CSC103 Javascript Lab 2012
--D. Thiebaut 21:07, 21 February 2012 (EST)
<meta name="keywords" content="computer science, How Computers Work, Dominique Thiebaut, smith college" />
<meta name="description" content="Dominique Thiebaut's Web Page" />
<meta name="title" content="Dominique Thiebaut -- Computer Science" />
<meta name="abstract" content="Dominique Thiebaut's Computer Science Web pages" />
<meta name="author" content="thiebaut at cs.smith.edu" />
<meta name="distribution" content="Global" />
<meta name="revisit-after" content="10 days" />
<meta name="copyright" content="(c) D. Thiebaut 2000, 2001, 2002, 2003, 2004, 2005, 2006, 2007,2008" />
<meta name="robots" content="FOLLOW,INDEX" />
This lab is your first introduction to a high-level language. The language is javascript and is used to create programs inside Web pages, to generate dynamic elements of Web pages. Writing programs in javascript is extremely easy in terms of the tools you need. All you need is a computer with an editor, and a Web browser.
Although we will use a special editor that is on the Web for this lab, all you need is simply a browser, and an editor. Notepad in Windows, or TextEdit with the Mac are good editors. If you have Dreamweaver installed on your computer, you can also use it write javascript programs. Dreamweaver is very sophisticated, and you may like it better than the simpler solutions we have adopted here. <P> Go through the lab at your own space. Working in pairs is a good way to perform the lab.
The Tools
In this section you will pick the environment that is best for you. Depending on whether you are using a Mac or Windows computer, and whether it has Dreamweaver installed on it or not, pick the subsection that applies to you, perform the different steps, and you will be ready for the remainder of the lab.
If you have a Mac but no Internet connection
- If you have a Mac but are not connected to the Internet, here is a way to write a javascript program and test it.
- Open the application TextEdit
- Go in the Preference Menu, and click on Plain Text
- Create a new document
- Copy/Paste the code below in the TextEdit window
<html><head><title>CSC103 Javascript Program</title></head>
<body>
<h1>My first program</h1>
<script language="JavaScript" type="text/javascript"><!--
//Enter your code below this line:
//Do not write below this line.
//-->
</script>
</body></html>
- Save the file on your Desktop as skel.html (TextEdit will not be happy about the .html extension, but force it to accept it!)
- Go to your Desktop and double-click on the skel.html icon. You should see your default browser open up and show you the Web page you just created.
- That is the simplest way to write java script programs on a Mac if you do not have a Web connection. You can do all the steps of this lab this way if you elect to.
If you have Windows but no Internet connection
- The steps are similar:
- Open the program Notepad
- Create a new document
- Copy/Paste the code below in Notepad:
<html><head><title>CSC103 Javascript Program</title></head>
<body>
<h1>My first program</h1>
<script language="JavaScript" type="text/javascript"><!--
//Enter your code below this line:
//Do not write below this line.
//-->
</script>
</body></html>
- Save the file to your Desktop as skel.html
- Go to your Desktop and double-click on the skel.html icon. You should see your default browser open up and show you the Web page you just created.
- That is the simplest way to write javascript programs on Windows if you do not have a Web connection. You can do all the steps of this lab this way if you elect to.
If you have a Mac or Windows machine with Internet connection
- There is a very nice and easy to use editor at this location: http://htmledit.squarefree.com/
- (If that site is not reachable, try it here: http://xgridmac.dyndns.org/htmlEditor.html )
- Go to the site and paste the skeleton program above in the top, blue frame.
- You should see the result displayed directly in the lower, white frame.
