CSC111 Car With Top and Without Hard Top
--D. Thiebaut (talk) 21:05, 17 April 2014 (EDT)
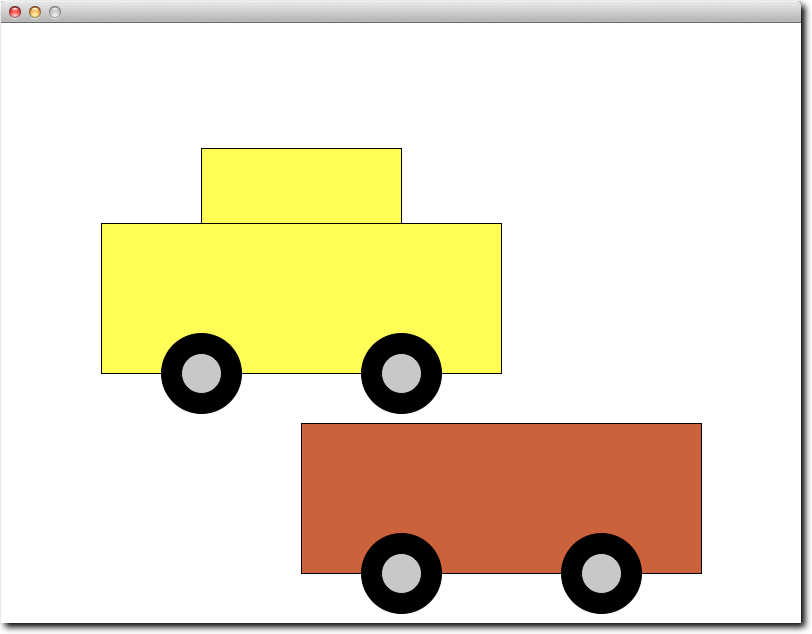
Example 1: Two Classes
# graphicsCar1.py
# D. Thiebaut
# implements two classes, one for a car with a top,
# one for a car without one.
from graphics import GraphicsWindow
from random import seed
from random import randrange
MAXWIDTH = 800
MAXHEIGHT = 600
# ----------------------------------------------------------------------------------------
class Circle2:
def __init__( self, x, y, diameter, color ):
self._x = x
self._y = y
self._diameter= diameter
self._color = color # list of 3 ints between 0 and 255
def draw( self, canvas ):
canvas.setFill( self._color[0], self._color[1], self._color[2] )
canvas.drawOval( self._x-self._diameter//2,
self._y-self._diameter//2,
self._diameter,
self._diameter )
# ----------------------------------------------------------------------------------------
class Wheel:
def __init__( self, x, y, diameter ):
self._c1 = Circle2( x, y, diameter, ( 0,0,0 ) )
self._c2 = Circle2( x, y, diameter/2, ( 200, 200, 200 ) )
def draw( self, canvas ):
self._c1.draw( canvas )
self._c2.draw( canvas )
# ----------------------------------------------------------------------------------------
class Rectangle:
def __init__( self, x, y, width, height, color ):
self._x = x
self._y = y
self._width = width
self._height = height
self._color = color # list of 3 ints between 0 and 255
def draw( self, canvas ):
canvas.setFill( self._color[0], self._color[1], self._color[2] )
canvas.drawRect( self._x, self._y, self._width, self._height )
def getWidth( self ):
return self._width
def getHeight( self ):
return self._height
#----------------------------------------------------------------------
# CarNoTop: A class implementing a car with only a body, 2 wheels, and
# no top.
class CarNoTop:
def __init__( self, x, y, width, height, color ):
# build the body
self._body = Rectangle( x, y, width, height, color )
# build the wheels
self._w1 = Wheel( x + width//4, y + height, width//5 )
self._w2 = Wheel( x + 3*width//4, y + height, width//5 )
def draw( self, canvas ):
self._body.draw( canvas )
self._w1.draw( canvas )
self._w2.draw( canvas )
#----------------------------------------------------------------------
# CarTop: A class implementing a car with only a body, 2 wheels, and
# a top above the body.
class CarTop:
def __init__( self, x, y, width, height, color ):
# build the body
self._body = Rectangle( x, y, width, height, color )
self._top = Rectangle( x+width//4, y-height//2,
width//2, height//2, color )
# build the wheels
self._w1 = Wheel( x + width//4, y + height, width//5 )
self._w2 = Wheel( x + 3*width//4, y + height, width//5 )
def draw( self, canvas ):
self._body.draw( canvas )
self._top.draw( canvas )
self._w1.draw( canvas )
self._w2.draw( canvas )
def main():
seed()
win = GraphicsWindow(MAXWIDTH, MAXHEIGHT)
canvas = win.canvas()
c1 = CarTop( 100,200, 400, 150, ( 255, 255, 0 ))
c1.draw( canvas )
c2 = CarNoTop( 300, 400, 400, 150, ( 200, 100, 50) )
c2.draw( canvas )
win.wait()
win.close()
main()
