CSC111 Homework 11 Solution Program 2014
--D. Thiebaut (talk) 12:56, 29 April 2014 (EDT)
Source
#----------------------------------------------------------------------
# hw11b.py
# Julieanna Niu (bi)
# 4/24/2014
#
# Program containing seven classes: Rectangle, Polygon, Curves, Windows,
# Wheels, Cars, and oldCar. Descriptions of each class can be found
# below, along with methods associated with each class.
#
# This program uses the graphics library graphics.py to generate an
# image that contains alternatingrows of cars, with a message in a
# text box in the center of the image. The scheme, colors, car style,
# and message have all been predefined.
#----------------------------------------------------------------------
from graphics import GraphicsWindow
from random import seed
from random import randrange
from random import choice
# width and height of canvas
MAXWIDTH = 800
MAXHEIGHT = 600
# list of colors that will be available as car colors.
colors = ['RoyalBlue4','RoyalBlue1','dodger blue','light sky blue',
'deep sky blue','gold','light goldenrod','goldenrod4',
'goldenrod2', 'khaki1','red','tomato','salmon2', 'firebrick4',
'OrangeRed2']
#----------------------------------------------------------------------
# Rectangle: a class containing 5 private member variables:
# - x coordinate of the top left corner of the rectangle
# - y coordinate of the top left corner of the rectangle
# - width of the rectangle
# - height of the rectangle
# - color of the rectangle (can either be a list of 3 ints
# between 0 and 255, or a string of a color name recognized
# by TKinter)
# Methods supported:
# - draw(canvas): specifies color and draws rectangle onto the
# canvas.
#----------------------------------------------------------------------
class Rectangle:
def __init__( self, x, y, width, height, color ):
self._x = x
self._y = y
self._width = width
self._height = height
self._color = color
def draw( self, canvas ):
if isinstance(self._color, str): # if color is string
canvas.setFill(self._color)
else: # if color is 3 ints
canvas.setFill( self._color[0], self._color[1], self._color[2] )
canvas.drawRect( self._x, self._y, self._width, self._height )
#----------------------------------------------------------------------
# Polygon: a class containing 15 private member variables:
# - Seven pairs of x and y coordinates specifying the placement
# of the vertices of a seven-sided polygon shape.
# - color of the polygon (string of a color name recognized
# by TKinter)
# Methods supported:
# - draw(canvas): specifies color and draws polygon onto the
# canvas.
#----------------------------------------------------------------------
class Polygon:
def __init__( self, x1, y1, x2, y2, x3, y3, x4, y4, x5, y5, x6, y6, \
x7, y7, color ):
self._x1 = x1
self._y1 = y1
self._x2 = x2
self._y2 = y2
self._x3 = x3
self._y3 = y3
self._x4 = x4
self._y4 = y4
self._x5 = x5
self._y5 = y5
self._x6 = x6
self._y6 = y6
self._x7 = x7
self._y7 = y7
self._color = color
def draw( self, canvas ):
canvas.setLineWidth(3) # increases width of outline
canvas.setFill( self._color )
canvas.drawPolygon( self._x1, self._y1, self._x2, self._y2,\
self._x3, self._y3, self._x4, self._y4,\
self._x5, self._y5, self._x6, self._y6, \
self._x7, self._y7)
#----------------------------------------------------------------------
# Curves: a class containing 6 private member variables:
# - x and y coordinates of the top-left corner of the square that
# encloses the curve
# - the diameter of the circle that contains the curve
# - the angle at which the curve begins. Angles are taken with
# reference to the axis between the fourth and first quadrants.
# - angle of curve
# - color of the enclosed curve (string of a color name recognized
# by TKinter)
# Methods supported:
# - draw(canvas): specifies color and draws the curve onto the
# canvas. The 'curve' appears as a part of a circle (i.e.
# like a pie)
#----------------------------------------------------------------------
class Curves:
def __init__( self, x, y, diam, start, extent, color ):
self._x = x
self._y = y
self._diam = diam
self._extent = extent
self._start = start
self._color = color
def draw( self, canvas ):
canvas.setLineWidth(3)
canvas.setFill (self._color)
canvas.drawArc(self._x, self._y, self._diam, self._start, \
self._extent)