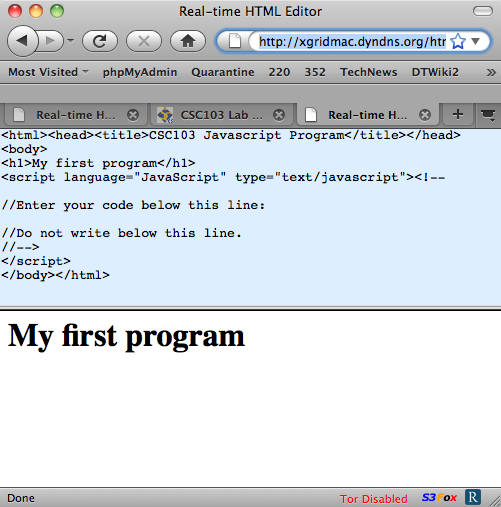
If you have Dreamweaver on your computer
- Start Dreamweaver
- Start Dreamweaver, and click on Code at the top left of the window as shown in the image to the right.
- Type in the following code in the edit window:
<html><head><title>CSC103 Javascript Program</title></head>
<body>
<h1>My first program</h1>
<script language="JavaScript" type="text/javascript"><!--
//Enter your code below this line:
//Do not write below this line.
//-->
</script>
</body></html>
- Save your file on your Desktop, or to your H: drive, and call it skel.html.
- View your file. Click on the globe icon, and select one of the browsers listed.
- That's it.
You now have the tools required to write and test Javascript programs, and observe their output.
Printing text on the Web page
Printing text on the Web page in Javascript is done with the command document.write( "..." ); where you replace the ellipsis with the text you want to see appear.
Here's an example, which you should type in your file:
//Enter your code below this line: document.write( "<h2>Monday, Feb 27, 2012</h2>" ); document.write( "<h3>CSC103 Lab with my partner</h3>" ); //Do not write below this line.
- Save your file and give it a name such as printText.html.
- Load this file in the browser using one of the methods illustrated in Section 1 above.
- Modify your printText.html file and make your Javascript program display the following information with two document.write( ... ) statements.
<P>Our class web site can be found
<a href='http://cs.smith.edu/dftwiki/index.php/CSC103_Weekly_Schedule_2012'>here</a>.
<P>The main page for Smith College is <a href='http://www.smith.edu'>here</a>.
Here's the way it should look like:
document.write( "<P>Our Web site is <a href='http://cs.smith.edu/dftwiki/index.php/CSC103_Weekly_Schedule_2012'>here</a>.");
Adding comments to your program
Comments are created by putting // on a line of a Javascript program. Everything that follows the // is not read by the browser.
Example:
//Enter your code below this line:
// display the date
document.write( "<h2>Monday, Feb 27 , 2012</h2>" );
// display subtitle
document.write( "<h3>CSC103 Lab with my partner</h3>" );
// show links to useful pages:
document.write( "<P>Our class web site can be found <a href="href='http://cs.smith.edu/dftwiki/index.php/CSC103_Weekly_Schedule_2012">here</a>.");
document.write( "<P>The main page for Smith College is <a href="http://www.smith.edu">here</a>.");
//Do not write below this line.
- Modify your program and add comments to indicate what the different sections do.
Using variables to store strings of characters
Most programming languages support variables. A variable is a name associated with some area of memory containing information that the program needs.
Example:
// Define identity of programmer
var firstName = "Carol";
var lastName = "Christ";
var office = "College Hall";
document.write( "<P>Smith College president:" + firstName + " " + lastName );
The <P> string is an html tag that starts a new paragraph, forcing the text that follows to be on a new line.
Exercise
- Modify your Javascript program so that it uses 4 variables for your first name, last name, your class, and for your box number. Make the program output this information so that it displays in this fashion:
Alex Andra , Class of 2012.
Box 1234
Using variables to store numbers
Example:
// Number of operations performed by a 3GHz processor in one second
var numberOpsPerSec = 3000000000;
// Length of time required for a human being to perform same number of 1-second actions
var numberSeconds = numberOpsPerSec;
var numberMinutes = numberSeconds / 60;
var numberHours = numberMinutes / 60;
var numberDays = numberHours / 24;
var numberYears = numberDays / 365;
// display the information
document.write( "<P>If a human being were to carry out the same number of" );
document.write( " basic operations a 3 GHz processor performs in one second," );
document.write( " it would take:<P>" );
document.write( numberSeconds + " seconds, or<P>" );
document.write( numberMinutes + " minutes, or<P>" );
document.write( numberHours + " hours, or<P>" );
document.write( numberDays + " days, or<P>" );
document.write( numberYears + " years!" );
Exercise
- Change the 3000000000 number and replace it by 1000000000.
