CSC111 Lab 7 2014
--D. Thiebaut (talk) 12:45, 10 March 2014 (EDT)
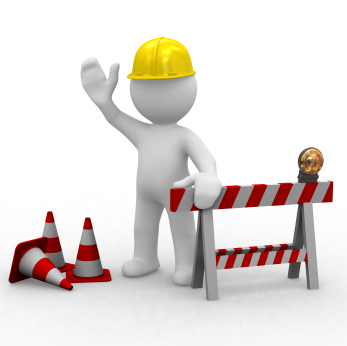
Contents
JES and Image Processing
Skeleton Program
# JES Picture processing
# D. Thiebaut
# A demo of some of the functions available in JES for manipulating
# the pixels of an image.
# the image we are playing with
image = None
# changeColor( image ).
# Changes the amount of red, green and blue that is in an image
def changeColor( image ):
for x in range(0,getWidth(image)):
for y in range(0,getHeight(image)):
pixel = getPixel (image, x, y)
red = getRed(pixel)
green = getGreen(pixel)
blue = getBlue(pixel)
# the line below replaces the pixel with its original color. Change
# the amount of red, green and blue to see some change in the colors
newColor = makeColor( red, green, blue )
setColor( pixel, newColor )
return image
# ==================================================================
# MAIN PROGRAM
# ==================================================================
file = pickAFile()
image = makePicture( file )
show( image )
#image = changeColor( image )
#repaint( image )
Problem 1: random processing
Write a function (use the skeleton program for a skeleton function with nested for-loops) that modifies the red, green and blue components of each pixel.
Problem 2: black and white
- Make your image black and white by storing the same value in the green, red, and blue component of a pixel. In a first step, pick the amount of red of the pixel and store that number in the 3 components.
- Next take the average amount of red, green and blue and store that value (store it in a variable called grey for example), in all three components.
- Better: initial the grey variable as follows:
grey = int( 0.3 * red +0.6 * green +0.11 * blue )
Problem 3
Copy paste this function that contains a call to repaint( image ) inside the for x in range(...) loop. See how the program "sweeps" through the image.
- Modify the function so that the sweep goes from left to right.
- Modify the function so that the sweep goes from top to bottom
- Modify the function so that the sweep goes from bottom to top
Problem 4
Pick one of the functions you have written for the problems above and make a new copy of it under a different name.
Replace the nested for-loop with this loop:
for x in range( getWidth( image ) ): for y in range( min( x, getHeight( image ) ):
Predict the way the image is going to be transformed by these nested for-loops.
Problem 5: Border
Write a new function called addBorder( image, borderWidth ) that will put a red border around the image.
Modify the program and ask the user to pick a color first, then pass this color to the function addBorder( image, borderWidth, color ) which will put a border all around the image.
Problem 6: B&W image with Colored Border
Make your program output a version of your colored image that will be black and white with a colored border around it.