CSC111 Final Exam 2014
Contents
CSC111 Final Take-Home Exam 2014
This final exam is take-home. It is open-books, open-notes, and open-Web. It is due a week after it is made available, at 4:00 p.m. on Friday May 9th, 2014.
You cannot discuss the details of this exam with anyone except your instructor. You cannot work in pair-mode. The TAs are not allowed to help you out in any way. No question will be answered in person after the last CSC111 class. All questions about the exam should be posted on Piaza. If you are not sure whether your question should be made public because it might contain revealing information about your code, make your question private when you post it.
The exam is given under the rules of the Smith College Honor Code.
Make sure you reference all work/resources in the header of your code. This includes Web sites you visited and from which you may have taken some code sections. You do not need to reference any code taken from the CSC111 class pages.
You must submit a solution for Problem 1, and one solution for either Problem 2 or Problem 3. You may submit solutions for all three problems. In this case the exam grade will be computed as gradeProblem1 + max( gradeProblem2, gradeProblem3 ). All programs are worth 50 points max.
Problem #1 (50 points)
Store the code below in a file called finaltext.py.
text = """person, Marion, 31, married, Amherst, Marion887@gmail.com
person, George, 66, single, Cambridge, George113@gmail.com
person, Alexandra, 79, single, Northampton, Alexandra2013yoyo@gmail.com
person, Francis, 79, single, Northampton, Francis2013yoyo@gmail.com
person, Cesca, 55, married, Amherst, Cesca2013yoyo@gmail.com
person, Stella, 31, single, Boston, Stella113@gmail.com
person, Alma, 20, single, Cambridge, Alma2013yoyo@gmail.com
dog, Miko, 4, Australian Shepherd, vaccinated, not trained, tattooed, Cesca
dog, Fido, 4, Australian Shepherd, not vaccinated, not trained, not tattooed, Stella
dog, Max, 3, Australian Shepherd, not vaccinated, trained, not tattooed, Francis
dog, Bergere, 4, Australian Shepherd, vaccinated, not trained, tattooed, Marion
dog, Mika, 1, Beagle, vaccinated, not trained, tattooed, Stella
dog, Luna, 1, Terrier, vaccinated, not trained, not tattooed, Alma
dog, Bella, 3, Akita, not vaccinated, not trained, not tattooed, Stella
dog, Rintintin, 2, Terrier, not vaccinated, trained, tattooed, Marion
dog, Oscar, 4, Beagle, not vaccinated, not trained, tattooed, Alexandra
dog, Mirabelle, 4, Beagle, vaccinated, trained, not tattooed, Alexandra
cat, Jabba, 3, Persian, vaccinated, outdoors, not tattooed, Francis
cat, Yago, 3, Toyger, vaccinated, outdoors, tattooed, George
cat, Calico, 5, Persian, not vaccinated, indoors, not tattooed, Alexandra
cat, Callas, 4, Toyger, not vaccinated, outdoors, tattooed, George"""
The list above is a list of people, dogs, and cats. In order to use it in your program simply add this line at the top of your program:
from finaltext import text
Your Assignment
Write a program called finala.py that will read this string text, parse it, store it in some data structure, and output the answers to the following questions:
- Question 1
- Print a list of all the people, nicely formatted, followed by a list of the dogs, followed by a list of the cats. An example of the format to use is shown below:
George (George113@gmail.com), 66 years old, single, lives in Cambridge Mirabelle (dog), 4 years old, Beagle, vaccinated, trained, not tattooed, (owner: Alexandra) Calico (cat), 5 years old, Poodle, not vaccinated, indoors, not tattooed, (owner: Alexandra)
- Question 2
- Print the name of the people only using the format shown above, and follow each one by the number of dogs or cats he or she owns. Example:
Alexandra (Alexandra2013yoyo@gmail.com), 79 years old, single, lives in Northampton, 2 dogs, 1 cat
- If the person does not own pets, then do not add anything after the city where they live. Do not print a comma at the end of the line!
- Question 3
- Print the name and city only of all the owners of Australian Shepherd dogs.
- Question 4
- Print the name and city only of the owner or owners of the oldest dog
- Question 5
- Print the name and city of the owners with the most pets
- Question 6
- Print the email addresses, and only the email addresses, of all the people who have unvaccinated pets, so that we can remind them to have them vaccinated.
Grading
- A maximum of 30 points will be given to well documented programs that answer all questions using regular parsing methods, but not using any new classes.
- A maximum of 40 points will be given to well documented programs that answer all the questions and that create new classes and objects to hold the various pieces of information.
- A maximum of 50 points will be given to well documented programs that answer all the questions and that use classes derived from the People and Dog classes of Problem 2 of Homework 10. In this case you must copy and not modify the two classes into your program, and create new classes derived from them. Feel free to organize your new classes as you feel will help you answer the questions the most easily. In other words, make life easy for yourself!
Submission
Submit the program finala.py at this url.
Problem #2 (50 points)
Write a JES program that takes an image and generates a circular soft mask around the center of the image, as illustrated below. On the left is the original image, and on the right the image generated by JES.
