Difference between revisions of "CSC270 Final Exam 2012"
(→Problem #2 (1 point)) |
|||
(36 intermediate revisions by the same user not shown) | |||
Line 2: | Line 2: | ||
---- | ---- | ||
− | |||
− | + | =<center>CSC270 Final Exam 2012</center>= | |
− | Your assignment is to design a system that will | + | <bluebox> |
+ | ::''This exam is given under the rules of the <b>[http://www.smith.edu/sao/handbook/socialconduct/honorcodestatement.php honor code].</b><br>It is open books, open notes, open Web. However, until the deadline is passed, you cannot discuss any of the details of this exam with anybody except your instructor. This includes live conversation, email, sms, any mode of electronic communication.'' | ||
+ | |||
+ | ::''If you have questions about this exam, email them to your instructor and the question and its answer will be emailed back to the whole class. This way everybody will have access to the same material and information.'' | ||
+ | |||
+ | ::''The exam is due any time before <b>noon</b> on Thursday, <b> May 10th</b>''. ''You must demonstrate the correct operation of your hardware. Please make an appointment in advance for a 10-minute demonstration. The demonstration cannot be made past '''6:00 p.m. on 5/9/12'''. Once the demonstration is done, you have until the deadline to hand in a report showing the details of your design. If you cannot handin the exam in person, place it with the Arduino and USB cable in the envelop provided , write your name on it, and give it to Daryl Jett in the main office, on the 2nd floor.'' | ||
+ | </bluebox> | ||
+ | <br><br><br> | ||
+ | ==Problem #1 (3.7 points)== | ||
+ | |||
+ | You have to design some hardware that will interface with an [http://www.arduino.cc/ Arduino] microcontroller. | ||
+ | |||
+ | The Arduino uses the handshake protocol to send characters out. It sends all the ASCII characters from 'A' to 'Z', and starts over. It sends an active-low Strobe signal and listens to an active-low Ack signal. The Arduino waits 1/2 second between each character. Whenever it sends 'A' it activates its yellow on-board LED. The LED is turned off when a 'B' (or any character other than 'A' is sent). This LED turns ON every 13 seconds approximately, and stays ON for approximately 1/2 second (26 characters * 0.5 sec = 13 sec). | ||
+ | |||
+ | Your assignment is to design a system that will receive the characters, and turn ON its own LED whenever it sees the code for 'A'. This way your receiver hardware will blink synchronously with the Arduino. The LED should stay ON for about 1/2 second. | ||
<br /> | <br /> | ||
− | <center><videoflash> | + | <center><videoflash>KktRpt3Kymk</videoflash></center> |
<br /> | <br /> | ||
Line 17: | Line 30: | ||
<source lang="C"> | <source lang="C"> | ||
/* | /* | ||
− | ParallelPortSender | + | ParallelPortSender.pde |
D. Thiebaut | D. Thiebaut | ||
Line 31: | Line 44: | ||
The arduino waits 0.5 seconds between characters (as long as the receiver is absorbing them | The arduino waits 0.5 seconds between characters (as long as the receiver is absorbing them | ||
as fast as they are generated). | as fast as they are generated). | ||
+ | |||
+ | This example code is in the public domain. | ||
*/ | */ | ||
Line 42: | Line 57: | ||
int READY_TO_START = 0; | int READY_TO_START = 0; | ||
+ | int SENDING = 1; | ||
char ch = 'A'; | char ch = 'A'; | ||
int state = -1; // uninitialized | int state = -1; // uninitialized | ||
Line 47: | Line 63: | ||
// takes the character ch and puts its 5 LSBs on | // takes the character ch and puts its 5 LSBs on | ||
// pins D0, D1, D2, D3, and D4. | // pins D0, D1, D2, D3, and D4. | ||
− | void sendChar( ) { | + | void sendChar( char c ) { |
byte mask=1; | byte mask=1; | ||
int pin = D0; | int pin = D0; | ||
+ | |||
for ( int i=0; i<5; i++ ) { | for ( int i=0; i<5; i++ ) { | ||
− | if ( | + | if ( c & mask ) |
