Difference between revisions of "CSC111 Lab 1 2011"
(→Python on the MAC) |
(→Python on the MAC) |
||
Line 19: | Line 19: | ||
* When prompted for an account and password, use the <b>111a-''xx''</b> account that will be given to you in the lab. | * When prompted for an account and password, use the <b>111a-''xx''</b> account that will be given to you in the lab. | ||
− | <font color="magenta">Note: if you are joining the class late and do not have a Linux account, <b>don't worry</b>, just use your Groupwise account and password, and you will be able to do the lab without problem</b>. However, the '''Idle''' program may not available on all the computers in the science center. If you cannot find it on the computer you are using, try going to Room 241 or 243 in Ford Hall. | + | ::<font color="magenta">Note: if you are joining the class late and do not have a Linux account, <b>don't worry</b>, just use your Groupwise account and password, and you will be able to do the lab without problem</b>. However, the '''Idle''' program may not available on all the computers in the science center. If you cannot find it on the computer you are using, try going to Room 241 or 243 in Ford Hall. |
</font> | </font> | ||
Revision as of 08:32, 11 September 2011
--D. Thiebaut 12:12, 6 September 2011 (EDT)
FUN WITH PYTHON
This lab is just an introduction to having fun with Python. It's purpose is to have you explore the different systems that are available to you, and get a sense of how to program in an "intuitive" fashion.
Python on the MAC
- If your computer is not on, turn it ON!
- When prompted for booting Mac or Windows, select the Mac
- When prompted for an account and password, use the 111a-xx account that will be given to you in the lab.
- Note: if you are joining the class late and do not have a Linux account, don't worry, just use your Groupwise account and password, and you will be able to do the lab without problem</b>. However, the Idle program may not available on all the computers in the science center. If you cannot find it on the computer you are using, try going to Room 241 or 243 in Ford Hall.
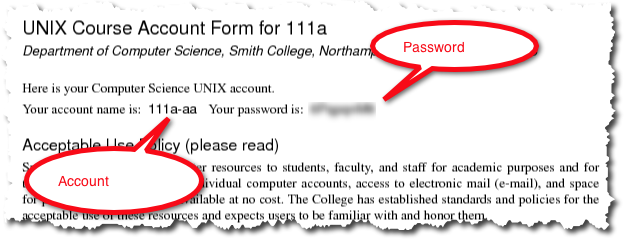
- Once you see the Desktop, click on the Finder (bottom left icon on the Desktop), and look in the Science Apps folder for Python 3.2.
- Start IDLE
Tip: if you can't find Idle, use the magnifying glass icon at the top right of the screen and search for idle and you should find it!
Idle
- Idle is the name of an editor one can use to create Python program on the Mac.
- If the window you start with looks like this (see below), then use the top menu and click File, New Window to open an editing window.
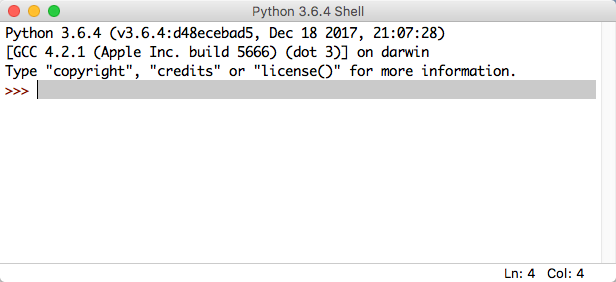
- You should now have two windows on your screen, one the editing window, the other the shell window, where the program will send its output.
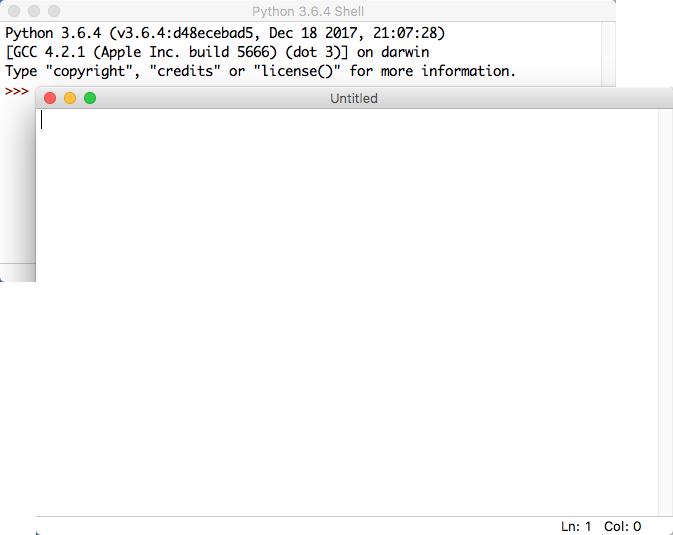
Some Python Code to play with
Playing with numbers
- Enter the following code in the Untitled window:
a = 3 b = 5 c = 10 print( a, b, c, a+b+c )
- Press F5 to run the program. When prompted to save the program, save it as lab1.py
- Look at the Console window. Some numbers appear... Do they make sense?
