Difference between revisions of "CSC111 Programs for Week 10 2015"
(→Playing Checkers) |
(→Program Created in Class) |
||
Line 251: | Line 251: | ||
</source> | </source> | ||
<br /> | <br /> | ||
− | =Program Created in Class= | + | =Checkers Program Created in Class= |
<br /> | <br /> | ||
<source lang="python"> | <source lang="python"> | ||
# checkers00.py | # checkers00.py | ||
# D. Thiebaut | # D. Thiebaut | ||
− | # | + | # This is the program we developed in class. |
− | # | + | # It displays a board with dark and white cells. |
− | # | + | # It displays images for the checkers on dark cells only. |
+ | # | ||
from graphics import * | from graphics import * | ||
− | WIDTH = 680 # width of the window | + | WIDTH = 680 # width of the window |
+ | #(adjusted to be slightly bigger than the image of the checkers piece) | ||
HEIGHT= 680 # height of the window | HEIGHT= 680 # height of the window | ||
SIDE = 680//8 # the side of a cell of the 8x8 board | SIDE = 680//8 # the side of a cell of the 8x8 board | ||
class Piece: | class Piece: | ||
+ | """A class defining an individual checkers piece.""" | ||
+ | |||
def __init__( self, ii, jj, col ): | def __init__( self, ii, jj, col ): | ||
+ | """constructor. Given an position on the board, ii, jj, and a color col, | ||
+ | creates a checkers piece of that color on the cell ii, jj on the board. We | ||
+ | assume that the top-left cell is at 0, 0.""" | ||
self.i = ii | self.i = ii | ||
self.j = jj | self.j = jj | ||
Line 272: | Line 279: | ||
x = ii * SIDE + SIDE//2 | x = ii * SIDE + SIDE//2 | ||
y = jj * SIDE + SIDE//2 | y = jj * SIDE + SIDE//2 | ||
+ | |||
""" | """ | ||
+ | # old code: creates a piece using concentrinc circles | ||
self.circ1 = Circle( Point( x, y ), SIDE//2 ) | self.circ1 = Circle( Point( x, y ), SIDE//2 ) | ||
if col=="white": | if col=="white": | ||
Line 285: | Line 294: | ||
self.circ2.setFill( color_rgb( 50, 50, 50 ) ) | self.circ2.setFill( color_rgb( 50, 50, 50 ) ) | ||
""" | """ | ||
+ | |||
+ | # new code: creates a piece using an image, either "piece.gif" which is | ||
+ | # black, or "piece2.gif" which is purple. | ||
if col=="white": | if col=="white": | ||
self.image = Image( Point( x, y ), "piece.gif" ) | self.image = Image( Point( x, y ), "piece.gif" ) | ||
Line 291: | Line 303: | ||
def draw( self, win ): | def draw( self, win ): | ||
+ | """ draw the piece on the graphic window. | ||
""" | """ | ||
+ | """ | ||
+ | # old code | ||
self.circ1.draw( win ) | self.circ1.draw( win ) | ||
self.circ2.draw( win ) | self.circ2.draw( win ) | ||
""" | """ | ||
+ | |||
+ | # new code | ||
self.image.draw( win ) | self.image.draw( win ) | ||
Line 301: | Line 318: | ||
for i in range( 8 ): | for i in range( 8 ): | ||
for j in range( 8 ): | for j in range( 8 ): | ||
+ | # compute the coordinates of the top-left point that will define | ||
+ | # the rectangular cell | ||
x = i*SIDE | x = i*SIDE | ||
y = j*SIDE | y = j*SIDE | ||
+ | |||
+ | # create a rectangle that is square, with width and height equal to SIDE. | ||
