Difference between revisions of "CSC111 Homework 10 2015"
Line 4: | Line 4: | ||
<!--center> <font size="+2">Page under construction!</font> </center> <P> <center> [[File:UnderConstruction.jpg|300px]] </center--> | <!--center> <font size="+2">Page under construction!</font> </center> <P> <center> [[File:UnderConstruction.jpg|300px]] </center--> | ||
+ | <showafterdate after="20150408 12:00" before="20150601 00:00"> | ||
<br /> | <br /> | ||
<bluebox> | <bluebox> | ||
Line 92: | Line 93: | ||
<br /> | <br /> | ||
<br /> | <br /> | ||
+ | </showafterdate> | ||
+ | |||
+ | <!-- ================================================================= --> | ||
+ | <!-- ================================================================= --> | ||
+ | <!-- ================================================================= --> | ||
+ | <!-- ================================================================= --> | ||
<showafterdate after="20150415 00:00" before="20150601 00:00"> | <showafterdate after="20150415 00:00" before="20150601 00:00"> |
Revision as of 15:40, 5 April 2015
--D. Thiebaut (talk) 20:19, 4 April 2015 (EDT)
<showafterdate after="20150408 12:00" before="20150601 00:00">
This assignment is due Tuesday night (4/14/15) at 11:55 p.m. You will need to submit your code as well as a copy of the graphic window to Moodle. Programs that use graphics cannot be automatically tested on Moodle, so your program will be graded by hand by inspecting the image and code submitted. You will be able to run your program on Moodle. This will run a quick analysis of your program and will indicate whether your code contains important features (nested for-loops, for example).
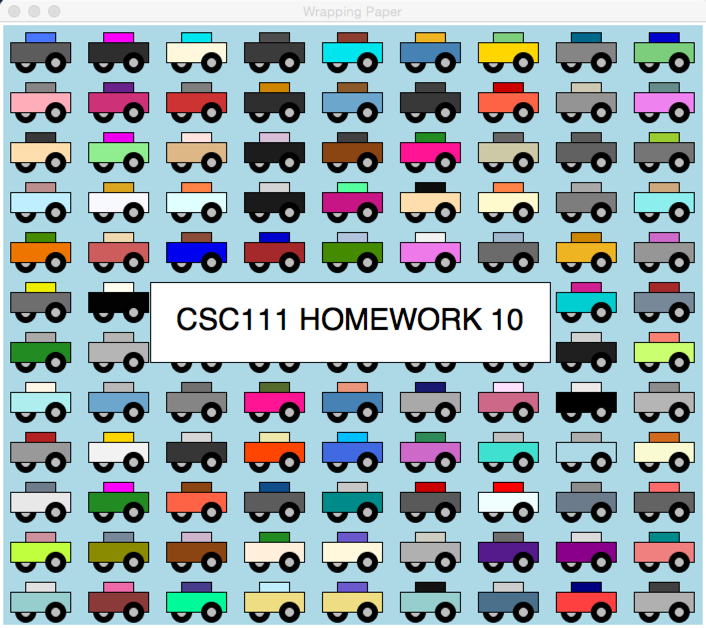
Contents
Problem 1
You have been hired for the summer by a company that makes wrapping paper. The company has a Web form where customers can pick different colors, a general theme, and a message to print in the middle of the page. Then a Python program generates an image that is printed by a large format printer.
The image above shows one particular output, for somebody who has selected Cars as the theme, Blue as the background, and "CSC111 HOMEWORK 10" as the message.
Your assignment
You must demonstrate that you should be hired for the programming job by writing a program that generates a sample output, with a predefined color, a predefined scheme, and a predefined message. Your program will NOT ask the user for any information, but instead will generate the information internally, on its own.
Submission
You must submit 2 files on Moodle:
- Your program, which should be called hw10_1.py
- An image of the output generated by your program. It should be called hw10_1.png or hw10_1.jpg, depending on how you capture the image. You can find information on the Web on ways to capture a window on your desktop to an image. Here are just a couple links that should be useful:
Requirements
- Your program should print the cars using two nested for-loops. Programs that create the cars using code not repeated by loops will be given a low grade. Your window should have at least 6 cars per row, and at least 6 rows of cars.
- The cars are not moving. They are generated at a particular location, and do not move. Your program does not need an animation loop.