- change the numbers that are assigned to a, b, or c and see how your program reacts when you run it.
Using Strings of characters
- Modify the print statement so that it reads like this:
print (a, b, c, "sum = ", a+b+c )
- Run your new program. Do you understand how your modification made the program run differently? Does the output still make sense? Don't worry too much for today if it doesn't. Be sure to ask questions to your instructor or to the TAs if you're unsure about anything.
- Another modification. Replace your print statement by two statements:
print( a, b, c ) print( "sum = ", a+b+c )
- Run your program again. How does having a second print statement modify the behavior of your program?
Challenge #1 |
- Now, use your intuition and what you have just learned and see if you can modify your program to make it display this output:
a = 3 b = 10 c = 20 sum = 33
- Keep at it if you don't get there right away. Remember, this is a language, and you have many different ways to say the same thing...
Playing with Strings
- The goal of this section if for you to modify your program and try different Python statements and use intuition to understand how they work, and how the Python language is organized.
- Type the following three lines of code in your program (you can keep the old lines and add new lines at the end, or you can erase everything and type the new ones), and run the program.
print( "hello" ) print( "hello", "there!" ) print( "hello" + "there!" )
- Observe how the different ways to write the print statments influence how the information is printed.
- Now, try these lines of code:
word1 = "hello" word2 = "there!" print( word1, word2 ) print( word1, "who is", word2 )
- Question: What is the role of word1 or word2 in the program?
- Question: What is the role of the double quote character in Python?
Challenge #2 |
- Modify your program but keep the two variables word1 and word2, and make it print this:
hello there! there! hello hello hello hello hello there! there! hello
Repeating Strings
- Try this:
word1 = "hello"
print( word1 * 2 ) print( word1 * 5 ) print( word1 * 10 )
- See how you can multiply a string?
Challenge #3 |
- Modify your program and make it print this:
hello hello hello hello there! hello there! there! there! there! there! hello
Challenge #4 (tricky) |
- See if you can make your program print this, using string multiplication:
hellothere!hellothere!hellothere!hellothere!hellothere!
Python on Windows
- You will now continue the lab with Windows.
- Restart your computer, and pick Windows as the starting Operating System
- When prompted for an account and password, use the same 111a-xx account information as for the Mac. If this does not work, then use your regular Smith login information (the one you use to access your Smith mail).
- Look in the Start/Programs for Python 3.2 and Idle.
- Start Idle
Playing with Lists and Loops
- Create a new program in Idle with this new piece of code:
list = [ "Lea Jones", "Julie Fleur", "Anu Vias" ] for name in list: print( name )
Observe that the line that starts with print is indented from the line that starts with for. This is a very important detail: Python uses indentation to influence how statements depend on each other.
- Press F5. Observe the output.
Challenge #5 |
- Add three new names to the list and make your program output them all one above the other.
Repeating several statements
- Play with these different code segments, each one on its own. Make sure you understand how each one works when you execute the program.
list = [ "Lea Jones", "Julie Fleur", "Anu Vias" ] for name in list: print( name ) print( "-" )
- and this one:
list = [ "Lea Jones", "Julie Fleur", "Anu Vias" ] for name in list: print( name, len( name ) )
- or:
list = [ "Lea Jones", "Julie Fleur", "Anu Vias" ] for name in list: print( name ) print ( len( name ) )
- Still more
list = [ "Lea Jones", "Julie Fleur", "Anu Vias" ] for name in list: print( name, '-' * len( name ) )
Challenge #6 |
- Make your program display the names with a bar made of minus signs under each name. The length of the bar should be the same as the length of the name. For example:
Lea Jones --------- Julie Fleur ----------- Anu Vias --------
Challenge #7 |
- Similar question, but now like this:
--------- Lea Jones ---------
----------- Julie Fleur -----------
-------- Anu Vias --------
Challenge of the Day |
- Make your program display a box around each name:
------------- | Lea Jones | -------------
--------------- | Julie Fleur | ---------------
------------ | Anu Vias | ------------
- And... if you are done, and are really rolling and want some more of a challenge, try to make your program output this:
+-----------+ | Lea Jones | +-----------+
+-------------+ | Julie Fleur | +-------------+
+----------+ | Anu Vias | +----------+
Useful Links
- If you want to install Python 3 on your Mac or Windows laptop/desktop, check out this page and follow the link for the appropriate operating system.
- Once installed, you should be able to access Idle for the new version of Python:
- On a Mac: click on Finder, Applications, Python 3.2, and finally IDLE
- On Windows 7: click on Start, All Programs, Python 3.2, and finally IDLE (Python GUI).