− | + | digitalWrite( pin, HIGH ); | |
else | else | ||
− | + | digitalWrite( pin, LOW ); | |
pin += 1; | pin += 1; | ||
mask <<= 1; | mask <<= 1; | ||
} | } | ||
− | |||
− | |||
} | } | ||
Line 66: | Line 81: | ||
// initialize the digital pin as an output. | // initialize the digital pin as an output. | ||
// Pin 13 has an LED connected on most Arduino boards: | // Pin 13 has an LED connected on most Arduino boards: | ||
− | pinMode(13, OUTPUT); | + | pinMode( 13, OUTPUT); // on-board LED always connected to Pin 13 |
− | pinMode( ACK, INPUT ); // | + | pinMode( ACK, INPUT ); // the ACK signal sent by your hardware |
− | pinMode( STB, OUTPUT); // strobe | + | pinMode( STB, OUTPUT); // strobe signal sent to your hardware |
pinMode( D0, OUTPUT ); | pinMode( D0, OUTPUT ); | ||
pinMode( D1, OUTPUT ); | pinMode( D1, OUTPUT ); | ||
Line 85: | Line 100: | ||
return; | return; | ||
+ | state = SENDING; | ||
+ | |||
// if character we're about to send is 'A' turn LED on | // if character we're about to send is 'A' turn LED on | ||
if ( ch == 'A' ) | if ( ch == 'A' ) | ||
digitalWrite( 13, HIGH ); | digitalWrite( 13, HIGH ); | ||
− | + | else | |
− | + | digitalWrite( 13, LOW ); // turn LED OFF | |
− | + | sendChar( ch ); | |
// set STB low | // set STB low | ||
Line 97: | Line 114: | ||
// wait for ACK to go low | // wait for ACK to go low | ||
while ( digitalRead( ACK ) == HIGH ) | while ( digitalRead( ACK ) == HIGH ) | ||
− | delay( | + | delay( 10 ); // wait |
// bring STB back up | // bring STB back up | ||
Line 103: | Line 120: | ||
// wait for ACK to get back up | // wait for ACK to get back up | ||
− | + | while ( digitalRead( ACK ) == LOW ) | |
− | delay( | + | delay( 10 ); // wait |
+ | |||
+ | // When we're here, the character has been absorbed by the receiver. | ||
+ | // send 0 on all output data bits | ||
+ | sendChar( 0 ); | ||
+ | |||
+ | // wait 1/2 second before sending new char | ||
+ | delay( 500 ); | ||
+ | |||
+ | // next char in alphabet | ||
+ | ch = ch + 1; | ||
+ | if ( ch > 'Z' ) ch = 'A'; | ||
state = READY_TO_START; | state = READY_TO_START; | ||
Line 111: | Line 139: | ||
</source> | </source> | ||
<br /> | <br /> | ||
+ | Feel free to modify this program if needed. A good introduction to the Arduino and how to download programs to it can be found [http://arduino.cc/en/Guide/HomePage here]. Your solution circuit, however, '''must''' work with the original program shown above. | ||
+ | |||
Your solution circuit should interface with the Arduino as illustrated below: | Your solution circuit should interface with the Arduino as illustrated below: | ||
Line 117: | Line 147: | ||
<br /> | <br /> | ||
+ | The physical location of the different signals is illustrated in the figure below. | ||
+ | |||
+ | <br /> | ||
+ | <center>[[Image:ArduinoParallelPortPinOut.png]]</center> | ||
+ | <br /> | ||
+ | <center>[[Image:ArduinoParallelPortPinOut2.jpg|500px]]</center> | ||
+ | <br /> | ||
The Arduino must be connected to a computer via the USB cable to get the appropriate power. The computer does not need to have the arduino software installed for the Arduino to work. The Arduino keeps its program in memory when it is powered down. | The Arduino must be connected to a computer via the USB cable to get the appropriate power. The computer does not need to have the arduino software installed for the Arduino to work. The Arduino keeps its program in memory when it is powered down. | ||
− | </ | + | ==Misc. Information== |
+ | ===Arduino=== | ||
+ | * The Arduino only sends the lower 5 bits of the ASCII characters because the first 3 bits for 'A' to 'Z' are always 010. 'A' is '''010'''00001. 'B' is '''010'''00010, ... 'Z' is '''010'''11010. | ||
+ | * The Arduino will keep its program in memory when it is powered off. This means that as soon as you connect it to a live USB port, the sending program will start. The Arduino will wait for the receiving hardware to activate ACK and get characters. | ||
