Difference between revisions of "CSC111 Lab 11 2014"
(→Challenge 3: 2, random, 500) |
(→Create a Rectangle Class) |
||
Line 102: | Line 102: | ||
<br /> | <br /> | ||
− | = | + | =Building a Rectangle Class= |
+ | <br /> | ||
+ | Add a new class to your program using the code below. The class holds a rectangle defined by the coordinates of its top corner, its width and height, and its color defined as a triplet of integers in the range 0 to 255, included. | ||
+ | <br /> | ||
<source lang="python"> | <source lang="python"> | ||
from graphics import GraphicsWindow | from graphics import GraphicsWindow | ||
Line 133: | Line 136: | ||
rect2.draw( canvas ) | rect2.draw( canvas ) | ||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
def main(): | def main(): | ||
Line 151: | Line 142: | ||
canvas = win.canvas() | canvas = win.canvas() | ||
− | + | twoRects( canvas ) | |
− | |||
− | |||
win.wait() | win.wait() |
Revision as of 17:18, 15 April 2014
--D. Thiebaut (talk) 17:42, 13 April 2014 (EDT)
Contents
- 1 Creating the Graphics Library
- 2 Simple Test
- 3 Drawing circles
- 4 Building a Rectangle Class
- 5 Create a Circle Class
- 6 Create a Circle Class with a Center
- 7 Create a class Wheel that contains two concentric circles
- 8 Generate 200 random wheels on the canvas
- 9 Create a new Class that is a car
- 10 Create a car with a top
Creating the Graphics Library
The graphics library written by Horstmann, the author of our textbook is available here. Download it and create a file called graphics.py in your current directory.
Simple Test
Verify that your library is correctly installed by running the python program below. Call it lab10.py. Make sure to save it in the same directory where you saved graphics.py.
from graphicsH import GraphicsWindow
MAXWIDTH = 800
MAXHEIGHT = 600
def main():
win = GraphicsWindow(MAXWIDTH, MAXHEIGHT)
canvas = win.canvas()
canvas.setFill( 255, 0, 0 ) # full red
canvas.drawRect( 100, 100, 300, 200 )
win.wait()
win.close()
main()
Challenge 1: Two rectangle, random colors |
- Change the color of the rectangle.
- Add a second rectangle with a different color
- Make the color of the two rectangles random.
- Hints: to generate a random number between 0 and 255, you can do this:
# at the top of the program from random import seed from random import randrange # at the beginning of main() seed() # where you need a random number between 0 and 255 (included): x = randrange( 256 ):
Challenge 2: 500 random rects |
- Generate 500 random rectangles of random colors at random locations, and with random width and height.
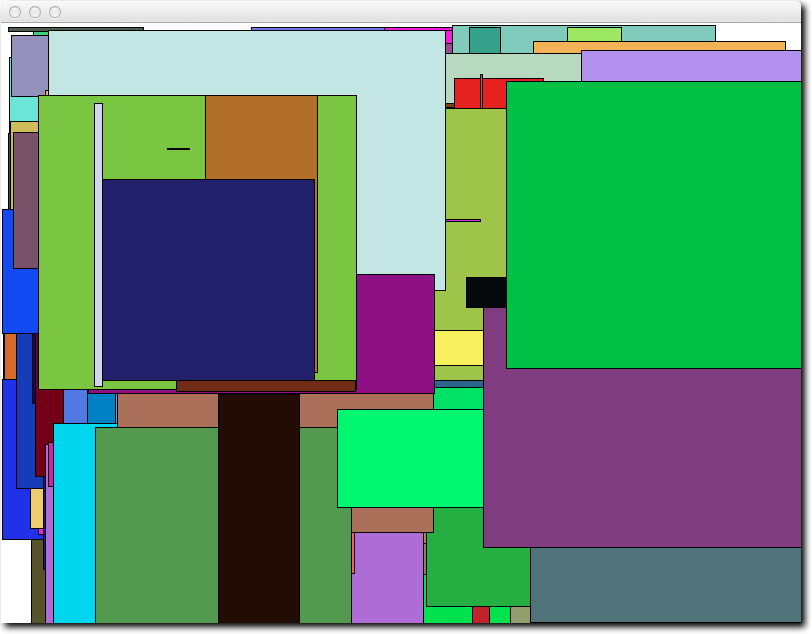
Drawing circles
The graphics library also supports circles with the method drawOval( x, y, w, h ), where x, and y represent to top corner of the invisible rectangle that contains the oval, and w and h represent the width and height of the invisible rectangle.