- The color of the cars should be randomly generated.
- Your program organizes the cars in rows, one above the other. The car may or may not align vertically (the image above shows them aligned vertically).
- You are free to design the shape of your car. It must contain at least 2 rectangles (or other shapes) and at least two wheels.
- A given car can have differently colored shapes, or the same color for all its shapes. It is your design decision.
- Cars are not allowed to extend past the boundaries of the window.
- The message in the middle should appear inside a rectangle that hides the cars underneath it. It is fine to have cars partially covered by the box containing the message the message.
- The message should be centered in the rectangle.
- Adjust the font size so that the message is large enough to be easily readable.
- The screen capture of the image should show the sides and top of the window that contains the image, so that it can be easily verified that the cars are not cut at the edges of the window.
- The actual text of the message should be your name, consisting of your first name, and your last name. This way, the images can be easily assigned to you and accordingly graded.
- Your program should have a main() function defined at the end, and one final call to main() at the end of the program.
- The image you submit should not show a "click to stop" or "click to start" message.
Hints
- The document Graphic Module Reference, authored by Zelle, provides all the information you need for figuring out:
- How to set the background of the window
- How to set the size, or face (family), of the font used to display the message.
- Do not hesitate to give your classes additional methods that can make your life easier in your main program.
- The colors available can be found on this page.
Submission
Remember that you must submit 2 files, the program and a screen capture of your window.
The submission URL is http://cs.smith.edu/~thiebaut/111b/submit11.php
Problem 2
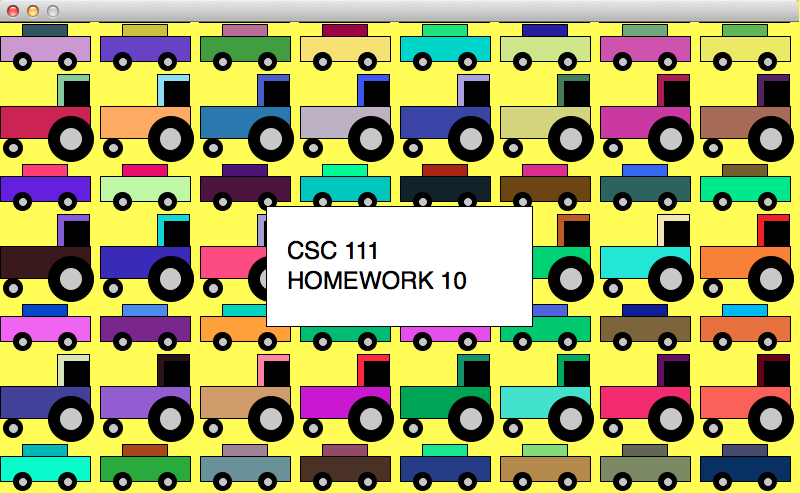
- This second problem is similar to Problem 1, but this time you are required to use two different types of cars. They should be different enough to be easily distinguishable from one another.
- The program must generate rows of cars, alternating between the two types of car. The first row should have cars of Type 1, the second row cars of Type 2, and will continue alternating between the two.
- The program must use nested for-loops to display the rows of cars.
- You need to submit two files on Moodle, a python program called hw10_2.py and a screen copy of your image, called hw10_2.jpg or hw10_2.png (all lower-case), depending on how you captured your window.
- The image above shows the caption displayed on two lines. For your program, make it display your name, on one line, as for Problem 1.
Fancy Cars
Do not hesitate to explore the graphics library and generate more sophisticated cars!
