Difference between revisions of "CSC111 Lab 4 2014"
(→Submission) |
|||
Line 281: | Line 281: | ||
<br /> | <br /> | ||
<br /> | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <br /> | ||
+ | <onlydft> | ||
+ | <source lang="python"> | ||
+ | # Solutions for Lab 4 | ||
+ | # D. Thiebaut | ||
+ | |||
+ | # =========================================================== | ||
+ | # Exercise 1 | ||
+ | print( "\n========================\nExercise 1\n========================" ) | ||
+ | |||
+ | x = int( input( "enter an integer less than 3 or greater than 9: " ) ) | ||
+ | while 3 <= x <= 9: | ||
+ | print( "invalid input!" ) | ||
+ | x = int( input( "enter an integer less tan 3 or greater than 9: " ) ) | ||
+ | |||
+ | print( "x = ", x ) | ||
+ | |||
+ | # =========================================================== | ||
+ | # challenge 1 | ||
+ | print( "\n========================\nChallenge 1\n========================" ) | ||
+ | x = int( input( "enter an integer between 3 and 9: " ) ) | ||
+ | while not (3 <= x <= 9 ): | ||
+ | print( "invalid input!" ) | ||
+ | x = int( input( "enter an integer between 3 and 9: " )) | ||
+ | |||
+ | print( "x = ", x ) | ||
+ | |||
+ | # =========================================================== | ||
+ | # Exercise 2 | ||
+ | print( "\n========================\nExercise 2\n========================" ) | ||
+ | |||
+ | #--- ask user question --- | ||
+ | answer = input( "Do you like chocolate (Y/N)? " ) | ||
+ | answer = answer.lower().strip() | ||
+ | |||
+ | #--- keep on asking while invalid --- | ||
+ | while answer != 'y' and answer != 'yes' and answer != 'n' and answer != 'no': | ||
+ | answer = input( "Please reenter: " ) | ||
+ | answer = answer.lower().strip() | ||
+ | |||
+ | #--- now that we know the answer, give feedback --- | ||
+ | if answer == 'n' or answer == 'no' : | ||
+ | print( "That's terrible" ) | ||
+ | else: | ||
+ | print( "That's great!" ) | ||
+ | |||
+ | # =========================================================== | ||
+ | # Exercise 3 | ||
+ | print( "\n========================\nExercise 3\n========================" ) | ||
+ | |||
+ | human = input( "what is your play (R, S, P)? " ) | ||
+ | human = human.strip().lower() | ||
+ | while human != 'p' and human != 's' and human != 'r': | ||
+ | human = input( "I didn't get that. Please reenter: " ) | ||
+ | |||
+ | print( "You have played", human.upper() ) | ||
+ | |||
+ | # =========================================================== | ||
+ | # Challenge 2 | ||
+ | # buggy program that is supposed to compute | ||
+ | # the sum of even numbers and stop as soon as | ||
+ | # the sum is greater than or equal to 20. Your | ||
+ | # job is to fix the program using PythonTutor. | ||
+ | |||
+ | print( "\n========================\nChallenge 2\n========================" ) | ||
+ | |||
+ | # solution 1 | ||
+ | sum = 0 | ||
+ | num = 0 | ||
+ | |||
+ | while sum < 20: | ||
+ | num = num + 2 # <--- fix by switching statements | ||
+ | sum = sum + num | ||
+ | |||
+ | print( "the sum of the even numbers from 0 to %d is %d\n" | ||
+ | % ( num, sum ) ) | ||
+ | |||
+ | # solution 2 | ||
+ | sum = 0 | ||
+ | num = 0 | ||
+ | |||
+ | while sum < 20: | ||
+ | sum = sum + num | ||
+ | num = num + 2 | ||
+ | |||
+ | # num is always 2 larger that the associated sum | ||
+ | # decrease num to undo the last increment statement in the loop | ||
+ | num = num - 2 | ||
