Difference between revisions of "CSC111 Lab 5 2014"
(→Submission) |
(→Challenge #9) |
||
Line 333: | Line 333: | ||
| | | | ||
− | ===Challenge #9=== | + | ===Challenge #9 (Optional)=== |
|} | |} | ||
[[Image:QuestionMark9.jpg|right|120px]] | [[Image:QuestionMark9.jpg|right|120px]] |
Revision as of 21:42, 25 February 2014
--D. Thiebaut (talk) 18:16, 25 February 2014 (EST)
This lab presents JES as a practical way of manipulating sound files. This requires only single for-loops. The remaining part of the lab contains many small exercises dealing with for-loop with the intent of mastering the use of single for loops in many different situations.
Single for-loops
- Create a new program called lab5.py
# lab5.py for i in range( 3, 8 ): print( i )
- Verify that you get the correct output:
python3 lab5.py 3 4 5 6 7
Challenge Block 1 |
- Now, your turn!
- List of numbers, Version 2
- Modify your loop so that it does not use the range( ) function (Hint: you have to list all the values)
- List of even numbers
- write a loop that prints all the even numbers between 4 and 20, 4, and 20 included (you can use range or list all the numbers).
- List of odd numbers
- write a loop that prints all the odd numbers between -3 and 19, -3, and 19 included.
- User-controlled loops (more difficult)
- Write a new Python program that prints a series of numbers, after having asked the user where the series should start, and where it should end.
- Here is an example of how it should run (the user input is underlined):
python3 userLoop.py I will print a list of numbers for you. Where should I start? 3 What is the last number of the series? 10 3 4 5 6 7 8 9 10
- Go ahead and write it. What happens if you ask the program to count from -5 to 5? Does it work correctly?
- Does your program work if you ask your program to go from 1 to 1?
- Can you predict what will be printed if you ask your program to count from 10 to 1?
Challenge 2 |
- Make the last program you wrote print the series numbers between the two numbers entered by the user, wether the first one is less than the second one, or vice versa.
Challenge 3 |
Write a program that prints the pattern shown below. Do not start right away! Instead read the directions, please!
>>> ================================ RESTART ================================
>>>
----
*..-----??
**....------????
***......-------??????
****........--------????????
*****..........---------??????????
******............----------????????????
*******..............-----------??????????????
********................------------????????????????
*********..................-------------??????????????????
**********....................--------------????????????????????
>>>
- Instead of starting to program, write down on a piece of paper the number of symbols on each line. For example,
- on the first line, zero stars, zero dots, zero minuses, zero question marks.
- on the 2nd line, two dots, two question marks.
- keep on writing how many characters appear on each line.
- then write a program with a loop that will print just the number of stars on each line.
Nested For-Loops
This is taken from Section 4.7 in the textbook:
for i in range(4):
for j in range( i+1 ):
print( "*", end="" )
print()
The output is
*
**
***
****
Challenge 3 |
- Modify the program so that it outputs this pattern
****
***
**
*
Challenge 4 |
- Modify the program so that it outputs this pattern:
*
**
***
****
Challenge 5 |
- Now try this pattern:
****
***
**
*
- What about this one?
1234
567
89
10
JES
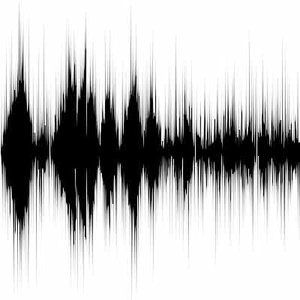
Download JES
- Download JES 4-3 to your computer from https://code.google.com/p/mediacomp-jes/downloads/list.
- If you are running Windows, pick jes-4-3.exe. Once downloaded, click on the exe file to start JES.
- If you are running Mac OS X, pick jes-4-3-mac.zip, click on the downloaded zip file. Then click on the extracted file to start JES.
Download a Sound File
- Download the following file to your computer:
Writing Your First JES Program
- Open JES
- Type the following program in the top window:
file = pickAFile() sound = makeSound( file ) blockingPlay( sound )
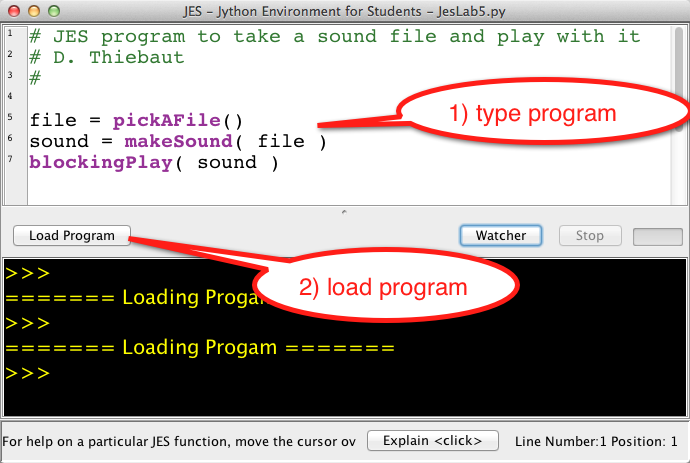
- click on "Load Program" and save the program in the folder of your choice (a good place would be where you saved the Force.wav file).
