Difference between revisions of "CSC111 Homework 9 2011"
(→Problem #2: Elevator to the Stars) |
|||
(23 intermediate revisions by the same user not shown) | |||
Line 3: | Line 3: | ||
__TOC__ | __TOC__ | ||
<br /> | <br /> | ||
− | < | + | |
− | < | + | <bluebox>This assignment is due Tuesday night, 11/22, at midnight. You can work on this assignment in pair-programming mode. |
− | <br | + | </bluebox> |
− | </ | + | <br /> |
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
− | =Problem #1= | + | =Problem #1: Average Presidency= |
* Write a program called hw9a.py that outputs the average length of the presidency of US presidents, categorized by party. Your program should get the information from a file called '''presidents.txt''', whose contents is available [[CSC111 presidents.txt| here]]. | * Write a program called hw9a.py that outputs the average length of the presidency of US presidents, categorized by party. Your program should get the information from a file called '''presidents.txt''', whose contents is available [[CSC111 presidents.txt| here]]. | ||
Line 52: | Line 55: | ||
:if you worked on beowulf, or submit your program using this page: http://cs.smith.edu/~111a/submit9.htm | :if you worked on beowulf, or submit your program using this page: http://cs.smith.edu/~111a/submit9.htm | ||
− | =Problem #2= | + | =Problem #2: Elevator to the Stars= |
+ | <center>[[Image:spaceElevator.jpg]]</center> | ||
− | |||
− | |||
<P> | <P> | ||
Line 72: | Line 74: | ||
</pre></code> | </pre></code> | ||
− | * We have a text file called ''' | + | * We have a text file called '''candidates.txt''', and this file contains lines of text, as illustrated above. |
− | * Each line represents a person. The first string is that person's name (or initials), followed by a space, followed by a number representing that person's weight, in pounds. | + | * Each line represents a person. The first string is that person's name (or initials), followed by a space, followed by a number representing that person's weight, in pounds. Any other line that doesn't follow this format is invalid. |
− | * This list is the list of the first people who have signed up to use Google's first elevator to the moon when it becomes available (Check this [http://www.nytimes.com/2011/11/14/technology/at-google-x-a-top-secret-lab-dreaming-up-the-future.html NYT article] to see | + | * This list is actually the list of the first people who have signed up to use Google's first elevator to the moon when it becomes available (Check this [http://www.nytimes.com/2011/11/14/technology/at-google-x-a-top-secret-lab-dreaming-up-the-future.html NYT article] to see what '''Google X''' is up to!) |
− | * The only problem is that | + | * The only problem is that there is a maximum weight allowed, and that we may have to prevent some people from boarding. The maximum weight allowed is input by the user, as illustrated below (the user input is underlined): |
− | * Our only criterion for boarding the elevator will be | + | >>> |
+ | Google X Elevator | ||
+ | ____ _ __ __ | ||
+ | / ___| ___ ___ __ _| | ___ \ \/ / | ||
+ | | | _ / _ \ / _ \ / _` | |/ _ \ \ / | ||
+ | | |_| | (_) | (_) | (_| | | __/ / \ | ||
+ | \____|\___/ \___/ \__, |_|\___| /_/\_\ | ||
+ | |___/ | ||
+ | |||
+ | Candidates for first boarding of elevator: | ||
+ | JT (150 lbs) | ||
+ | TJ (80 lbs) | ||
+ | TT (120 lbs) | ||
+ | BT (170 lbs) | ||
+ | Mike (12 lbs) | ||
+ | AF (27 lbs) | ||
+ | Lea (78 lbs) | ||
+ | Leo (180 lbs) | ||
+ | AI (55 lbs) | ||
+ | GK (110 lbs) | ||
+ | |||
+ | Max weight? <u>2 hundred</u> | ||
+ | Invalid weight. Please try again. | ||
+ | Max weight? <u>infinite</u> | ||
+ | Invalid weight. Please try again. | ||
+ | Max weight? <u>-10</u> | ||
+ | Invalid weight. Please try again. | ||
+ | Max weight? <u>200</u> | ||
+ | 4 people can board the elevator with a weight limit of 200 lbs. | ||
+ | Their combined weight is 172 lbs. | ||
+ | The selected group includes: AF, AI, Lea, Mike | ||
+ | The rejected group includes: BT, GK, JT, Leo, TJ, TT | ||
+ | |||
+ | * Our only criterion for boarding the elevator will be the weight of the candidates. | ||
− | Write a program called '''hw9b.py''' that will | + | ==Your Assignment== |
+ | Write a program called '''hw9b.py''' that will read the file '''candidates.txt''', get a maximum allowed weight for the user, and output the answers to the following questions: | ||
;Question 1 | ;Question 1 | ||
− | :What is the maximum number of people that can fit in the elevator? | + | :What is the '''maximum''' number of people that can fit in the elevator? |