+ | * You can reset the Arduino and force it to restart the program by pressing the small push-button in the middle of the board. Upon reset, the Arduino will right away start sending an 'A'. | ||
+ | * You can easily test whether your Arduino is running properly by connecting its STB to its ACK signal in a loop. In effect you make it think it has a very very fast receiver that sets its ACK simultaneously as the STB is activated. In other words, if you connect Pin 3 of the Arduino to its Pin 4, it will think it has a live receiver and will send all the letters of the alphabet in 13 seconds, flashing its LED for 1/2 second once a loop. | ||
+ | * You can download the Arduino IDE (free from [http://arduino.cc/hu/Main/Software http://arduino.cc]). If you want to upload a modified version of the software to the Arduino board, make sure you set the board type in the '''Tools''' section to '''Diecimila'''. | ||
+ | |||
+ | ===Arduino Receiver Code=== | ||
+ | For completeness, the code for the receiving Arduino is shown below. | ||
+ | |||
+ | <br /><source lang="C"> | ||
+ | /* | ||
+ | parallelPortReceiver.pde | ||
+ | D. Thiebaut | ||
+ | |||
+ | Uses a handshake protocol (STB & ACK) to receive characters sent in 5-bit ASCII to it. | ||
+ | The characters are 'A' to 'Z' and repeat forever. All 26 characters of the alphabet are | ||
+ | sent. | ||
+ | |||
+ | Whenever a character is received, the bits 010 are appended to it to form an 8-bit ASCII. | ||
+ | |||
+ | When 'A' is recognized (hex 41), the receiver activates the on-board LED. When any other | ||
+ | character is received, the LED is turned off. | ||
+ | |||
+ | The program outputs each character to the serial monitor, which must be set to a baud rate | ||
+ | of 115200 baud. | ||
+ | |||
+ | |||
+ | This example code is in the public domain. | ||
+ | */ | ||
+ | |||
+ | int STB = 3; | ||
+ | int ACK = 4; | ||
+ | int D0 = 5; | ||
+ | int D1 = 6; | ||
+ | int D2 = 7; | ||
+ | int D3 = 8; | ||
+ | int D4 = 9; | ||
+ | int state = -1; | ||
+ | int READY_TO_START = 1; | ||
+ | |||
+ | // takes the character ch and puts its 5 LSBs on | ||
+ | // pins D0, D1, D2, D3, and D4. | ||
+ | char receiveChar( ) { | ||
+ | char ch = 0x40; | ||
+ | int pin = D0; | ||
+ | byte mask = 1; | ||
+ | |||
+ | for ( int i=0; i<5; i++ ) { | ||
+ | if ( digitalRead( pin ) == HIGH ) | ||
+ | ch = ch + mask; | ||
+ | mask <<= 1; | ||
+ | pin += 1; | ||
+ | } | ||
+ | |||
+ | return ch; | ||
+ | } | ||
+ | |||
+ | void setup() { | ||
+ | // initialize the digital pin as an output. | ||
+ | // Pin 13 has an LED connected on most Arduino boards: | ||
+ | pinMode(13, OUTPUT); | ||
+ | pinMode( ACK, OUTPUT ); // ack to kit | ||
+ | pinMode( STB, INPUT); // strobe from kit | ||
+ | pinMode( D0, INPUT ); | ||
+ | pinMode( D1, INPUT ); | ||
+ | pinMode( D2, INPUT ); | ||
+ | pinMode( D3, INPUT ); | ||
+ | pinMode( D4, INPUT ); | ||
+ | |||
+ | // set data communication speed | ||
+ | Serial.begin( 115200 ); // 115,200 baud, or bits/sec | ||
+ | |||
+ | // set STB high | ||
+ | digitalWrite( ACK, HIGH ); | ||
+ | state = READY_TO_START; | ||
+ | |||
+ | } | ||
+ | |||
+ | void loop() { | ||
+ | char ch; | ||
+ | |||
+ | // if we haven't been initialized, wait... | ||
+ | if ( state != READY_TO_START ) | ||
+ | return; | ||
+ | |||
+ | // wait till STB == 0 | ||
+ | while ( digitalRead( STB ) == HIGH ) | ||
+ | delay( 10 ); // wait 10 ms | ||
+ | |||
+ | // STB is low. Read the data | ||
+ | ch = receiveChar(); | ||
+ | //Serial.println( ch ); | ||
+ | |||
+ | // set ACK LOW | ||
+ | if ( ch=='A' ) | ||
+ | digitalWrite( 13, HIGH ); // turn LED OFF | ||
+ | else | ||
+ | digitalWrite( 13, LOW ); | ||
+ | |||
+ | // set ACK low | ||
+ | digitalWrite( ACK, LOW ); | ||