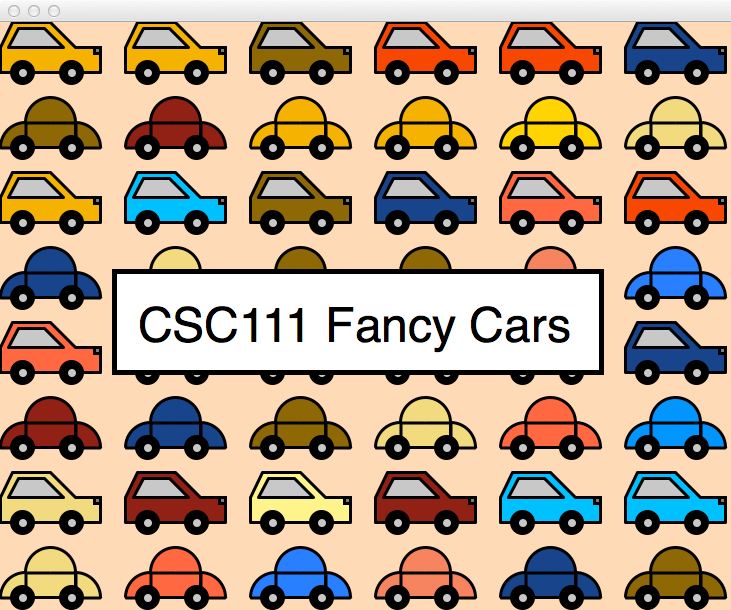
</showafterdate>
<showafterdate after="20150415 00:00" before="20150601 00:00">
Solution Programs
Part 1
# wheel1.py # D. Thiebaut # a skeleton program to get started with the graphics # from graphics import * import random import tkcolors # define geometry (constants) WIDTH = 700 HEIGHT = 600 class Wheel: """a Wheel is 2 concentric circles, the larger one black, the smaller one grey.""" def __init__(self, centr, rad1, rad2 ): # make circ1 the smaller of the 2 circles self.circ1 = Circle( centr, min( rad1, rad2 ) ) self.circ2 = Circle( centr, max( rad1, rad2 ) ) self.circ1.setFill( "grey" ) self.circ2.setFill( "black" ) def draw( self, win ): self.circ2.draw( win ) self.circ1.draw( win ) def move( self, dx, dy ): self.circ1.move( dx, dy ) self.circ2.move( dx, dy ) class Car: """a class containing a rectangle and 2 wheels, forming a simple car""" def __init__( self, refPoint ): # create rectangle self.width = 60 self.height = 20 self.radius = 10 P1 = refPoint xP1 = P1.getX() yP1 = P1.getY() xP2 = xP1 + self.width yP2 = yP1 + self.height P2 = Point( xP2, yP2 ) self.body = Rectangle( P1, P2 ) self.body.setFill( random.choice( tkcolors.colors ) ) P3 = Point( xP1+self.width//4, yP1 ) P4 = Point( xP1+self.width*3//4, yP1-self.height//2 ) self.top = Rectangle( P3, P4 ) self.top.setFill( random.choice( tkcolors.colors ) ) # create the Wheels self.w1 = Wheel( Point( xP1+self.width//4, yP1+self.height ), self.radius//2, self.radius ) self.w2 = Wheel( Point( xP1++self.width*3//4, yP1+self.height ), self.radius//2, self.radius ) # set the initial speed self.dx = 1 self.dy = 0 def setSpeed( self, deltaX, deltaY ): self.dx = deltaX self.dy = deltaY def draw( self, win ): self.w1.draw( win ) self.body.draw( win ) self.w2.draw( win ) self.top.draw( win ) def autoMove( self ): self.w1.move( self.dx, self.dy ) self.w2.move( self.dx, self.dy ) self.body.move( self.dx, self.dy ) def reverseDirection( self ): self.dx = -self.dx def getX( self ): return self.body.getP1().getX() #---------------------------------------------------------------- def waitForClick( win, message ): """ waitForClick: stops the GUI and displays a message. Returns when the user clicks the window. The message is erased.""" # wait for user to click mouse to start startMsg = Text( Point( win.getWidth()/2, win.getHeight()/2 ), message ) startMsg.draw( win ) # display message win.getMouse() # wait startMsg.undraw() # erase def main(): global W, H win = GraphWin( "Wrapping Paper", WIDTH, HEIGHT ) win.setBackground( "lightblue" ) #waitForClick( win, "click to start" ) cars = [] for x in range( 10, WIDTH, 78 ): for y in range( 20, HEIGHT, 50 ): car1 = Car( Point( x, y ) ) car1.draw( win ) cars.append( car1 ) Banner = Rectangle( Point( WIDTH//2-200, HEIGHT//2-40 ), Point( WIDTH//2+200, HEIGHT//2+40 ) ) Banner.setFill( "white" ) Banner.draw( win ) BannerText = Text( Point( WIDTH//2, HEIGHT//2 ), "CSC111 HOMEWORK 10" ) BannerText.setSize( 30 ) BannerText.draw( win ) waitForClick( win, "" ) win.close() main()
Part 2