rect = Rectangle( Point( x, y ), Point( x+SIDE, y+SIDE ) ) | rect = Rectangle( Point( x, y ), Point( x+SIDE, y+SIDE ) ) | ||
if (i+j)%2 == 0: | if (i+j)%2 == 0: | ||
Line 308: | Line 329: | ||
else: | else: | ||
rect.setFill( color_rgb( 100, 100, 100 ) ) | rect.setFill( color_rgb( 100, 100, 100 ) ) | ||
+ | |||
+ | # draw the square rectangle | ||
rect.draw( win ) | rect.draw( win ) | ||
def isBlack( i, j ): | def isBlack( i, j ): | ||
+ | """A utility function that returns True if the cell at i, j should be | ||
+ | black. We found out that if all the cells for which i+j is even should | ||
+ | be the same color. So we decided that if (i+j) is odd, the cell should be | ||
+ | black. If i+j is odd, then the remainder of the division by 2 is not 0""" | ||
if (i+j)%2 == 0: | if (i+j)%2 == 0: | ||
return False | return False | ||
Line 317: | Line 344: | ||
def displayCheckers( win ): | def displayCheckers( win ): | ||
+ | """displays the pieces on the board, on the top and bottom 3 rows, and | ||
+ | on black cells only.""" | ||
checkers = [] | checkers = [] | ||
for i in range( 8 ): | for i in range( 8 ): |
Revision as of 13:33, 8 April 2015
--D. Thiebaut (talk) 05:48, 6 April 2015 (EDT)
Contents
Skeleton Program for Displaying an Image
The program below will work with this image, named "catGlasses.gif". It should be located in the same directory where the program is saved.
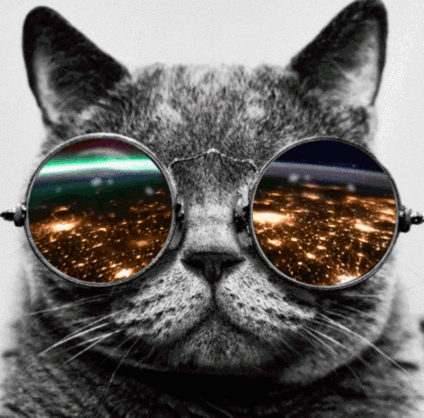
# imageProcessingSkel.py
# D. Thiebaut
# A skeleton program to start doing image processing
# in Python
from graphics import *
# image geometry
# 424x18
# make the window the same geometry
WIDTH = 424
HEIGHT = 418
IMAGEFILENAME = "catGlasses.gif"
#----------------------------------------------------------------
def waitForClick( win, message ):
""" waitForClick: stops the GUI and displays a message.
Returns when the user clicks the window. The message is erased."""
# wait for user to click mouse to start
startMsg = Text( Point( win.getWidth()/2, win.getHeight()-15 ), message )
startMsg.draw( win ) # display message
win.getMouse() # wait
startMsg.undraw() # erase
def main():
# open the window
win = GraphWin( "Image Editor", WIDTH, HEIGHT )
# open the cat image
cat = Image( Point(WIDTH//2, HEIGHT//2), IMAGEFILENAME )
cat.draw( win )
waitForClick( win, "click to close" )
win.close()
main()
Skeleton Program for Processing an Image
# pixelProcessingSkel.py
# D. Thiebaut
# Simple skeleton program for processing
# the pixels of an image
from graphics import *
# image geometry
# 424x18
# make the window the same geometry
WIDTH = 424
HEIGHT = 418
IMAGEFILENAME = "catGlasses.gif"
#----------------------------------------------------------------
def waitForClick( win, message ):
""" waitForClick: stops the GUI and displays a message.
Returns when the user clicks the window. The message is erased."""
# wait for user to click mouse to start
startMsg = Text( Point( win.getWidth()/2, win.getHeight()-15 ), message )
startMsg.draw( win ) # display message
win.getMouse() # wait
startMsg.undraw() # erase
def makeRed( win, img ):
""" set the red component of all the pixels to 255, the
maximum value.