+ | |||
+ | // wait for STOB to go high | ||
+ | while ( digitalRead( STB ) == LOW ) | ||
+ | delay( 10 ); // wait | ||
+ | |||
+ | // bring ACK back up | ||
+ | digitalWrite( ACK, HIGH ); | ||
+ | |||
+ | state = READY_TO_START; | ||
+ | } | ||
+ | |||
+ | </source><br /> | ||
+ | |||
+ | ==Problem #2 (1 point)== | ||
+ | |||
+ | You have several choices. Pick one of the 3 following circuits | ||
+ | * Your solution to the Homework 10 where you had to create a FSM to handle a handshake protocol, or | ||
+ | * Your solution to Problem 1 of this exam if you selected not to use a 6811 | ||
+ | * You create a new solution for Problem 1 that uses only gates and flip-flops, in case you selected a solution for this exam with a 6811. | ||
+ | |||
+ | Once you have selected a circuit: | ||
+ | * Create and simulate your circuit with the Xilinx ISE. | ||
+ | * You can use either schematics or Verilog to generate a description of your circuit. | ||
+ | * If you use Verilog, include the schematics (drawn by hand is fine) of the circuit so that I can understand it better. | ||
+ | * Include a screen capture of | ||
+ | *# your schematics, if you used this method, or a copy of the Verilog description of your circuit. | ||
+ | *# a capture of the Isim window. Either the waveform or the output in the console is sufficient. | ||
+ | |||
+ | ==Submission== | ||
+ | |||
+ | * Make an appointment to demonstrate the correct operation of your circuit. | ||
+ | * Please return your exam with the Arduino microcontroller and the USB cable in the envelop provided. You can drop it off at my office or leave it with Daryl Jett in the main office. | ||
+ | |||
+ | ==Grading== | ||
+ | |||
+ | * 4.5 = A+, 4.0 = A, 3.7 = A-, 3.3 = B+, 3.0 = B, etc... | ||
+ | |||
+ | ==Important Information== | ||
+ | * If you decide to design an input port for the 6811, <font color="red">you must wait and not connect the output of the tristate buffers to the data bus '''until''' you are sure that you have a short negative pulse on the enable of your drivers (assuming an active-low enable)</font>. Once you observe the negative pulse for the enable of the drivers can you complete the connection to the data bus. See the '''yellow signal''' in the photo below for reference. | ||
+ | <br /> | ||
+ | <center>[[File:6811IO1.jpg|500px]]<br />(Waveform captured by Tiffany Liu)</center> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <center>'''Good Luck!'''</center> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | |||
+ | [[Category:6811]][[Category:CSC270]][[Category:arduino]][[Category:Verilog]][[Category:Xilinx]] |
Latest revision as of 12:43, 2 May 2012
--D. Thiebaut 23:04, 25 April 2012 (EDT)
Contents
CSC270 Final Exam 2012
- This exam is given under the rules of the honor code.
It is open books, open notes, open Web. However, until the deadline is passed, you cannot discuss any of the details of this exam with anybody except your instructor. This includes live conversation, email, sms, any mode of electronic communication.
- This exam is given under the rules of the honor code.
- If you have questions about this exam, email them to your instructor and the question and its answer will be emailed back to the whole class. This way everybody will have access to the same material and information.
- The exam is due any time before noon on Thursday, May 10th. You must demonstrate the correct operation of your hardware. Please make an appointment in advance for a 10-minute demonstration. The demonstration cannot be made past 6:00 p.m. on 5/9/12. Once the demonstration is done, you have until the deadline to hand in a report showing the details of your design. If you cannot handin the exam in person, place it with the Arduino and USB cable in the envelop provided , write your name on it, and give it to Daryl Jett in the main office, on the 2nd floor.
