Difference between revisions of "CSC212 Lab 7 2014"
(→Developing Java Programs with Eclipse) |
|||
(10 intermediate revisions by the same user not shown) | |||
Line 1: | Line 1: | ||
--[[User:Thiebaut|D. Thiebaut]] ([[User talk:Thiebaut|talk]]) 14:47, 6 October 2014 (EDT) | --[[User:Thiebaut|D. Thiebaut]] ([[User talk:Thiebaut|talk]]) 14:47, 6 October 2014 (EDT) | ||
---- | ---- | ||
+ | <br /> | ||
+ | {| width="100%" | ||
+ | | | ||
+ | __TOC__ | ||
+ | | | ||
+ | [[File:EclipseLogo.png|right|200px|right]] | ||
+ | |} | ||
=Installing Eclipse= | =Installing Eclipse= | ||
Line 7: | Line 14: | ||
</tanbox> | </tanbox> | ||
<br /> | <br /> | ||
− | ==On Windows== | + | ==Installing On Windows== |
<br /> | <br /> | ||
Follow the directions from this video and installe Eclipse and the Java JDK. | Follow the directions from this video and installe Eclipse and the Java JDK. | ||
Line 13: | Line 20: | ||
<center><videoflash>4T1iY3CeBGg</videoflash></center> | <center><videoflash>4T1iY3CeBGg</videoflash></center> | ||
<br /> | <br /> | ||
− | == | + | |
+ | ==Installing on a Mac== | ||
<br /> | <br /> | ||
* Follow the directions shown in this video: | * Follow the directions shown in this video: | ||
<br /> | <br /> | ||
<center><videoflash>nFhNDFrf6UM</videoflash></center> | <center><videoflash>nFhNDFrf6UM</videoflash></center> | ||
+ | <br /> | ||
+ | * If you get a message "''Eclipse cannot be opened because of an unidentified developer''", go to '''System Preferences''', '''Security and Privacy''', and click on '''Open Anyway'''. | ||
+ | <br /> | ||
+ | |||
+ | <br /> | ||
+ | =<center>Developing Java Programs with Eclipse</center>= | ||
+ | <br /> | ||
+ | {| width="100%" | ||
+ | | | ||
+ | <bluebox> | ||
+ | This section of the lab assumes that you have installed Eclipse on your system. If not, then use one of the Linux Mint machines in the lab. For the purpose of discovering Eclipse and its amazing features, we will develop a program using the ''top-down'' approach. | ||
+ | </bluebox> | ||
+ | | | ||
+ | [[Image:EclipseLogo.png|100px]] | ||
+ | |} | ||
+ | <br /> | ||
+ | |||
+ | <br /> | ||
+ | |||
+ | =Problem of the Day= | ||
+ | <br /> | ||
+ | Write a program that reads a collection of lines from the standard input (either from the keyboard or redirected from a file). The lines contain a list of '''file names''' along with their '''size''', expressed in Kilobytes. Here's an example of the type of input your program will receive: | ||
+ | <br /> | ||
+ | ::<source lang="text"> | ||
+ | ./ArtOfAssembly 10012 | ||
+ | ./ArtOfAssembly/CH01 276 | ||
+ | ./ArtOfAssembly/CH02 300 | ||
+ | ./ArtOfAssembly/CH03 480 | ||
+ | ./ArtOfAssembly/CH04 272 | ||
+ | ./ArtOfAssembly/CH05 328 | ||
+ | ./ArtOfAssembly/CH06 620 | ||
+ | ./ArtOfAssembly/CH07 148 | ||
+ | ./ArtOfAssembly/CH08 748 | ||
+ | ./ArtOfAssembly/CH09 436 | ||
+ | ./ArtOfAssembly/CH10 248 | ||
+ | ./ArtOfAssembly/CH11 436 | ||
+ | ./ArtOfAssembly/CH12 348 | ||
+ | ./ArtOfAssembly/CH13 524 | ||
+ | ./ArtOfAssembly/CH14 500 | ||
+ | ./ArtOfAssembly/CH14/org_gifs 72 | ||
+ | ./ArtOfAssembly/CH15 380 | ||
+ | ./ArtOfAssembly/CH16 772 | ||
+ | ./ArtOfAssembly/CH16/chars 32 | ||
+ | ./ArtOfAssembly/CH17 248 | ||
+ | ./ArtOfAssembly/CH18 304 | ||
+ | ./ArtOfAssembly/CH19 612 | ||
+ | ./ArtOfAssembly/CH20 348 | ||
+ | ./ArtOfAssembly/CH21 200 | ||
+ | ./ArtOfAssembly/CH22 196 | ||
+ | ./ArtOfAssembly/CH23 60 | ||
+ | ./ArtOfAssembly/CH24 352 | ||
+ | ./ArtOfAssembly/CH25 256 | ||
+ | ./ArtOfAssembly/chars 16 | ||
+ | ./ArtOfAssembly/fwd 68 | ||
+ | </source> | ||
+ | <br /> | ||
+ | The last line indicates that the file '''fwd''' in the directory '''ArtOfAssembly''' is 68 KBytes in size. | ||
+ | <br /> | ||
+ | The Assignment is to keep track of all the files that are larger than 100 KBytes and store them in a list for later processing (for example because we may want to compress them). | ||
+ | <br /> | ||
+ | Your program will print the list of the large files (>100K). | ||
+ | <br /> | ||
+ | |||
+ | =Top Level= | ||
+ | <br /> | ||
+ | Think for a minute about what your program needs to do: | ||
+ | |||
+ | 1. get the input, one line at a time, | ||
+ | 2. extract the size from the line. | ||
+ | 3. if the size is larger than 100, add the file name and its size to a list | ||
+ | 4. once the input has been completely read, output the list of the name and size of the large files | ||
+ | |||
+ | <br /> | ||
+ | Ready? You are now going to write this program with '''Eclipse''' as your IDE (Integrated Development Environment). | ||
+ | <br /> | ||
+ | |||
+ | =Launch Eclipse= | ||
+ | <br /> | ||
+ | <center>[[Image:CSC212_Ecplise1.png|600px]]</center> | ||
+ | <br /> | ||
+ | * Close the Welcome Window | ||
+ | <br /> | ||
+ | <center>[[Image:CSC212_Ecplise2.png|600px]]</center> | ||
+ | <br /> | ||
+ | =Create A Project= | ||
+ | <br /> | ||
+ | * Create a new '''Project'''. Eclipse organizes Java programs into project. Let's create a project called '''CSC212''' where you'll store your Java file(s). You should have to do this only once this semester (unless you want to organize your files by, say, homework or lab sections). | ||
+ | :* '''File''' | ||
+ | :* '''New''' | ||
+ | :* '''Java Project''' | ||
+ | :* '''CSC212''' | ||
+ | :* '''Finish''' | ||
+ | <br /> | ||
+ | <center>[[Image:CSC212_Ecplise3.png|400px]]</center> | ||
+ | <br /> | ||
+ | =Create a new Java File= | ||
+ | <br /> | ||
+ | * Select '''CSC212''' | ||
+ | * Right-Click or Control-Click on it, depending on your type of machine, and pick | ||
+ | :* '''File''' | ||
