CSC103 Processing Lab -- Part 2
--D. Thiebaut 20:23, 27 February 2012 (EST)
Revised --D. Thiebaut (talk) 10:49, 10 October 2017 (EDT)
This is Part 2 of a lab on the Processing language. You can find Part 1 here. There is a moodle submission for this lab. See Challenge #3.
Contents
Testing Conditions with the if-Statement
Testing the Mouse Position
- Let's change the color of the circles being drawn by our last sketch so that the circles are red when they appear in the left side of the window, and orange when they appear in the right side of the window.
- This is done by using and if statement, with its else counterpart. The pseudo-code for doing this reads as follows:
if ( mouseX is in the left half ) { // set the color of the circle to red } else { // set the color of the circle to orange }
- Writing this in Processing, we get
void setup() {
size(480, 480 );
smooth();
}
void draw() {
//background( 200 );
if ( mouseX < 240 ) {
fill( 231, 47, 39 ); // red
}
else {
fill( 255, 200, 8 ); // orange
}
ellipse(mouseX, mouseY, 80, 80);
}
- Try it!
Variation #1 |
See what happens when you replace mouseX with mouseY in the if statement...
Testing If the Mouse Button is Pressed
- Processing uses a predefined boolean variable, i.e. a variable that contains either true or false to indicate whether the user is currently pressing the left mouse button or not.
- We can test for this condition easily:
if ( mousePressed == true ) {
// put code here to be executed if the button is pressed
}
else {
// put code here to be executed if the button is not pressed
}
- Let's use this to change the color of the circle depending on whether the mouse is pressed or not:
void setup() {
size(480, 480 );
smooth();
}
void draw() {
//background( 200 );
if ( mousePressed == true ) {
fill( 231, 47, 39 ); // red
}
else {
fill( 255, 200, 8 ); // orange
}
ellipse(mouseX, mouseY, 80, 80);
}
- Try it!
Challenge #1 |
Make the program switch between a circle and a rectangle as the user presses the mouse button. For example, the program will display a circle if the mouse is not pressed, and will display a rectangle if the mouse is pressed.
Challenge #2 |
Make your program behave the opposite way: it will display a circle if the mouse is pressed, and display a rectangle if it is not.
- Hints: You can use "!=" instead of "==" to test if some quantity is not equal to something.
Challenge #3 |
Make the program switch between a red circle and an orange rectangle as the user presses the mouse button. The program will keep on displaying several shapes, and you will simply control their color with the mouse bouton. Make sure you include your name on the graphic window, and in the code of your sketch. (See Youtube video below for an example of what your sketch should do.)
Moodle Submission
Submit a screen shot of your graphic window with circles red circle and orange rectangles. The submit section is labeled "Processing Lab 2." You have until Thursday 10/19, 12:00 p.m. for the submission.
This is the only submission you need to do for Lab 2, but do not stop here. Keep on going till the end of the lab.
Previous Mouse Position
- Processing keeps track of where the mouse currently is by its mouseX, and mouseY coordinates, but also where it was the last time the function draw() was called, and this old position is kept by Processing in two variables called pmouseX and pmouseY.
- We can use this feature to draw lines between the successive positions of the mouse.
- Try this code:
void setup() {
size(480, 480 );
smooth();
}
void draw() {
//background( 200 );
line( mouseX, mouseY, pmouseX, pmouseY );
}
- You can now draw with Processing!
Challenge #4 |
Modify the program so that the mouse draws the line only when the mouse button is pressed.
Note: if you want to make your line fatter, call the function strokeWeight( 10 ) inside draw(), just before calling the line() function. You may use other numbers besides 10.
Another Control Structure: Loops
- Study the sketch below taken from openprocessing.org, and then run it.
void setup() {
size( 400, 400 );
smooth();
}
void draw() {
background(200);
// count from 20 to 380 jumping 20 each time.
for( int x = 20; x < 400; x = x + 20 ) {
// and draw a circle in that position, on a horizontal line.
ellipse( x, 200, 15,15 );
}
}
- Notice that the sketch creates 19 circles on a horizontal lines.
Challenge #5 |
- Modify your sketch so that it displays the circles vertically, as shown below (you can add a call to fill( 250, 200, 8 ) in the loop to create the orange fill color):
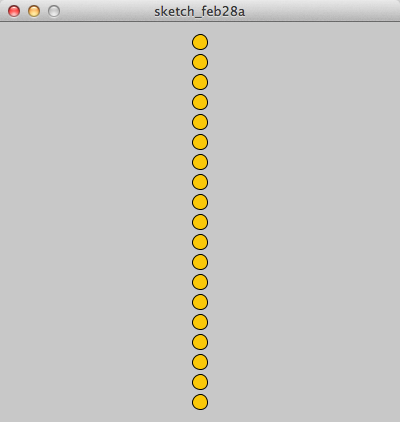
- Try generating this pattern:
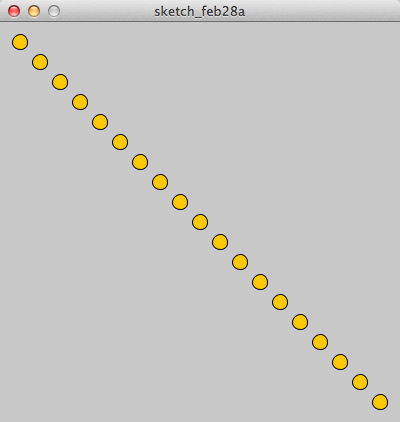
Challenge #6 |
- Make the mouse control how many circles are displayed, so that no circles can be shown on the right-hand side of the mouse, as illustrated below. As the mouse moves to the right, more circles are shown. As the mouse moves to the left, fewer.
Last Challenge of the Day |
- Try to create a sketch whose behavior is similar to what is displayed in the image below. Your sketch should have the following properties:
- It is based on the same sketch you have been working with
- If the center of a circle is to the right of the mouse, the circle is not displayed
- The circles have radii that increase as they get lower in the window
- The color of the circles changes with each circle.
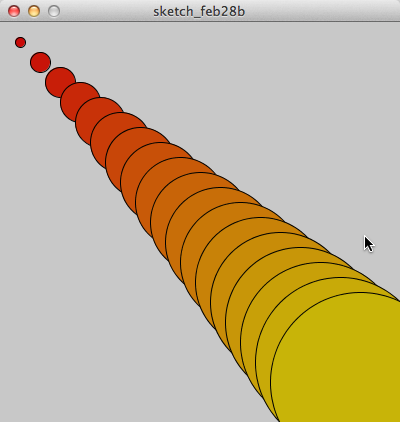
Useful Trick: Displaying the Mouse Coordinates
Option 1: In the Console
- To display the mouse coordinates in the Console Window, you can call the println() function inside the draw() function:
void setup() {
size(480, 480 );
smooth();
}
void draw() {
background(200);
fill( 0 );
ellipse( mouseX, mouseY, 80, 80 );
println( mouseX+" "+mouseY )
}
Option 2: Inside the Graphics Window (optional for now)
- To display the mouse coordinates in the Graphics Window, you can use the text() function, which requires you to give it a string and two numbers defining where to display the string in the window. Here's an example below:
void setup() {
size(480, 480 );
smooth();
}
void draw() {
background(200);
fill( 0 );
text( "("+mouseX+" "+mouseY+")", mouseX, mouseY );
}
Optional Section, if you are done early
- Visit the Learning section on Processing.org and play with some of the examples. It is a good way to learn a programming language. You may want to copy/paste some examples and modify their code to see the result.
- Have fun!
Solution sketches for all the challenges are available here.