Problem #1 (3.7 points)
You have to design some hardware that will interface with an Arduino microcontroller.
The Arduino uses the handshake protocol to send characters out. It sends all the ASCII characters from 'A' to 'Z', and starts over. It sends an active-low Strobe signal and listens to an active-low Ack signal. The Arduino waits 1/2 second between each character. Whenever it sends 'A' it activates its yellow on-board LED. The LED is turned off when a 'B' (or any character other than 'A' is sent). This LED turns ON every 13 seconds approximately, and stays ON for approximately 1/2 second (26 characters * 0.5 sec = 13 sec).
Your assignment is to design a system that will receive the characters, and turn ON its own LED whenever it sees the code for 'A'. This way your receiver hardware will blink synchronously with the Arduino. The LED should stay ON for about 1/2 second.
The program running in the Arduino is listed below:
/*
ParallelPortSender.pde
D. Thiebaut
Sends the lower 5-bits of an ASCII character between 'A' and 'Z', included, over
and over to a receiver.
The 5 bits are sent following a handshake protocol.
The arduino sends a Strobe signal on its digital pin 3.
It listens to an ACK signal from the receiver on its pin 4.
The least significant bit of the byte is sent on Pin 5.
The most significant bit of the 5-bit word is sent on Pin 9.
Whenever the character 'A' is sent, the LED connected to Pin 13 is turned ON.
It is turned off when 'B' is sent.
The arduino waits 0.5 seconds between characters (as long as the receiver is absorbing them
as fast as they are generated).
This example code is in the public domain.
*/
int STB = 3;
int ACK = 4;
int D0 = 5;
int D1 = 6;
int D2 = 7;
int D3 = 8;
int D4 = 9;
int READY_TO_START = 0;
int SENDING = 1;
char ch = 'A';
int state = -1; // uninitialized
// takes the character ch and puts its 5 LSBs on
// pins D0, D1, D2, D3, and D4.
void sendChar( char c ) {
byte mask=1;
int pin = D0;
for ( int i=0; i<5; i++ ) {
if ( c & mask )
digitalWrite( pin, HIGH );
else
digitalWrite( pin, LOW );
pin += 1;
mask <<= 1;
}
}
void setup() {
// initialize the digital pin as an output.
// Pin 13 has an LED connected on most Arduino boards:
pinMode( 13, OUTPUT); // on-board LED always connected to Pin 13
pinMode( ACK, INPUT ); // the ACK signal sent by your hardware
pinMode( STB, OUTPUT); // strobe signal sent to your hardware
pinMode( D0, OUTPUT );
pinMode( D1, OUTPUT );
pinMode( D2, OUTPUT );
pinMode( D3, OUTPUT );
pinMode( D4, OUTPUT );
// set STB high
digitalWrite( STB, HIGH );
state = READY_TO_START;
}
void loop() {
// if we haven't been initialized, wait...
if ( state != READY_TO_START )
return;
state = SENDING;
// if character we're about to send is 'A' turn LED on
if ( ch == 'A' )
digitalWrite( 13, HIGH );
else
digitalWrite( 13, LOW ); // turn LED OFF
sendChar( ch );
// set STB low
digitalWrite( STB, LOW );
// wait for ACK to go low
while ( digitalRead( ACK ) == HIGH )
delay( 10 ); // wait
// bring STB back up
digitalWrite( STB, HIGH );
// wait for ACK to get back up
while ( digitalRead( ACK ) == LOW )
delay( 10 ); // wait
// When we're here, the character has been absorbed by the receiver.
// send 0 on all output data bits
sendChar( 0 );
// wait 1/2 second before sending new char
delay( 500 );
// next char in alphabet
ch = ch + 1;
if ( ch > 'Z' ) ch = 'A';
state = READY_TO_START;
}
Feel free to modify this program if needed. A good introduction to the Arduino and how to download programs to it can be found here. Your solution circuit, however, must work with the original program shown above.