+ | :* '''New''' | ||
+ | :* '''Class''' | ||
+ | :* '''Lab7a''' (don't add the .java extension) | ||
+ | :* Click on '''public static void main( String[] args )''' | ||
+ | <br /> | ||
+ | <center>[[Image:CSC212_Ecplise4.png|500px]]</center> | ||
+ | <br /> | ||
+ | :* '''Finish''' | ||
+ | <br /> | ||
+ | <center>[[Image:CSC212_Ecplise5.png|500px]]</center> | ||
+ | <br /> | ||
+ | =Reading Input= | ||
+ | <br /> | ||
+ | In the main program, declare an '''ArrayList''' called lines. | ||
+ | <br /> | ||
+ | ::<source lang="java"> | ||
+ | ArrayList lines = null; | ||
+ | </source> | ||
+ | <br /> | ||
+ | * Notice that the ArrayList word is underlined in <font color="red">red</font>, and there's a small red <font color="red">'''x'''</font> in the left margin. | ||
+ | * Click on the red <font color="red">'''x'''</font>. A menu of suggestions will appear. Usually the top suggestion will be the right one. Sometimes not, so make sure you read what it suggested as a fix: | ||
+ | <br /> | ||
+ | <center>[[Image:CSC212_Ecplise6.png|600px]]</center> | ||
+ | <br /> | ||
+ | * Double-click the '''import 'ArrayList' (Java.util)''' suggestion. This will clear both the red marker, and the red underline. | ||
+ | * Add more code: | ||
+ | <br /> | ||
+ | ::<source lang="java"> | ||
+ | ArrayList lines = null; | ||
+ | lines = readInput(); | ||
+ | </source> | ||
+ | <br /> | ||
+ | * Note the new red underline and cross. Click on the cross and accept for Eclipse to create a new method for you. You will get a new method in your code: | ||
+ | <br /> | ||
+ | ::<source lang="java"> | ||
+ | |||
+ | private static ArrayList readInput() { | ||
+ | // TODO Auto-generated method stub | ||
+ | return null; | ||
+ | } | ||
+ | </source> | ||
+ | <br /> | ||
+ | * Instead of making the method read from the standard input (i.e. the keyboard), make it create a list of string and return it: | ||
+ | <br /> | ||
+ | ::<source lang="java"> | ||
+ | |||
+ | private static ArrayList readInput() { | ||
+ | ArrayList l = new ArrayList(); | ||
+ | l.add( "ArtOfAssembly 10012" ); | ||
+ | l.add( "ArtOfAssembly/CH01 276" ); | ||
+ | l.add( "ArtOfAssembly/CH02 300" ); | ||
+ | l.add( "ArtOfAssembly/CH03 480" ); | ||
+ | l.add( "ArtOfAssembly/CH04 272" ); | ||
+ | l.add( "ArtOfAssembly/CH05 328" ); | ||
+ | l.add( "ArtOfAssembly/CH06 620" ); | ||
+ | l.add( "ArtOfAssembly/CH07 148" ); | ||
+ | l.add( "ArtOfAssembly/CH08 748" ); | ||
+ | l.add( "ArtOfAssembly/CH09 436" ); | ||
+ | l.add( "ArtOfAssembly/CH10 248" ); | ||
+ | l.add( "ArtOfAssembly/CH11 436" ); | ||
+ | l.add( "ArtOfAssembly/CH12 348" ); | ||
+ | l.add( "ArtOfAssembly/CH13 524" ); | ||
+ | l.add( "ArtOfAssembly/CH14 500" ); | ||
+ | l.add( "ArtOfAssembly/CH14/org_gifs 72" ); | ||
+ | l.add( "ArtOfAssembly/CH15 380" ); | ||
+ | l.add( "ArtOfAssembly/CH16 772" ); | ||
+ | l.add( "ArtOfAssembly/CH16/chars 32" ); | ||
+ | l.add( "ArtOfAssembly/CH17 248" ); | ||
+ | l.add( "ArtOfAssembly/CH18 304" ); | ||
+ | l.add( "ArtOfAssembly/CH19 612" ); | ||
+ | l.add( "ArtOfAssembly/CH20 348" ); | ||
+ | l.add( "ArtOfAssembly/CH21 200" ); | ||
+ | l.add( "ArtOfAssembly/CH22 196" ); | ||
+ | l.add( "ArtOfAssembly/CH23 60" ); | ||
+ | l.add( "ArtOfAssembly/CH24 352" ); | ||
+ | l.add( "ArtOfAssembly/CH25 256" ); | ||
+ | l.add( "ArtOfAssembly/chars 16" ); | ||
+ | l.add( "ArtOfAssembly/fwd 68" ); | ||
+ | return l; | ||
+ | } | ||
+ | </source> | ||
+ | <br /> | ||
+ | |||
+ | =Testing Method readInput()= | ||
+ | <br /> | ||
+ | * It is a good idea to always test a new method once it is added to the code. | ||
+ | * Change main() as follows: | ||
+ | <br /> | ||
+ | ::<source lang="java"> | ||
+ | public static void main(String[] args) { | ||
+ | ArrayList lines = null; | ||
+ | |||
+ | // get lines from input | ||
+ | lines = readInput(); | ||
+ | |||
+ | // display input lines (for debugging) | ||
+ | Iterator<String> it = lines.iterator(); | ||
+ | while ( it.hasNext() ) { | ||
+ | String line = (String) it.next(); | ||
+ | System.out.println( line ); | ||
+ | } | ||
+ | } | ||
+ | </source> | ||
+ | * You will have noticed that Eclipse suggests code as you type. This is a nice feature. | ||
+ | * Run the program by clicking on the white triangle in a green circle in the top bar. If you get a window opening up asking you to '''select a resource to save''', simply check the box '''Always save resource before launching''', and click '''OK'''. | ||
+ | * Notice that a new tab opens up in the bottom pane for the '''Console''' output. Your output will be there. You can scroll it up or down. | ||
+ | <br /> | ||
+ | =Minimizing The Methods= | ||
+ | <br /> | ||
+ | * Now that we see that '''readInput()''' works according to plans, let's ''minimize'' it, as it is a very long method. | ||
+ | * Locate the small minus-sign in the margin, to the left of '''private static ArrayList readInput() {'''. | ||
+ | * Click on it. Notice that the code of '''readInput()''' disappears. It's still part of the method, but not shown in the editor to save space. You can get it "back" by clicking on the plus-sign. | ||
+ | <br /> | ||
+ | |||
+ | =Adding a Private Function= | ||
+ | <br /> | ||
+ | * The code to display the list of lines can be useful in other parts of our program. Let's make it a method. | ||
+ | * Change your main() function to this code: | ||
+ | <br /> | ||
+ | ::<source lang="java" highlight="8"> | ||
+ | public static void main(String[] args) { | ||
+ | ArrayList lines = null; | ||
+ | |||
+ | // get lines from input | ||
+ | lines = readInput(); | ||
+ | |||
