CSC111 List of Objects
--D. Thiebaut 09:36, 1 November 2011 (EDT)
# ListsAndLoops.py
# D. Thiebaut
# This program illustrates how to use lists to
# keep track of objects, and for loops to iterate
# through the objects
#
from graphics import *
def main():
""" below is a very uninspiring main program that needs
some attention. It is inefficient. Code is repeated for
each of the 4 rectangles. Run it to see that it creates
an orange border around the graphic window"""
w = 600
h = 400
win = GraphWin( "Lists and Loops", w, h )
r1 = Rectangle( Point( 0, 0 ), Point( w, 10 ) )
r1.setFill( "orange" )
r1.setWidth( 0 )
r1.draw( win )
r2 = Rectangle( Point( 0, 0 ), Point( 10, h ) )
r2.setFill( "orange" )
r2.setWidth( 0 )
r2.draw( win )
r3 = Rectangle( Point( w, h ), Point( w-10, 0 ) )
r3.setFill( "orange" )
r3.setWidth( 0 )
r3.draw( win )
r4 = Rectangle( Point( w, h ), Point( 0, h-10 ) )
r4.setFill( "orange" )
r4.setWidth( 0 )
r4.draw( win )
win.getMouse()
win.close()
#========================<<< ANOTHER VERSION >>>==========================
def main2():
"""This is the more efficient version of main(). It puts the
rectangles in a list and uses a for loop to go through the
rectangles and draw them with the right attributes"""
w = 600
h = 400
win = GraphWin( "Lists and Loops", w, h )
border = [ Rectangle( Point( 0, 0 ), Point( w, 10 ) ),
Rectangle( Point( 0, 0 ), Point( 10, h ) ),
Rectangle( Point( w, h ), Point( w-10, 0 ) ),
Rectangle( Point( w, h ), Point( 0, h-10 ) ) ]
for r in border:
r.setFill( "orange" )
r.setWidth( 0 )
r.draw( win )
win.getMouse()
win.close()
#========================<<< ANOTHER VERSION >>>==========================
def drawBorder( win, x1, y1, x2, y2, x3, y3, x4, y4, x5, y5,
x6, y6, x7, y7, x8, y8, color):
"""gets the coordinates of 8 corner points and draws 4 rectangles
between them to form a border. The color of the border is passed
as a parameter"""
border = [ Rectangle( Point( x1, y1 ), Point( x2, y2 ) ),
Rectangle( Point( x3, y3 ), Point( x4, y4 ) ),
Rectangle( Point( x5, y5 ), Point( x6, y6 ) ),
Rectangle( Point( x7, y7 ), Point( x8, y8 ) ) ]
for r in border:
r.setFill( color )
r.setWidth( 0 )
r.draw( win )
def main3():
"""The same version as in main2(), but this time we use a function
to do most of the work"""
w = 600
h = 400
win = GraphWin( "Lists and Loops", w, h )
drawBorder( win, 0, 0, w, 10, 0, 0, 10, h, w, h, w-10, 0,
w, h, 0, h-10, "magenta" )
win.getMouse()
win.close()
#========================<<< ANOTHER VERSION >>>==========================
def main4():
"""Creating multiple borders. We parameterize the width of the rectangles
and loop to create sevral borders overlapping each other"""
w = 600
h = 400
win = GraphWin( "Lists and Loops", w, h )
# bw is the border width, and we start with a larger border first,
# going down to smaller ones, each border overlapping somewhat the
# preceding one.
for bw in [ 100, 80, 60, 40, 20, 10 ]:
drawBorder( win, 0, 0, w, bw, 0, 0, bw, h, w, h, w-bw, 0,
w, h, 0, h-bw, color_rgb( bw, bw*2, 200-bw ) )
win.getMouse()
win.close()
main4()
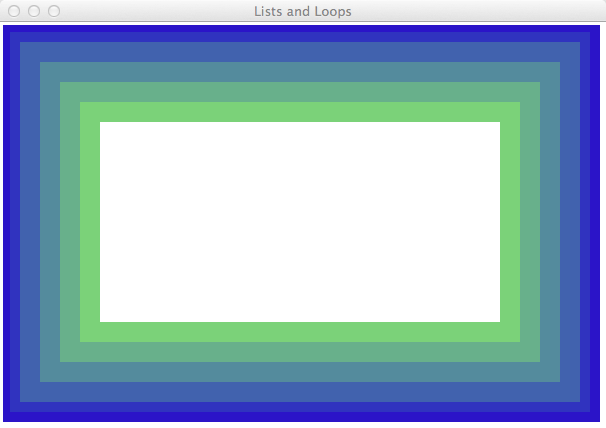