CSC111 Final Exam 2015b
--D. Thiebaut (talk) 21:22, 6 December 2015 (EST)
This exam is a take-home exam given under the rules of the Smith College Honor-Code. Please make sure you read it and understand it.
The exam is open book, open notes, and open Web.
You have to do the work individually. No pair-programming allowed! You cannot seek help from anybody for this exam. The TAs will not hold hours, and will not be allowed to answer questions relating to the final exam, Python, or to the class material. All questions relating to this Final should be directed to your instructor, and posted on Piazza. Posts on piazza should not contain any code. Only questions meant to clarify the exam are allowed. Questions on piazza should only be answered by the instructor. No debugging-related questions will be answered.
The deadline for the exam is Tuesday, Dec. 22, at 4:00 p.m.' While you are given 8 days on this exam, the programming should take you no more than a day, two at most, so organize your time well. No extensions will be granted beyond the due date and time.
All three problems are worth the same number of points.
<showafterdate after="20151213 00:00" before="20160931 00:00">
Contents
Problem 1: Fractal Orange Tree
This is based on Lab #13, which you may want to review before working on this problem.
Write a well documented and organized Python program that will generate a tree identical to the tree shown below. Your program must generate a tree as close as possible to the one shown. You should not reproduce the text' in the image, which is there to mark this image as part of the exam; Your program needs to generate just the tree, its colors, its leaves, and its fruit.
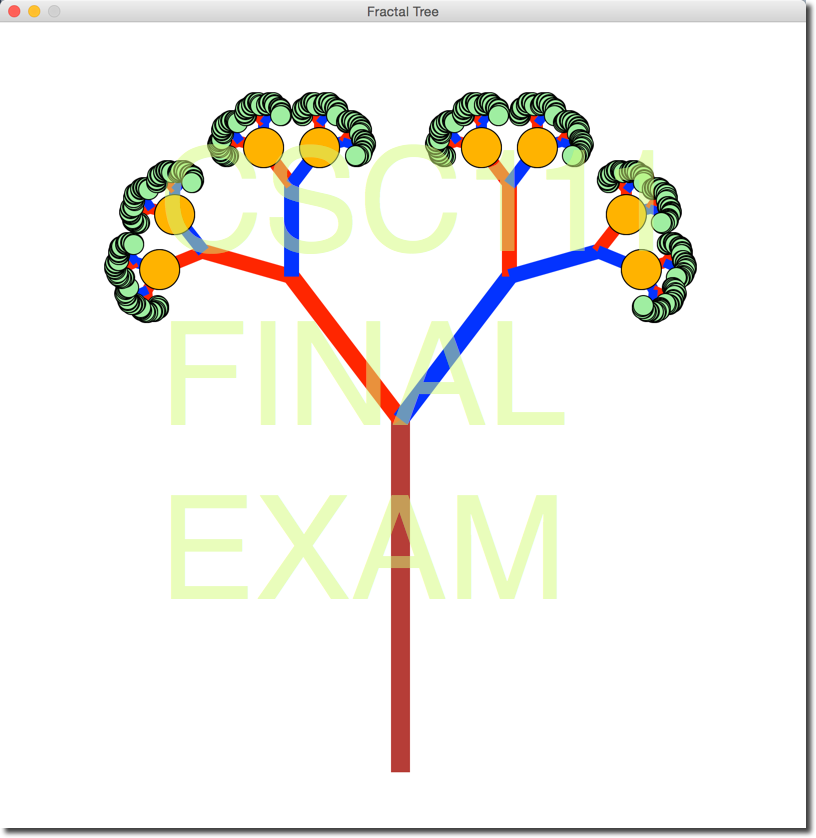
- Make sure your name is included in the header of your program.
- Call your program final1.py.
- I only used "red", "orange", "blue", "lightgreen," and "brown" in the program that generated the image above.
- Note that the width of the branches are different. If I ask the program to print the width of each branch as it draws them, I get this output (which is truncated, as it gets very long):
width = 19 width = 17 width = 15 width = 13 width = 11 width = 9 width = 7 width = 5 width = 3 width = 1 ...
- Here is the main() function of the solution program:
def main(): win = GraphWin("Fractal Tree", MAXWIDTH, MAXHEIGHT ) theta = 0.65 # use 0.02 for tall skinny trees, 0.7 for fat trees draw_tree(win, 9, theta, MAXWIDTH*0.9, MAXWIDTH//2, MAXHEIGHT-50, -math.pi/2, "brown" ) win.getMouse() win.close()
Misc. Important Notes
- You are allowed to add parameters to the recursive function.
- The variable trunk_ration is set to 0.49 in the recursive function.
- You have to figure out how to modify the recursive function to make it display the leaves and the oranges. Your program should not generate the leaves or oranges outside the recursive function.
- The leaves have radius 10. The oranges have radius 20.
Submission
- Submit your program, along with a capture of the image to Moodle, in the Final 1 Program and Final 1 Image sections. If you need help capturing the final image, please visit this page.
- Make sure your program is documented well, and that your name is written at the top of the header.
Problem 2: Find the Largest Element of a List of Lists
You are given the following program to get you started on this problem.