canvas.drawOval( x, y, w, h )
Challenge 3: 2, random, 500 |
- Make the program display two circles of random colors side by side, horizontally
- Make your program display touching circles covering the whole window (hints: use nested for loops!)
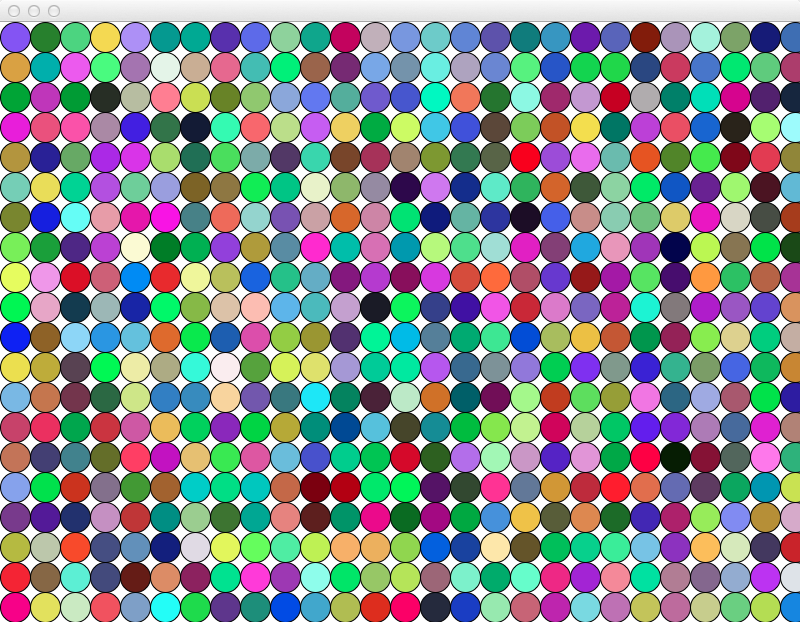
Building a Rectangle Class
Add a new class to your program using the code below. The class holds a rectangle defined by the coordinates of its top corner, its width and height, and its color defined as a triplet of integers in the range 0 to 255, included.
from graphics import GraphicsWindow
from random import randrange
from random import seed
from time import sleep
MAXWIDTH = 800
MAXHEIGHT= 600
class Rectangle:
def __init__( self, x, y, width, height, color ):
self._x = x
self._y = y
self._width = width
self._height = height
self._color = color # list of 3 ints between 0 and 255
def draw( self, canvas ):
canvas.setFill( self._color[0], self._color[1], self._color[2] )
canvas.drawRect( self._x, self._y, self._width, self._height )
def twoRects( canvas ):
rect1 = Rectangle( 100, 100, 500, 20, (255, 0, 0 ) )
rect1.draw( canvas )
rect2 = Rectangle( 200, 200, 50, 200, (165, 76, 89 ) )
rect2.draw( canvas )
def main():
seed()
win = GraphicsWindow(MAXWIDTH, MAXHEIGHT)
canvas = win.canvas()
twoRects( canvas )
win.wait()
win.close()
main()
Create a Circle Class
Use the example of Rectangle
Create a Circle Class with a Center
Modify the class so that the x and y passed are the x and y of the center of the circle.
Create a class Wheel that contains two concentric circles
The inside circle will be concentric with the outside one, and will always have a radius half the radius of the large one.
Generate 200 random wheels on the canvas
Create a new Class that is a car
- one rectangle
- two wheels
- x, y of top left corner of rectangle
- width and height of rectangle.
- center of 1st wheel 1/4 width from left of car
- center of 2nd wheel 1/4 width from right of car
- radius of wheel = 1/8 of car width