#----------------------------------------------------------------------
# Windows: a class containing 9 private member variables:
# - Four pairs of x and y coordinates specifying the placement
# of the vertices of a four-sided polygon shape.
# - color of the polygon (3 ints between 0 and 255)
# Methods supported:
# - draw(canvas): specifies color and draws the polygon on the
# canvas.
#----------------------------------------------------------------------
class Windows:
def __init__( self, x1, y1, x2, y2, x3, y3, x4, y4, color ):
self._x1 = x1
self._y1 = y1
self._x2 = x2
self._y2 = y2
self._x3 = x3
self._y3 = y3
self._x4 = x4
self._y4 = y4
self._color = color
def draw( self, canvas ):
canvas.setFill(self._color[0], self._color[1], self._color[2] )
canvas.drawPolygon( self._x1, self._y1, self._x2, self._y2,\
self._x3, self._y3, self._x4, self._y4)
#----------------------------------------------------------------------
# Wheels: a class containing 3 private member variables:
# - x and y coordinates of the center of the circle
# - the diameter of the circle
# Methods supported:
# - draw(canvas): draws a 'wheel' on the canvas, which consists
# of one larger circle with the specified diameter, and a
# smaller circle centrally superimposed on the large circle.
# Color is also specified (black for the large circle, grey
# for the small circle).
#----------------------------------------------------------------------
class Wheel:
def __init__( self, x, y, diam ):
self._x = x
self._y = y
self._diam = diam
def draw( self, canvas ):
canvas.setFill( 0, 0, 0 ) # sets color to black
# changes the x and y coordinates in order to use drawOval method
# on the canvas as specified by the graphics library. In drawOval,
# the x and y coordinates are the top-left corner of the rectangle
# that encloses the oval/circle.
canvas.drawOval( self._x-(self._diam//2), self._y-(self._diam//2),\
self._diam, self._diam)
canvas.setFill(200, 200, 200 ) # sets color to grey
canvas.drawOval( self._x-(self._diam//4), self._y-(self._diam//4), \
self._diam//2, self._diam//2)
#----------------------------------------------------------------------
# Car: a class containing 5 private member variables:
# - x and y coordinates of the top left corner of the rectangle
# that encloses the car
# - the width of the rectangle
# - the height of the rectangle
# - color of the body of the car (string of a color name recognized
# by TKinter)
#
# The body of this car is made up of one polygon. There is a light made
# of a rectangle, and windows made of a polygon. There are two wheels.
#
# Methods supported:
# - draw(canvas): draws all components of the car onto the canvas
#----------------------------------------------------------------------
class Car:
def __init__( self, x, y, width, height, color ):
# All components of the car have been initialized into their
# respective classes, with variables written with reference
# to the x and y coordinates of the top-left corner of the
# rectangle that encloses the car, as well as the width and
# height of the car.
self._bodypoly = Polygon(x, y+height, x, y+height//2, x+width//8,\
y, x+width//2, y, x+3*(width//4), y+height//2,\
x+width, y+height//2, x+width, y+height, color)
self._lights = Rectangle(x+width-width//16, y+height//2,
width//16, height//8, [125,125,125]) # white lights
self._windows= Windows( x+width//12,y+height//2, \
x+width//8+width//16, y+height//8, \
x+width//2-width//16, y+height//8, \
x+3*(width//4)-width//8, y+height//2, \
[200,200,200]) # grey windows
self._w1 = Wheel( x + 2*width//9, y+height, (width//9)*2)
self._w2 = Wheel( x + 5*width//7, y+height, (width//9)*2)
def draw( self, canvas ):
self._bodypoly.draw(canvas)
self._lights.draw(canvas)
self._windows.draw(canvas)
self._w1.draw(canvas)
self._w2.draw(canvas)
#----------------------------------------------------------------------
# oldCar: a class containing 5 private member variables:
# - x and y coordinates of the top left corner of a rectangle
# that encloses the car
# - the width of the rectangle
# - the height of the rectangle
# - color of the body of the car (string of a color name recognized
# by TKinter)
#
# The body of this car is made up of one rectangle, one semicircle (as the
# top of the car) and two quarter-circles (as the front and back of the car).
# There are two wheels.
#
# Methods supported:
# - draw(canvas): draws all components of the car onto the canvas
#----------------------------------------------------------------------
class oldCar:
def __init__(self, x, y, width, height, color):
self._body = Rectangle(x+width//4, y+height//2, 50, 25, color)
self._top = Curves( x+width//4, y, width//2, 0, 180, color)
self._left = Curves( x, y+height//2, 50, 90, 90, color)
self._right = Curves (x+width//2,y+height//2, 50,0, 90, color)
self._w1 = Wheel( x + 2*width//9, y+height, (width//9)*2)
self._w2 = Wheel( x + 5*width//7, y+height, (width//9)*2)
def draw (self, canvas):
self._body.draw(canvas)
self._top.draw(canvas)
self._left.draw(canvas)
self._right.draw(canvas)
self._w1.draw(canvas)
self._w2.draw(canvas)