+ | // display input lines (for debugging) | ||
+ | displayListOfLines( lines ); | ||
+ | Iterator<String> it = lines.iterator(); | ||
+ | while ( it.hasNext() ) { | ||
+ | String line = (String) it.next(); | ||
+ | System.out.println( line ); | ||
+ | } | ||
+ | } | ||
+ | </source> | ||
+ | <br /> | ||
+ | * Eclipse will suggest adding the method you just called. Just do it, and put the 4 lines of code required for printing the list inside the new method. | ||
+ | * Fix errors (small red crosses) that Eclipse points out to you, if any. | ||
+ | * Main should look like this: | ||
+ | <br /> | ||
+ | ::<source lang="java"> | ||
+ | public static void main(String[] args) { | ||
+ | ArrayList lines = null; | ||
+ | |||
+ | // get lines from input | ||
+ | lines = readInput(); | ||
+ | |||
+ | // display input lines (for debugging) | ||
+ | displayListOfLines( lines ); | ||
+ | } | ||
+ | </source> | ||
+ | <br /> | ||
+ | * Check that everything works by running your code again. | ||
+ | <br /> | ||
+ | =A Side-Step: Testing the String.split() method= | ||
+ | <br /> | ||
+ | * Each line our program has to process is a String with a very simple format: | ||
+ | |||
+ | file-name integer | ||
+ | |||
+ | * We need to extract the integer part of this string. Let's take a side-step and do some testing in a different program. | ||
+ | * Right/Control click on '''(default package)''' in the '''Package Explorer''' pane. | ||
+ | * Click '''New''', then '''class'''. Call it '''TestSplit''' and ask Eclipse to add a main() method. | ||
+ | * Add this code to main(): | ||
+ | <br /> | ||
+ | ::<source lang="java"> | ||
+ | public static void main(String[] args) { | ||
+ | String line = "ArtOfAssembly/CH17 248"; | ||
+ | |||
+ | String[] words = line.split( " " ); | ||
+ | System.out.println( "words[0] = " + words[0] ); | ||
+ | System.out.println( "words[1] = " + words[1] ); | ||
+ | } | ||
+ | </source> | ||
+ | <br /> | ||
+ | * Run it. | ||
+ | * Observe the output of the program. | ||
+ | * Sometimes it is worth creating a small java file to test something simple. This way we don't have to modify the main program we're working on. | ||
+ | * We are now ready to scan the list of lines, split each one into words, get the second word, transform it into an int, and if it is greater than 100, add the line to a new list. | ||
+ | |||
+ | <br /> | ||
+ | |||
+ | =Adding a Scan Method to Extract the Large Files= | ||
+ | <br /> | ||
+ | * Call a yet to be created method at the end of your main() function: | ||
+ | <br /> | ||
+ | ::<source lang="java" highlight="10-12"> | ||
+ | public static void main(String[] args) { | ||
+ | ArrayList lines = null; | ||
+ | |||
+ | // get lines from input | ||
+ | lines = readInput(); | ||
+ | |||
+ | // display input lines (for debugging) | ||
+ | //displayListOfLines( lines ); | ||
+ | |||
+ | // scan list and select lines with size > 100 | ||
+ | ArrayList largeFilesList = scan( lines); | ||
+ | displayListOfLines( largeFilesList ); | ||
+ | } | ||
+ | </source> | ||
+ | <br /> | ||
+ | * Follow Eclipse's recommendation to add the method '''scan( )'''. | ||
+ | * Here is an outline of the steps it must follow: | ||
+ | <br /> | ||
+ | :# <tt>create a new empty list.</tt> | ||
+ | :# <tt>for each line in the list of lines:</tt> | ||
+ | :## <tt>split the line into words,</tt> | ||
+ | :## <tt>if the number of words is not 2, skip this line,</tt> | ||
+ | :## <tt>get the second word and make it an int (with Integer.parseInt( ''string'' ) ),</tt> | ||
+ | :## <tt>if the int is greater than 100, add the line to the new list,</tt> | ||
+ | :# <tt>when done iterating over the lines, return the new list.</tt> | ||
+ | <br /> | ||
+ | * You know all the java parts of this algorithm. Go at it and code it! | ||
+ | <br /> | ||
+ | ==Expected Output== | ||
+ | <br /> | ||
+ | * Here's what your program should output: | ||
+ | <br /> | ||
+ | ::<source lang="text"> | ||
+ | ArtOfAssembly 10012 | ||
+ | ArtOfAssembly/CH01 276 | ||
+ | ArtOfAssembly/CH02 300 | ||
+ | ArtOfAssembly/CH03 480 | ||
+ | ArtOfAssembly/CH04 272 | ||
+ | ArtOfAssembly/CH05 328 | ||
+ | ArtOfAssembly/CH06 620 | ||
+ | ArtOfAssembly/CH07 148 | ||
+ | ArtOfAssembly/CH08 748 | ||
+ | ArtOfAssembly/CH09 436 | ||
+ | ArtOfAssembly/CH10 248 | ||
+ | ArtOfAssembly/CH11 436 | ||
+ | ArtOfAssembly/CH12 348 | ||
+ | ArtOfAssembly/CH13 524 | ||
+ | ArtOfAssembly/CH14 500 | ||
+ | ArtOfAssembly/CH15 380 | ||
+ | ArtOfAssembly/CH16 772 | ||
+ | ArtOfAssembly/CH17 248 | ||
+ | ArtOfAssembly/CH18 304 | ||
+ | ArtOfAssembly/CH19 612 | ||
+ | ArtOfAssembly/CH20 348 | ||
+ | ArtOfAssembly/CH21 200 | ||
+ | ArtOfAssembly/CH22 196 | ||
+ | ArtOfAssembly/CH24 352 | ||
+ | ArtOfAssembly/CH25 256 | ||
+ | </source> | ||
+ | <br /> | ||
+ | |||
+ | =Refactoring= | ||
+ | <br /> | ||
+ | ==Refactoring A Method Name== | ||
+ | * "Refactoring" with Eclipse means renaming something. But Eclipse is quite clever about renaming things. | ||
+ | * For example, the name we have given to our last method, '''scan''', is not very imaginative. Let's call it something more self-documenting, like '''scanLinesGreater100'''. | ||
+ | * Locate the call to '''scan''' in the main function. Control/Right click on the word "scan". | ||
+ | * In the menu that appears, select '''Refactor''', then '''Rename''': | ||
+ | <br /> | ||
+ | <center>[[Image:EclipseRefactorRename.png|600px]]</center> | ||
+ | <br /> | ||
+ | * A small box appears around the word '''scan'''. While the cursor is in this box, change the name, and press ENTER when done. | ||
