CSC111 Lab 1 2015
--D. Thiebaut (talk) 16:21, 19 January 2015 (EST)
--D. Thiebaut (talk) 10:39, 28 January 2014 (EST)
Contents
- 1 FUN WITH PYTHON
- 2 Option 1: Installing and Running Python on your Laptop
- 3 Option 2: Using Python on a Lab Computer
- 3.1 Idle
- 3.2 Some Python Code to play with
- 3.2.1 Playing with numbers
- 3.2.2 Variation 1
- 3.2.3 Variation 2
- 3.2.4 Playing with Numbers and Strings
- 3.2.5 Challenge #1
- 3.2.6 Repeating Strings
- 3.2.7 Challenge #3
- 3.2.8 Challenge #4 (tricky)
- 3.2.9 Playing with Lists and Loops
- 3.2.10 Challenge #5
- 3.2.11 Repeating several statements
- 3.2.12 Challenge #6
- 3.2.13 Challenge #7
- 3.2.14 Challenge of the Day
FUN WITH PYTHON
This lab is just an introduction to having fun with Python. It's purpose is to have you explore the different systems that are available to you, and get a sense of how to program in an "intuitive" fashion.
Option 1: Installing and Running Python on your Laptop
- If you want to work on your own laptop, you need to install Python 3. Open this page and follow the instructions for downloading Python 3 to your laptop.
Option 2: Using Python on a Lab Computer
- If your computer is not on, turn it ON, please!
- When prompted for booting Mac or Windows, select the Mac if you have the choice.
- Login using the Smith credentials (or the account that was given to you as a 5-college or high school student)
- Once you see the Desktop, click on the Finder (bottom left icon on the Desktop), and look in the Science Apps folder for Python 3.2.
- Start IDLE
Tip: if you can't find Idle, use the magnifying glass icon at the top right of the screen and search for idle and you should find it!
Idle
- Idle is the name of an editor one can use to create Python program on the computer.
- If the window you start with looks like this (see below), then use the top menu and click File, New Window to open an editing window.
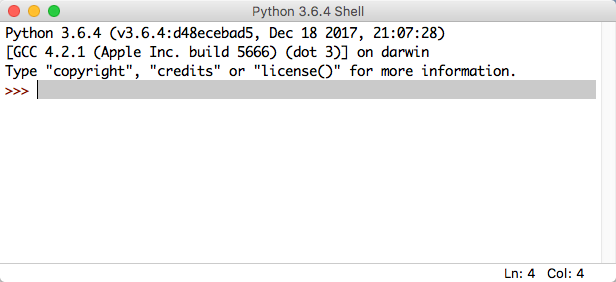
- You should now have two windows on your screen, one the editing window, the other the shell window, where the program will send its output.
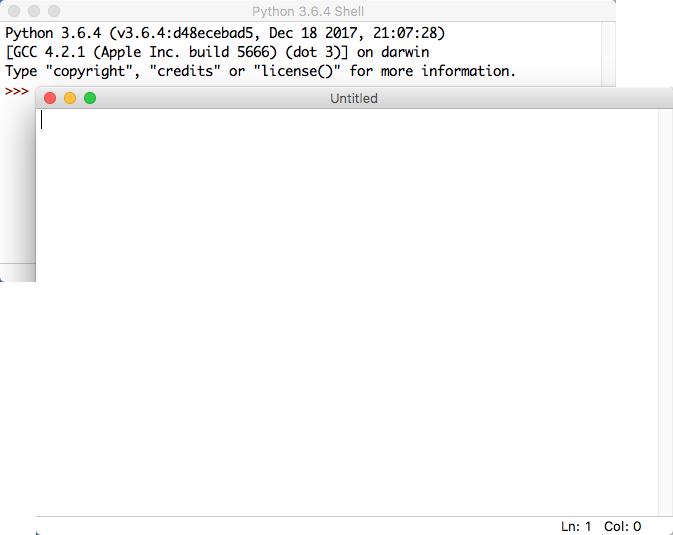
Some Python Code to play with
Playing with numbers
- Enter the following code in the Untitled window:
age = 20 year = 2015 print( age ) print( year ) print( year - age )
- Press F5 to run the program. When prompted to save the program, save it as lab1.py
- Look at the Console window. Some numbers appear... Do they make sense?
