Difference between revisions of "CSC231 Homework 7 Fall 2012"
(→Problem #2) |
(→Problem #2) |
||
Line 30: | Line 30: | ||
The purpose of this problem is for you to take a string created using this method, decode it, and print it on the screen. | The purpose of this problem is for you to take a string created using this method, decode it, and print it on the screen. | ||
− | Let's look at an example. Look at the 2 variables below. '''xLen''' is a double word, and contains 18. That represents the number of characters | + | Let's look at an example. Look at the 2 variables below. '''xLen''' is a double word, and contains 18. That represents the number of bytes containing the characters of the original string. Since each byte of x contains 2 nybbles that are the lower parts of two different characters, then '''xLen''' is the number of pairs of nybbles. |
+ | |||
+ | Let's take a look at '''x''' now. The first byte of '''x''' is 0xd1, which means that the first two characters of the original string are 0x4'''d''' and 0x4'''1''', or 'M' and 'A'. So your program will output 'M' then 'A' when it decodes the string. | ||
Line 40: | Line 42: | ||
Your program must decode the two strings listed below and print them on the screen. Your program should replace the character '@' with a space. | Your program must decode the two strings listed below and print them on the screen. Your program should replace the character '@' with a space. | ||
− | yLen dd | + | yLen dd 18 |
− | y db 0xd5, 0x54, | + | y db 0xd5, 0x54, 0x00, 0x31, 0xe4, 0x94, 0x0f, 0x21, 0xd1 |
− | db | + | db 0x01, 0xe4, 0x02, 0xfe, 0x90, 0xf6, 0x07, 0x1e, 0x10 |
xLen dd 18 | xLen dd 18 | ||
x db 0xd1, 0x41, 0xd5, 0x03, 0x81, 0xee, 0x5c, 0x0d | x db 0xd1, 0x41, 0xd5, 0x03, 0x81, 0xee, 0x5c, 0x0d |
Revision as of 13:34, 24 October 2012
--D. Thiebaut 13:47, 24 October 2012 (EDT)
This assignment is due the evening of Oct. 31st, at midnight (booo!). You can work on this assignment in pairs.
Problem #1
Write a program that displays the contents of a double-word in hexadecimal. Your program should store the value 0x1234ABCD in a variable called x and should display this information:
x = 305441741 = 0x1234ABCD
Submission
Submit your program (called hw7a.asm) as follows:
rsubmit hw7 hw7a.asm
Problem #2
Take a look at the section of the ASCII table below:
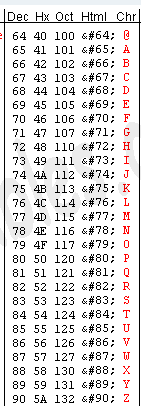
Concentrate on the hexadecimal code for each letter. You will notice that 'A' through 'O' have the same upper nybble. 'A' is 0x41 and 'O' is 0x4F. So, if I wanted to squeeze a string made of upper-case letters between 'A' and 'O', I would only need to store the lower nybble, and I'd save some memory.
The purpose of this problem is for you to take a string created using this method, decode it, and print it on the screen.
Let's look at an example. Look at the 2 variables below. xLen is a double word, and contains 18. That represents the number of bytes containing the characters of the original string. Since each byte of x contains 2 nybbles that are the lower parts of two different characters, then xLen is the number of pairs of nybbles.
Let's take a look at x now. The first byte of x is 0xd1, which means that the first two characters of the original string are 0x4d and 0x41, or 'M' and 'A'. So your program will output 'M' then 'A' when it decodes the string.
xLen dd 18 x db 0xd1, 0x41, 0xd5, 0x03, 0x81, 0xee, 0x5c, 0x0d db 0x55, 0x40, 0x21, 0x40, 0xd1, 0xe0, 0x9e, 0x0c, 0x9d, 0x10
Your program must decode the two strings listed below and print them on the screen. Your program should replace the character '@' with a space.
yLen dd 18 y db 0xd5, 0x54, 0x00, 0x31, 0xe4, 0x94, 0x0f, 0x21, 0xd1 db 0x01, 0xe4, 0x02, 0xfe, 0x90, 0xf6, 0x07, 0x1e, 0x10 xLen dd 18 x db 0xd1, 0x41, 0xd5, 0x03, 0x81, 0xee, 0x5c, 0x0d db 0x55, 0x40, 0x21, 0x40, 0xd1, 0xe0, 0x9e, 0x0c, 0x9d, 0x10
Submission
rsubmit hw7 hw7b.asm