Difference between revisions of "CSC212 Syllabus 2014"
(→Professor) |
(→Textbooks) |
||
Line 75: | Line 75: | ||
;For Data Structures: | ;For Data Structures: | ||
− | :''Data Structures and Algorithms in Java'' ( | + | : [http://www.amazon.com/Data-Structures-Algorithms-Java-Second/dp/0534492525/ref=sr_1_1?s=books&ie=UTF8&qid=1405693928&sr=1-1 Drozdek]'s ''Data Structures and Algorithms in Java.'' (2nd or 3rd Ed.). Either the book or the electronic version are fine. This book is '''required'''. It's a very expensive book, but one that will be a good reference to keep. |
;For Java: | ;For Java: | ||
− | :''Head Frist Java.'' | + | : [http://www.amazon.com/s/ref=nb_sb_ss_i_0_4?url=search-alias%3Dstripbooks&field-keywords=head+first+java&sprefix=head%2Cstripbooks%2C130 Sierran and Bates]' ''Head Frist Java.'' This book is '''recommended,''' and you can substitute another Java reference for it if you prefer, as long as it supports your learning in the course. Plan to hang on to these books, so you can brush up your skills later on and teach yourself new ones. |
− | + | ||
− | |||
=Requirements= | =Requirements= | ||
Revision as of 12:57, 28 August 2014
--D. Thiebaut (talk) 10:33, 18 July 2014 (EDT)
<meta name="keywords" content="computer science, assembly language, pentium, linux, programming" /> <meta name="description" content="Dominique Thiebaut's Web Page" /> <meta name="title" content="Dominique Thiebaut -- Computer Science" /> <meta name="abstract" content="Dominique Thiebaut's Computer Science Web pages" /> <meta name="author" content="thiebaut at cs.smith.edu" /> <meta name="distribution" content="Global" /> <meta name="revisit-after" content="10 days" /> <meta name="copyright" content="(c) D. Thiebaut 2000, 2001, 2002, 2003, 2004, 2005, 2006, 2007,2008,2009,2010,2011,2014" /> <meta name="robots" content="FOLLOW,INDEX" />
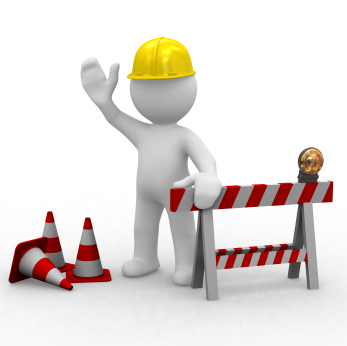
Data Structures in Java
CSC 212, Fall 2014
(http://cs.smith.edu/dftwiki/index.php/CSC212_Syllabus_2014)
|
Professor
Dominique Thiébaut |
Go to the class Class Homepage for exercises, demo programs, and misc. information.
Course Description and Overview
CSC 212 is a required programming course in the Computer Science major sequence. It addresses more advanced programming techniques using the Java programming language, and will examine in detail a selection of useful data structures. While the projects in the course will examine the minutiae of specific programming tasks, we also aim to develop a global understanding of the art of programming. Group discussion and sharing of ideas will support this endeavor. Along the way we will also learn some additional useful tricks with the Unix operating system.
This is a programming intensive course. There are weekly lab and homework assignments and a final programming project, totaling 70% of the grade. It is imperative that you do not fall behind in the assignments because this most often proves unrecoverable and leads to dropping the course. Many resources (professor, TA hours, textbook, Web) are available to assist you in completing the coursework, and you are expected to take advantage of them. This applies regardless of how well you are performing: even excellent programmers have room for further improvement. The programming assignments cover practical aspects of the course material, and two exams (in-class midterm and take-home final) will test both theoretical and practical aspects of the concepts presented.
Topics
- Object-oriented programming in Java.
- Basic GUI (Graphical User Interface) design
- Event-driven programming
- Concepts of pointers, references and indirection.
- Theory and usage of fundamental data structures:
- Concepts of pointers, references and indirection.
- Arrays
- Linked Lists
- Stacks
- Queues
- Hash Tables
- Heaps
- Trees
- Graphs
- Searching and Sorting Algorithms
- Recursion
Textbooks
I recommend two books for this class: one covering Java, one covering data structures.
- For Data Structures
- Drozdek's Data Structures and Algorithms in Java. (2nd or 3rd Ed.). Either the book or the electronic version are fine. This book is required. It's a very expensive book, but one that will be a good reference to keep.
- For Java
- Sierran and Bates' Head Frist Java. This book is recommended, and you can substitute another Java reference for it if you prefer, as long as it supports your learning in the course. Plan to hang on to these books, so you can brush up your skills later on and teach yourself new ones.
Requirements
Normally students will be expected to take CSC 111 before this course. Students who have taken an equivalent programming course or who have otherwise achieved the requisite programming skills should consult the instructor.
- Expected work includes
- Weekly programming assignments (labs and homeworks)
- Occasional written commentary and reflection
- An in-class midterm exam
- A self-scheduled final during the final exam period
- Final programming project with in-class presentation
This is a programming-intensive course, designed to help neophyte programmers make the transition into developing software engineers. While programming is one of the most interesting, challenging and rewarding intellectual activities out there, it is almost impossible to learn it without a lot of practice. This takes time, especially for debugging a troublesome program that isn't quite working right yet. Often the final small details can be the hardest to fix.
If you find yourself spending inordinate time debugging your programs, you should consult with me very soon -- before it becomes a problem and you fall behind or it affects your work in other courses. I can help you find strategies to use your time more effectively. Don't allow yourself to fall behind early in the course; it will be many times harder to catch up later!