CSC111 Adding a Red Disk to an Image
--D. Thiebaut (talk) 21:33, 13 March 2014 (EDT)
Contents
A Large Image for Processing
and an inspiration for Spring Break...
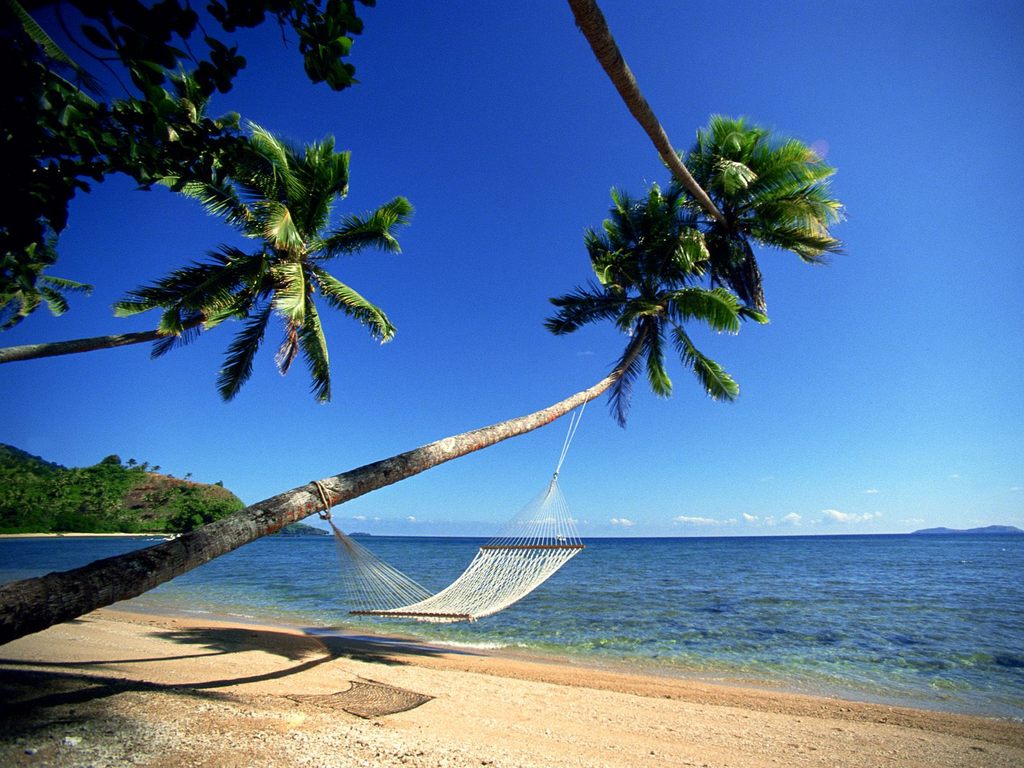
beach.jpg JPEG 1024x768 1024x768+0+0 8-bit sRGB 154KB 0.000u 0:00.000 786,432 pixels
Version 1: The first-pass program
# addRedDisk.py
# adds a disc in the middle of the image.
# The program measures the execution time of the function addRedDisk()
# and prints it on the screen. The time is in seconds.
from time import time
# ==================================================================
# distance()
# computes the distance squared between two points in
# a cartesian system.
def distance( x1, y1, x2, y2 ):
return sqrt( (x1-x2)*(x1-x2) + (y1-y2)*(y1-y2) )
# ==================================================================
# addRedDisk:
# adds a red disk to an image, located at center xCenter, yCenter,
# and with radius "radius"
def addRedDisk( image, xCenter, yCenter, radius ):
for x in range(0,getWidth(image)):
for y in range(0,getHeight(image)):
if distance( x, y, xCenter, yCenter ) < radius:
pixel = getPixel (image, x, y)
setColor( pixel, makeColor( 255, 0, 0 ) )
return image
# ==================================================================
# MAIN PROGRAM
# ==================================================================
file = pickAFile()
image = makePicture( file )
show( image )
t1 = time()
image = addRedDisk( image, getWidth(image)/2, getHeight(image)/2, 20 )
t2 = time()
repaint( image )
#writePictureTo( image, file )
printNow( t2 - t1 )
Output
======= Loading Progam ======= 2.6670000553131104 >>>
Version 2: Restricting the number of iterations
# addRedDisk.py
# adds a disc in the middle of the image.
# The program measures the execution time of the function addRedDisk()
# and prints it on the screen. The time is in seconds.
from time import time
# distance()
# computes the distance squared between two points in
# a cartesian system.
def distance( x1, y1, x2, y2 ):
return sqrt( (x1-x2)*(x1-x2) + (y1-y2)*(y1-y2) )
# addRedDisk:
# adds a red disk to an image, located at center xCenter, yCenter,
# and with radius "radius"
def addRedDisk( image, xCenter, yCenter, radius ):
for x in range( xCenter-radius, xCenter+radius+1 ):
for y in range( yCenter-radius, yCenter+radius+1 ):
if x > 0 and y > 0 \
and x < getWidth( image ) and y < getHeight( image ) \
and distance( x, y, xCenter, yCenter ) < radius:
pixel = getPixel (image, x, y)
setColor( pixel, makeColor( 255, 0, 0 ) )
return image
# ==================================================================
# MAIN PROGRAM
# ==================================================================
file = pickAFile()
image = makePicture( file )
show( image )
t1 = time()
image = addRedDisk( image, getWidth(image)/2, getHeight(image)/2, 20 )
t2 = time()
repaint( image )
#writePictureTo( image, file )
printNow( t2 - t1 )
Output
>>> ======= Loading Progam ======= 0.13000011444091797 >>>
Version 3: Squeezing some more Computation out of the Loop
# addRedDisk.py
# adds a disc in the middle of the image.
# The program measures the execution time of the function addRedDisk()
# and prints it on the screen. The time is in seconds.
from time import time
# distance()
# computes the distance squared between two points in
# a cartesian system.
def distance2( x1, y1, x2, y2 ):
return (x1-x2)*(x1-x2) + (y1-y2)*(y1-y2)
# addRedDisk:
# adds a red disk to an image, located at center xCenter, yCenter,
# and with radius "radius"
def addRedDisk( image, xCenter, yCenter, radius ):
radius2 = radius * radius
for x in range( xCenter-radius, xCenter+radius+1 ):
for y in range( yCenter-radius, yCenter+radius+1 ):
if x > 0 and y > 0 \
and x < getWidth( image ) and y < getHeight( image ) \
and distance2( x, y, xCenter, yCenter ) < radius2:
pixel = getPixel (image, x, y)
setColor( pixel, makeColor( 255, 0, 0 ) )
return image
# ==================================================================
# MAIN PROGRAM
# ==================================================================
file = pickAFile()
image = makePicture( file )
show( image )
t1 = time()
image = addRedDisk( image, getWidth(image)/2, getHeight(image)/2, 20 )
t2 = time()
repaint( image )
#writePictureTo( image, file )
printNow( t2 - t1 )
Output
>>> ======= Loading Progam ======= 0.0969998836517334 >>>