+ | * Notice that Eclipse will have also changed the name of the method definition! Eclipse would also change all the calls to scan if we had had several classes in our project, and the method scan() had been called in them. | ||
+ | <br /> | ||
+ | |||
+ | ==Refactoring A Class Name== | ||
+ | <br /> | ||
+ | * Eclipse is also quite clever about file names and class names. | ||
+ | * Go back to the edit window for the '''TestSplit''' program. | ||
+ | * Refactor/rename the name of the class, from '''TestSplit''' to '''SplitString'''. | ||
+ | * Type '''Continue''' in the warning window that appears. | ||
+ | * When the refactoring is done, check that Eclipse changed not only the name of the class, but the name of the file containing it, as well. If you had included a constructor in this class, Eclipse would have changed its name as well. | ||
+ | <br /> | ||
+ | |||
+ | =Adding JavaDoc= | ||
+ | <br /> | ||
+ | * Let's document the Lab7a program. | ||
+ | * Put your cursor on the empty line, just above this line: | ||
+ | <br /> | ||
+ | ::<source lang="java"> | ||
+ | private static ArrayList scanLinesGreater100( ArrayList lines) { | ||
+ | </source> | ||
+ | <br /> | ||
+ | :and type '''/**''' aligned with the word '''private''' underneath. | ||
+ | * Press Enter. | ||
+ | * Notice that Eclipse added all the required field to the java doc. You just have to complete them! | ||
+ | <br /> | ||
+ | ::<source lang="java"> | ||
+ | /** | ||
+ | * get a list of lines with a file name followed by a size, and collect all the | ||
+ | * lines whose size is greater than 100. | ||
+ | * @param lines the list of lines | ||
+ | * @return a new list of lines where the sizes are all greater than 100. | ||
+ | */ | ||
+ | private static ArrayList scanLinesGreater100( ArrayList lines) { | ||
+ | </source> | ||
+ | <br /> | ||
+ | * Now put your cursor on the call to the method '''scanLinesGreater100()''' in '''main()'''. | ||
+ | * Just leave your cursor on the word. No need to click. | ||
+ | * After about a second, the javadoc for the method will open up. | ||
+ | * Neat, isn't it? | ||
+ | <br /> | ||
+ | ==Javadoc for Library Methods/Objects== | ||
+ | <br /> | ||
+ | * You can also get the javadocs for methods belonging to library objects. | ||
+ | * For example, put your cursor on the method '''split()''', and leave it there. You should see a description of the method you're using. | ||
+ | <br /> | ||
+ | |||
+ | =Your Assignment For This Lab= | ||
+ | <br /> | ||
+ | <!-- du -h wikipedia | awk '{print $2,$1;}' | sort --> | ||
+ | * The list of files and their sizes that we are using in this lab is generated by the command '''du''', for '''d'''isk '''u'''sage. It's a linux command that can be typed at the command line. | ||
+ | * To get a friendlier output, one can add the -h switch to the command. -h stands for '''h'''uman-readable. | ||
+ | * In this case the output of '''du''' will look something like this: | ||
+ | <br /> | ||
+ | ::<source lang="text"> | ||
+ | wikipedia/accessHistory 36K | ||
+ | wikipedia/gettreemap 84K | ||
+ | wikipedia/images/dewiki-images 590 | ||
+ | wikipedia/images/enwiki-images 98 | ||
+ | wikipedia/images/frwiki-images 85K | ||
+ | wikipedia/php 110 | ||
+ | wikipedia/php/images 78 | ||
+ | wikipedia/php/include 36 | ||
+ | wikipedia/php/include/images 104K | ||
+ | wikipedia/php/libs 504 | ||
+ | wikipedia/php/libs/internals 96K | ||
+ | wikipedia/php/libs/plugins 20M | ||
+ | </source> | ||
+ | <br /> | ||
+ | * Some of the numbers are suffixed with a '''K''', indicating '''Kilobyte''', or with an '''M''', indicating '''Megabyte'''. A Kilobyte is 1000 bytes (roughly), and a Megabyte, 1 million bytes. | ||
+ | * Write a new program called '''Lab7_2.java''', from scratch (do not copy paste the previous one, just use the same approach on your own), that will read these lines and print only the ones with a size larger than or equal to a 100 Kilobytes. | ||
+ | * You do not need to make your program actually read the lines from the input. Its '''readInput()''' method should simply store the list of lines shown above into an ArrayList, and return it. | ||
+ | * <font color="magenta">'''Hints''':</font> | ||
+ | :* You can access the last character of a string this way: | ||
+ | <br /> | ||
+ | :::<source lang="java"> | ||
+ | yourString.substring( yourString.length() - 1) ) | ||
+ | </source> | ||
+ | <br /> | ||
+ | ::and if you leave your cursor on the method ''substring()'' in your code, you will get its javadoc... | ||
+ | <br /> | ||
+ | :* Remember that to compare strings you need to use the '''.equals()''' method... | ||
+ | <br /> | ||
+ | ==Example Output== | ||
+ | <br /> | ||
+ | * Here is the output of the program Moodle expects: | ||
+ | |||
+ | wikipedia/php/include/images 104000 | ||
+ | wikipedia/php/libs/plugins 20000000 | ||
+ | |||
+ | <br /> | ||
+ | ==Submission to Moodle== | ||
+ | <br /> | ||
+ | * Add this line above the name of your class: | ||
+ | <br /> | ||
+ | |||
+ | @SuppressWarnings("unchecked") | ||
+ | |||
+ | <br /> | ||
+ | :This should prevent warnings from the compiler, and will prevent Moodle from thinking there's an error in your program. | ||
+ | |||
+ | * Submit your program to moodle. | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | [[Category:CSC212]][[Category:Java]][[Category:Labs]] | ||
+ | <br /> | ||
<br /> | <br /> |
Latest revision as of 10:50, 10 October 2014
--D. Thiebaut (talk) 14:47, 6 October 2014 (EDT)
Installing Eclipse
Installing On Windows
Follow the directions from this video and installe Eclipse and the Java JDK.