Your solution circuit should interface with the Arduino as illustrated below:
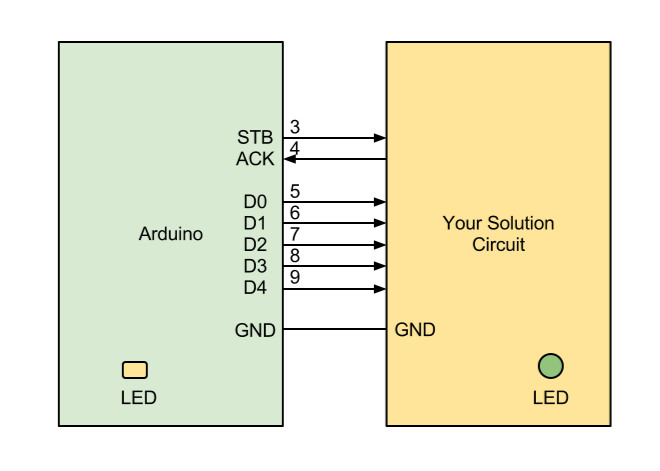
The physical location of the different signals is illustrated in the figure below.
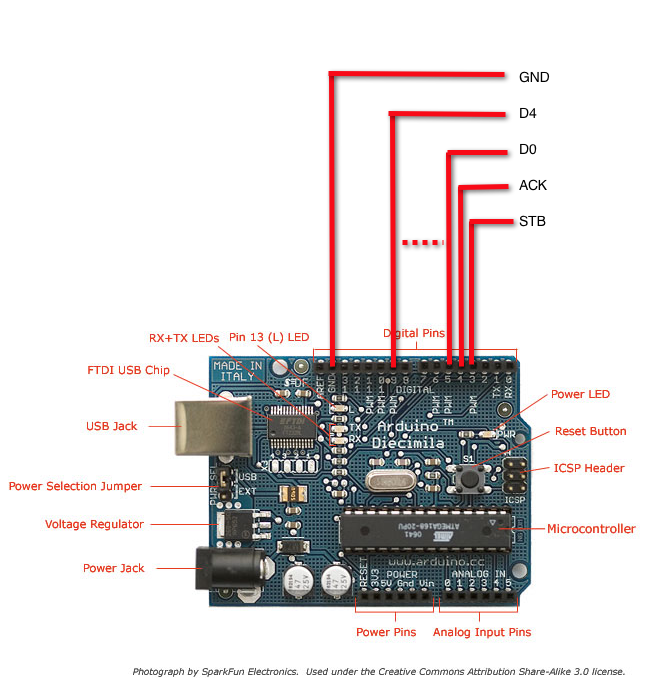
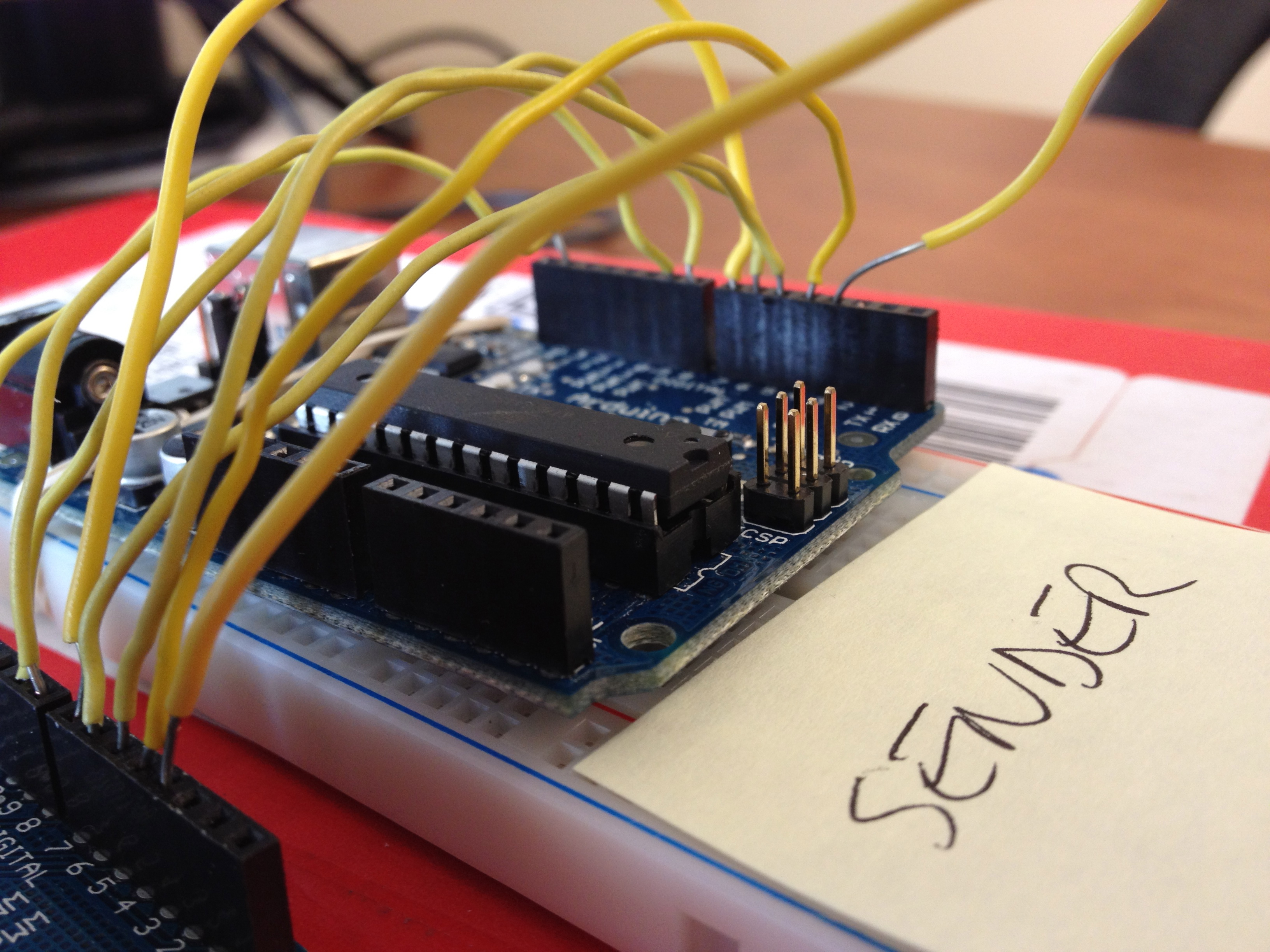
The Arduino must be connected to a computer via the USB cable to get the appropriate power. The computer does not need to have the arduino software installed for the Arduino to work. The Arduino keeps its program in memory when it is powered down.
Misc. Information
Arduino
- The Arduino only sends the lower 5 bits of the ASCII characters because the first 3 bits for 'A' to 'Z' are always 010. 'A' is 01000001. 'B' is 01000010, ... 'Z' is 01011010.
- The Arduino will keep its program in memory when it is powered off. This means that as soon as you connect it to a live USB port, the sending program will start. The Arduino will wait for the receiving hardware to activate ACK and get characters.
- You can reset the Arduino and force it to restart the program by pressing the small push-button in the middle of the board. Upon reset, the Arduino will right away start sending an 'A'.
- You can easily test whether your Arduino is running properly by connecting its STB to its ACK signal in a loop. In effect you make it think it has a very very fast receiver that sets its ACK simultaneously as the STB is activated. In other words, if you connect Pin 3 of the Arduino to its Pin 4, it will think it has a live receiver and will send all the letters of the alphabet in 13 seconds, flashing its LED for 1/2 second once a loop.
- You can download the Arduino IDE (free from http://arduino.cc). If you want to upload a modified version of the software to the Arduino board, make sure you set the board type in the Tools section to Diecimila.
Arduino Receiver Code
For completeness, the code for the receiving Arduino is shown below.
/*
parallelPortReceiver.pde
D. Thiebaut
Uses a handshake protocol (STB & ACK) to receive characters sent in 5-bit ASCII to it.
The characters are 'A' to 'Z' and repeat forever. All 26 characters of the alphabet are
sent.
Whenever a character is received, the bits 010 are appended to it to form an 8-bit ASCII.
When 'A' is recognized (hex 41), the receiver activates the on-board LED. When any other
character is received, the LED is turned off.
The program outputs each character to the serial monitor, which must be set to a baud rate
of 115200 baud.
This example code is in the public domain.
