CSC111 Lab 8 2018
D. Thiebaut (talk) 14:42, 25 March 2018 (EDT)
This lab continues on with the lab from last week. You will need to add obstacles in the graphic window, and make your circle bounce on obstacles, or get stuck to obstacles.
Contents
Adding an Obstacle
- Take your graphic program from last week, where you made a colored circle move around the graphic window, bouncing off the sides.
- Add a rectangle in the middle of the graphic window. You should declare it at the beginning of your main() function, as illustrated here:
def main(): win = GraphWin( "Lab 7 Moving ball", 600, 400 ) # create a green obstacle in the middle of the window x1 = 200 # feel free to use different coordinates y1 = 200 x2 = 250 y2 = 250 obstacle1 = Rectangle( Point( x1, y1 ), Point( x2, y2 ) ) obstacle1.setFill( "green" ) obstacle1.draw( win ) # the remaining part of your code follows below...
Challenge 4 |
- Make your ball stop when its center enters the green rectangle. The image below illustrates this situation.
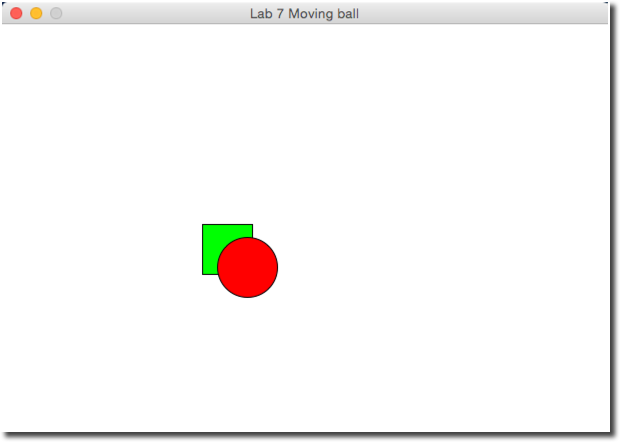
- Hints: to make an object stop, you can simply set its displacement to 0, so that the while-loop makes it move by 0 pixels every loop.
Challenge 5 |
- Modify your program one more time, and this time the ball will bounce off the obstacle. When you detect that the center of the ball is inside the green rectangle, change dx and dy to their opposite. Note that this will make the ball bounce back on the same path it came from. It is quite challenging to make the ball bounce in a realistic way, and you are welcome to try to make it happen, but it's trickier. Bouncing off in the opposite direction is fine for this lab!
if ... : dx = -dx dy = -dy
Adding a Second Obstacle
- Add a second rectangle in the graphic window. Make it magenta in color. You should declare it at the beginning of your main() function, as illustrated here:
def main(): win = GraphWin( "Lab 7 Moving ball", 600, 400 ) # create a green obstacle in the middle of the window x1 = 200 y1 = 200 x2 = 250 y2 = 250 obstacle1 = Rectangle( Point( x1, y1 ), Point( x2, y2 ) ) obstacle1.setFill( "green" ) obstacle1.draw( win ) # create another green rectangle on the right of the first one x3 = 350 y3 = 200 x4 = 400 y4 = 250 obstacle2 = Rectangle( Point( x3, y3 ), Point( x4, y4 ) ) obstacle2.setFill( "magenta" ) obstacle2.draw( win ) # the remaining part of your code follows below...
- Feel freel to position it at a different location than the one used above.
Challenge 6 |
- Make your ball stop when its center is fully inside the first green obstacle, and bounce off the magenta obstacle. For this challenge, simply make the ball bounce off the second obstacle when its center enters the obstacle.
- If you find that the ball never gets to hit both boxes, you may want to change the initial direction and add decimal digits to the dx and dy to create an angle that yields a path that eventually will hit the obstacles. For example:
dx = 5.111 dy = -2.51
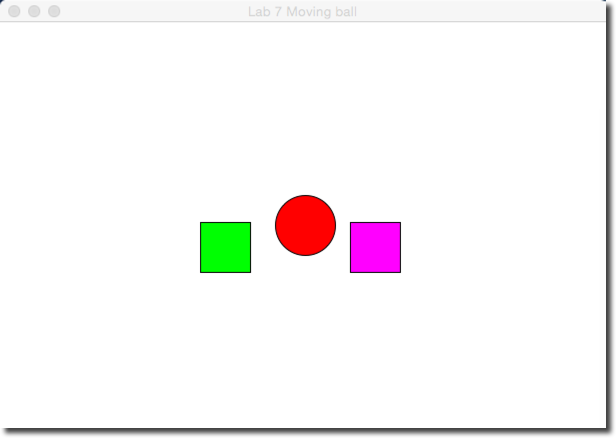
Moodle Submission
- Take a screen capture of the graphics window showing the ball and the two obstacles, and submit the jpg or png file to Moodle, in the Lab 7 section.