Installing on a Mac
- Follow the directions shown in this video:
- If you get a message "Eclipse cannot be opened because of an unidentified developer", go to System Preferences, Security and Privacy, and click on Open Anyway.
Developing Java Programs with Eclipse
This section of the lab assumes that you have installed Eclipse on your system. If not, then use one of the Linux Mint machines in the lab. For the purpose of discovering Eclipse and its amazing features, we will develop a program using the top-down approach. |
Problem of the Day
Write a program that reads a collection of lines from the standard input (either from the keyboard or redirected from a file). The lines contain a list of file names along with their size, expressed in Kilobytes. Here's an example of the type of input your program will receive:
./ArtOfAssembly 10012 ./ArtOfAssembly/CH01 276 ./ArtOfAssembly/CH02 300 ./ArtOfAssembly/CH03 480 ./ArtOfAssembly/CH04 272 ./ArtOfAssembly/CH05 328 ./ArtOfAssembly/CH06 620 ./ArtOfAssembly/CH07 148 ./ArtOfAssembly/CH08 748 ./ArtOfAssembly/CH09 436 ./ArtOfAssembly/CH10 248 ./ArtOfAssembly/CH11 436 ./ArtOfAssembly/CH12 348 ./ArtOfAssembly/CH13 524 ./ArtOfAssembly/CH14 500 ./ArtOfAssembly/CH14/org_gifs 72 ./ArtOfAssembly/CH15 380 ./ArtOfAssembly/CH16 772 ./ArtOfAssembly/CH16/chars 32 ./ArtOfAssembly/CH17 248 ./ArtOfAssembly/CH18 304 ./ArtOfAssembly/CH19 612 ./ArtOfAssembly/CH20 348 ./ArtOfAssembly/CH21 200 ./ArtOfAssembly/CH22 196 ./ArtOfAssembly/CH23 60 ./ArtOfAssembly/CH24 352 ./ArtOfAssembly/CH25 256 ./ArtOfAssembly/chars 16 ./ArtOfAssembly/fwd 68
The last line indicates that the file fwd in the directory ArtOfAssembly is 68 KBytes in size.
The Assignment is to keep track of all the files that are larger than 100 KBytes and store them in a list for later processing (for example because we may want to compress them).
Your program will print the list of the large files (>100K).
Top Level
Think for a minute about what your program needs to do:
1. get the input, one line at a time, 2. extract the size from the line. 3. if the size is larger than 100, add the file name and its size to a list 4. once the input has been completely read, output the list of the name and size of the large files
Ready? You are now going to write this program with Eclipse as your IDE (Integrated Development Environment).
Launch Eclipse
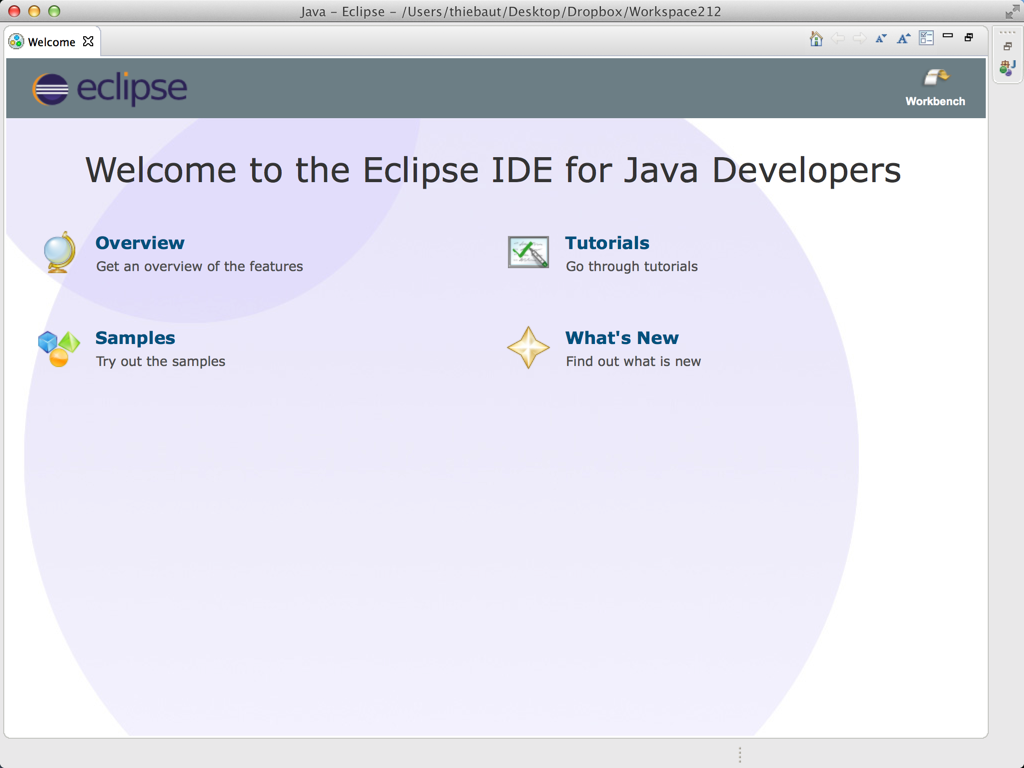
- Close the Welcome Window
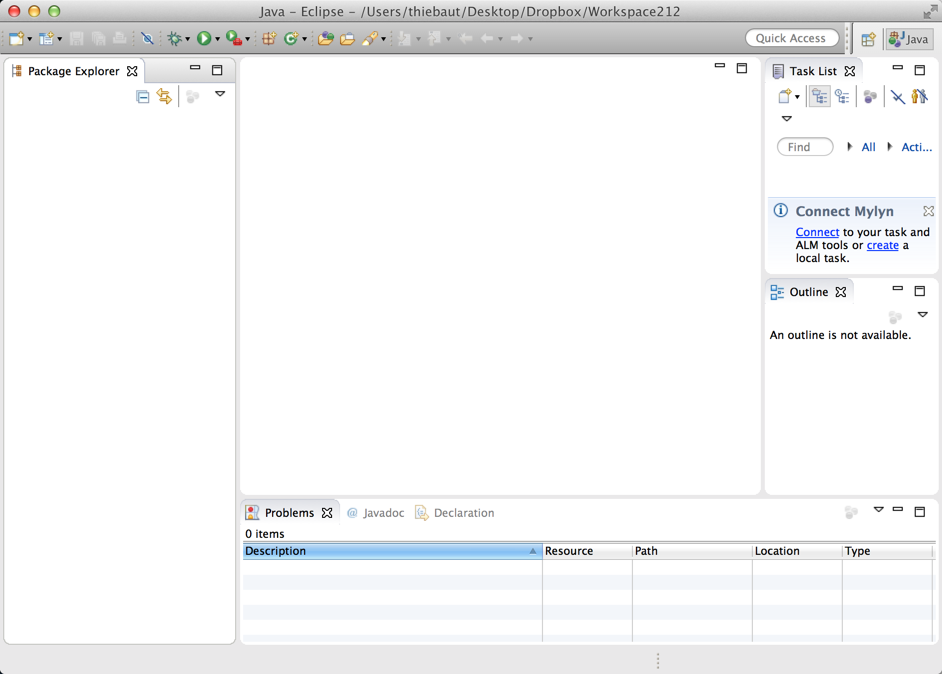
Create A Project
- Create a new Project. Eclipse organizes Java programs into project. Let's create a project called CSC212 where you'll store your Java file(s). You should have to do this only once this semester (unless you want to organize your files by, say, homework or lab sections).
