CSC212 Midterm Exam Prep 2014
--D. Thiebaut (talk) 17:52, 7 October 2014 (EDT)
This is a sample of problems to get you ready for the midterm exam. The midterm will be closed-book, closed-computers, and on paper.
Problem #1
- Fill in the spaces marked with ____ to complete the following Boolean expressions. Do not use the Boolean operator "!" in your answer. Write only in the spaces marked with ____.
- Assume that X, Y and Z are integer variables.
- Question 1
- Write the expression that is true if X is strictly the largest of the three variables X, Y and Z. For example, if X is 50, Y is 40 and Z is 45, the expression should be true, but if X is 50, Y is 40, and Z is 50, the expression should be false, since X is not strictly greater than Z.
(______) _____ (______)
- Question 2
- Assume that s1, s2 and s3 are Strings.
- Write the expression that is true if s1 is strictly the largest of the three variables s1, s2 and s3, in alphabetical order. For example, if s1 is "alpha", s2 is "beta" and s3="gamma", the expression should be false, but if s1 is "zeta", s2 is "epsilon", and s3 is "alpha", the expression should be true.
(______) _____ (______)
Problem #2
- Assume that we have an array of ints, table, declared as follows:
int[] table = new int[] { 10, 20, 5, 15, 25, 0, 3 };
- Question 1
- Write the java code required to print all the elements of the array table.
- Question 2
- write the java code required to find the largest element of table and print it on the screen.
- Question 3
- write the java code required to replace all the 0 element of table with -1.
Problem #3
Given this code, and assuming that the user enters the number 3 at the keyboard, followed by ENTER; what is the output of the program?
Scanner scan = new Scanner( System.in ); int n, k, max; max = scan.nextInt(); /* read value for max */ for (n = 0; n < max; n++) { for (k = 0; k < max; k++) { if (n > k) System.out.print(" G "); else if (n < k) System.out.print(" L "); else System.out.print(" E "); } System.out.println(); }
Problem #4
- Assume the ArrayList fibonacci is initialized as follows:
ArrayList fibonacci = new ArrayList(); fibonacci.add( 1 ); fibonacci.add( 1 );
- Question 1
- Write a for-loop that will compute 10 more fibonacci term and store them in the array.
- Question 2
- Same question, but using a LinkedList instead of an ArrayList.
- ArrayList
- use add( Object o ) to add an element, and get( int index) to access an element at some location.
- LinkedList
- use add( Object o ) to add an element, and get( int index ) to access an element at some location.
Problem #5
- Question 1
- In a linked list containing several thousands of items, do the remove-from-tail and the remove-from-front operations take the same amount of time to operate? Why or why not?
Problem #6
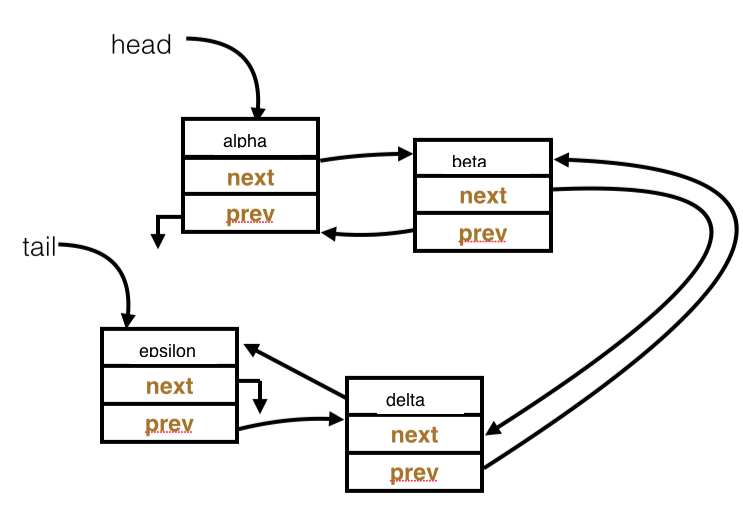
Problem #6