Difference between revisions of "CSC111 Lab 12 2011"
Line 185: | Line 185: | ||
==Challenge 5: Optional== | ==Challenge 5: Optional== | ||
|} | |} | ||
− | [[Image: | + | [[Image:QuestionMark5.jpg|right|120px]] |
<br /> | <br /> | ||
Revision as of 10:36, 30 November 2011
--D. Thiebaut 09:42, 30 November 2011 (EST)
This lab will have you play with classes and objects: the essence of Object Oriented Programming!
Contents
Part 1: A Bus
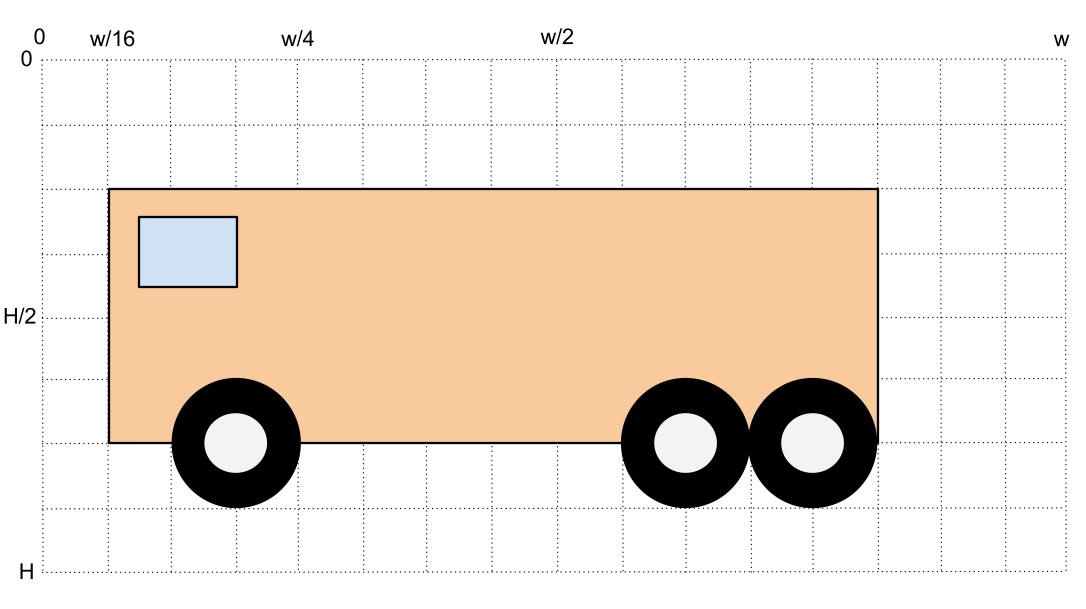
- Using the example we did in class on Tuesday as inspiration (see similar code below), create a new program that draws the bus shown above.
- Your program should have two classes:
- class Wheel
- class Bus
# A program with a car and a wheel class.
# This is slightly different from what we did in class, but works
# the same. Figure out what the differences are.
#
from graphics import *
W = 800
H = 600
#----------------------------------------------------------------
class Wheel:
"""A class with two concentric circles"""
def __init__( self, center, r1, r2 ):
self.circ1 = Circle( center, r1 )
self.circ2 = Circle( center, r2 )
r1, r2 = min( r1, r2 ), max( r1, r2 )
self.radius1 = r1
self.radius2 = r2
def draw( self, win ):
"""draws the wheel on the window"""
self.circ2.draw( win )
self.circ1.draw( win )
def setFill( self, color1, color2 ):
"""colors the outside and inside circles. First color for small circle"""
self.circ1.setFill( color1 )
self.circ2.setFill( color2 )
def getRadius1( self ):
"""returns radius of small circle"""
return self.radius1
def getRadius2( self ):
"""returns radius of large circle"""
return self.radius2
#----------------------------------------------------------------
class Car:
"""A class for a car, which is a rectangle and two wheels"""
def __init__( self, P1, P2 ):
"""Get 2 points and create the box and two wheels from the dimensions"""
self.P1 = P1
self.P2 = P2
self.width = abs( P1.getX()-P2.getX() )
self.height= abs( P1.getY()-P2.getY() )
self.rect = Rectangle( P1, P2 )
center1 = Point( P1.getX()+self.width/8, P2.getY() )
center2 = Point( P1.getX()+self.width*7/8, P2.getY() )
radius2 = self.height/3
radius1 = radius2/2
self.wheel1 = Wheel( center1, radius1, radius2 )
self.wheel2 = Wheel( center2, radius1, radius2 )
def draw( self, win ):
"""draws the car on the screen. One of the wheels is covered"""
self.wheel2.draw( win )
self.rect.draw( win )
self.wheel1.draw( win )
def move( self, dx, dy ):
"""moves the car by dx and dy"""
self.wheel2.move( dx, dy )
self.rect.move( dx, dy )
self.wheel1.move( dx, dy )
#----------------------------------------------------------------
def waitForClick( win, message ):
""" waitForClick: stops the GUI and displays a message.