"""
global WIDTH, HEIGHT
for x in range( WIDTH ):
for y in range( HEIGHT ):
red, green, blue = img.getPixel( x, y )
img.setPixel( x, y, color_rgb(255, green, blue ) )
def main():
win = GraphWin( "Image Editor", WIDTH, HEIGHT )
img = Image( Point(WIDTH//2, HEIGHT//2), IMAGEFILENAME )
img.draw( win )
makeRed( win, img )
waitForClick( win, "click to close" )
win.close()
main()
Image Processing: Program developed in class
# pixelProcessingSkel.py
# D. Thiebaut
# Simple skeleton program for processing
# the pixels of an image
# This is the code we developed in class
from graphics import *
# image geometry
# make the window the same geometry
WIDTH = 424 #243
HEIGHT = 418 #207
IMAGEFILENAME = "catGlasses.gif" #"catHat.gif"
# other image we can play with
#WIDTH = 243
#HEIGHT = 207
#IMAGEFILENAME = "catHat.gif"
def waitForClick( win, message ):
""" waitForClick: stops the GUI and displays a message.
Returns when the user clicks the window. The message is erased."""
# wait for user to click mouse to start
startMsg = Text( Point( win.getWidth()/2, win.getHeight()-15 ), message )
startMsg.draw( win ) # display message
win.getMouse() # wait
startMsg.undraw() # erase
def saturate( x ):
"""given a color amount, 0-255, returns 0 if the amount is closer to 0 than 255,
and returns 255 if not."""
if x < 255//2:
return 0
else:
return 255
def makeRed( win, img ):
""" set the red component of all the pixels to 255, the
maximum value.
"""
global WIDTH, HEIGHT
for y in range( HEIGHT ):
win.update()
for x in range( WIDTH ):
red, green, blue = img.getPixel( x, y )
green, blue, red = red, green, blue
red = saturate( red )
green = saturate( green )
blue = saturate( blue )
img.setPixel( x, y, color_rgb(red, green, blue ))
def makeBlackAndWhite( win, img ):
""" Make the image black and white, by making each pixel
grey. A grey pixel has the same amount of red, green, and blue.
"""
global WIDTH, HEIGHT
for y in range( HEIGHT ):
win.update()
for x in range( WIDTH ):
red, green, blue = img.getPixel( x, y )
# make the pixel grey, and use the average of the 3 values
# as the amount of grey.
avg = (green+blue+red)//3
img.setPixel( x, y, color_rgb(avg, avg, avg ) )
def addBorder( win, img ):
"""add a red border at the top of the image"
for y in range( 0, 15 ):
for x in range( WIDTH ):
img.setPixel( x, y, color_rgb( 255, 0, 0 ) )
def main():
win = GraphWin( "Dominique Thiebaut", WIDTH, HEIGHT )
img = Image( Point(WIDTH//2, HEIGHT//2), IMAGEFILENAME )
img.draw( win )
makeRed( win, img )
addBorder( win, img )
waitForClick( win, "click to close" )
win.close()
main()
Playing Checkers
Checkers0.py
This program is the bare skeleton for display the board. You have to add the code inside the displayBoard() function.
# checkers0.py
# D. Thiebaut
# A first program to display an 8x8 chessboard
# in a graphic window.
#
from graphics import *
WIDTH = 600 # width of the window
HEIGHT= 600 # height of the window
SIDE = 600//8 # the side of a cell of the 8x8 board
def displayBoard( win ):
"""displays an 8x8 board in the graphic window. The board extends to the sides of the window'''
# add your code here...
return
def waitForClick( win, message ):
""" waitForClick: stops the GUI and displays a message.
Returns when the user clicks the window. The message is erased."""
