Difference between revisions of "CSC212 Lab 4 2014"
(→Modification) |
(→Adding the Javadoc Comments) |
||
Line 157: | Line 157: | ||
* @param n the name of the animal | * @param n the name of the animal | ||
* @param a the age of the animal | * @param a the age of the animal | ||
− | * @param v true if animal is vaccinated, false otherwise | + | * @param v true if animal is vaccinated, false otherwise |
* @param t true if animal is tattooed, false otherwise | * @param t true if animal is tattooed, false otherwise | ||
*/ | */ | ||
Line 184: | Line 184: | ||
/** | /** | ||
* Main entry point. | * Main entry point. | ||
− | * @param args | + | * @param args the command line arguments. |
*/ | */ | ||
public static void main(String[] args) { | public static void main(String[] args) { | ||
Line 195: | Line 195: | ||
</source> | </source> | ||
<br /> | <br /> | ||
+ | |||
==Create the Javadoc== | ==Create the Javadoc== | ||
<br /> | <br /> |
Revision as of 22:10, 17 September 2014
--D. Thiebaut (talk) 21:12, 17 September 2014 (EDT)
Contents
Lab 4 deals with private member variables, and javadoc.
Private Member Variables
- Login to beowulf2.csc.smith.edu, grendel.csc.smith.edu, or use one of the Linux Mint machines.
- Create a new program called Animal1.java containing the code below:
public class Animal1 { boolean isVaccinated; boolean isTattooed; String name; int age; Animal1( String n, int a, boolean v, boolean t ) { name = n; age = a; isVaccinated = v; isTattooed = t; } public void displayBasicInfo( ) { String v = "vaccinated"; if ( !isVaccinated ) v = "not " + v; String t = "tattooed"; if ( !isTattooed ) t = "not " + t; System.out.println( String.format( "%s (%d), %s, %s", name, age, t, v ) ); } }
- In the same directory, create a second file called TestAnimal1.java, which contains this code:
class TestAnimal1 { TestAnimal1() { } public static void main(String[] args) { // create a new animal Animal1 a = new Animal1( "Max", 3, false, true ); a.displayBasicInfo(); // modify it. Then display it. a.isVaccinated = true; a.isTattooed = false; a.age = 5; a.displayBasicInfo(); // modify it some more, then display it. a.isTattooed = ! a.isTattooed; a.age = a.age + 1; a.displayBasicInfo(); } }
- Compile and run both:
javac Animal1.java TestAnimal1.java java TestAnimal1
- Verify that you get the following output:
Max (3), tattooed, not vaccinated Max (5), not tattooed, vaccinated Max (6), tattooed, vaccinated
Modification
- Modify the Animal1.java program and make all its member variables private.
private boolean isVaccinated; private boolean isTattooed; private String name; private int age;
- Recompile both java programs. Notice that, now, TestAnimal1.java generates many errors. Why?
- If you answer that it is because TestAnimal1 cannot access the member variables of a any longer, you are right!
- Modify both Animal1 and TestAnimal1 so that
- Animal1 will now have mutator and inspector methods to access its member functions, and
- TestAnimal1 will access the member variables of a by using the new accessors and mutators
- Here is an example of a modification you can make on Animal1.java
// mutator public void setAge( int n ) { age = n; } // ispector public int getAge( ) { return age; }
- And here is an example of a modification you can make on TestAnimal1.java:
a.setAge( 5 );
- When you have been able to get rid of all the compiler errors for TestAnimal1.java, run the program TestAnimal1 and verify that you get the same output as before:
Max (3), tattooed, not vaccinated Max (5), not tattooed, vaccinated Max (6), tattooed, vaccinated
Javadoc
Adding the Javadoc Comments
- You need to be connected to your 212a-xx account on one of the Linux machines for this all section to work.
- Locate your old Animal.java class from a previous lab, or copy/paste it from here.
- Don't worry if the member variables are not private. In this section we're interested in generating the javadoc for this program.
- Add documentation to your program using the same format as shown below. While it might be tempting to copy/paste it, please type it. It's the best way to remember how the javadoc syntax works:
/** * implements a base class for an animal. * @author thiebaut * */ public class Animal { private boolean isVaccinated; // boolean holding the property for vaccination private boolean isTattooed; // boolean holding the property for tattoo private String name; // contains the animal's name private int age; // equal to the animal's age /** * constructor * @param n the name of the animal * @param a the age of the animal * @param v true if animal is vaccinated, false otherwise * @param t true if animal is tattooed, false otherwise */ Animal( String n, int a, boolean v, boolean t ) { name = n; age = a; isVaccinated = v; isTattooed = t; } /** * displays all the information about the animal. * <P> * Format:<br /> * Rex (3), tattoed, not vaccinated */ public void displayBasicInfo( ) { String v = "vaccinated"; if ( !isVaccinated ) v = "not " + v; String t = "tattooed"; if ( !isTattooed ) t = "not " + t; System.out.print( String.format( "%s (%d), %s, %s", name, age, t, v ) ); } /** * Main entry point. * @param args the command line arguments. */ public static void main(String[] args) { Animal a = new Animal( "Max", 3, false, true ); a.displayBasicInfo(); System.out.println(); } }
Create the Javadoc
- At the Linux prompt, enter the following commands:
command | description |
---|---|
javadoc -d ~/public_html/myjavadoc Animal.java |
creates the javadoc for Animal.java, and stores the resulting html files in the public_html folder of your home directory, in a subdirectory called myjavadoc. The -d switch specifies that we want the output files generated to go into a specific directory. |
chmod -R a+rx ~/public_html/myjavadoc |
changes the access permissions to all the files belonging to the subirectory you just created, named myjavadoc, in the directory public_html in your account. -R means: visit recursively all the files and subdirectories found inside myjavadoc. a+rx means: allow all to read and execute the files found. This way the Web Server on beowulf2 will be able to make your files available when requested by a Web browser. |
View the Javadoc
- Open a Web Browser, either on the Linux machine you are connected to, or on your laptop.