Returns when the user clicks the window. The message is erased."""
# wait for user to click mouse to start
startMsg = Text( Point( win.getWidth()/2, win.getHeight()/2 ), message )
startMsg.draw( win ) # display message
win.getMouse() # wait
startMsg.undraw() # erase
def main():
global W, H
win = GraphWin( "wheel demo", W, H )
waitForClick( win, "click to start" )
car = Car( Point( 100,100 ), Point( 250, 170 ) )
car.draw( win )
waitForClick( win, "click to end" )
main()
Challenge 1 |
- Add a for-loop that makes your bus disappear from the window. Make it go in the forward direction (i.e. left for the bus in the image above).
class Tree:
def __init__( ... ):
...
def draw( ... ):
...
def move( ... ):
...
- Make your program display the tree with the bus. No need for any of them to move, but that's fine if the bus is moving out of the window...
Part 2: Trees
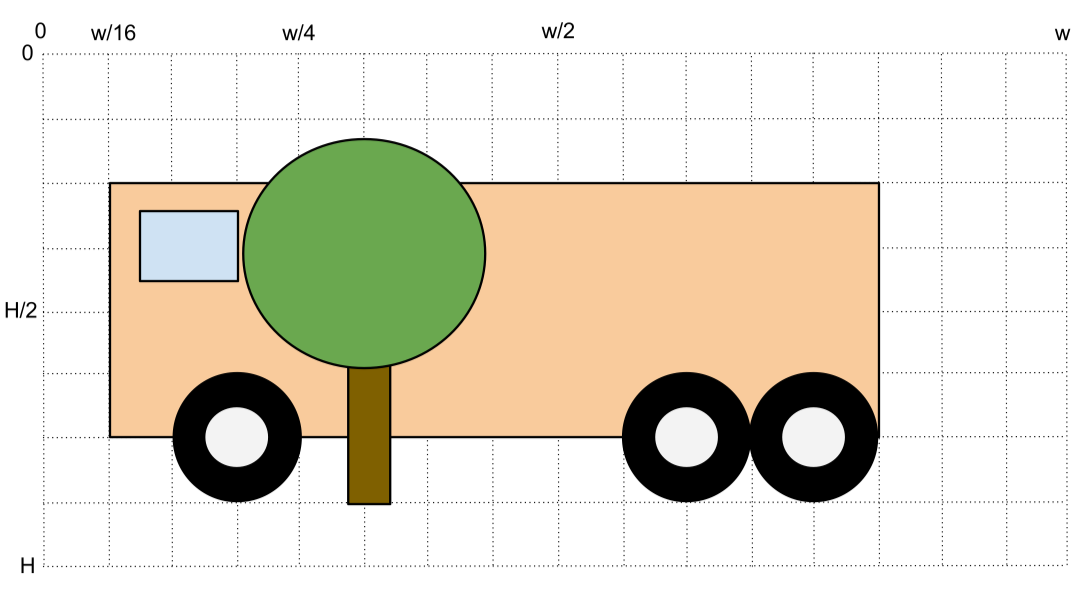
- Create a new class in your program called Tree. It will represent a simplified tree, with its green head of leaves, and its brown trunk.
- Make your program display the bus and the tree. It doesn't matter if the tree is in front or behind the bus.
Challenge 2 |
- Make the bus stand still and the tree move in the direction opposite of the bus direction.
- Make the tree move out of the window
Challenge 3 |
- Add another tree object. One class: two objects.
- Locate the trees in different locations so that they do not overlap (hint: you can change the constructor so that you can specify the x-coordinate of the tree object when you create it:)
- Make your program display the two trees and the bus
Challenge 4 |
- Make the two trees move to the right and disappear from the window
Challenge 5: Optional |
- Make the trees that disappear from the right reappear on the left, giving the illusion of a real cartoon!