- When prompted to enter a file in the Pick A File window, navigate through your folders and pick force.wav or Force.wav, which ever way you named it.
- Make sure the speakers are on so that you can hear your computer play the file. Don't worry about making sounds in this lab: this is what this lab is about!
Visualizing the Waveform of the Sound File
- Once you have played a sound in JES you can access its waveform using the Sound Tool of the MediaTools menu at the top of the window (or the screen).
- Open the Sound Tool. This is what you should see:
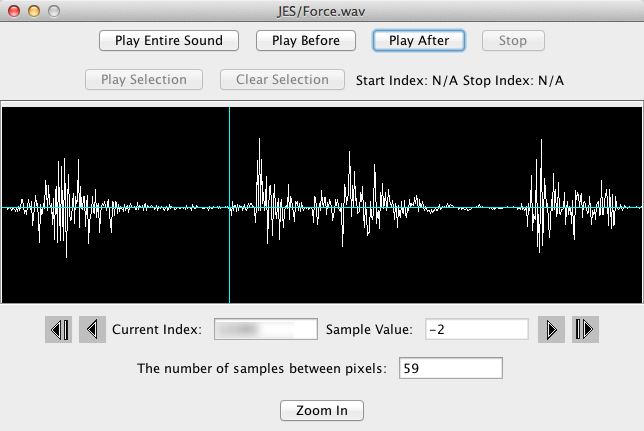
- Move the cursor around the waveform and click on different buttons (Play before, Play after, Play selection, etc.)
Clearing Part Of The Waveform
- Position the cursor in the waveform as shown in the image above, just before the words "the force" are uttered by Sir Alec Guinness. Note the index of the sample where the cursor and assign it to a variable in your JES program, as shown below. Just replace the ... with the actual number
file = pickAFile() sound = makeSound( file ) blockingPlay( sound ) # get start index for clearing startIndex = ... # index just before "the force..."
- Now that you have the beginning index of the phrase "the force will be with you, always", you can clear it by setting all the samples to 0, as follows:
# clear all the samples from startIndex to end: for i in range( startIndex, noSamples ): setSampleValueAt( sound, i, 0 ) # play sound again blockingPlay( sound )
- Add this new code to the end of your program and run it. It should just play "Remember" and nothing else. Look at the new waveform of your sound with the Sound Tool (close it first to get rid of the original waveform and reopen it).
Copying Parts of the Waveform
- The goal of this part is to copy the samples corresponding to "Remember" so that the waveform becomes "Remember Remember".
- You need two functions to perform this operation:
value = getSampleValueAt( sound, i )
- which gets the sample at Index i and stores it in the variable value, and
setSampleValueAt( sound, i, value )
- which sets the sample at Index i to value.
Challenge #6 |
Add a for loop that copies all the samples of "remember" and duplicates them in the waveform, so that when you play it you hear two "remember" words.
Challenge #7 |
Modify your program so that it transforms the waveform in such a way that when you play it at then end of the transformation it will play "Remember, always!"
Challenge #8 |
Modify the last waveform ("Remember always") and reverse it so that it plays "backwards" when you play it. You have two ways of performing it. One simpler than the other one.
- Simpler method: Assume the following numbers represent the samples of the waveform:
17 18 17 16 11 0 0 0 0 0 0 0 0 0
- then reversing it would yield:
0 0 0 0 0 0 0 0 0 11 16 17 18 17
- The more elegant method (feel free to attempt it) would yield the reversed numbers in the following way:
11 16 17 18 17 0 0 0 0 0 0 0 0 0
Challenge #9 (Optional) |
Modify your program so that it transforms the waveform in such a way that when you play it at then end of the transformation it will play "Remember" with an echo! (Remember, member, mber, ber...).
Be sure to make me listen to this new waveform before you move on to the next part!
Submission
Copy/paste your JES program into Idle and save the file as lab5.py. Submit it to this URL: http://cs.smith.edu/~dthiebaut/111b/submitL5.php