- File
- New
- Java Project
- CSC212
- Finish
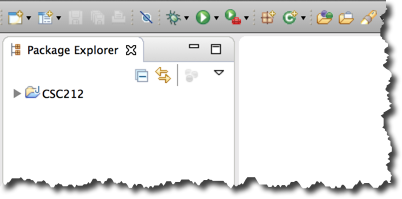
Create a new Java File
- Select CSC212
- Right-Click or Control-Click on it, depending on your type of machine, and pick
- File
- New
- Class
- Lab7a (don't add the .java extension)
- Click on public static void main( String[] args )
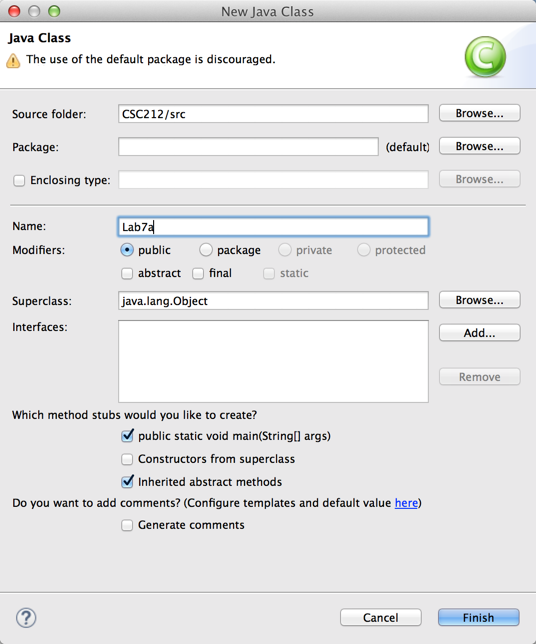
- Finish
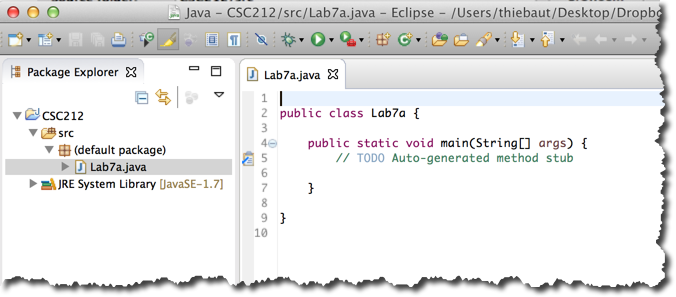
Reading Input
In the main program, declare an ArrayList called lines.
ArrayList lines = null;
- Notice that the ArrayList word is underlined in red, and there's a small red x in the left margin.
- Click on the red x. A menu of suggestions will appear. Usually the top suggestion will be the right one. Sometimes not, so make sure you read what it suggested as a fix:
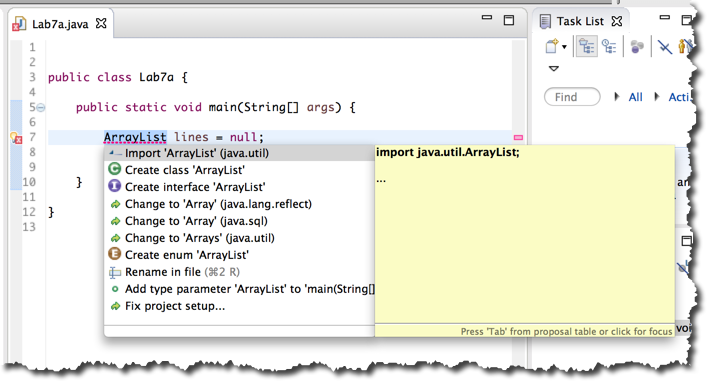
- Double-click the import 'ArrayList' (Java.util) suggestion. This will clear both the red marker, and the red underline.
- Add more code:
ArrayList lines = null; lines = readInput();
- Note the new red underline and cross. Click on the cross and accept for Eclipse to create a new method for you. You will get a new method in your code:
private static ArrayList readInput() { // TODO Auto-generated method stub return null; }
- Instead of making the method read from the standard input (i.e. the keyboard), make it create a list of string and return it:
private static ArrayList readInput() { ArrayList l = new ArrayList(); l.add( "ArtOfAssembly 10012" ); l.add( "ArtOfAssembly/CH01 276" ); l.add( "ArtOfAssembly/CH02 300" ); l.add( "ArtOfAssembly/CH03 480" ); l.add( "ArtOfAssembly/CH04 272" ); l.add( "ArtOfAssembly/CH05 328" ); l.add( "ArtOfAssembly/CH06 620" ); l.add( "ArtOfAssembly/CH07 148" ); l.add( "ArtOfAssembly/CH08 748" ); l.add( "ArtOfAssembly/CH09 436" ); l.add( "ArtOfAssembly/CH10 248" ); l.add( "ArtOfAssembly/CH11 436" ); l.add( "ArtOfAssembly/CH12 348" ); l.add( "ArtOfAssembly/CH13 524" ); l.add( "ArtOfAssembly/CH14 500" ); l.add( "ArtOfAssembly/CH14/org_gifs 72" ); l.add( "ArtOfAssembly/CH15 380" ); l.add( "ArtOfAssembly/CH16 772" ); l.add( "ArtOfAssembly/CH16/chars 32" ); l.add( "ArtOfAssembly/CH17 248" ); l.add( "ArtOfAssembly/CH18 304" ); l.add( "ArtOfAssembly/CH19 612" ); l.add( "ArtOfAssembly/CH20 348" ); l.add( "ArtOfAssembly/CH21 200" ); l.add( "ArtOfAssembly/CH22 196" ); l.add( "ArtOfAssembly/CH23 60" ); l.add( "ArtOfAssembly/CH24 352" ); l.add( "ArtOfAssembly/CH25 256" ); l.add( "ArtOfAssembly/chars 16" ); l.add( "ArtOfAssembly/fwd 68" ); return l; }
Testing Method readInput()
- It is a good idea to always test a new method once it is added to the code.
