CSC103 Assembly Language Lab 2012
--D. Thiebaut 07:15, 20 February 2012 (EST)
Revised --D. Thiebaut 07:06, 4 October 2012 (EDT)
This lab deals with assembly language and the computer simulator. Work on this lab with a partner. It's easier to figure out some of these questions with another person.
Contents
Preparation
Start
- Point your browser to the simulator and start it (third button from the bottom)
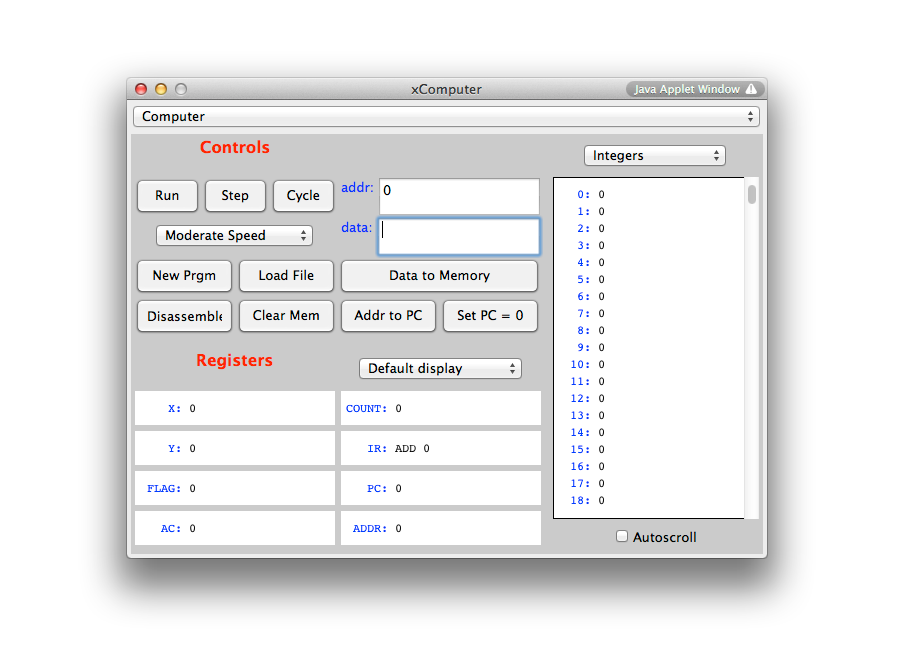
Editing Program
- Click on the top bar at the top of the simulator applet, which says computer
- In the edit window that opens up, enter this simple program:
- Enter a simple program in the window:
start: lod-c 1
sto var1
hlt
@10
var1: data
Translating/loading into RAM
- Click on translate
- Observe that your program has been translated, or coded into numbers. Numbers are the only thing the processor can understand.
- If the top right button says Integer, click on it so that it shows Instructions. Observe that numbers can mean instructions as well as numbers for the processor.
- Click on the top right button and see how the memory can be seen many different ways. The real contents of the memory is when you ask to see it in binary. That's what inside the memory of a computer at any given time. Bits. But because these bits are used in a code, they can mean different things.
Execute the Progam
Single Stepping
- Click on the button that says "Set PC = 0" to make sure the processor will execute the program starting at 0
- Click on the Cycle button and observe that the number 1 is going into the AC register and then into memory, at Address 10.
Running the program
- If you want to run the program full speed rather that executing it a step at a time, the procedure is slightly different.
- First, change the program so that it loads a number different from 1 into AC:
lod-c 55
- Translate
- set PC = 0
- Click Run instead of Cycle.
- This runs the program.
- Observe that 55 will be loaded up from memory into AC, and then stored into the variable var1, at Address 10.
- You now know the basics of programming in assembly language.
Problem 1
- Using the steps you have just gone through, write a program that initializes 3 variables called var1, var2, and var3 to the value 23. You should end up with the number 23 in three different memory locations.
Problem 2
- Something very similar to the previous problem, but instead of storing the same value in all three variables, store 10, 4, 12 (this could be today's date) var1, var2, and var3, respectively.
Problem 3
- Try this program out:
start: lod var1
sto var2
hlt
@10
var1: 55
var2: 0
- Run it.
- Observe the result, and in particular what happens to var2.
- Add two new variables to the data section: var3, and var4.
- Modify the program so that it copies the contents of var1 into var2, var3, and var4.
- Verify that your program works
- Modify the number in var1 and restart the program. Does the program put the new value in the other 3 variables?
Problem 4
- Try this program out:
start: lod var1
add-c 1
sto var1
hlt
@10
var1: 55
var2: 66
var3: 3
- Run the program
- What does it do? What's the result of the computation?
- Once you've figured it out, modify the program so that var1 originally starts with the value 5 in it, and then end with the value 7.
- Verify that your program will add 2 to var1 whatever its original value.
- Once your programs works, modify the program so that it adds two to all three variables.
Problem 5
- Try this new program out:
start: lod var1
loop: add-c 1
sto var1
jmp loop
lt
@10
var1: 55
var2: 55
- Run the program. Study it.
- Modify it so that as the program runs, var1 increases by 1 every step through the loop, and var2 decreases by one through the loop. In other words, when the program starts, var1 and var2 are 55. Then, a few cycles later, var1 is 56 and var2 is 54. Then a few cycles later, var1 is 57, and var2 is 53, etc.
Mini Challenge of the Day |
Write a program that contains 4 variables, var1, var2, var3 and sum. The first 3 are initialized with some numbers of your choice. sum initially contains 0.
Your program should computes 3 * var1 + 2 * var2 + var3 and store the result into the variable sum. For example, if var1 contains 10, var2 20, and var3 30, your program will perform the operations that will compute 3*10+ 2*20+ 30 = 100 which it will store in sum.
Hints: If you look closely at the set of instructions for our processor, there is no multiplication instruction... So your solution has to use another method for computing the value of 3 * var1...
Solutions
P1
; Solution for Problem 1
; D. Thiebaut
start: lod-c 23
sto var1
sto var2
sto var3
hlt
@10
var1: 0
var2: 0
var3: 0
P2
; Solution for Problem 2
; D. Thiebaut
start: lod var1
sto var2
sto var3
sto var4
hlt
@10
var1: 55
var2: 0
var3: 0
var4: 0
P3
; Solution for Problem 3
; D. Thiebaut
start: lod var1
add-c 1
sto var1
lod var2
inc
sto var2
lod var3
add-c 1
sto var3
hlt
@10
var1: 55
var2: 66
var3: 3
P4
start: lod var1
add-c 2
sto var1
lod var2
add-c 2
sto var2
lod var3
add-c 2
sto var3
hlt
@10
var1: 55
var2: 66
var3: 3
P5
; Solution for Problem 5
; D. Thiebaut
; First initialize both variables with 55
start: lod-c 55
sto var1
sto var2
; now loop and increment var1 and decrement var2
; the loop is endless.
loop: lod var1
add-c 1
sto var1
lod var2
dec
sto var2
jmp loop
hlt
@15
var1: 0
var2: 0