;Question 2 | ;Question 2 | ||
− | :What is the total weight of the people who fit in the elevator? | + | :What is the '''total weight''' of the people who fit in the elevator? |
;Question 3 | ;Question 3 | ||
− | :What are the names of the people | + | :What are the '''names''' of the lucky selected people allowed to board the elevator? |
;Question 4 | ;Question 4 | ||
− | :What are the names of the people who will have to wait? | + | :What are the '''names''' of the people who will have to wait and go in the second elevator? |
+ | |||
+ | * Use while or for loops, as you see fit. | ||
+ | |||
+ | * Make sure your program is robust! (It could be that the file does not exist, or that a line contains more than two words, or just one word, or is empty. It is also possible for a weight to be a real number, e.g. 180.5, which is acceptable. ) | ||
+ | |||
+ | * Make sure your program works for any valid maximum weight provided by the user. A weight of 0 is valid. Any positive number is valid (integer or real). | ||
+ | |||
+ | |||
+ | ==Optional and Extra Credit (+2/3 points)== | ||
+ | |||
+ | * Make your program output the list of candidates into batches, as illustrated below: | ||
+ | |||
+ | <code><pre> | ||
+ | >>> | ||
+ | Google X Elevator | ||
+ | ____ _ __ __ | ||
+ | / ___| ___ ___ __ _| | ___ \ \/ / | ||
+ | | | _ / _ \ / _ \ / _` | |/ _ \ \ / | ||
+ | | |_| | (_) | (_) | (_| | | __/ / \ | ||
+ | \____|\___/ \___/ \__, |_|\___| /_/\_\ | ||
+ | |___/ | ||
+ | |||
+ | |||
+ | Candidates for first boarding of elevator: | ||
+ | JT (150 lbs) | ||
+ | TJ (80 lbs) | ||
+ | TT (120 lbs) | ||
+ | BT (170 lbs) | ||
+ | Mike (12 lbs) | ||
+ | AF (27 lbs) | ||
+ | Lea (78 lbs) | ||
+ | Leo (180 lbs) | ||
+ | AI (55 lbs) | ||
+ | GK (110 lbs) | ||
+ | |||
+ | Max weight? 200 | ||
+ | |||
+ | --- BATCH 1 --- | ||
+ | 4 people can board the elevator with a weight limit of 200 lbs. | ||
+ | Their combined weight is 172 | ||
+ | The selected group includes: AF, AI, Lea, Mike | ||
+ | The rejected group includes: BT, GK, JT, Leo, TJ, TT | ||
+ | |||
+ | --- BATCH 2 --- | ||
+ | 2 people can board the elevator with a weight limit of 200 lbs. | ||
+ | Their combined weight is 190 | ||
+ | The selected group includes: GK, TJ | ||
+ | The rejected group includes: BT, JT, Leo, TT | ||
+ | |||
+ | --- BATCH 3 --- | ||
+ | 1 person can board the elevator with a weight limit of 200 lbs. | ||
+ | Their combined weight is 120 | ||
+ | The selected group includes: TT | ||
+ | The rejected group includes: BT, JT, Leo | ||
+ | |||
+ | --- BATCH 4 --- | ||
+ | 1 person can board the elevator with a weight limit of 200 lbs. | ||
+ | Their combined weight is 150 | ||
+ | The selected group includes: JT | ||
+ | The rejected group includes: BT, Leo | ||
+ | |||
+ | --- BATCH 5 --- | ||
+ | 1 person can board the elevator with a weight limit of 200 lbs. | ||
+ | Their combined weight is 170 | ||
+ | The selected group includes: BT | ||
+ | The rejected group includes: Leo | ||
+ | |||
+ | --- BATCH 6 --- | ||
+ | 1 person can board the elevator with a weight limit of 200 lbs. | ||
+ | Their combined weight is 180 | ||
+ | The selected group includes: Leo | ||
+ | The rejected group includes: | ||
+ | </pre></code> | ||
+ | |||
+ | * Note that we try to send as many people up as possible, and as early as possible. In other words, the program '''always''' maximizes the number of people it can send up. | ||
+ | * You do not need to store your file under a different name if you do this optional part. | ||
+ | * Make sure your program stops if it cannot let the last people in the group enter the elevator (it could be that the weight of some people is larger than the maximum allowed weight). | ||
+ | |||
+ | ==Submission== | ||
+ | * Submit your program as follows: | ||
+ | |||
+ | rsubmit hw9 hw9b.py | ||
+ | |||
+ | :if you worked on beowulf, submit your program using this page: http://cs.smith.edu/~111a/submit9.htm | ||
+ | : | ||
+ | <br /> | ||
+ | <center>[[Image:elevatorOutOfOrder.jpg|300px]]</center> | ||
+ | |||
+ | <br /> | ||
+ | |||
+ | <br /> | ||
+ | |||
+ | <br /> | ||
+ | |||
+ | <br /> | ||
− | + | <br /> | |
− | + | <br /> | |
− | + | <br /> | |
− | + | <br /> | |
+ | [[Category:CSC111]][[Category:Homework]][[Category:Python]] |
Latest revision as of 16:39, 15 November 2011
--D. Thiebaut 12:28, 13 November 2011 (EST)
Contents
This assignment is due Tuesday night, 11/22, at midnight. You can work on this assignment in pair-programming mode.