- Change main() as follows:
public static void main(String[] args) { ArrayList lines = null; // get lines from input lines = readInput(); // display input lines (for debugging) Iterator<String> it = lines.iterator(); while ( it.hasNext() ) { String line = (String) it.next(); System.out.println( line ); } }
- You will have noticed that Eclipse suggests code as you type. This is a nice feature.
- Run the program by clicking on the white triangle in a green circle in the top bar. If you get a window opening up asking you to select a resource to save, simply check the box Always save resource before launching, and click OK.
- Notice that a new tab opens up in the bottom pane for the Console output. Your output will be there. You can scroll it up or down.
Minimizing The Methods
- Now that we see that readInput() works according to plans, let's minimize it, as it is a very long method.
- Locate the small minus-sign in the margin, to the left of private static ArrayList readInput() {.
- Click on it. Notice that the code of readInput() disappears. It's still part of the method, but not shown in the editor to save space. You can get it "back" by clicking on the plus-sign.
Adding a Private Function
- The code to display the list of lines can be useful in other parts of our program. Let's make it a method.
- Change your main() function to this code:
public static void main(String[] args) { ArrayList lines = null; // get lines from input lines = readInput(); // display input lines (for debugging) displayListOfLines( lines ); Iterator<String> it = lines.iterator(); while ( it.hasNext() ) { String line = (String) it.next(); System.out.println( line ); } }
- Eclipse will suggest adding the method you just called. Just do it, and put the 4 lines of code required for printing the list inside the new method.
- Fix errors (small red crosses) that Eclipse points out to you, if any.
- Main should look like this:
public static void main(String[] args) { ArrayList lines = null; // get lines from input lines = readInput(); // display input lines (for debugging) displayListOfLines( lines ); }
- Check that everything works by running your code again.
A Side-Step: Testing the String.split() method
- Each line our program has to process is a String with a very simple format:
file-name integer
- We need to extract the integer part of this string. Let's take a side-step and do some testing in a different program.
- Right/Control click on (default package) in the Package Explorer pane.
- Click New, then class. Call it TestSplit and ask Eclipse to add a main() method.
- Add this code to main():
public static void main(String[] args) { String line = "ArtOfAssembly/CH17 248"; String[] words = line.split( " " ); System.out.println( "words[0] = " + words[0] ); System.out.println( "words[1] = " + words[1] ); }
- Run it.
- Observe the output of the program.
- Sometimes it is worth creating a small java file to test something simple. This way we don't have to modify the main program we're working on.
- We are now ready to scan the list of lines, split each one into words, get the second word, transform it into an int, and if it is greater than 100, add the line to a new list.
Adding a Scan Method to Extract the Large Files
- Call a yet to be created method at the end of your main() function:
public static void main(String[] args) { ArrayList lines = null; // get lines from input lines = readInput(); // display input lines (for debugging) //displayListOfLines( lines ); // scan list and select lines with size > 100 ArrayList largeFilesList = scan( lines); displayListOfLines( largeFilesList ); }
- Follow Eclipse's recommendation to add the method scan( ).
- Here is an outline of the steps it must follow:
- create a new empty list.
- for each line in the list of lines:
- split the line into words,
- if the number of words is not 2, skip this line,
- get the second word and make it an int (with Integer.parseInt( string ) ),
- if the int is greater than 100, add the line to the new list,
- when done iterating over the lines, return the new list.
- You know all the java parts of this algorithm. Go at it and code it!
Expected Output
- Here's what your program should output:
ArtOfAssembly 10012 ArtOfAssembly/CH01 276 ArtOfAssembly/CH02 300 ArtOfAssembly/CH03 480 ArtOfAssembly/CH04 272 ArtOfAssembly/CH05 328 ArtOfAssembly/CH06 620 ArtOfAssembly/CH07 148 ArtOfAssembly/CH08 748 ArtOfAssembly/CH09 436 ArtOfAssembly/CH10 248 ArtOfAssembly/CH11 436 ArtOfAssembly/CH12 348 ArtOfAssembly/CH13 524 ArtOfAssembly/CH14 500 ArtOfAssembly/CH15 380 ArtOfAssembly/CH16 772 ArtOfAssembly/CH17 248 ArtOfAssembly/CH18 304 ArtOfAssembly/CH19 612 ArtOfAssembly/CH20 348 ArtOfAssembly/CH21 200 ArtOfAssembly/CH22 196 ArtOfAssembly/CH24 352 ArtOfAssembly/CH25 256
Refactoring
Refactoring A Method Name
- "Refactoring" with Eclipse means renaming something. But Eclipse is quite clever about renaming things.
- For example, the name we have given to our last method, scan, is not very imaginative. Let's call it something more self-documenting, like scanLinesGreater100.
- Locate the call to scan in the main function. Control/Right click on the word "scan".
