CSC111 Lab 7 2011
--D. Thiebaut 10:37, 19 October 2011 (EDT)
Contents
Testing with the if statement
A great reference for this part of the lab is the section on the if statement on Python.org. Take a quick look at it to see what it describes so that you will know where to go while working on this lab.
- Create a program with the following code:
def main():
print( "Please enter 2 numbers:" )
x = eval( input( "> " ) )
y = eval( input( "> " ) )
print( x, "/", y, "=", x/y )
main()
- Run the program a few times and check that it returns the correct information.
- Run it again and enter 0 for the second value. See what happens.
- Make your program robust by using an if-statement:
def main():
print( "Please enter 2 numbers:" )
x = eval( input( "> " ) )
y = eval( input( "> " ) )
if ( y != 0 ):
print( x, "/", y, "=", x/y )
else:
print( "Illegal value for y: division by 0 is not allowed or defined" )
main()
- Try it now! Verify that your program is now robust, i.e. you cannot make it crash (at least if you are entering numbers and not random strings!)
Challenge 1 |
- Using the above code as example, modify the program so that it asks for two numbers and prints out which number is the smallest and which is the largest.
Challenge 2 |
- Modify your code for the previous challenge and remove the two eval functions. This way your two variables will contain strings, and not numbers. Do not change the remaining part of your program and run it.
- Question 1
- What does your program do? What does it detect in the two strings you enter. For example if you enter "chocolate" and "milk" as the two strings, which will it report as the smallest? Why? What about "chocolate" and "MILK"? Why?
- Question 2
- Think of a way to make your program report that "chocolate" is lower than "milk", and that "chocolate" is also lower than "MILK"
Tests and Graphics
- Create the following program and run it.
from graphics import *
import time
def main():
w = 500
h = 500
radius = 20
win = GraphWin( "lab 7", w, h )
c = Circle( Point( w/2, h/2 ), radius )
c.setFill( "magenta" )
c.draw( win )
dirX = 5 # speed in the horizontal direction
for step in range( 1000 ):
c.move( dirX, 1 )
# uncomment next line if ball moves too fast
# time.sleep( 0.05 ) # 5/100th sec.
if c.getCenter().getX() < 0 or c.getCenter().getX() > w ):
dirX = -dirX
Text( Point( w//2, h//2 ), "Click me to quit!" ).draw( win )
win.getMouse()
win.close()
main()
- Notice how the ball moves. dirX is the amount of pixels it goes to the right (if dirX is positive), and 1 is the amount of pixels it goes down.
- The if statement says "if the X coordinate of the center of the circle is less than 0 or greater than w, then change the horizontal direction." Changing the direction here means simply changing its sign. If dirX is +5, that means the ball is going to the right, then making it -5 will make the ball go to the left. If dirX is -5, then changing it to +5 means that the ball was going left, and then will go right.
Challenge 3 |
- Make the ball bounce off the horizontal and vertical sides of the graphics window
Challenge 4 |
- Make the ball change color when it crosses the middle of the graphics window. In other words, make it be, say, yellow, in the left half of the window, and red in the right half of the window.
Challenge 5 |
- You will notice that the ball bounces off the "wall" when it is already half way through it (as illustrated below). Modify your code so that the ball bounces off the side of the window when it "touches" the side.
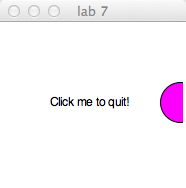
Happy Birthday with Functions!
- Write a program that will use functions and that will display a "Happy Birthday" message on the screen for somebody who's name is provided by the user. Example
Please enter name of birthday person: Alicia
Happy birthday to you, Happy birthday to you, Happy birthday to you, Dear Alicia, Happy birthday to you!
- Requirements. Your program should contain:
- One function, hbday(), that prints "Happy birthday to you"
- One function, dear( ... ), that receives the name of the person as a parameter and prints "Happy birthday, dear xxxxx", where xxxx represents that name.
- One function, singSong(), that gets the name of the person and prints the whole song (as illustrated above) with the name of the person.
- The main program, which you cannot modify, asks the user for a name and "sings" the song:
def main(): name = input( "Please enter name of birthday person: " ) singSong( name )
Challenge 6 |
- Go ahead and write the program.
Challenge 7 |
- Assume that you need to print the song for everybody in the class. We saw earlier how to write a loop that prints all the accounts of the form 111a-aa, 111a-ab, 111a-ac, ... 111a-az. Modify your program so that it prints a happy birthday song for every 111a account!
- For example, the output will look like this:
Happy birthday to you, Happy birthday to you, Happy birthday to you, Happy birthday, dear 111a-aa, Happy birthday to you! Happy birthday to you, Happy birthday to you, Happy birthday to you, Happy birthday, dear 111a-ab, Happy birthday to you! Happy birthday to you, Happy birthday to you, Happy birthday to you, Happy birthday, dear 111a-ac, Happy birthday to you! ...
Done? If so, you may start working on your homework assignment
for this week.
It doesn't require you to use if-statements or functions, but feel free to do so if it makes
your life easier.