+ | print( "the sum of the even numbers from 0 to %d is %d\n" | ||
+ | % ( num, sum ) ) | ||
+ | |||
+ | # =========================================================== | ||
+ | # Exercise 5 | ||
+ | print( "\n========================\nExercise 5\n========================" ) | ||
+ | |||
+ | initBalance = 1000.00 | ||
+ | intRate = 0.05 | ||
+ | |||
+ | print( "initial balance = $%1.2f" % initBalance ) | ||
+ | |||
+ | newBalance = initBalance | ||
+ | period = 0 | ||
+ | while period < 4: | ||
+ | newBalance = newBalance + newBalance * intRate | ||
+ | period = period + 1 | ||
+ | print( "after Investment Period %d, new balance = $%1.2f" | ||
+ | % ( period, newBalance ) ) | ||
+ | |||
+ | |||
+ | |||
+ | # =========================================================== | ||
+ | # Challenge 3 | ||
+ | print( "\n========================\nChallenge 3\n========================" ) | ||
+ | |||
+ | initBalance = 1000.00 | ||
+ | intRate = 0.05 | ||
+ | |||
+ | print( "initial balance = $%1.2f" % initBalance ) | ||
+ | |||
+ | newBalance = initBalance | ||
+ | period = 0 | ||
+ | |||
+ | targetBalance = float( input( "What is your target balance? " ) ) | ||
+ | |||
+ | while newBalance < targetBalance: | ||
+ | newBalance = newBalance + newBalance * intRate | ||
+ | period = period + 1 | ||
+ | if period == 1: | ||
+ | plural = "" | ||
+ | else: | ||
+ | plural = "s" | ||
+ | print( "after %2d investment period%s, balance = $%6.2f" | ||
+ | % ( period, plural, newBalance ) ) | ||
+ | |||
+ | |||
+ | </source> | ||
+ | </onlydft> | ||
<br /> | <br /> | ||
<br /> | <br /> |
Revision as of 13:08, 18 February 2014
--D. Thiebaut (talk) 15:11, 17 February 2014 (EST)
This lab deals with while loops and introduces a new tool: PythonTutor.
Contents
Exercise 1
- Observe the program below
- Once you have figured out how it works, enter it in Idle and run it. Verify that it runs correctly.
x = int( input( "enter an integer less than 3 or greater than 9: " ) )
while 3 <= x <= 9:
print( "invalid input!" )
x = int( input( "enter an integer less tan 3 or greater than 9: " ) )
print( "x = ", x )
Challenge 1 |
With the least amount of change to your code (we don't count the characters inside strings) make it accept only numbers that are between 3 and 9, 3 and 9 included.
Exercise 2
Write a Python program that asks the user to input the answer to a Yes/No question. The user is allowed to enter 'Y', 'y', 'yes', 'YES' for yes, and 'N', 'n', 'no', 'NO' for no.
Your program will contain a while loop that will force the user to keep entering an answer until it is one of the authorized inputs shown above.
You can use the skeleton program shown below as a starting point. Add different parts to make it robust
#--- ask user question ---
answer = input( "Do you like chocolate (Y/N)? " )
...
#--- keep on asking while invalid ---
while ... :
answer = input( "Please reenter: " )
...
#--- now that we know the answer, give feedback ---
if answer == ... :
print( "That's terrible" )
else:
print( "That's great!" )
- Make sure the program rejects anything that is not 'y', 'Y', 'yes', 'YES', 'n', 'N', 'no', and 'NO'.
- Also make sure the program accepts " yes " (lots of spaces around it).
Exercise 3
Write a program that reads a character for playing the game of Rock-Paper-Scissors. If the character entered by the user is not one of 'P', 'R' or 'S', the program keeps on prompting the user to enter a new character.