- Verify that the program outputs a new series of numbers that are 1/3 the numbers above.
That's what programs are good for! They allow us to encapsulate recipes of actions in scripts and by submitting different inputs to these scripts, we get new outputs!
A Javascript Program that adds two numbers together!
Exercise
- Using the example of the previous section, write a program that will store two numbers, say 3 and 9 in two different variables, say x and y, and that computes the sum of the two and stores the result in a third variable, say z.
- Make your program output the result in the following way:
x = 3 |
y = 9 |
z = 3 + 9 = 12 |
- Modify the line that defines the variable x and store a different number into it:
var x = 10;
- Do not modify any other line in your code!
- Run your program. Verify that the output of the program is consistent with the new change:
x = 10 |
y = 9 |
z = 10 + 9 = 19 |
Loops in Javascript
A typical loop in Javascript looks like this:
var i; for ( i = 1; i <= 10; i++ ) { // javascript statements }
First, we define a variable i which will be our loop counter. Then we have a construct with three clauses:
- i = 1 : this indicates that when the loop starts, i takes on the value 1.
- i <= 10 : as long as i is less than or equal to 10, the loop keeps on going.
- i++ : every time we are done doing all the statements in the body of the loop, i get incremented.
To see this in action, create a new javascript program with the following code in it:
// define loop counter and "accumulator" variable var i; var x = 1; // loop 10 times for ( i=1; i<=10; i++ ) { document.write("Number " + x + " is " + x ); document.write("<br>"); x = x + 1; // double value of x }
- Run it. Do you recognize something we have seen before?
- Modify the program so that it prints all the numbers between 10 and 20.
- Modify the program so that it computes and display the sum of all the numbers, from 1 to 10.
Challenging problem of the day
First a story (taken from Wikipedia):
- When the creator of the game of chess (in some tellings, an ancient Indian mathematician, in others, a legendary dravida vellalar named Sessa or Sissa) showed his invention to the ruler of the country, the ruler was so pleased that he gave the inventor the right to name his prize for the invention. The man, who was very wise, asked the king this: that for the first square of the chess board, he would receive one grain of wheat (in some tellings, rice), two for the second one, four on the third one and so forth, doubling the amount each time. The ruler, who was not strong in math, quickly accepted the inventor's offer, even getting offended by his perceived notion that the inventor was asking for such a low price, and ordered the treasurer to count and hand over the wheat to the inventor. However, when the treasurer took more than a week to calculate the amount of wheat, the ruler asked him for a reason for his tardiness. The treasurer then gave him the result of the calculation, and explained that it would be impossible to give the inventor the reward. The ruler then, to get back at the inventor who tried to outsmart him, told the inventor that in order for him to receive his reward, he was to count every single grain that was given to him, in order to make sure that the ruler was not stealing from him.
- Write a Javascript program that displays the number of grains of wheat (or rice) that one must put on the 64th square of the chess board. Your program should display only one number, as follows:
The number of grains of wheat on the 64th square of the board is xxxxxxxxxxxxx.
- You must figure out how to make your program compute xxxxxxxxxxxx!
- To test your program, make it compute first the number of grains on the 1st square, or the 5th square, for which the answers are easy to check. There should be 1 grain on the first square, and 16 on the 5th square.
- The big picture
- Ask Google "What is the weight of a grain of rice?" and you will find some good answers, probably from the site answers.com.
- Once you know the answer, ask wolframAlpha how much xxxxxxxxxxxxx times the weight of a grain of rice weigh...
- Of course, you could have made the Javascript program compute that answer... :-)
Javascript Resources
Here is a list of good resources for learning/playing with Javascript.
- Tutorials and examples of Javascript code. Try the editor, where you can test code and see the result of executing it in the same window.
- A complete tutorial from Brown University.
- Javascript for the non programmer. Another nicely done tutorial.
THE END!