CSC111 Homework 9
This homework assignment is due on 4/8/10, at 11:59 p.m. plus 1 minute. It deals with while loops. |
Problem #1
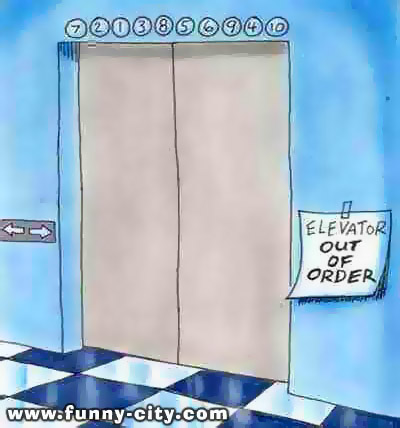
def main():
people = [ ("JT", 150), ("TJ", 80), ("TT", 120), ("BT", 170), ("Mike", 12), \
("AF", 27), ("Lea", 78), ("Leo", 180), ("Al", 55), ("GK", 110), \
("JR", 111), ("VB", 76) ]
Above is a list of people and their weight. They are waiting in front of an elevator. The maximum weight allowed on the elevator is 300 lbs.
Write a program called hw9a.py that will compute and output the answers to the following questions:
- Question 1
- What is the maximum number of people that can fit in the elevator?
- Question 2
- What is the total weight of the people who fit in the elevator?
- Question 3
- What is the name of the people in the elevator?
- Use while loops!
- Once your program works, modify it and make it input the total weight allowed in the elevator from the user. The allowed range allowed by your program should be any number between 0 and 1000. If the number entered by the user is less than 0 or greater than 1000, your program should prompt the user to input that number again.
- Make sure your program works if the maximum weight allowed is 0, or when it is set to 1000.
Recommendations
- A full 1.5 point will be given to documentation. Make sure you look at past solutions of homework assignments to see what is expected for the program header, and for in-line comments.
- Use functions!
Submission
- Submit your program as follows:
submit hw9 hw9a.py
Problem 2. Computing e
The mathematical constant e is equal to 2.718281828459045...
It can be computed by adding the terms of the infinite series: e = 1 + 1/1! + 1/2! + 1/3! + 1/4! + ... where 4! is the factorial of 4, and is equal to 4 * 3 * 2 * 1.
- How many terms do we need to sum up to get the first 12 digits of e correctly, i.e. to obtain 2.71828182845? For reference, we will say that the series shown above for e contains 5 terms, starting with 1, and ending with 1/4!
- To answer this question, write a program that will compute and print out the answer. Store your program in a file called hw9b.py, and submit it as follows:
submit hw9 hw9b.py
- The answer should be at least something like this:
Number of terms added: 100 Final value of approximation of e = 2.71828182845823018710
- Pay attention to style and documentation, please!
- This program is very short, but tricky. It is very easy to compute the wrong terms and to be off by one when counting the number of terms! Be very careful, and make sure you test your code carefully! If your program outputs 100 and the answer is supposed to be 99, then your program will be judged as yielding the wrong output.
Problem 3. Optional and Extra Credit
Write a python program that reads a text file containing a description of your schedule and that outputs it in the form of a Web page, in html.
Call your program hw9c.py.
Reading Text from a File
This is covered in Chapter 8 of Zelle's text book, in Section 8.3.3 in particular. Your program will prompt the user for the name of the text file containing the schedule, and for the name of the html file where your page will be stored. It will then read the text file, parse the information in it, and then create the Web page.
Example of the user interaction with your program:
python hw9c.py input file name? schedule.txt output html file name? myschedule.htm schedule written to public_html/myschedule.htm file public_html/myschedule.htm readable by all
Format of the input file
Here is an example of a text file containing the schedule for Jane Moore:
name Jane Moore semester fall 2010 MWF 9 GEO 370 TR 9 CSC 111 MW 10 GOV 228 MWF 13 DAN 113 TR 13 FRN 254 F 15 ESS 940 F 14 ESS 940
If a line starts with the word "name", then the remainder of that line contains the name of the person. The name can be any number of words, but must fit on one line only. In other words, "Jane", "Jane Moore", "Jane O. Moore", "The soon to graduate Jane Moore" are all valid strings for the name.
If a line starts with the word "semester", then the remainder of that line is the semester for which the schedule applies. Any string that follows the word "semester" is a valid semester. So "Fall 2009", "S10", "Spring '11" are all valid names.
Any other line that starts with a word containing the letters M, T, W, R, or F corresponds to a course the person is taking. We will call such a line a schedule line. The first word of a schedule line always represents the day or days the course takes place. If the first word contains several of these letters, they are all "glued" together as one word. So "MWF" is valid, but "M W F" is not, and you can assume that your program will never be presented with invalid schedule lines.
The second word of a schedule line is the time at which the course starts. This is always an integer number in the range 8 to 16 included.
The last two words represent the department and course number. Each schedule line always has four words in it: a set of days, a time (in 24-hour format), a department designation, and a course number.
The text file may contain 0, 1, or several schedule lines. There are no restrictions on the number of such lines.
You may assume that the first two lines will always be the name and semester lines, and all the remaining lines will be schedule lines.
The HTML Output File
The html file output by your program will contain the schedule nicely formatted in a table, as illustrated below:
The html code for this page is shown below:
<html>
<title>Jane Moore's Schedule</title>
<body>
<h1>Jane Moore's Schedule</h1>
<h2>Fall 2010<h2>
<table border="1">
<tr><td>Day</td><td>8:00</td><td>9:00</td><td>10:00</td><td>11:00</td>
<td>12:00</td><td>13:00</td><td>14:00</td><td>15:00</td><td>16:00</td></tr>
<tr><td>Monday</td><td> </td><td>GEO 370</td><td>GOV 228</td><td> </td>
<td> </td><td>DAN 113</td><td> </td><td> </td><td> </td></tr>
<tr><td>Tuesday</td><td> </td><td>CSC 111</td><td> </td><td> </td>
<td> </td><td>FRN 254</td><td> </td><td> </td><td> </td></tr>
<tr><td>Wednesday</td><td> </td><td>GEO 370</td><td>GOV 228</td><td> </td>
<td> </td><td>DAN 113</td><td> </td><td> </td><td> </td></tr>
<tr><td>Thursday</td><td> </td><td>CSC 111</td><td> </td><td> </td>
<td> </td><td>FRN 254</td><td> </td><td> </td><td> </td></tr>
<tr><td>Friday</td><td> </td><td>GEO 370</td><td> </td><td> </td>
<td> </td><td>DAN 113</td><td>ESS 940</td><td>ESS 940</td><td> </td></tr>
</table>
</body>
</html>
To better understand how html table are created, visit the following links: Tutorial #1, Tutorial #2, Page 1, and Page 2.
Note that when you want a cell in a table to be empty, you need to store the string " " in it. stands for "non-breakable space". In other words, each cell of a table should contain some string, and if we do not want to see anything in a cell, we still have to store in it.
Submission
submit hw9 hw9c.py