# final2.py # Display the contents of a list of lists. # It uses the standard Python function type() which can be used to # test what the type of a variable is. def display( List ): for item in List: # is item an integer? if type( item )==type( 3 ): # yes, then print it print( item, " ", end="", sep="" ) else: # no, it's a list, call display # recursively on this list. display( item ) def main(): # get a list from the user, and evaluate it as a Python list L = eval( input( "Enter a list: " ) ) # display the raw list print( "L = ", L ) # display all the elements, one by one. display( L ) print() main()
Example of user interaction with the program:
>>> Enter a list: [ 1, 2, [3, 4], 5, [6, 7], [8,[9,10] ], (11, 12) ] L = [1, 2, [3, 4], 5, [6, 7], [8, [9, 10]], (11, 12)] 1 2 3 4 5 6 7 8 9 10 11 12 >>>
Your Assignment
Using the program above as inspiration, write a program called final2.py that contains a recursive function that will be used to find the largest integer in a list of lists. Your program will get the main list from the user, then it will compute and print out only the largest element of the list. The list entered by the user may contain sub-lists, as illustrated above.
Additional Information
- Your program will be tested only with lists that contain only integers and possibly other lists. A list or its sublists will always include at least 1 item, which will be an integer, or a sublist. In other words, lists and sublists will never be empty.
Restrictions
Your program should
- input a list from the user,
- output a line with the string -*- in it,
- output the largest integer found in the list.
Example:
>>> Enter a list: [ 1, 2, [3, 4], 5, [6, 7], [8,[9,100] ], (11, 12) ] -*- 100 >>>
Submission
- Submit your program to the Final PB 2 section on Moodle. The automatic evaluation of the program is turned off for this exam. Make sure it works well with all kinds of lists!
Problem 3: Frogger Game
(if the video does not show in the page, you can watch it on YouTube: https://www.youtube.com/watch?v=plgm_p2zAek)
Your assignment is to write a program called final3.py that uses graphics, and that implements the game illustrated in the video, with a few additional features.
The program you have to write requires graphics.py, which you can download from [ http://mcsp.wartburg.edu/zelle/python/graphics.py http://mcsp.wartburg.edu/zelle/python/graphics.py]
Note that the cars appearing on the left of the screen in the video, blink. This is a side-effect of the graphics library, and not a feature of the program. You do not have to emulate this strange behavior.
Frog
You can use the frog image shown on the right for your game, or use one you will have found on the Web. Or you can draw it using ovals and/or circles. The URL for the image on the right is: cs.smith.edu/dftwiki/images/f/f6/Frog3.gif. If you use your own image, make sure you call it Frog3.gif. When your program is tested, it will use the Frog3.gif image shown here. You will not be able to upload your own image.
The Banner
The banner at the top of the screen is an object of a class called Banner, which is given below, along with a main program that illustrates how to use it.
# bannerDemo.py # D. Thiebaut # A short program illustrating the use of a banner in # graphics. from graphics import * WIDTH=800 HEIGHT=600 class Banner: def __init__( self, message ): """constructor. Creates a message at the top of the graphics window""" self.text = Text( Point( WIDTH//2, 20 ), message ) self.text.setFill( "black" ) self.text.setTextColor( "black" ) self.text.setSize( 20 ) def draw( self, win ): """draws the text of the banner on the graphics window""" self.text.draw( win ) self.win = win def setText( self, message ): """change the text of the banner.""" self.text.setText( message ) def main(): # creates a window win = GraphWin( "Demo", WIDTH, HEIGHT ) # draw the banner at the top banner = Banner( "Demo. Please click the mouse!" ) banner.draw( win ) # wait for the user to click the mouse before continuing... p = win.getMouse() # update the banner banner.setText( "Mouse clicked at Point({0:1}, {1:1})" .format( p.getX(), p.getY() ) ) # wait again, and update the banner one more time p = win.getMouse() banner.setText( "Mouse clicked again, at Point({0:1}, {1:1})" .format( p.getX(), p.getY() ) ) win.getMouse() win.close() main()
Implementation
- Call your program final3.py
- You must use objects to define the cars. Your program should implement cars going both sides of the road, in opposite directions.
- The number of cars used by the program is fixed and does not grow as the game evolves. In the video, the number of cars is 3, but you can use more. When a car disappears on one end of the screen it reappears on the other side, after a short delay. The delay is up to you.
- The frog moves up or down by a fixed amount. To make the frog move up, the user must click the mouse in the area of the window above the road. To make the frog move down, the user clicks the mouse in the area of the window below the road.
- The frog "dies," or loses a life point when it is run over by a car. You are free to determine the conditions for which the program will determine that the car is running over the frog. In the video the top or bottom of the frog can overlap slightly the body of a car without triggering the program to detect an overlap. There is flexibility.
- The program automatically brings the frog back down to its initial position when a car runs over it, or when the frog has fully crossed the road.
Grading
- Assuming that your program is well documented, and nicely organized with functions and classes, the following features will be worth various number of points. A grade of 100 will be given to a well documented and organized program that behaves similarly to the game illustrated in the YouTube video, but with two lanes of cars, one lane going left to right, one going right to left. The number of cars must remain constant, and cars disappearing on one end are made to reappear on the other side.
- Banner working correctly: 5 points
- Cars moving off the graphics window and reappearing on the other side: 5 points.
- Game stopping when the number of life points reaches 0: 5 points
- Frog and car intersection works well: 5 points
- Frog moved back to original position when run over, or when fully crossed: 5 points
- Frog moves up and down on mouse clicks: 10 points.
Submission
- Submit your program in the Final 3 Section area on Moodle.
</showafterdate>