CSC111 Lab 1 2015b
--D. Thiebaut (talk) 15:31, 5 September 2015 (EDT)
Contents
- 1 Lab 1: HAVING FUN WITH PYTHON
- 2 Pair Programming
- 3 Option 1: Installing and Running Python on your Laptop
- 4 Option 2: Using Python on a Lab Computer
- 5 Create a Folder to Keep All Your Programs
- 6 A Quick Video Overview
- 7 Editing Programs with the Idle Editor
- 7.1 Some Python Code to play with
- 7.1.1 Playing with numbers
- 7.1.2 Variation 1
- 7.1.3 Variation 2
- 7.1.4 Playing with Numbers and Strings
- 7.1.5 Challenge #1
- 7.1.6 Repeating Strings
- 7.1.7 Challenge #2
- 7.1.8 Challenge #3 (tricky)
- 7.1.9 Playing with Lists and Loops
- 7.1.10 Challenge #4
- 7.1.11 Repeating several statements
- 7.1.12 Challenge #5
- 7.1.13 Challenge #6
- 7.1.14 Challenge of the Day
- 7.1 Some Python Code to play with
- 8 Submission of Lab 1 Program on Moodle
- 9 Optional Challenge
- 10 Solution Programs
Lab 1: HAVING FUN WITH PYTHON
This lab is just an introduction to having fun with Python. It's purpose is to have you explore the different systems that are available to you, and get a sense of how to program in an "intuitive" fashion. Do not worry about the details. You will likely not finish the work presented in this lab during the 2-hour session reserved for it. This will give you a chance to work on your own during the week and practice coding in Python.
We haven't had time to fully understand what variables are, what strings are, or how the print command works. Today is just a day for you to explore the tools, run some Python code in Idle, and submit a program on Moodle. If you submit a file on Moodle, you'll get an automatic A for this lab, regardless of whether the program is correct or not (of course try to submit a program that works well!), but the emphasis this week is on making sure you know how to use all the tools for this course.
Pair Programming
This week you will work in pair programming mode with a partner. You will change partner next week. This will allow you to get to know people in the class who are in your lab session, and also, you will experience a very creative, and constructive way of coding, and of learning Python.
When working with a partner, follow these simple rules:
- SHARE
- "In pair programming, two programmers are assigned to jointly produce one artifact (design, algorithm, code, among others). ... One person is typing or writing [driving], the other is continually reviewing the work [navigating]. ... Both partners own everything."
Not only is this an effective learning technique, it is also more productive than splitting a task in two, working on each half separately, and then combining the two.
- PLAY FAIR
- It is important to take turns typing or writing [driving], so that the other person gets a chance to review [navigate].
- CLEAN UP
- Defects belong to the pair. Having two sets of eye balls is much better than one.
- HOLD HANDS AND STAY TOGETHER
- All programming should be done together; do not create things done alone. The literature of pair programming shows that most defects are traceable to things done singly. It also inhibits learning.
- SAY YOU'RE SORRY
- "Ego-less programming ... is essential for effective pair programming." Do not insist on having things your way or else. Do not get defensive about criticism. Work things out as a pair.
Option 1: Installing and Running Python on your Laptop
- If you want to work on your own laptop, you need to install Python 3. Open this page and follow the instructions for downloading Python 3 to your laptop.
Option 2: Using Python on a Lab Computer
- If the iMac is not ON, turn it ON, please!
- When prompted for booting Mac or Windows, select the Mac if you have the choice.
- Login using the Smith credentials (or the account that was given to you as a 5-college or high school student)
- Once you see the Desktop, click on the Finder (bottom left icon on the Desktop), and look in the Science Apps folder for Python 3.X (X may be 2, 3, 4, or 5, depending on the current version installed).
- Start IDLE
Tip: if you can't find Idle, use the magnifying glass icon at the top right of the screen and search for idle and you should find it!
Create a Folder to Keep All Your Programs
You will create many programs this semester. It is best to keep them well organized in a system of folders in your computer (or in your Home folder on your Smith drive).
