Difference between revisions of "CSC111 Lab 9 2015"
(→Part 2: Exercise) |
(→Part 2: Exercise) |
||
Line 101: | Line 101: | ||
def main(): | def main(): | ||
+ | # create a text file for use later... | ||
createTextFile( "csc111.txt" ) | createTextFile( "csc111.txt" ) | ||
+ | # test first function | ||
example1() | example1() | ||
+ | # test second function | ||
L = [ 10, 3, 5, 6, 9, 3 ] | L = [ 10, 3, 5, 6, 9, 3 ] | ||
example2( L ) | example2( L ) | ||
− | example2( [ 10, 3, 5, 6, "NA", 3 | + | #example2( [ 10, 3, 5, 6, "NA", 3 ] ) |
− | |||
− | + | # test third function | |
+ | fileName = input( "Enter name of file to display (type csc111.txt): " ) | ||
+ | printUpperFile( fileName ) | ||
main() | main() | ||
Line 118: | Line 122: | ||
− | * The program | + | * The program above has many flaws; it is not very robust. We can easily make it crash. |
− | * Observe each function | + | * Observe each function. Run your program a few times. |
− | * | + | * Figure out how to make each function crash |
+ | * Go ahead and start forcing the first function to crash. Register the <font color="red">'''XXXXError'''</font> that is generated. For example, if the output of the crash looks like this: | ||
<tt><font color="red"> | <tt><font color="red"> | ||
Traceback (most recent call last): | Traceback (most recent call last): | ||
Line 143: | Line 148: | ||
</pre></code> | </pre></code> | ||
− | * | + | * Add the try/except statement to the function, and verify that your code does not crash on the same input. |
<br /> | <br /> | ||
Revision as of 08:00, 29 March 2015
--D. Thiebaut (talk) 07:14, 29 March 2015 (EDT)
Exceptions
Part 1: Preparation
- Create a new program called lab9_1.py, and copy this code to the new Idle window.
# lab9_1.py # Your name here # getInput: returns an integer larger # than 0. Expected to be robust def getInput(): while True: x = int( input( "Enter an integer greater than 0: " ) ) if x <= 0: print( "Invalid entry. Try again!" ) else: return x def main(): num = getInput() print( "You have entered", num ) main()
- Test it with numbers such as -3, -10, 0, 5. Verify that the input function works well when you enter numbers.
- Test your program again, and this time enter expressions such as "6.3", or "hello" (without the quotes).
- Make a note of the Error reported by Python:
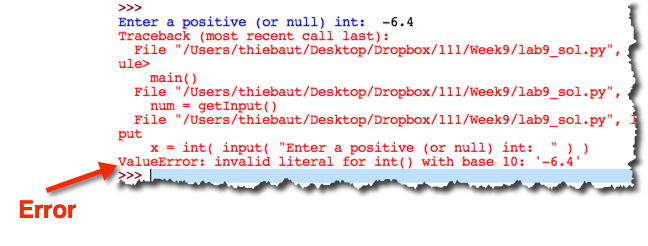
- Modify your function and add the highlighted code below by hand. This code will catch the ValueError exception.
# getInput: returns an integer larger # than 0. Catches Value errors def getInput(): # repeat forever... while True: # try to get an int try: x = int( input( "Enter an integer greater than 0: " ) ) except ValueError: # the user must have entered something other than an int print( "Invalid entry. Not an integer. Try again!" ) continue # No errors caught. See if the number is negative if x <= 0: print( "You entered a negative number. Try again!" ) else: # finally, we can return x as it is an int that is >0 return x
- Run your program and try different invalid inputs, such as strings or floats. You can also try just pressing the Return key, indicating that you are not providing anything to the input function. Verify that your program catches all these invalid entries and does not crash.
Part 2: Exercise
- Create a new program called lab9_2.py with the code below:
def example1(): for i in range( 3 ): x = int( input( "enter an integer: " ) ) y = int( input( "enter another integer: " ) ) print( x, '/', y, '=', x/y ) def example2( L ): print( "\n\nExample 2" ) sum = 0 for i in range( len( L ) ): sum += L[i] print( "sum of items in ", L, "=", sum ) def printUpperFile( fileName ): file = open( fileName, "r" ) for line in file: print( line.upper() ) file.close() def createTextFile( fileName ): file = open( fileName, "w" ) file.write( "Welcome\nto\nCSC111\nIntroduction\nto\nComp.\nSci.\n" ) file.close() def main(): # create a text file for use later... createTextFile( "csc111.txt" ) # test first function example1() # test second function L = [ 10, 3, 5, 6, 9, 3 ] example2( L ) #example2( [ 10, 3, 5, 6, "NA", 3 ] ) # test third function fileName = input( "Enter name of file to display (type csc111.txt): " ) printUpperFile( fileName ) main()
- The program above has many flaws; it is not very robust. We can easily make it crash.
- Observe each function. Run your program a few times.
- Figure out how to make each function crash
- Go ahead and start forcing the first function to crash. Register the XXXXError that is generated. For example, if the output of the crash looks like this:
Traceback (most recent call last): File "/Users/thiebaut/Desktop/except0.py", line 29, in <module> main() File "/Users/thiebaut/Desktop/except0.py", line 27, in main example3( [ 10, 3, 5, 6 ] ) File "/Users/thiebaut/Desktop/except0.py", line 18, in example3 sum = sum + L[i] IndexError: list index out of range
- what you are interested in is IndexError. This is the exception you want to guard your code against.
try:
........
........
except IndexError:
.........
- Add the try/except statement to the function, and verify that your code does not crash on the same input.
Solution
def example1():
while True:
try:
x = int( input( "enter a number: " ) )
y = int( input( "enter another number: " ) )
print( x, '/', y, '=', x/y )
break
except ZeroDivisionError:
print( "Can't divide by 0!" )
except ValueError:
print( "That doesn't look like a number!" )
except:
print( "something unexpected happend!" )
def example2( L ):
print( "\n\nExample 2" )
print( "L = ", L )
sum = 0
sumOfPairs = []
for i in range( len( L ) ):
try:
sumOfPairs.append( L[i]+L[i+1] )
except IndexError:
continue
except TypeError:
continue
print( "sumOfPairs = ", sumOfPairs )
def printUpperFile( fileName ):
try:
file = open( fileName, "r" )
except FileNotFoundError:
print( "***Error*** File", fileName, "not found!" )
return False
for line in file:
print( line.upper() )
file.close()
return True
def main():
example1()
L = [ 10, 3, 5, 6, 9, 3 ]
example2( L )
L = [ 10, 3, "NA", 6, 9, 3 ]
example2( L )
open( "doesNotExistYest.txt", "w" ).close()
printUpperFile( "doesNotExistYest.txt" ):
printUpperFile( "./Dessssktop/misspelled.txt" )
main()