Qt4/Qt-Creator Read MySql Table (Console Mode)
--D. Thiebaut 15:11, 24 February 2011 (EST)
Contents |
Setup
We assume that you have installed Qt Creator and Qt 4 on your Mac (or Windows/Linux platform).
Steps
- Follow the steps of the Hello World example, and create a project called mySqlSimpleTable.
- Enter the code below in main.cpp:
// mySqlSimpleTable project
// main.cpp
// D. Thiebaut
// This program accesses a MySql database server, opens up a
// connection to a database (called dummy), with user name
// "dummy" and password "xxxxxx", and reads the full contents
// of a table called "table1".
// The contents of the table is dumped on the console.
// Good information on Qt and QSqlQueries can be found here:
// http://doc.qt.nokia.com/latest/qsqlquery.html
// Table format:
//CREATE TABLE IF NOT EXISTS `table1` (
// `Id` int(11) NOT NULL auto_increment,
// `word` varchar(45) default NULL,
// PRIMARY KEY (`Id`)
//) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
//
#include <QtCore/QCoreApplication>
#include <QtSql>
#include <qtextstream.h>
#include <iostream>
using namespace std;
int main(int argc, char *argv[]) {
//--- use a QTextStream: it makes it easier to output ---
//--- QString to cout. ---
QTextStream cout(stdout, QIODevice::WriteOnly);
//--- define the database connection ---
QSqlDatabase db = QSqlDatabase::addDatabase("QMYSQL");
db.setHostName("xgridmac.dyndns.org"); // host
db.setDatabaseName("dummy"); // database
db.setUserName("dummy"); // user
db.setPassword("dummy"); // password
//--- attempt to open it ---
bool ok = db.open();
if ( ok ) {
//--- we're good! ---
cout << "Database open\n";
//--- run a query and print data returned ---
QSqlQuery query( "select * from table1" );
while (query.next()) {
int Id = query.value(0).toInt();
QString word = query.value(1).toString();
cout << QString( "%1\t%2\n").arg( Id).arg( word );
}
//--- add a new entry to the table ---
query.prepare( "INSERT INTO table1 (Word) VALUES ( :word ) ");
query.bindValue( ":word", "Banana" );
query.exec();
//--- close connection to database
db.close();
}
else
//--- something went wrong ---
cout << "Error opening database\n";
return 0;
}
QtCreator Screen-Shot
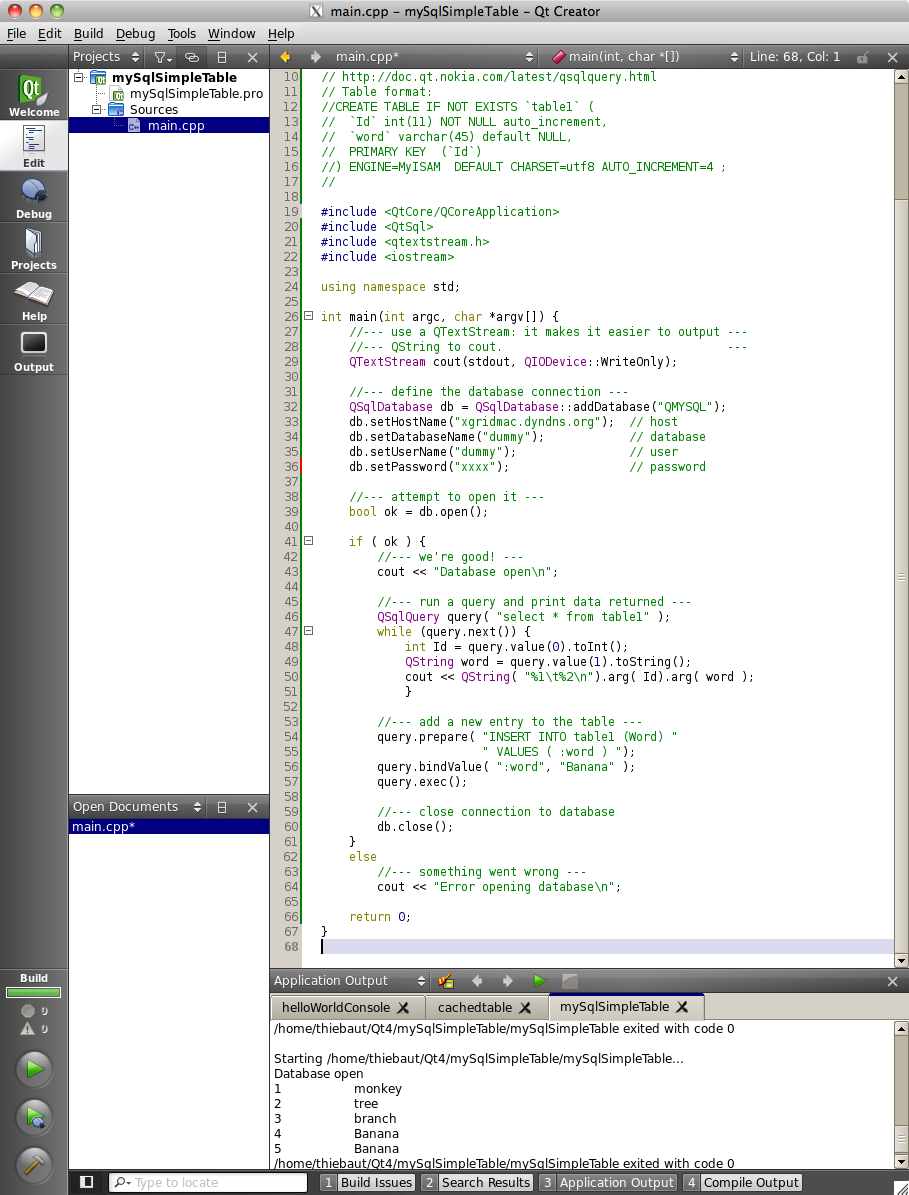