- In the menu that appears, select Refactor, then Rename:
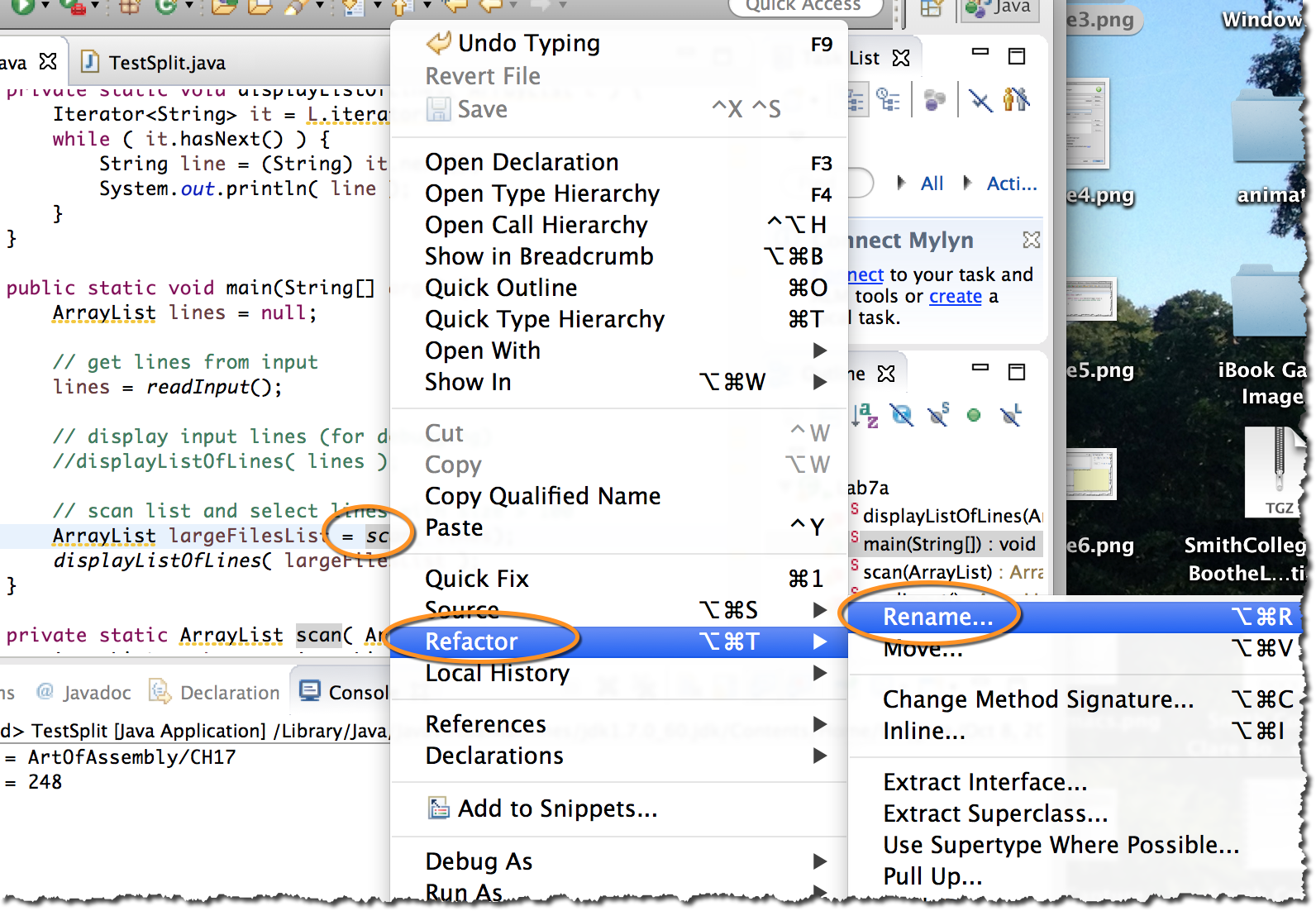
- A small box appears around the word scan. While the cursor is in this box, change the name, and press ENTER when done.
- Notice that Eclipse will have also changed the name of the method definition! Eclipse would also change all the calls to scan if we had had several classes in our project, and the method scan() had been called in them.
Refactoring A Class Name
- Eclipse is also quite clever about file names and class names.
- Go back to the edit window for the TestSplit program.
- Refactor/rename the name of the class, from TestSplit to SplitString.
- Type Continue in the warning window that appears.
- When the refactoring is done, check that Eclipse changed not only the name of the class, but the name of the file containing it, as well. If you had included a constructor in this class, Eclipse would have changed its name as well.
Adding JavaDoc
- Let's document the Lab7a program.
- Put your cursor on the empty line, just above this line:
private static ArrayList scanLinesGreater100( ArrayList lines) {
- and type /** aligned with the word private underneath.
- Press Enter.
- Notice that Eclipse added all the required field to the java doc. You just have to complete them!
/** * get a list of lines with a file name followed by a size, and collect all the * lines whose size is greater than 100. * @param lines the list of lines * @return a new list of lines where the sizes are all greater than 100. */ private static ArrayList scanLinesGreater100( ArrayList lines) {
- Now put your cursor on the call to the method scanLinesGreater100() in main().
- Just leave your cursor on the word. No need to click.
- After about a second, the javadoc for the method will open up.
- Neat, isn't it?
Javadoc for Library Methods/Objects
- You can also get the javadocs for methods belonging to library objects.
- For example, put your cursor on the method split(), and leave it there. You should see a description of the method you're using.
Your Assignment For This Lab
- The list of files and their sizes that we are using in this lab is generated by the command du, for disk usage. It's a linux command that can be typed at the command line.
- To get a friendlier output, one can add the -h switch to the command. -h stands for human-readable.
- In this case the output of du will look something like this:
wikipedia/accessHistory 36K wikipedia/gettreemap 84K wikipedia/images/dewiki-images 590 wikipedia/images/enwiki-images 98 wikipedia/images/frwiki-images 85K wikipedia/php 110 wikipedia/php/images 78 wikipedia/php/include 36 wikipedia/php/include/images 104K wikipedia/php/libs 504 wikipedia/php/libs/internals 96K wikipedia/php/libs/plugins 20M
- Some of the numbers are suffixed with a K, indicating Kilobyte, or with an M, indicating Megabyte. A Kilobyte is 1000 bytes (roughly), and a Megabyte, 1 million bytes.
- Write a new program called Lab7_2.java, from scratch (do not copy paste the previous one, just use the same approach on your own), that will read these lines and print only the ones with a size larger than or equal to a 100 Kilobytes.
- You do not need to make your program actually read the lines from the input. Its readInput() method should simply store the list of lines shown above into an ArrayList, and return it.
- Hints:
- You can access the last character of a string this way:
yourString.substring( yourString.length() - 1) )
- and if you leave your cursor on the method substring() in your code, you will get its javadoc...
- Remember that to compare strings you need to use the .equals() method...
Example Output
- Here is the output of the program Moodle expects:
wikipedia/php/include/images 104000 wikipedia/php/libs/plugins 20000000
Submission to Moodle
- Add this line above the name of your class:
@SuppressWarnings("unchecked")
- This should prevent warnings from the compiler, and will prevent Moodle from thinking there's an error in your program.
- Submit your program to moodle.