- change the numbers that are assigned to age, and year and see how your program reacts when you run it.
age, and year are variables. You can give variables any name you want, as long as you use letters or digits, and as long as the first character is a letter. R2d2 is a valid name for a variable. And so is alpha, or c3po. Variables are used to hold information, in our case, numbers.
Variation 1
- Modify your program slightly, as shown below:
age = 20 year = 2015 print( age, year )
- Run your program
- Notice the slightly different output.
Variation 2
- Try this:
age = 20 year = 2015 yearBorn = year - age print( "you are", age ) print( "you were born in", yearBorn )
- Run your program. Change the year and the age. Run your program again and see if you can predict the output.
Playing with Numbers and Strings
- This time we add new variables that contain text. In computer science we refer to text as strings of characters, or strings for short.
name = "Alex" college = "Smith College" age = 20 year = 2015 print( name, "goes to", college ) print( name, "is", age, "years old" )
- Run your program a few times, each time changing some of the variables.
Challenge #1 |
- Write a new program that contains these variables:
name = "Alex" college = "Smith College" age = 20 year = 2015
- Figure out how to add new variables and write several print statements that will make your program output:
Alex goes to Smith College and is 20 years old Alex was born in 1995
- If your program is well written, and if you change the variable name to be "Francis", college to be "Umass", and age to be 18, then your program should automatically output:
Francis goes to Umass and is 18 years old Francis was born in 1997
- Verify that your program works correctly
Repeating Strings
- Write a new program:
word1 = "hello" word2 = "there" print( word1 * 2 ) print( word1 * 5 ) print( word2 * 10 )
- See how you can multiply a string?
- Try this:
print( word1 * 2, word2 * 4 )
- Run the program. Does the output make sense?
Challenge #3 |
- Modify your program and make it print this:
hello hello hello hello there! hello there! there! there! there! there! hello
Challenge #4 (tricky) |
- See if you can make your program print this, using string multiplication:
hellothere!hellothere!hellothere!hellothere!hellothere!
Playing with Lists and Loops
- Create a new program in Idle with this new piece of code:
list = [ "Lea Jones", "Julie Fleur", "Anu Vias" ]
for name in list:
print( name )
- Press F5. Observe the output.
Challenge #5 |
- Add three new names to the list and make your program output them all one above the other.
Repeating several statements
- Play with these different code segments, each one on its own. Make sure you understand how each one works when you execute the program.
list = [ "Lea Jones", "Julie Fleur", "Anu Vias" ]
for name in list:
print( name )
print( "-" )
- and this one:
list = [ "Lea Jones", "Julie Fleur", "Anu Vias" ]
for name in list:
print( name, len( name ) )
- or:
list = [ "Lea Jones", "Julie Fleur", "Anu Vias" ]
for name in list:
print( name )
print ( len( name ) )
- Still some more
list = [ "Lea Jones", "Julie Fleur", "Anu Vias" ]
for name in list:
print( name, '-' * len( name ) )
Challenge #6 |
- Make your program display the names with a bar made of minus signs under each name. The length of the bar should be the same as the length of the name. For example:
Lea Jones --------- Julie Fleur ----------- Anu Vias --------
Challenge #7 |
- Similar question, but now like this:
--------- Lea Jones --------- ----------- Julie Fleur ----------- -------- Anu Vias --------
Challenge of the Day |
- Make your program display a box around each name:
------------- | Lea Jones | ------------- --------------- | Julie Fleur | --------------- ------------ | Anu Vias | ------------
- And... if you are done, and are really rolling and want some more of a challenge, try to make your program output this:
+-----------+ | Lea Jones | +-----------+ +-------------+ | Julie Fleur | +-------------+ +----------+ | Anu Vias | +----------+