CSC111 Midterm Exam Preparation 2015
--D. Thiebaut (talk) 18:08, 28 February 2015 (EST)
Contents
Midterm Exam Prep
The midterm will be an in-class exam given under the rules of the honor code. It is a 70-minute exam. It will be closed books, closed notes, closed computers. The exam is comprehensive and covers all the material seen in class, up to the date of the exam. The questions below will give you a taste for the type of questions you will find in the exam. Work on each question on paper, possibly interacting with people around you if you get stuck. You can find the answers different ways: a) by going to the special lab session dedicated to the midterm preparation, b) by reading the text book, c) by using Idle and writing up a solution, and/or d) by reviewing the lectures notes for each week.
Question 1
What does OOP refer to?
Question 2
What does camelcase refer to? Give an example of camelcase.
Question 3
What is the output of the following program?
def main():
print( "main" )
for i in range( 3 ):
print( i, end="-" )
print( "done!" )
main()
Question 4
What is this?
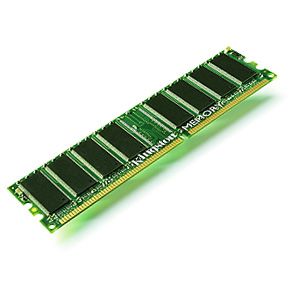
How does it relate to programming and to Python?
Question 5
What is printed by this program?
a = "Hello" b = "There" c = 123 d = a, b, c c, a, b = d print( a, b, c, d )
Question 6
Write a for-loop that prints all the numbers multiple of 5, ranging from -100 and 100, included. The numbers should be output one per line. The first line will contain -100, the next line, -95, then -90, down to, 95, and 100, on the last line.
Question 7
Study this piece of code:
a = 3 b = 11 c = 21 d = (b+c) / a
When the last assignment is executed, will d be a float or an integer?
Question 8
amount = float( input( "Enter amount: " ) )
Continue writing code that will output the number of $5-bills, $1-bills, and quarters that makes up the amount entered by the user. We assume the user can enter integer numbers or real numbers. 21 is a valid input. 7.76 is also valid. For the first number, your program will output 4, 1, 0, indicating that 4 $5-bills, 1 $1-bill, and 0 quarters. For the second number, the program will output 1, 2, 3, indicating 1 $5-bill, 2 $1-bill, and 3 quarters.
Question 9
a = eval( input( "Enter a number" ) ) print( ... ) print( ... ) print( ... )
Assuming that the user enters the number 123.456789, complete the 3 print statements so that the output of the program becomes:
a = 123.46# a = ##123## a = 123.4568 #
Note that there is 1 space between the equal sign and the number on the first line. There are 2 spaces between the equal sign and the #-sign on the second line. There is one space before and after the number on the third line.
Question 10
Part 1
What is printed by this program?
def main0(): print( "Hello World" ) main() def main1(): print( "Hello CSC111!" ) main0() def main(): print( "This line is the only one printed" ) main()
Part 2
What is printed by this program:
def main0(): print( "Hello World" ) def main1( d, n ): print( "Hello {0:3}-{1:3}!".format(d,n ) ) def main( n ): print( "This line is the only one printed" ) main1( "CSC", n ) main(111)
Question 11
Assume that we have a list called numbers (shown below) containing many numbers. We only show 5 numbers, but the list contain many more. Write the code that will add all the numbers up and print out their sum.
numbers = [ 1, 10, -3, 20, ..., 5 ]
Question 12
Assume that farm is a Python list that contains several names of animals. It contains more than 20 different animals. Only four are shown in the code below, for simplicity.
farm = [ "dog", "cat", "horse", ... , "mouse" ]
Write the code that will print the sum of the length of all the words. For example, if the list contains [ "dog", "horse" ], the program would print 8, which is 3 + 5, the length of "dog" plus the length of "horse".
Question 13
What is a bit?
Question 14
Write a function called firstLetter that takes one argument, a list of farm animals like ['pig' ...]. The function should print the first letter of every animal in the list on a separate line.
For example, if the list is equal to farm = [ "Horse", "Cat", "Dog", "Mouse"], the program will print H, C, D, and M, on separate lines.
Question 15
What is printed by this program?
line = "Hello World!" for i in range( 1, 4 ): print( line[ :i ], line[i: ] )
Question 16
What does immutable mean? What does this term apply to?
Question 17
Assume that we have strings that containing several words, including a phone number. All the strings are known to contain a phone number. The area code of the phone number is made of 3 digits, and is always included in parentheses. There are never spaces between the parentheses and the digits. There is only 1 open-parenthesis in the strings.
Below is an example of a string containing such a pattern.
Smith College, Massachusetts, Hampshire County, +1 (413) 585 2700, student population: 2,400.
Write the python code necessary to extract the area code and store it in a variable called areaCode. The contents of the variable will be just 3 digits. For the string shown above, the variable areaCode would be assigned "413" by your code.