#----------------------------------------------------------------------
# main(): The main function. Generates an image that has rows of cars
# of the same style, of a random color. The center of the image has a
# message within a rectangle ('Unhappy Easter') that hides the cars
# underneath it.
#----------------------------------------------------------------------
def main():
win = GraphicsWindow(MAXWIDTH, MAXHEIGHT)
canvas = win.canvas()
# for loop that draws the cars onto the canvas row by row, of
# alternating type
# for aesthetic purposes, the value for height should be between 1/3
# to 1/2 the value of the width.
width = 100 # width of rectangle that encloses car
height = 50 # height of rectangle that encloses car
y = 0
count = 1 # when count is odd, use Car class, else use oldCar
for y in range( 0, MAXHEIGHT, height+height//2):
x = 0
if count%2 != 0:
for x in range ( 0, MAXWIDTH, width+width//4):
c = Car( x, y, width, height, choice(colors))
c.draw( canvas )
count = count + 1
else:
for x in range ( 0, MAXWIDTH, width+width//4):
c = oldCar( x, y, width, height, choice(colors))
c.draw( canvas )
count = count + 1
y = y + height + 20
# creates textbox
textbox = Rectangle(200,250,400,100,'lemon chiffon')
textbox.draw(canvas)
# text and adjustments
canvas.setTextAnchor("center") # centers text
canvas.setTextFont( 'helvetica', 50, 'bold' )
canvas.drawText( MAXWIDTH//2, MAXHEIGHT//2, "Unhappy Easter" )
# background color of image
canvas.setBackground( 'peach puff' )
win.wait()
win.close()
# main entry point for the program
main()
Output
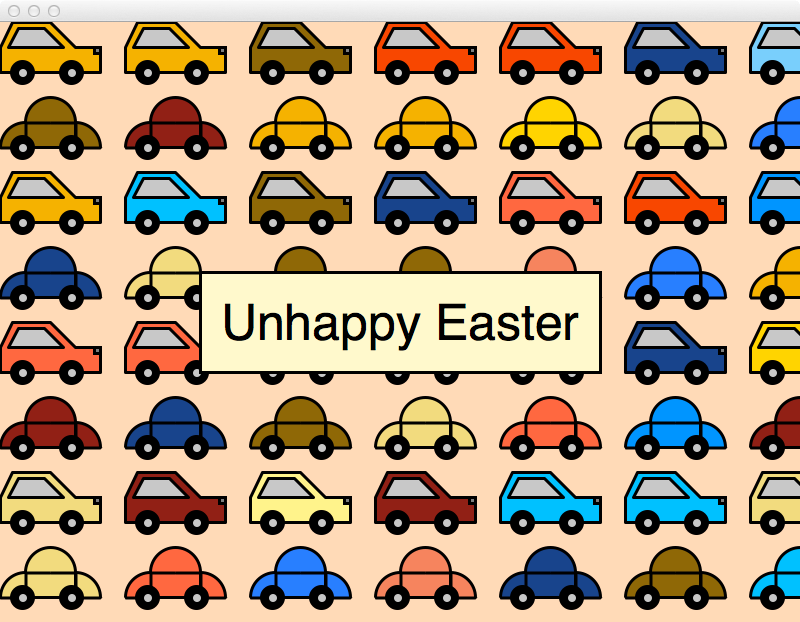
Program 2
This program uses inheritance to define the car classes.
