|
|
Line 190: |
Line 190: |
| |- | | |- |
| | | | | |
− | ADDXm [''address'']
| + | ADDX [''address''] |
| | | | | |
| 30 | | 30 |
Revision as of 19:57, 27 August 2014
--D. Thiebaut (talk) 16:57, 26 August 2014 (EDT)
This page documents all the instructions that are supported by the Simple Computer Simulator shown below. You can click on the image to go to the Javascript simulator. This simulator is used in the CSC103 How Computers Work course at Smith College.
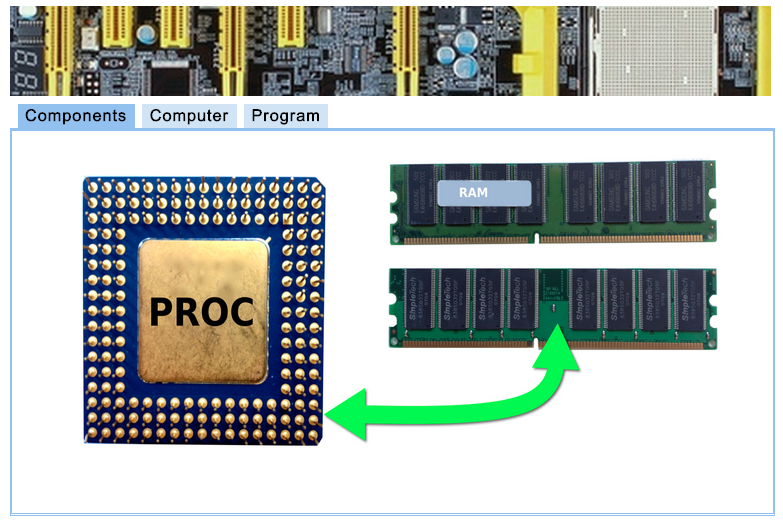
Simple Computer Simulator
Instructions Using the Accumulator and a Number
These instructions operate with a single number (we refer to them as constants) that is either loaded into, added, or subtracted from the accumulator register.
Instruction
|
Code (decimal)
|
Code ( binary)
|
Description
|
ADD number
|
24
|
00011000
|
- This instruction adds the number to the one already in the accumulator. For example, if the accumulator register already contains 10, and the processor executes ADD 3 the result is that the accumulator will contain 13 after the instruction.
|
COMP number
|
84
|
01010100
|
- This instruction compares the number to the one already in the accumulator. For example, if the accumulator register already contains 10, and the processor executes COMP 3 the result is the comparison of 10 to 3. 10 is greater, and is not equal to 3. This will prevent a JLT (jump if less than) to jump to its target, and will prevent a JEQ instruction from jumping. On the other hand, if the accumulator had contained 2, then a COMP 2 would have allowed a subsequent JEQ instruction to jump to its target.
|
DIV number
|
44
|
00101100
|
- This instruction divides the contents of the accumulator by number, and keeps the integer part of the result. For example, if the accumulator register already contains 10, and the processor executes DIV 4 the result is that the accumulator will contain 2 after the instruction.
|
LOAD number
|
4
|
00000100
|
- This instruction puts the number into the accumulator. Whatever was in the accumulator prior to the operation is lost. , if the accumulator register already contains 10, and the processor executes ADD 3 the result is that the accumulator will contain 13 after the instruction.
|
MUL number
|
40
|
00101000
|
- This instruction multiplies the contents of the accumulator by number, and replaces the contents of the accumulator by the result. For example, if the accumulator register already contains 10, and the processor executes MUL 4 the result is that the accumulator will contain 40 after the instruction.
|
SUB number
|
32
|
00100000
|
- This instruction subtracts the number from the one already in the accumulator. For example, if the accumulator register already contains 10, and the processor executes SUB 3 the result is that the accumulator will contain 7 after the instruction.
|
Instructions Using the Accumulator and Memory
These instructions are followed by a number in brackets. This number refers to the location, or address in memory where the actual operand is located. So, if the number following the instruction is [100], it means that the instruction will use whatever number is stored at 100. A simple analogy might help here. Think of the difference between these two statements:
- "Please read the book The Little Prince."
and the statement
- "Please read the book from the library, with Call Number PQ2637.A274 P4613 2000".
Both statements refer to reading the same book. The first one refers to the book directly. The instructions in the section above operate similarly with their operands. The second statement refers to the book indirectly, by giving you its address in the library. The instructions in this section perform the same way. By putting brackets around the numbers that follow the instructions, we indicate that the numbers used are not the actual numbers we want to combine with the accumulator, but the address of the cells where we will find the numbers of interest.
This may still be a bit obscure, but read on the description for each instruction and this will hopefully become a bit clearer.