- In the URL bar, enter this URL, replacing the xx letters by your own 2-letter Id:
- Verify that you get html pages describing your program. Explore the various pages and views of your program documentation.
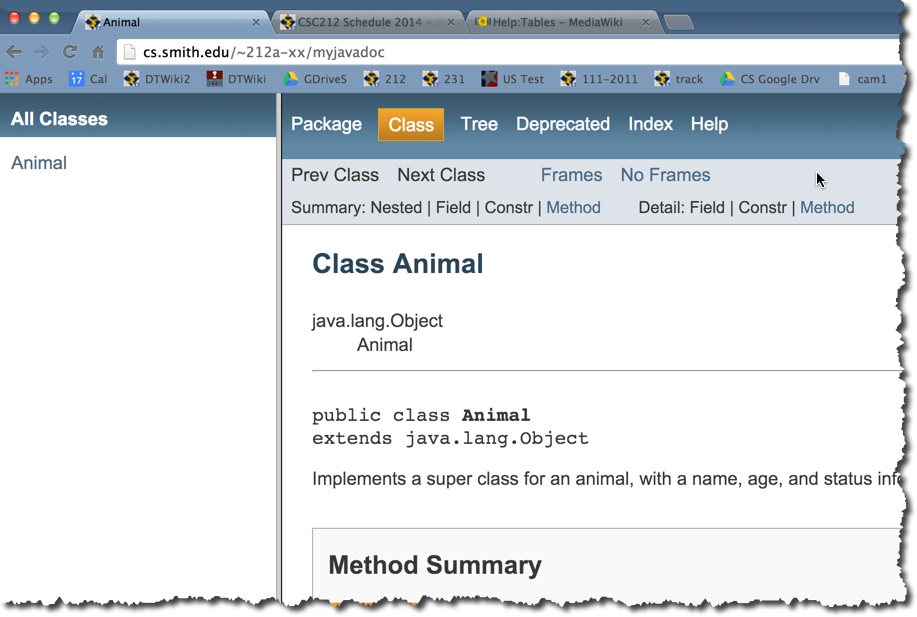
- Congratulations, you now know how to generate a sophisticated documentation package for your java programs!
Exercise
- Generate the javadoc for the Dog class, also available here.
- When generating the javadoc, use the following command:
javadoc -d ~/public_html/myjavadoc Animal.java Dog.java
- Verify that you get both the dog and the animal classes listed on the front page of the html javadoc
Moodle Question
- Answer the Moodle question for Lab 4, Question 1
Accessing Command Line Arguments
- Create this new Java program:
class PrintArgs { public static void main( String[] args ) { for ( int i=0; i<args.length; i++ ) System.out.println( String.format( "args[%d] = %s", i, args[i] ) ); } }
- Run it and pass it several words on the command line, as illustrated below (try different combinations of words and numbers):
javac PrintArgs.java java PrintArgs 1 2 3 4 5 java PrintArgs Thursday afternoon lab on a warm September day java PrintArgs "Smith College" is where "Sophia Smith" still lives java PrintArgs "Thursday afternoon lab on a warm September day" java PrintArgs "Thursday afternoon" lab on a warm September day java PrintArgs Thursday afternoon lab on a \"warm September\" day
Modification
- Modify the program so that if the first argument is "-up", the program will print all the arguments that follow in uppercase, otherwise it will display the arguments as it received them.
- To get the uppercase version of a string, use the toUpperCase() method of a String variable:
String name = "Smith College"; System.out.println( name.toUpperCase() ); // will print SMITH COLLEGE
- Example
[23:09:21] ~/public_html/classes/212$: java PrintArgs2 the quick red fox jumped over the dog the quick red fox jumped over the dog [23:10:04] ~/public_html/classes/212$: java PrintArgs2 -up the "quick red fox" jumped over the dog THE QUICK RED FOX JUMPED OVER THE DOG [23:11:38] ~/public_html/classes/212$: java PrintArgs2
- Note the lack of output for the last command!