Problem #1: Average Presidency
- Write a program called hw9a.py that outputs the average length of the presidency of US presidents, categorized by party. Your program should get the information from a file called presidents.txt, whose contents is available here.
- If you are working on beowulf, you can get a copy of the file into your account this way:
getcopy presidents.txt
- Note that this file does not have triple double-quotes around the list of presidents, and that it does have a header, i.e. the first line defines what the different fields are, but is not a valid president line.
- You will get the contents of the file using a statement of the form
file = open( "presidents.txt", "r" )
Requirements
- Your program should use functions. Study Homework 7 a second time to get inspiration for your program organization.
- Your program will assume that if a president is incumbent, his time is the current year (2011) minus the time he came to office. So, for Obama, this would be 2011-2009 = 2 years. Don't use the number 2. Make your program compute the current year minus whatever the year in office is. This way your program can work for future presidents.
- To get the current year in Python, you need to import the datetime module, as illustrated below. now.year is an integer.
import datetime now = datetime.datetime.now() currentYear = now.year print( "The current year is", currentYear )
- The output of your program should be only a few lines long. We do not want to see the list of presidents, but just something like this:
Average length of presidency. File presidents.txt contains 44 presidents. Democratic presidents: x.xx years Republican presidents: x.xx years Others: x.xx years
- The number of presidents (44) should be the number found by your program in the text file. When I test your program, I may provide a presidents.txt file with fewer than 44 presidents. Or a file that has just a header and no presidents at all! Or even a file with nothing in it, just empty. Be sure you test your program well so that it doesn't crash on any of these potential input files.
Submission
- Submit your program as follows:
rsubmit hw9 hw9a.py
- if you worked on beowulf, or submit your program using this page: http://cs.smith.edu/~111a/submit9.htm
Problem #2: Elevator to the Stars
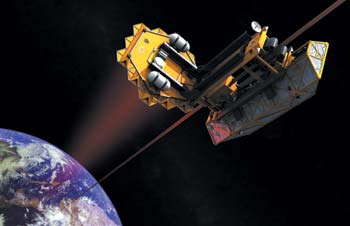
JT 150
TJ 80
TT 120
BT 170
Mike 12
AF 27
Lea 78
Leo 180
AI 55
GK 110
- We have a text file called candidates.txt, and this file contains lines of text, as illustrated above.
- Each line represents a person. The first string is that person's name (or initials), followed by a space, followed by a number representing that person's weight, in pounds. Any other line that doesn't follow this format is invalid.
- This list is actually the list of the first people who have signed up to use Google's first elevator to the moon when it becomes available (Check this NYT article to see what Google X is up to!)
