for Problem #3 is correct. |
Latest revision as of 14:05, 4 March 2009
This homework assignment is due on 3/11/09, at 7:00 pm.
Contents
Problem #1
Implement a GYR-light sequencer that is located on Elm St to allow students to cross.
The setup is the following.
- On both sides of the street we have a switch that can be ON or OFF. When the two switches are both ON, or both OFF, the lights go GREEN, YELLOW, RED, GREEN, YELLOW, RED, etc at a regular frequency, one time unit for each state.
- When a student wants to cross the street, she flips one of the switches. So now we have one switch ON, one switch OFF. As soon as the lights reach the RED state, the sequencer keeps outputting RED, and stays that way as long as the switches are different.
- The student crosses the street, safe in the knowledge that the traffic will stop at the red light.
- When she reaches the other side of the street, she flips the other switch, so now both switches are the same. The sequencer resumes its GREEN, YELLOW, RED cycle.
In summary: when both switches are the same, the sequencer cycles through all 3 colors. When the switches are different, the sequencer continues whatever it was doing until it reaches red, and then stays in red as long as the switches remain different. When the switches are both the same again, the sequencer moves on to Green and the cycle continues.
Implement this circuit with D flipflops.
Problem #2
Code your solution for Problem #1 in Python. Submit the code and a sample of its output.
Note: there are several ways to simulate the 2 switches. One way is to define ahead of time what their values will be at each step of the simulation.
For example, below is a python program simulating the D-flipflop and XOR gate of a Lab 4:
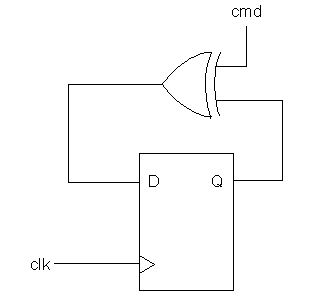
Q = 0
cmdValues = [0,0,0,0,0,0,1,1,1,1,1,1,1,1,0,0,0,0,0,0,0]
for cmd in cmdValues:
D = Q ^ cmd
print "cmd: %d Q: %d" % ( cmd, Q )
# tick!
Q = D
Problem #3
Implement a 3-bit counter that counts down with JK flip-flops. By counting down I mean the output goes 111, 110, 101, 100, 011, 010, 001, 000, 111, etc.
Problem #4
Submit the python code that verifies that your answer for Problem #3 is correct.