If you're using your own laptop
- Create a folder on your Desktop. Call it CSC111
- Open the folder you just created. In it, create a new folder called Lab1
- You will store the programs you create today in the folder Lab1 you just created.
If you're using a Lab computer
- Follow the same steps as above, but start in your home folder, instead of the desktop.
A Quick Video Overview
Here's a quick overview of how to use Idle to program in Python. You will notice that there are two distinct ways of typing in Python statements. The left window, in the video, is the console, where each line you type is interpreted by Python. The window on the right, however, allows you to write a collection of statements (a program), store the collection in a file, and then have Python execute the contents of the program. (You may want to turn the sound off. There is only background music in the video.)
Editing Programs with the Idle Editor
- Idle is the name of an editor one can use to create Python program on the computer.
- If the window you start with looks like this (see below), then use the top menu and click File, New Window to open an editing window.
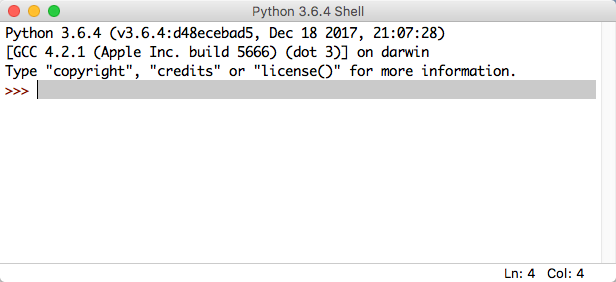
- You should now have two windows on your screen, one the editing window, the other the shell window, where the program will send its output.
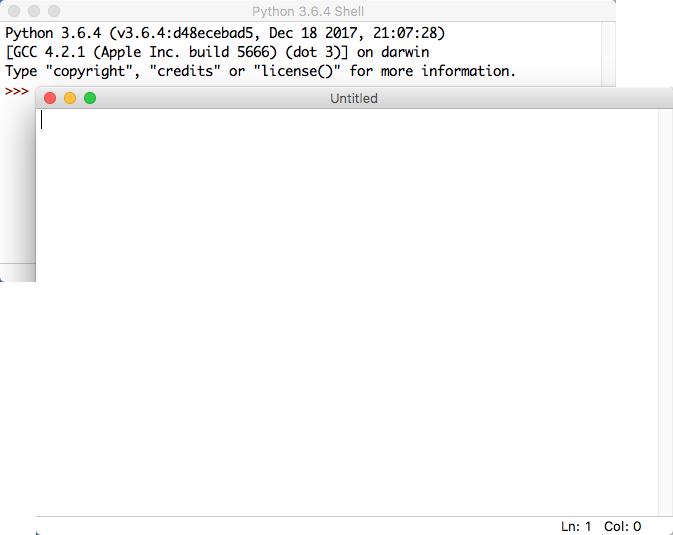
Some Python Code to play with
Playing with numbers
- Enter the following code in the Untitled window:
age = 20
year = 2015
print( age )
print( year )
print( year - age )
- Press F5 to run the program. When prompted to save the program, save it as lab1.py in the Lab1 folder you created previously.
- Look at the Console window. Some numbers appear... Do they make sense?
- change the numbers that are assigned to age, and year and see how your program reacts when you run it.
age, and year are variables. You can give variables any name you want, as long as you use letters or digits, and as long as the first character is a letter. R2d2 is a valid name for a variable. And so is alpha, or c3po. Variables are used to hold information, in our case, numbers.
Variation 1
- Modify your program slightly, as shown below:
age = 20
year = 2015
print( age, year )
- Run your program
- Notice the slightly different output.
Variation 2
- Try this:
age = 20
year = 2015
yearBorn = year - age
print( "you are", age )
print( "you were born in", yearBorn )
- Run your program. Change the year and the age. Run your program again and see if you can predict the output.