- The only problem is that there is a maximum weight allowed, and that we may have to prevent some people from boarding. The maximum weight allowed is input by the user, as illustrated below (the user input is underlined):
>>> Google X Elevator ____ _ __ __ / ___| ___ ___ __ _| | ___ \ \/ / | | _ / _ \ / _ \ / _` | |/ _ \ \ / | |_| | (_) | (_) | (_| | | __/ / \ \____|\___/ \___/ \__, |_|\___| /_/\_\ |___/ Candidates for first boarding of elevator: JT (150 lbs) TJ (80 lbs) TT (120 lbs) BT (170 lbs) Mike (12 lbs) AF (27 lbs) Lea (78 lbs) Leo (180 lbs) AI (55 lbs) GK (110 lbs) Max weight? 2 hundred Invalid weight. Please try again. Max weight? infinite Invalid weight. Please try again. Max weight? -10 Invalid weight. Please try again. Max weight? 200 4 people can board the elevator with a weight limit of 200 lbs. Their combined weight is 172 lbs. The selected group includes: AF, AI, Lea, Mike The rejected group includes: BT, GK, JT, Leo, TJ, TT
- Our only criterion for boarding the elevator will be the weight of the candidates.
Your Assignment
Write a program called hw9b.py that will read the file candidates.txt, get a maximum allowed weight for the user, and output the answers to the following questions:
- Question 1
- What is the maximum number of people that can fit in the elevator?
- Question 2
- What is the total weight of the people who fit in the elevator?
- Question 3
- What are the names of the lucky selected people allowed to board the elevator?
- Question 4
- What are the names of the people who will have to wait and go in the second elevator?
- Use while or for loops, as you see fit.
- Make sure your program is robust! (It could be that the file does not exist, or that a line contains more than two words, or just one word, or is empty. It is also possible for a weight to be a real number, e.g. 180.5, which is acceptable. )
- Make sure your program works for any valid maximum weight provided by the user. A weight of 0 is valid. Any positive number is valid (integer or real).
Optional and Extra Credit (+2/3 points)
- Make your program output the list of candidates into batches, as illustrated below:
>>>
Google X Elevator
____ _ __ __
/ ___| ___ ___ __ _| | ___ \ \/ /
| | _ / _ \ / _ \ / _` | |/ _ \ \ /
| |_| | (_) | (_) | (_| | | __/ / \
\____|\___/ \___/ \__, |_|\___| /_/\_\
|___/
Candidates for first boarding of elevator:
JT (150 lbs)
TJ (80 lbs)
TT (120 lbs)
BT (170 lbs)
Mike (12 lbs)
AF (27 lbs)
Lea (78 lbs)
Leo (180 lbs)
AI (55 lbs)
GK (110 lbs)
Max weight? 200
--- BATCH 1 ---
4 people can board the elevator with a weight limit of 200 lbs.
Their combined weight is 172
The selected group includes: AF, AI, Lea, Mike
The rejected group includes: BT, GK, JT, Leo, TJ, TT
--- BATCH 2 ---
2 people can board the elevator with a weight limit of 200 lbs.
Their combined weight is 190
The selected group includes: GK, TJ
The rejected group includes: BT, JT, Leo, TT
--- BATCH 3 ---
1 person can board the elevator with a weight limit of 200 lbs.
Their combined weight is 120
The selected group includes: TT
The rejected group includes: BT, JT, Leo
--- BATCH 4 ---
1 person can board the elevator with a weight limit of 200 lbs.
Their combined weight is 150
The selected group includes: JT
The rejected group includes: BT, Leo
--- BATCH 5 ---
1 person can board the elevator with a weight limit of 200 lbs.
Their combined weight is 170
The selected group includes: BT
The rejected group includes: Leo
--- BATCH 6 ---
1 person can board the elevator with a weight limit of 200 lbs.
Their combined weight is 180
The selected group includes: Leo
The rejected group includes:
- Note that we try to send as many people up as possible, and as early as possible. In other words, the program always maximizes the number of people it can send up.
- You do not need to store your file under a different name if you do this optional part.
- Make sure your program stops if it cannot let the last people in the group enter the elevator (it could be that the weight of some people is larger than the maximum allowed weight).
Submission
- Submit your program as follows:
rsubmit hw9 hw9b.py
- if you worked on beowulf, submit your program using this page: http://cs.smith.edu/~111a/submit9.htm
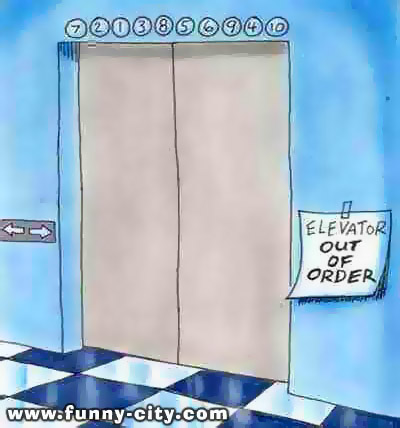