CSC103 Assembly Language Lab (version 2) 2013
--D. Thiebaut 07:15, 20 February 2012 (EST)
Revised --D. Thiebaut 07:06, 4 October 2012 (EDT) and --D. Thiebaut (talk) 08:28, 19 September 2013 (EDT)
This lab deals with assembly language and the computer simulator. Work on this lab with a partner. It's easier to figure out some of these questions with another person. This lab uses numbers instead of variables when referring to memory.
Contents
Preparation
Start
- Point your browser to the simulator and start it (third button at the bottom)
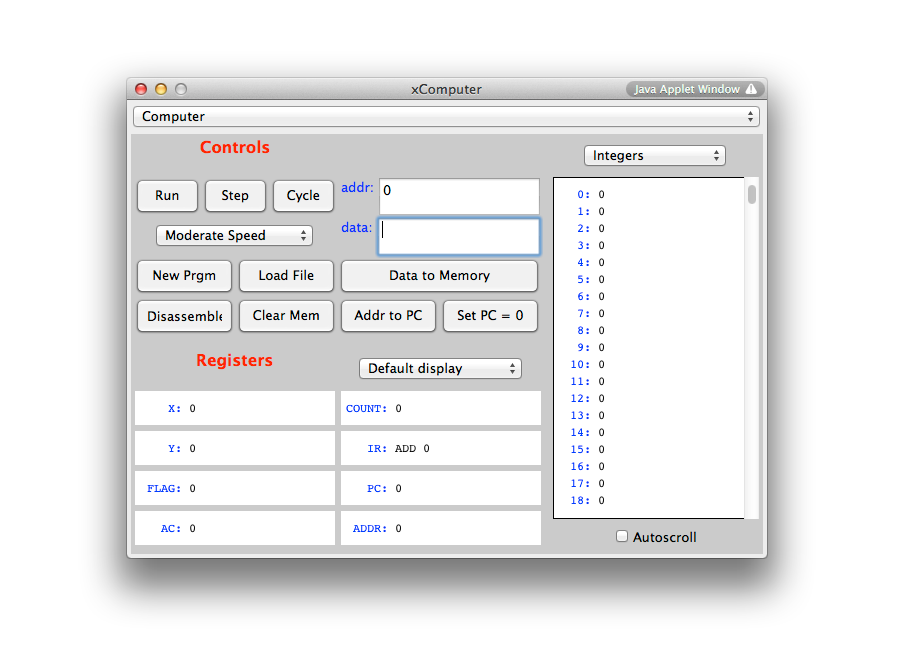
Editing Program
- Click on the top bar at the top of the simulator applet, which says computer
- In the edit window that opens up, enter this simple program:
- Enter a simple program in the window:
@0
lod-c 1
sto 10
hlt
@10
0
Translating/loading into RAM
- Click on translate
- Observe that your program has been translated, or coded into numbers. Numbers are the only thing the processor can understand.
- If the top right button says Integer, click on it so that it shows Instructions. Observe that numbers can mean instructions as well as numbers for the processor.
- Click on the top right button and see how the memory can be seen many different ways. The real contents of the memory is when you ask to see it in binary. That's what inside the memory of a computer at any given time. Bits. But because these bits are used in a code, they can mean different things.
Execute the Progam
Single Stepping
- Click on the button that says "Set PC = 0" to make sure the processor will execute the program starting at 0
- Click on the Cycle button and observe that the number 1 is going into the AC register and then into memory, at Address 10.
Running the program
- If you want to run the program full speed rather that executing it a step at a time, the procedure is slightly different.
- First, change the program so that it loads a number different from 1 into AC:
lod-c 55
- Translate
- set PC = 0
- Click Run instead of Cycle.
- This runs the program.
- Observe that 55 will be loaded up from memory into AC, and then stored into the variable var1, at Address 10.
- You now know the basics of programming in assembly language.
Problem 1
- Using the steps you have just gone through, write a program that initializes 3 memory locations at 10, 11, and 12 with the value 23. You should end up with the number 23 in three different memory locations.
Problem 2
- Something very similar to the previous problem, but instead of storing the same value in all three variables, store 9, 19, 13 (this could be today's date) at Addresses 10, 11, and 12, respectively.
Problem 3
- Try this program out:
@0
lod 10
sto 11
hlt
@10
55
0
- Run it.
- Observe the result, and in particular what happens to Memory Location 11.
- Add two new variables to the data section at Addresses 12 and 13.
- Modify the program so that it copies the contents of Address 10 into the locations at Addresses 11, 12, and 13.
- Verify that your program works
- Modify the number at Address 10 and restart the program. Does the program put the new value in the other 3 variables?
Problem 4
- Try this program out:
@0
lod 10
add-c 1
sto 10
hlt
@10
55
66
3
- Run the program
- What does it do? What's the result of the computation?
- Once you've figured it out, modify the program so that Address 10 originally starts with the value 5 in it, and then ends with the value 7.
- Verify that your program will add 2 to the variable at Address 10, whatever its original value.
- Once your programs works, modify the program so that it adds two to all three variables at Addresses 10, 11, and 12.
Problem 5
- Try this new program out:
@0
lod 10
add-c 1
sto 10
jmp 1
@10
55
55
- Run the program. Study it.
- Modify it so that as the program runs, the contents of Address 10 increases by 1 every step through the loop, and the contents of Address 11 decreases by one through the loop. In other words, when the program starts, Locations 10 and 11 contain 55. Then, a few cycles later, Location 10 becomes 56 and Location 11 54. Then a few cycles later, 57, and 53, etc.
Mini Challenge of the Day |
Write a program that contains 4 variables starting at Address 10. The first 3 are initialized with 10, 20, and 30. The last one contains 0. Let's call the Location 10 var1, Location 11 var2, Location 12 var3, and Location 13 sum.
Your program should computes 3 * var1 + 2 * var2 + var3 and store the result into the variable sum. In our case, your program will compute 3*10+ 2*20+ 30 = 100 which it will store in sum.
Hints: If you look closely at the set of instructions for our processor, there is no multiplication instruction... So your solution has to use another method for computing the value of 3 * var1...
THE END!
Solutions
P1
; Solution for Problem 1
; D. Thiebaut
start: lod-c 23
sto 10
sto 11
sto 12
hlt
@10
0
0
0
P2
; Solution for Problem 2
; D. Thiebaut
start: lod 10
sto 11
sto 12
sto 13
hlt
@10
55
0
0
0
P3
; Solution for Problem 3
; D. Thiebaut
start: lod 10
add-c 1
sto 10
lod 11
inc
sto 11
lod 12
add-c 1
sto 12
hlt
@10
55
66
3
P4
start: lod 10
add-c 2
sto 10
lod 11
add-c 2
sto 11
lod 12
add-c 2
sto 12
hlt
@10
55
66
3
P5
; Solution for Problem 5
; D. Thiebaut
; First initialize both variables with 55
start: lod-c 55
sto 15
sto 16
; now loop and increment var1 and decrement var2
; the loop is endless.
loop: lod 15
add-c 1
sto 15
lod 16
dec
sto 16
jmp loop
hlt
@15
0
0