Playing with Numbers and Strings
- This time we add new variables that contain text. In computer science we refer to text as strings of characters, or strings for short.
name = "Alex"
college = "Smith College"
age = 20
year = 2015
print( name, "goes to", college )
print( name, "is", age, "years old" )
- Run your program a few times, each time changing the value of a variable. For example change "Alex" to "Monique", or year to 2015.
Challenge #1 |
- Write a new program that contains these variables:
name = "Alex"
college = "Smith College"
age = 20
year = 2015
- Figure out how to add new variables and write several print statements that will make your program output:
Alex goes to Smith College and is 20 years old Alex was born in 1995
- If your program is well written, and if you change the variable name to be "Francis", college to be "Umass", and age to be 18, then your program should automatically output:
Francis goes to Umass and is 18 years old Francis was born in 1997
- Verify that your program works correctly
Repeating Strings
- Write a new program:
word1 = "hello"
word2 = "there "
print( word1 * 2 )
print( word1 * 5 )
print( word2 * 10 )
- See how you can multiply a string?
- Try this:
print( word1 * 2, word2 * 4 )
- Run the program. Does the output make sense?
Challenge #2 |
- Modify your program (you are allowed to change the contents of the variables), and make it print the output shown below. Note the new exclamation point:
hello hello hello hello there! hello there! there! there! there! there! hello
Challenge #3 (tricky) |
- Make your program print the output below, using a string and the string multiplication operation:
hellothere!hellothere!hellothere!hellothere!hellothere!
Playing with Lists and Loops
- Create a new program in Idle with this new piece of code:
for name in [ "Lea Jones", "Julie Fleur", "Anu Vias" ]:
print( name )
- Press F5. Observe the output.
Challenge #4 |
- Add three new names to the list and make your program output them all one above the other.
Repeating several statements
- Play with these different code snippets. Make sure you understand how your program works before moving on to the next sections.
The purpose is not to go quickly through the lab, but to start acquiring an intuition for the logic of programming.
for name in [ "Lea Jones", "Julie Fleur", "Anu Vias" ]:
print( name )
print( "---------------" )
- and this one:
for name in [ "Lea Jones", "Julie Fleur", "Anu Vias" ]:
print( name, len( name ) )
- or:
for name in [ "Lea Jones", "Julie Fleur", "Anu Vias" ]:
print( name )
print ( len( name ) )
- Still some more
for name in [ "Lea Jones", "Julie Fleur", "Anu Vias" ]:
print( name, '-' * len( name ) )
Challenge #5 |
- Make your program display the names with a bar made of minus signs under each name. The length of the bar should be the same as the length of the name that is above it. For example:
Lea Jones --------- Julie Fleur ----------- Anu Vias --------
Challenge #6 |
- Similar question, but now like this:
--------- Lea Jones --------- ----------- Julie Fleur ----------- -------- Anu Vias --------
Challenge of the Day |
Step 1
- Create a program called lab1COD.py with all the statements below. They contain the solution for this challenge. You will need to figure out how to rearrange them and modify them to create the answer for this challenge. So create this program and study carefully its code and its output.
name = "Julia Child"
nameLen = len( name )
nameLen4 = len( name ) + 4
nameLen2 = len( name ) + 2
x = 10
bar1 = "-" * x
bar2 = bar1 + "|"
bar3 = "<" + bar1 + ">"
bar4 = "-" * nameLen
print( "name = ", name )
print( "nameLen = ", nameLen )
print( "nameLen4 = ", nameLen4 )
print( "nameLen2 = ", nameLen2 )
print( "x = ", x )
print( "bar1 = ", bar1 )
print( "bar2 = ", bar2 )
print( "bar3 = ", bar3 )
print( "bar4 = ", bar4 )
- Change "Julia Child" for some other, longer, name. Run your program again and see how the different variables will change according to the length of the string you store in name.