# hw11b.py
# Thuy Nguyen (ce)
# This program prints out an example of a wrapping paper print
# where the theme is cars, the background color is light pink,
# and displays "Happy Easter" as the message. The uses two
# different types of cars where the program, generates rows of cars
# alternating between the two types of cars. The message is
# displayed in the middle of the wrapping paper inside a light
# blue rectangle.
#
# -------------------------------------------------------------------
from graphics import GraphicsWindow
from random import seed
from random import randrange
MAXWIDTH = 800
MAXHEIGHT = 600
class Circle:
# a class containing 4 private member variables:
# - center x position of circle (integer)
# - center y position of circle (integer)
# - diameter of circle (integer)
# - color of circle (list of 3 integers between 0 and 255)
# methods:
# draw( canvas ): the circle draws itself
# constructor: initializes a circle with all fields
def __init__( self, x, y, diameter, color ):
self._x = x
self._y = y
self._diameter= diameter
self._color = color
# draws a circle at defined (x,y) coordinates with specified color
def draw( self, canvas ):
canvas.setFill( self._color[0], self._color[1], self._color[2] )
canvas.drawOval( self._x-self._diameter//2,
self._y-self._diameter//2,
self._diameter,
self._diameter )
class Wheel:
# a class containing 3 private member variables:
# - center x position of wheel (integer)
# - center y position of wheel (integer)
# - diameter of wheel (integer)
# methods:
# draw( canvas ): the wheel draws itself
# constructor: initializes two wheels using Circle class
def __init__( self, x, y, diameter ):
self._c1 = Circle( x, y, diameter, ( 0,0,0 ) )
self._c2 = Circle( x, y, diameter/2, ( 200, 200, 200 ) )
# draws two wheels
def draw( self, canvas ):
self._c1.draw( canvas )
self._c2.draw( canvas )
class Car:
# a class containing 5 private member variables:
# - x position of car (integer)
# - y position of car (integer)
# - width of car (integer)
# - height of car (integer)
# - color of car (list of 3 integers between 0 and 255)
# methods:
# draw( canvas ): the car draws itself
# constructor: initializes a car with all fields
def __init__( self, x, y, width, height, color ):
self._x = x
self._y = y
self._width = width
self._height = height
self._color = color
# build the body
self._body = Rectangle( x, y, width, height, color )
# build the wheels
self._w1 = Wheel( x + width//4, y + height, width//5 )
self._w2 = Wheel( x + 3*width//4, y + height, width//5 )
# draws a car with its body and wheels
def draw( self, canvas ):
self._body.draw( canvas )
self._w1.draw( canvas )
self._w2.draw( canvas )
class CarTop( Car ):
# a class inherited from Car class with one additional private member variable
# - the top hood of a car
# methods:
# draw( canvas ): the car with top draws itself
def __init__( self, x, y, width, height, color, topColor ):
super().__init__( x, y, width, height, color )
self._top = Rectangle( x+width//4, y-height//2, width//2, height//2, topColor )
# draws a car with a top hood
def draw( self, canvas ):
super().draw( canvas )
self._top.draw( canvas )
class CarTop2( Car ):
# a class inherited from Car class with two additional private member variables
# - the top hood of a car
# - the window of a car
# methods:
# draw( canvas ): the car with top and window draws itself
def __init__( self, x, y, width, height, color, topColor ):
super().__init__( x, y, width, height, color )
self._top = Rectangle( x+width//2, y-height//2, width//2, height//2, topColor )
self._window = Rectangle( x+width//3, y+height//4, width//3, height//3, ( 0,0,0 ) )
# draws a car with a top hood and window
def draw( self, canvas ):
super().draw( canvas )
self._top.draw( canvas )
self._window.draw( canvas )
class Rectangle:
# a class containing 5 private member variables
# - x position of rectangle (integer)
# - y position of rectanle (integer)
# - width of rectangle (integer)
# - height of rectangle (integer)
# - color of rectangle (list of 3 integers between 0 and 255)
# methods:
# draw( canvas ): the rectangle draws itself
# constructor: initializes rectangle with all fields
def __init__( self, x, y, width, height, color ):
self._x = x
self._y = y
self._width = width
self._height = height
self._color = color
# draws a rectangle
def draw( self, canvas ):
canvas.setFill( self._color[0], self._color[1], self._color[2] )
canvas.drawRectangle( self._x, self._y, self._width, self._height )
# --------------------------------------------------------------------------------
# setRandomColor(): sets a random color to a car's body and hood
# --------------------------------------------------------------------------------
def setRandomColor():
r = randrange( 255 )
g = randrange( 255 )
b = randrange( 255 )
return r, g, b
# --------------------------------------------------------------------------------
# multipleCars( canvas ): displays the cars such that they are organized into
# rows, one above the other, and alternating each type of car in separate rows
# --------------------------------------------------------------------------------
def multipleCars( canvas ):
width = 100
height = 25
for x in range( 0, MAXWIDTH, 11*width//10 ):
for y in range( 0, MAXHEIGHT, 4*height ):
color = setRandomColor()
topColor = setRandomColor()
c = CarTop( x, y, width, height, color, topColor )
c.draw( canvas )
for y in range( 2*height, MAXHEIGHT, 4*height ):
color = setRandomColor()
topColor = setRandomColor()
c2 = CarTop2( x, y, width, height, color, topColor )
c2.draw( canvas )
# ================================================
# Main Program
# ================================================
def main():
seed()
win = GraphicsWindow(MAXWIDTH, MAXHEIGHT)
canvas = win.canvas()
multipleCars( canvas )
canvas.setBackground( 'thistle1' )
canvas.setFill( 'azure' )
canvas.drawRectangle( MAXWIDTH // 3, 2*MAXHEIGHT // 5, 325, 60 )
canvas.setTextFont( "times", 40, "bold" )
canvas.drawText( MAXWIDTH // 3, 2*MAXHEIGHT // 5, "Happy Easter" )
win.wait()
win.close()
main()