# wait for user to click mouse to start
startMsg = Text( Point( win.getWidth()/2, win.getHeight()-15 ), message )
startMsg.draw( win ) # display message
win.getMouse() # wait
startMsg.undraw() # erase
def main():
# open the window
win = GraphWin( "Checkers", WIDTH, HEIGHT )
# display 8x8 checkers board
displayBoard( win )
# wait for user to click before closing everything...
waitForClick( win, "click to close" )
win.close()
main()
Checkers Program Created in Class
# checkers00.py
# D. Thiebaut
# This is the program we developed in class.
# It displays a board with dark and white cells.
# It displays images for the checkers on dark cells only.
#
from graphics import *
WIDTH = 680 # width of the window
#(adjusted to be slightly bigger than the image of the checkers piece)
HEIGHT= 680 # height of the window
SIDE = 680//8 # the side of a cell of the 8x8 board
class Piece:
"""A class defining an individual checkers piece."""
def __init__( self, ii, jj, col ):
"""constructor. Given an position on the board, ii, jj, and a color col,
creates a checkers piece of that color on the cell ii, jj on the board. We
assume that the top-left cell is at 0, 0."""
self.i = ii
self.j = jj
self.color = col
x = ii * SIDE + SIDE//2
y = jj * SIDE + SIDE//2
"""
# old code: creates a piece using concentrinc circles
self.circ1 = Circle( Point( x, y ), SIDE//2 )
if col=="white":
self.circ1.setFill( color_rgb( 250, 250, 250 ) )
else:
self.circ1.setFill( color_rgb( 10, 10, 10 ) )
self.circ2 = Circle( Point( x, y ), SIDE//2 - 5 )
if col=="white":
self.circ2.setFill( color_rgb( 230, 230, 230 ) )
else:
self.circ2.setFill( color_rgb( 50, 50, 50 ) )
"""
# new code: creates a piece using an image, either "piece.gif" which is
# black, or "piece2.gif" which is purple.
if col=="white":
self.image = Image( Point( x, y ), "piece.gif" )
else:
self.image = Image( Point( x, y ), "piece2.gif" )
def draw( self, win ):
""" draw the piece on the graphic window.
"""
"""
# old code
self.circ1.draw( win )
self.circ2.draw( win )
"""
# new code
self.image.draw( win )
def displayBoard( win ):
"""display an 8x8 board"""
for i in range( 8 ):
for j in range( 8 ):
# compute the coordinates of the top-left point that will define
# the rectangular cell
x = i*SIDE
y = j*SIDE
# create a rectangle that is square, with width and height equal to SIDE.
rect = Rectangle( Point( x, y ), Point( x+SIDE, y+SIDE ) )
if (i+j)%2 == 0:
rect.setFill( color_rgb( 255, 255, 255 ) )
else:
rect.setFill( color_rgb( 100, 100, 100 ) )
# draw the square rectangle
rect.draw( win )
def isBlack( i, j ):
"""A utility function that returns True if the cell at i, j should be
black. We found out that if all the cells for which i+j is even should
be the same color. So we decided that if (i+j) is odd, the cell should be
black. If i+j is odd, then the remainder of the division by 2 is not 0"""
if (i+j)%2 == 0:
return False
else:
return True
def displayCheckers( win ):
"""displays the pieces on the board, on the top and bottom 3 rows, and
on black cells only."""
checkers = []
for i in range( 8 ):
for j in range( 3 ):
if isBlack( i, j ):
piece = Piece( i, j, "white" )
piece.draw( win )
checkers.append( piece )
for i in range( 8 ):
for j in range( 5, 8 ):
if isBlack( i, j ):
piece = Piece( i, j, "black" )
piece.draw( win )
checkers.append( piece )
def waitForClick( win, message ):
""" waitForClick: stops the GUI and displays a message.
Returns when the user clicks the window. The message is erased."""
startMsg = Text( Point( win.getWidth()/2, win.getHeight()-15 ),
message )
startMsg.draw( win ) # display message
win.getMouse() # wait
startMsg.undraw() # erase
def main():
# open the window
win = GraphWin( "Checkers", WIDTH, HEIGHT )
# display 8x8 checkers board
displayBoard( win )
displayCheckers( win )
# wait for user to click before closing everything...
waitForClick( win, "click to close" )
win.close()
main()