Step 2
- Modify your program as follows:
for name in [ "Julia Child", "Gloria Steinem" ]:
nameLen = len( name )
nameLen4 = len( name ) + 4
nameLen2 = len( name ) + 2
x = 10
bar1 = "-" * x
bar2 = bar1 + "|"
bar3 = "<" + bar1 + ">"
bar4 = "-" * nameLen
print( "nameLen = ", nameLen )
print( "nameLen4 = ", nameLen4 )
print( "nameLen2 = ", nameLen2 )
print( "x = ", x )
print( "name = ", name )
print( "bar1 = ", bar1 )
print( "bar2 = ", bar2 )
print( "bar3 = ", bar3 )
print( "bar4 = ", bar4 )
print()
- Carefully look at the output of your program. Because all the print statements are indented relative to the for statement, they are repeated for every name in the list. See how bar1 and bar2 do not change in length, but bar4 changes with each string.
- Here is your challenge for today: Make your program use a for-statement and a list of 3 names, and make it display a box around each name. The challenge here is that the box must fit exactly the length of the name, without extra spaces around.
------------- | Lea Jones | ------------- --------------- | Julie Fleur | --------------- ------------ | Anu Vias | ------------
Submission of Lab 1 Program on Moodle
- For this lab you need to submit your solution program on Moodle. This submission is graded and the grade counts toward your lab grade for this class (15% of the total grade).
- Go to this page and follow the directions for submitting your program. Remember that your program must be called lab1COD.py for you to submit it. Both partners in the Pair Programming team should submit under the program under their own name.
If you see a pop-up window appear when you try to evaluate you program, mentioning "encrypted connection," then follow the steps listed in this page to get rid of this annoyance.
- Deadline: Exceptionally, this week, the deadline is set to 9/19/15, to allow Add/Drop students to catch up.
- Grade:
- Program submitted (even if it doesn't work correctly or has errors): 100, or A
- Program not submitted: 0, or F
Optional Challenge |
- This is not required today, but if you want some extra challenging coding to do, try making your program generate the output below.
+-----------+ | Lea Jones | +-----------+ +-------------+ | Julie Fleur | +-------------+ +----------+ | Anu Vias | +----------+
- You do not have to submit this program.
<showafterdate after="20150920 00:00" before="20151231 00:00">
Solution Programs
# CSC111 Lab1
# D. Thiebaut
# solution programs for Lab1
# Challenge #1
name = "Alex"
college = "Smith College"
age = 20
year = 2015
print( name, "goes to", college, "and is", age, "years old" )
print( name, "was born in", year - age )
print()
# Challenge #2
word1 = "hello "
word2 = "there! "
print( word1 * 4, word2 )
print( word1, word2*5, word1 )
print()
# Challenge #3
word1 = "hello"
word2 = "there!"
word3 = word1 + word2
print( word3*5 )
print( ("hello" + "there!") * 5 )
print()
# Challenge #4
for name in [ "Lea Jones", "Julie Fleur", "Anu Vias",
"Mickey", "Minnie", "Pluto" ]:
print( name )
print()
# Challenge #5
for name in [ "Lea Jones", "Julie Fleur", "Anu Vias",
"Mickey", "Minnie", "Pluto" ]:
print( name )
print( "-" * len( name ) )
print()
# Challenge #6
for name in [ "Lea Jones", "Julie Fleur", "Anu Vias",
"Mickey", "Minnie", "Pluto" ]:
print( "-" * len( name ) )
print( name )
print( "-" * len( name ) )
print()
# Challenge #7
for name in [ "Lea Jones", "Julie Fleur", "Anu Vias",
"Mickey", "Minnie", "Pluto" ]:
name2 = "| " + name + " |"
bar = "-" * len( name2 )
print( bar )
print( name2 )
print( bar )
print()
print()
# Optional Challenge
for name in [ "Lea Jones", "Julie Fleur", "Anu Vias",
"Mickey", "Minnie", "Pluto" ]:
name2 = "| " + name + " |"
bar = "-" * len( name )
bar = "+-" + bar + "-+"
print( bar )
print( name2 )
print( bar )
print()
print()
</showafterdate>