Exercise 4: Using PythonTutor
- Point your browser to http://www.pythontutor.com/ and click on Start Using Python Tutor
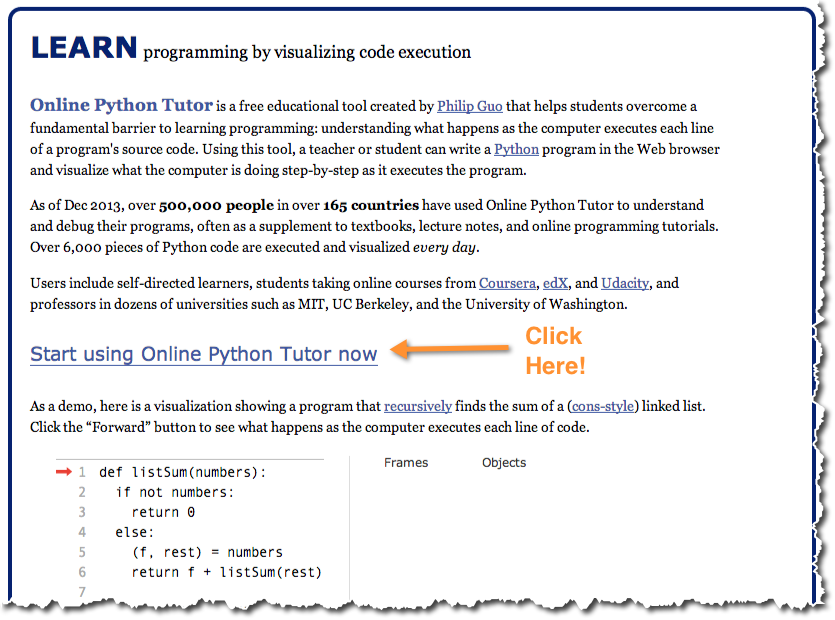
sum = 0
num = 1
while num <= 5:
sum = sum + num;
num = num + 1
print( "the sum of the numbers from 1 to %d is %d" % ( 5, sum ) )
- Enter the program above in the code window, select Python 3, and click on Visualize, as illustrated below. The image below is just an illustration. You should be working in another window or tab of your browser.
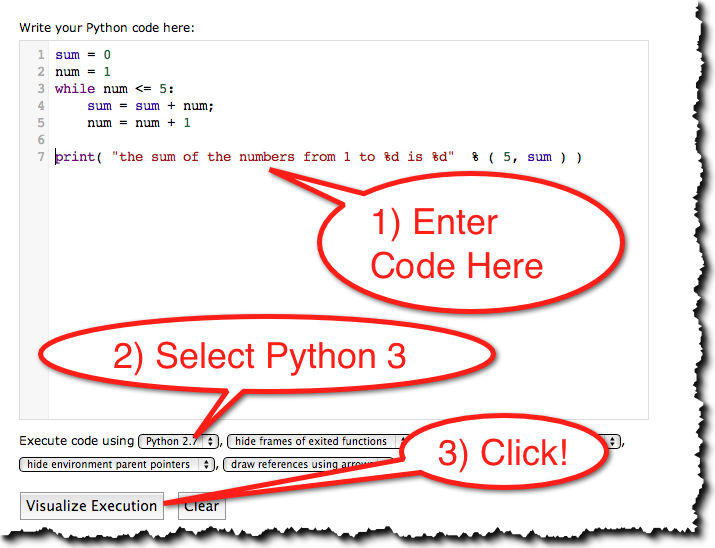
- In the new window that comes up, click on Forward and observe how the interpreter executes one line at a time and displays how the the variables change. We call this process single-stepping a program. In other word we force the interpreter to go one step (line) at a time.