Testing
Run your program on the following image, and submit a copy of the processed image under the name finalb.jpg or finalb.png.
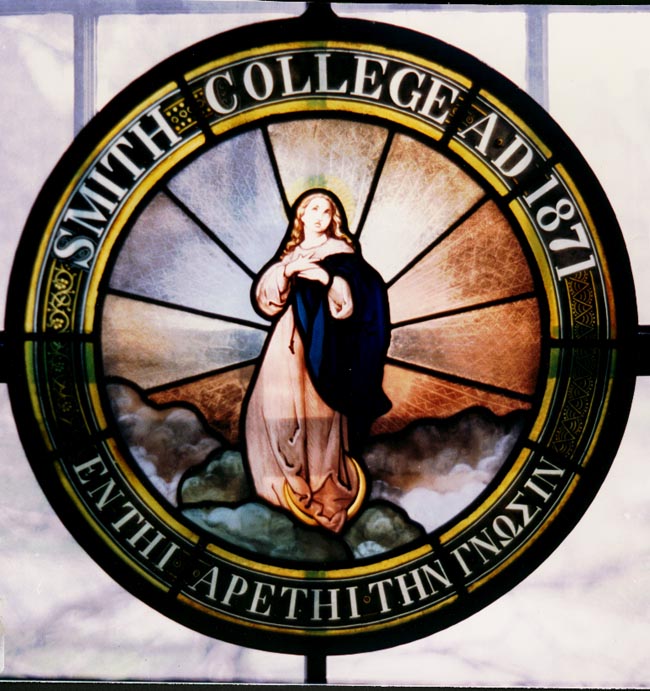
(click on the image twice to get to the real full size image)
Implementation Details
- The circular part of the mask should be as big as the width or the height of the image, whichever is smaller
- The mask color must be white.
- The resulting image should be fully white outside the circular mask.
- The amount of softness inside the circle is up to you. But there should be some softening of the colors! The pixel at the very center of the foggy image should retain its original color.
- Your JES program should only ask the user for a file name, nothing else.
- If you need help capturing the final image, please visit this page.
Submission
- Your program should be called finalb.py and the image finalb.png or finalb.jpg, and both should be submitted to the URL indicated in Problem #1 above.
Problem #3: An Orange Tree (50 points)
Write a well documented and organized Python program that will generate a tree as close to the tree shown below as possible. You should not reproduce the text in the image; just the tree, its colors, and its fruit.
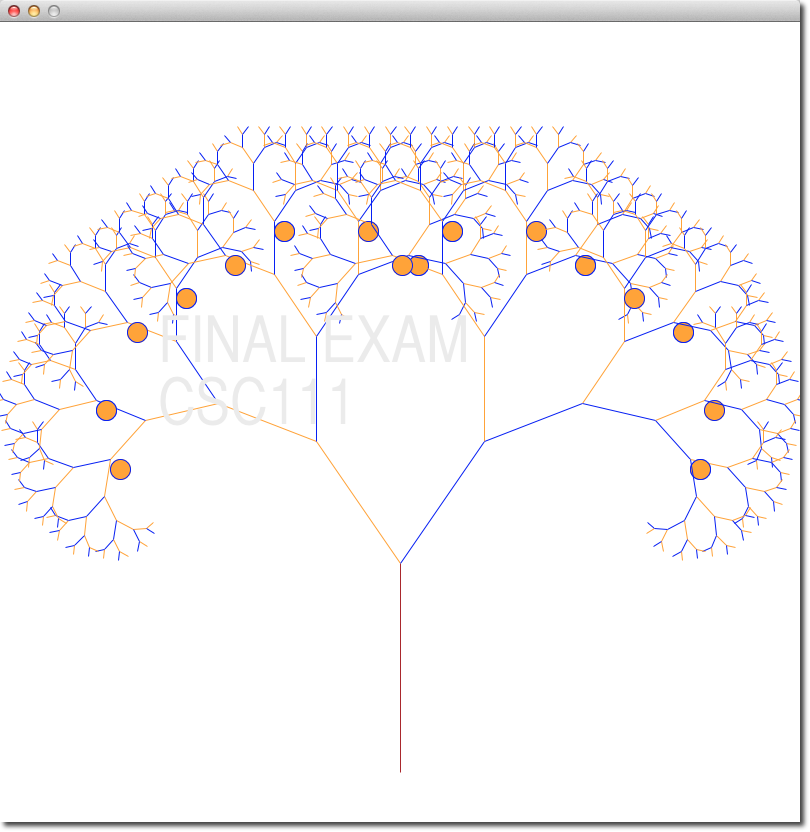
- Call your program finalc.py and the image finalc.jpg or finalc.png. Submit both to the same URL indicated in Problem #1.
- If you need help capturing the final image, please visit this page.
- Note: To change the color of a line with the graphics library (use graphics111.py, please), you should use the setOutline() method rather than the setFill() method.
- I used only 3 colors to generate the tree above: "orange", "blue", and "brown".
Good luck!