Instruction
|
Code (decimal)
|
Code ( binary)
|
Description
|
ADD [address]
|
26
|
00011010
|
- This instruction is similar to ADD number, except that now the number to add to the accumulator is located in the memory at the address specified in the instruction. For example, if the memory cell at Address 20 contains 4, and if the accumulator contains 10, then the instruction ADD [20] adds 4 to 10, resulting in 14, which becomes the new contents of the accumulator.
|
COMP [address]
|
86
|
01010110
|
- This instruction is similar to COMP number, except that now the number which is compared to the accumulator is located in the memory at the address specified in the instruction. For example, if the memory cell at Address 20 contains 4, and if the accumulator contains 10, then the instruction COMP [20] compares 10 to 4.
|
DIV [address]
|
46
|
00101110
|
- This instruction is similar to DIV number, except that now the the accumulator is divided by the number located in the memory at the address specified in the instruction. For example, if the memory cell at Address 20 contains 4, and if the accumulator contains 10, then the instruction DIV [20] divides 10 by 4, which results in 2. Fractional parts are not kept by our processor. In fact this is true also of real processors such as Intel's Pentium: only integers are stored in registers. Numbers with a decimal part require more sophisticated instructions and binary systems. This is beyond what we want to explore in this course.
|
LOAD [address]
|
6
|
00000110
|
- This instruction loads the number stored in memory at the address specified in the instruction, and puts it in the accumulator. For example, if the memory cell at Address 20 contains 4, and if the accumulator contains 10, then the instruction LOAD [20] replaces 10 in the accumulator by the number 4.
|
MUL [address]
|
42
|
00101010
|
- This instruction multiplies the contents of the accumulator by the number stored in memory at the specified address. For example, if the memory cell at Address 20 contains 4, and if the accumulator contains 10, then the instruction MUL [20] replaces the contents of the accumulator by 4 x 10, or 40.
|
STORE [address]
|
18
|
00010010
|
- This instruction makes a copy of the contents of the accumulator and stores it in memory at the address specified. For example, if the memory cell at Address 20 contains 4, and if the accumulator contains 10, then the instruction STORE [20] replaces the contents of the memory cell at Address 20 with the number 10. The accumulator value does not change.
|
SUB [address]
|
34
|
00100010
|
- This instruction subtracts the number stored in memory at the specified address from the number stored in the accumulator. For example, if the memory cell at Address 20 contains 10, and if the accumulator contains 40, then the instruction SUB [20] replaces the contents of the accumulator with 40 - 10, or 30.
|
Instructions Manipulating the Index Register
The Index, IX in the simulator, is a register in the processor that contains numbers, just as the Accumulator does. However, the numbers in the index represent addresses of cells containing numbers. The Index is useful is situations where we have several numbers in consecutive memory locations, and we want to perform the same operation on each one, say add 1 to each of the numbers. In this case we store in the Index the address of the first number, say, 100, and operate on that number through the Index. We'll need new instructions for that, but for right now we just want to see how we can load numbers in the index.
Instruction
|
Code (decimal)
|
Code ( binary)
|
Description
|
ADDX number
|
28
|
00011100
|
- This instruction adds a number to the contents of the Index register. For example, if the Index register contains the number 10, then ADDX 1 will add 1 to 10, and the Index will contain 11 after the instruction is executed.
|
ADDX [address]
|
30
|
00011110
|
- This instruction is similar to the one above, except that the value that is added to the contents of IX comes from a memory cell whose address is given in the instruction. For example, if IX contains 10, and the memory cell at Address 20 contains 5, then ADDX [20] will result in replacing the contents of IX with 10+5, or 15.
|
LOADX number
|
8
|
00001000
|
- This instruction sets the contents of the Index to the number. For example, LOADX 10 will result in the number 10 appearing inside the Index register.
|
STOREX [address]
|
22
|
00010110
|
- This instruction makes a copy of the contents of the Index register and saves it in a cell whose address is the one specified in the instruction. If IX contains 10, then the instruction STOREX [20] will copy the number 10 in the memory cell at Address 20. This does not affect the contents of IX. It does not get change by the copy operation.
|
SUBX number
|
36
|
00100100
|
- This instruction subtracts a number from the contents of the Index register. For example, if the Index register contains the number 10, then SUBX 1 will subtract 1 from 10, and the Index will contain 9 after the instruction is executed.
|
SUBX [address]
|
38
|
00100110
|
- Similarly to the way ADDX [address], this instruction takes the quantity found in memory at the location specified by address and subtracts it from the contents of the IX register.
|
Other
Instruction
|
Code (decimal)
|
Code ( binary)
|
Description
|
ADDXx
|
29
|
00011101
|
|
ADDx
|
25
|
00011001
|
|
COMPX
|
92
|
01011100
|
|
COMPXm
|
94
|
01011110
|
|
COMPXx
|
93
|
01011101
|
|
COMPx
|
85
|
01010101
|
|
DIVx
|
45
|
00101101
|
|
HALT
|
127
|
01111111
|
|
JEQ
|
68
|
01000100
|
|
JLT
|
72
|
01001000
|
|
JUMP
|
64
|
01000000
|
|
LOADXm
|
10
|
00001010
|
|
LOADXx
|
9
|
00001001
|
|
LOADx
|
5
|
00000101
|
|
MULx
|
41
|
00101001
|
|
NOP
|
0
|
00000000
|
|
STOREXx
|
21
|
00010101
|
|
STOREx
|
17
|
00010001
|
|
SUBXx
|
37
|
00100101
|
|
SUBx
|
33
|
00100001
|
|
TAX
|
79
|
01001111
|
|
TXA
|
83
|
01010011
|
|