Example 2: One Class with A Boolean Switch
# graphicsCar2.py
# D. Thiebaut
# implements a car that can have a hard top or not
# depending on a boolean member variable.
from graphics import GraphicsWindow
from random import seed
from random import randrange
MAXWIDTH = 800
MAXHEIGHT = 600
class Circle2:
def __init__( self, x, y, diameter, color ):
self._x = x
self._y = y
self._diameter= diameter
self._color = color # list of 3 ints between 0 and 255
def draw( self, canvas ):
canvas.setFill( self._color[0], self._color[1], self._color[2] )
canvas.drawOval( self._x-self._diameter//2,
self._y-self._diameter//2,
self._diameter,
self._diameter )
class Wheel:
def __init__( self, x, y, diameter ):
self._c1 = Circle2( x, y, diameter, ( 0,0,0 ) )
self._c2 = Circle2( x, y, diameter/2, ( 200, 200, 200 ) )
def draw( self, canvas ):
self._c1.draw( canvas )
self._c2.draw( canvas )
class Rectangle:
def __init__( self, x, y, width, height, color ):
self._x = x
self._y = y
self._width = width
self._height = height
self._color = color # list of 3 ints between 0 and 255
def draw( self, canvas ):
canvas.setFill( self._color[0], self._color[1], self._color[2] )
canvas.drawRect( self._x, self._y, self._width, self._height )
#----------------------------------------------------------------------
# Car: A class implementing a car with only a body, 2 wheels, and
# a top or no top, depending on the boolean _hasTop.�
class Car:
def __init__( self, x, y, width, height, color, hasTop ):
self._hasTop = hasTop
# build the body
self._body = Rectangle( x, y, width, height, color )
if self._hasTop:
self._top = Rectangle( x+width//4, y-height//2,
width//2, height//2, color )
# build the wheels
self._w1 = Wheel( x + width//4, y + height, width//5 )
self._w2 = Wheel( x + 3*width//4, y + height, width//5 )
def draw( self, canvas ):
self._body.draw( canvas )
self._w1.draw( canvas )
self._w2.draw( canvas )
if self._hasTop:
self._top.draw( canvas )
def main():
seed()
win = GraphicsWindow(MAXWIDTH, MAXHEIGHT)
canvas = win.canvas()
c1 = Car( 100,200, 400, 150, ( 255, 255, 0 ), True )
c1.draw( canvas )
c2 = Car( 300, 400, 400, 150, ( 200, 100, 50), False )
c2.draw( canvas )
win.wait()
win.close()
main()
Example 3: Two Classes, One Inherited from the Other
# graphicsCar3.py
# D. Thiebaut
# implements two classes, one inherited from
# the other, the base class
from graphics import GraphicsWindow
from random import seed
from random import randrange
MAXWIDTH = 800
MAXHEIGHT = 600
class Circle2:
def __init__( self, x, y, diameter, color ):
self._x = x
self._y = y
self._diameter= diameter
self._color = color # list of 3 ints between 0 and 255
def draw( self, canvas ):
canvas.setFill( self._color[0], self._color[1], self._color[2] )
canvas.drawOval( self._x-self._diameter//2,
self._y-self._diameter//2,
self._diameter,
self._diameter )
class Wheel:
def __init__( self, x, y, diameter ):
self._c1 = Circle2( x, y, diameter, ( 0,0,0 ) )
self._c2 = Circle2( x, y, diameter/2, ( 200, 200, 200 ) )
def draw( self, canvas ):
self._c1.draw( canvas )
self._c2.draw( canvas )
class Rectangle:
def __init__( self, x, y, width, height, color ):
self._x = x
self._y = y
self._width = width
self._height = height
self._color = color # list of 3 ints between 0 and 255
def draw( self, canvas ):
canvas.setFill( self._color[0], self._color[1], self._color[2] )
canvas.drawRect( self._x, self._y, self._width, self._height )
#----------------------------------------------------------------------
# Car: A class implementing a car with only a body, 2 wheels, and
# no top.
class Car:
def __init__( self, x, y, width, height, color ):
# build the body
self._body = Rectangle( x, y, width, height, color )
# build the wheels
self._w1 = Wheel( x + width//4, y + height, width//5 )
self._w2 = Wheel( x + 3*width//4, y + height, width//5 )
def draw( self, canvas ):
self._body.draw( canvas )
self._w1.draw( canvas )
self._w2.draw( canvas )
def getWidth( self ):
return self._width
def getHeight( self ):
return self._height
class CarTop( Car ):
def __init__( self, x, y, width, height, color ):
super().__init__( x, y, width, height, color )
self._top = Rectangle( x+width//4, y-height//2,
width//2, height//2, color )
def draw( self, canvas ):
super().draw( canvas )
self._top.draw( canvas )
def main():
seed()
win = GraphicsWindow(MAXWIDTH, MAXHEIGHT)
canvas = win.canvas()
c1 = CarTop( 100,200, 400, 150, ( 255, 255, 0 ) )
c1.draw( canvas )
c2 = Car( 300, 400, 450, 150, ( 200, 100, 50) )
c2.draw( canvas )
print( "width of Car c1 = ", c1.getWidth() )
print( "width of Car c2 = ", c2.getWidth() )
win.wait()
win.close()
main()