# homework 10 # D. Thiebaut from graphics import GraphicsWindow from random import seed from random import randrange MAXWIDTH = 800 MAXHEIGHT = 600 class Circle2: def __init__( self, x, y, diameter, color ): self._x = x self._y = y self._diameter= diameter self._color = color # list of 3 ints between 0 and 255 def draw( self, canvas ): xAnchor = self._x-self._diameter//2 yAnchor = self._y-self._diameter//2 canvas.setFill( self._color[0], self._color[1], self._color[2] ) canvas.drawOval( xAnchor, yAnchor, self._diameter, self._diameter ) class Wheel: def __init__( self, x, y, diameter ): self._c1 = Circle2( x, y, diameter, ( 0,0,0 ) ) self._c2 = Circle2( x, y, diameter/2, ( 200, 200, 200 ) ) def draw( self, canvas ): self._c1.draw( canvas ) self._c2.draw( canvas ) class Rectangle: def __init__( self, x, y, width, height, color ): self._x = x self._y = y self._width = width self._height = height self._color = color # list of 3 ints between 0 and 255 def setRandomColor( self ): r = randrange( 256 ) g = randrange( 256 ) b = randrange( 256 ) self._color = (r, g, b ) def draw( self, canvas ): canvas.setFill( self._color[0], self._color[1], self._color[2] ) canvas.drawRect( self._x, self._y, self._width, self._height ) class Car: def __init__( self, x, y, width, height, color ): self._x = x self._y = y self._width = width self._height = height self._color = color # list of 3 ints between 0 and 255 # build the body self._top = Rectangle( x+width//4, y-height//2, width//2, height//2, color ) self._body = Rectangle( x, y, width, height, color ) # build the wheels self._w1 = Wheel( x + width//4, y + height, width//5 ) self._w2 = Wheel( x + 3*width//4, y + height, width//5 ) def setRandomColor( self ): self._top.setRandomColor() self._body.setRandomColor() def getTotalHeight( self ): return self._height*2 + self._width//10 def getTotalWidth( self ): return self._width def draw( self, canvas ): self._body.draw( canvas ) self._top.draw( canvas ) self._w1.draw( canvas ) self._w2.draw( canvas ) class Truck: def __init__( self, x, y, width, color ): self._x = x self._y = y self._width = width height = 5 * width//14 self._height = height self._color = color # list of 3 ints between 0 and 255 # build the body self._top = Rectangle( x+ 9* width//14, y-height, width*5//14, height, color ) self._window = Rectangle( x+ 10* width//14, y-4*height//5, width*4//14, 4*height//5, (0, 0, 0 ) ) self._body = Rectangle( x, y, width, height, color ) # build the wheels self._w1 = Wheel( x + 2*width//14, y + 13*height//10, 3*height//5 ) self._w2 = Wheel( x + 11*width//14, y + height, width//2 ) def getTotalHeight( self ): return self._height*2 + self._width//2 def getTotalWidth( self ): return self._width def setRandomColor( self ): self._top.setRandomColor() self._body.setRandomColor() def draw( self, canvas ): self._body.draw( canvas ) self._top.draw( canvas ) self._window.draw( canvas ) self._w1.draw( canvas ) self._w2.draw( canvas ) def main(): seed() win = GraphicsWindow(MAXWIDTH, MAXHEIGHT) canvas = win.canvas() canvas.setBackground( 0, 250, 250 ) carWidth = 90 carHeight = 25 oddEven = True for y in range( 0, MAXHEIGHT, carHeight*2 ):#+20 ): for x in range( 0, MAXWIDTH, carWidth+10 ): if oddEven: c = Car( x, y, carWidth, carHeight, ( 0, 0, 0 ) ) else: c = Truck( x, y, carWidth, (0, 0, 0 ) ) c.setRandomColor() c.draw( canvas ) #oddEven = not oddEven banner = Rectangle( MAXWIDTH//3, 2*MAXHEIGHT//5, MAXWIDTH//3, MAXHEIGHT//5, (255, 255, 255 ) ) banner.draw( canvas ) canvas.setTextFont( 'arial', 40, 'bold' ) canvas.drawText( MAXWIDTH//3+10, 2*MAXHEIGHT//5+30, "Happy Easter" ) win.wait() win.close() main()
</showafterdate>