*/
int STB = 3;
int ACK = 4;
int D0 = 5;
int D1 = 6;
int D2 = 7;
int D3 = 8;
int D4 = 9;
int state = -1;
int READY_TO_START = 1;
// takes the character ch and puts its 5 LSBs on
// pins D0, D1, D2, D3, and D4.
char receiveChar( ) {
char ch = 0x40;
int pin = D0;
byte mask = 1;
for ( int i=0; i<5; i++ ) {
if ( digitalRead( pin ) == HIGH )
ch = ch + mask;
mask <<= 1;
pin += 1;
}
return ch;
}
void setup() {
// initialize the digital pin as an output.
// Pin 13 has an LED connected on most Arduino boards:
pinMode(13, OUTPUT);
pinMode( ACK, OUTPUT ); // ack to kit
pinMode( STB, INPUT); // strobe from kit
pinMode( D0, INPUT );
pinMode( D1, INPUT );
pinMode( D2, INPUT );
pinMode( D3, INPUT );
pinMode( D4, INPUT );
// set data communication speed
Serial.begin( 115200 ); // 115,200 baud, or bits/sec
// set STB high
digitalWrite( ACK, HIGH );
state = READY_TO_START;
}
void loop() {
char ch;
// if we haven't been initialized, wait...
if ( state != READY_TO_START )
return;
// wait till STB == 0
while ( digitalRead( STB ) == HIGH )
delay( 10 ); // wait 10 ms
// STB is low. Read the data
ch = receiveChar();
//Serial.println( ch );
// set ACK LOW
if ( ch=='A' )
digitalWrite( 13, HIGH ); // turn LED OFF
else
digitalWrite( 13, LOW );
// set ACK low
digitalWrite( ACK, LOW );
// wait for STOB to go high
while ( digitalRead( STB ) == LOW )
delay( 10 ); // wait
// bring ACK back up
digitalWrite( ACK, HIGH );
state = READY_TO_START;
}
Problem #2 (1 point)
You have several choices. Pick one of the 3 following circuits
- Your solution to the Homework 10 where you had to create a FSM to handle a handshake protocol, or
- Your solution to Problem 1 of this exam if you selected not to use a 6811
- You create a new solution for Problem 1 that uses only gates and flip-flops, in case you selected a solution for this exam with a 6811.
Once you have selected a circuit:
- Create and simulate your circuit with the Xilinx ISE.
- You can use either schematics or Verilog to generate a description of your circuit.
- If you use Verilog, include the schematics (drawn by hand is fine) of the circuit so that I can understand it better.
- Include a screen capture of
- your schematics, if you used this method, or a copy of the Verilog description of your circuit.
- a capture of the Isim window. Either the waveform or the output in the console is sufficient.
Submission
- Make an appointment to demonstrate the correct operation of your circuit.
- Please return your exam with the Arduino microcontroller and the USB cable in the envelop provided. You can drop it off at my office or leave it with Daryl Jett in the main office.
Grading
- 4.5 = A+, 4.0 = A, 3.7 = A-, 3.3 = B+, 3.0 = B, etc...
Important Information
- If you decide to design an input port for the 6811, you must wait and not connect the output of the tristate buffers to the data bus until you are sure that you have a short negative pulse on the enable of your drivers (assuming an active-low enable). Once you observe the negative pulse for the enable of the drivers can you complete the connection to the data bus. See the yellow signal in the photo below for reference.
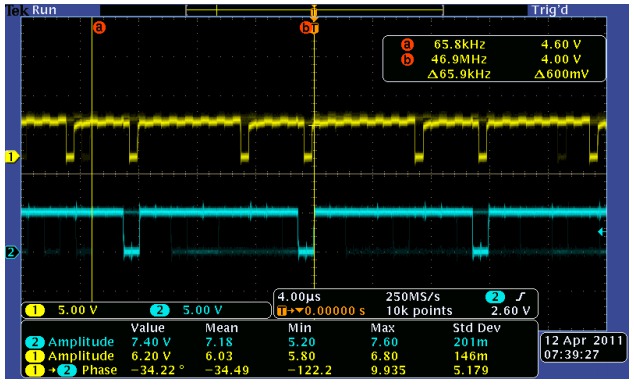
(Waveform captured by Tiffany Liu)