Difference between revisions of "Debugging with Eclipse: A Quick Introduction"
(Created page with "--~~~~ ---- __TOC__ <br /> <bluebox> This is a short tutorial on how to get started with the Eclipse Debugger. Mastering the debugger takes time. The purpose of this lab/tu...") |
(→Getting Started) |
||
Line 14: | Line 14: | ||
* Create a '''New''' '''Java Class'''. Call it '''DebugDemo'''. | * Create a '''New''' '''Java Class'''. Call it '''DebugDemo'''. | ||
* Enter this code in it: | * Enter this code in it: | ||
+ | <br /> | ||
::<source lang="java"> | ::<source lang="java"> | ||
+ | // DebugDemo.java | ||
+ | // D. Thiebaut | ||
+ | // CSC212 | ||
import java.util.ArrayList; | import java.util.ArrayList; | ||
import java.util.Iterator; | import java.util.Iterator; | ||
Line 52: | Line 56: | ||
* Observe what the program does. It simply creates an array of 2 pairs, containing Alice(10) and Bob(7), and modify the age of both by an increment of 3. | * Observe what the program does. It simply creates an array of 2 pairs, containing Alice(10) and Bob(7), and modify the age of both by an increment of 3. | ||
<br /> | <br /> | ||
+ | |||
=Debugging DebugDemo= | =Debugging DebugDemo= | ||
<br /> | <br /> |
Revision as of 09:44, 22 October 2014
--D. Thiebaut (talk) 10:44, 22 October 2014 (EDT)
Contents
This is a short tutorial on how to get started with the Eclipse Debugger. Mastering the debugger takes time. The purpose of this lab/tutorial is just to show you how to get started.
Getting Started
- Open Eclipse
- Create a New Java Class. Call it DebugDemo.
- Enter this code in it:
// DebugDemo.java // D. Thiebaut // CSC212 import java.util.ArrayList; import java.util.Iterator; class Pair { public String name; public int age; Pair( String f, int s ) { name=f; age=s; } public String toString() { return name+"("+age+") "; } } public class DebugDemo { static ArrayList<Pair> A = null; public static void main(String[] args) { A = new ArrayList<Pair>(); int increment = 3; A.add( new Pair( "Alice", 10 ) ); A.add( new Pair( "Bob", 7 ) ); Iterator<Pair> it = A.iterator(); while ( it.hasNext() ) { Pair p = it.next(); p.age += increment; } for ( int i=0; i<3; i++ ) { Pair p = A.get( i ); System.out.println( A.get( i ) ); } } }
- Run it.
- Observe what the program does. It simply creates an array of 2 pairs, containing Alice(10) and Bob(7), and modify the age of both by an increment of 3.
Debugging DebugDemo
- In the Package Explorer tab (left tab), right/control click on DebugDemo and select Debug As and Java Application.
- The windows will reorganize themselves:
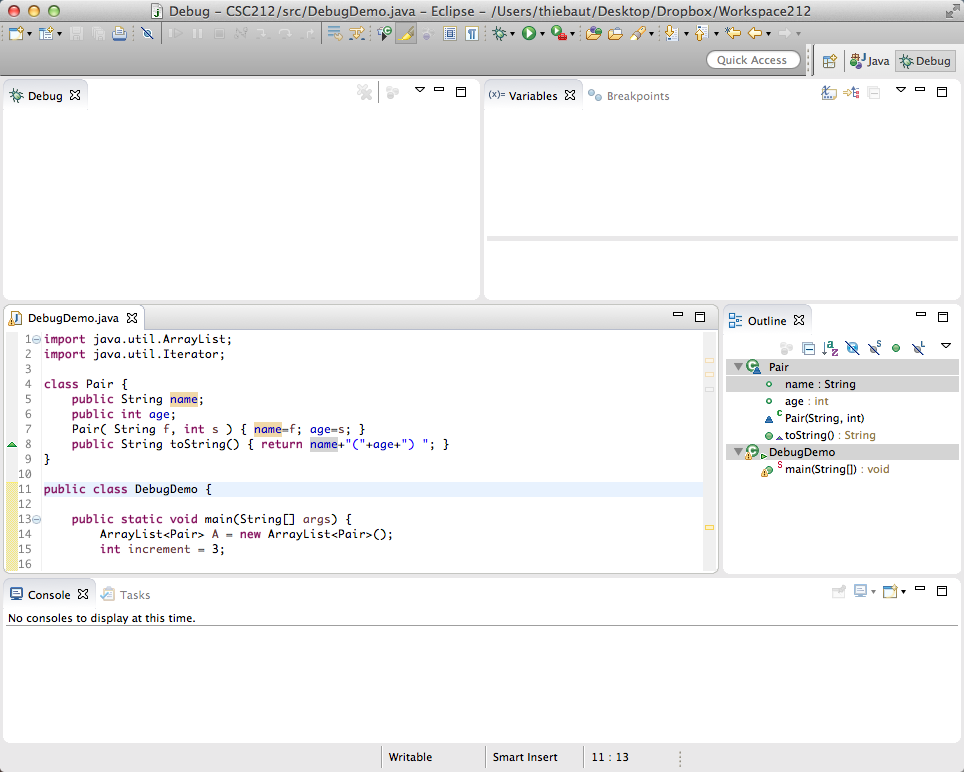