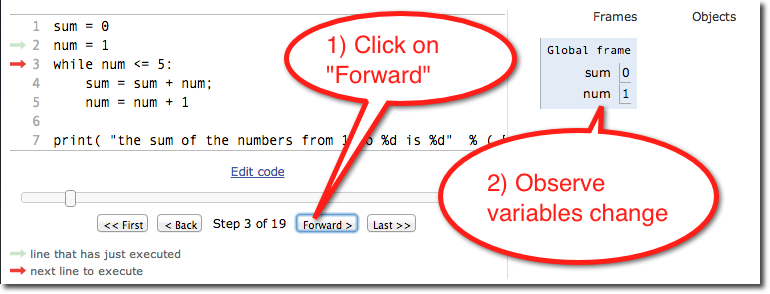
- This is a great way to observe how small code sections operate!
- Single-step the whole program to see that it works correctly.
Challenge 2: Buggy Program |
The program below is supposed to compute the sum of all the even numbers
such that their sum is less than or equal to 20. Use PythonTutor to figure out the bug!
# buggy program that is supposed to compute
# the sum of even numbers and stop as soon as
# the sum is greater than or equal to 20. Your
# job is to fix the program using PythonTutor.
sum = 0
num = 0
while sum < 20:
sum = sum + num
num = num + 2
print( "the sum of the even numbers from 0 to %d is %d\n"
% ( num, sum ) )
Exercise 5: Investment and Interest Rate with PythonTutor
- Use PythonTutor to write this program.
- Below is a program that prints how a $1000 investment grows at a compounding rate of 5% over 4 periods.
initBalance = 1000.00
intRate = 0.05
print( "initial balance = $%1.2f" % initBalance )
newBalance = initBalance
newBalance = newBalance + newBalance * intRate
print( "after 1 investment period, new balance = $%1.2f" % newBalance )
newBalance = newBalance + newBalance * intRate
print( "after 1 investment period, new balance = $%1.2f" % newBalance )
newBalance = newBalance + newBalance * intRate
print( "after 1 investment period, new balance = $%1.2f" % newBalance )
newBalance = newBalance + newBalance * intRate
print( "after 1 investment period, new balance = $%1.2f" % newBalance )
- Replace the 4 identical pairs of statements with a while loop that will repeat one pair 4 times. The output of your program should be the same as the original program above.
- Change your program so that it prints the number of investment periods, as illustrated below:
initial balance = $1000.00 after 1 investment period(s), new balance = $1050.00 after 2 investment period(s), new balance = $1102.50 after 3 investment period(s), new balance = $1157.62 after 4 investment period(s), new balance = $1215.51
Challenge 3 |
Make your program first prompt the user for the amount she would like the initial investment to grow to. Then the program will print the growth of the investment each period until the balance as grown past the user's selected amount.
Here's an example where the user's input is underlined. Note that the program stops after displaying the first balance that is greater than or equal to $1,500.
initial balance = $1000.00 What is your target balance? 1500 after 1 investment period, balance = $1050.00 after 2 investment periods, balance = $1102.50 after 3 investment periods, balance = $1157.62 after 4 investment periods, balance = $1215.51 after 5 investment periods, balance = $1276.28 after 6 investment periods, balance = $1340.10 after 7 investment periods, balance = $1407.10 after 8 investment periods, balance = $1477.46 after 9 investment periods, balance = $1551.33
Exercise 6: Sentinels
We return to Idle with this program. However, you should feel free to use PythonTutor anytime you'd like to single step a program that is not behaving the way you want. It's a great tool to see the true logic of a program dynamically!
- Type or copy the program below in Idle.
- Look at it and make sure you think you know how it will work
- Run the program
print( "please enter positive integers at the prompt. Enter -1 to stop" )
sum = 0
x = 0
while x != -1:
x = int( input( "Enter a positive integer (-1 to stop): " ) )
if x != -1:
sum = sum + x
print( "The sum of the numbers you entered is", sum )
Challenge 4 |
Modify the program above so that it stops when the sum of the numbers is greater than 20.
Challenge 5 |
Modify the program so that this time the program outputs the number of positive integers entered.
Submission
Store a copy of the last working program, or of all the sections you worked on in a program called lab4.ph and submit it to this address: cs.smith.edu/~thiebaut/111b/submitL4.php.