CSC111 Mapping Page
--D. Thiebaut (talk) 21:16, 6 April 2014 (EDT)
Contents
Resources
Map of Smith College
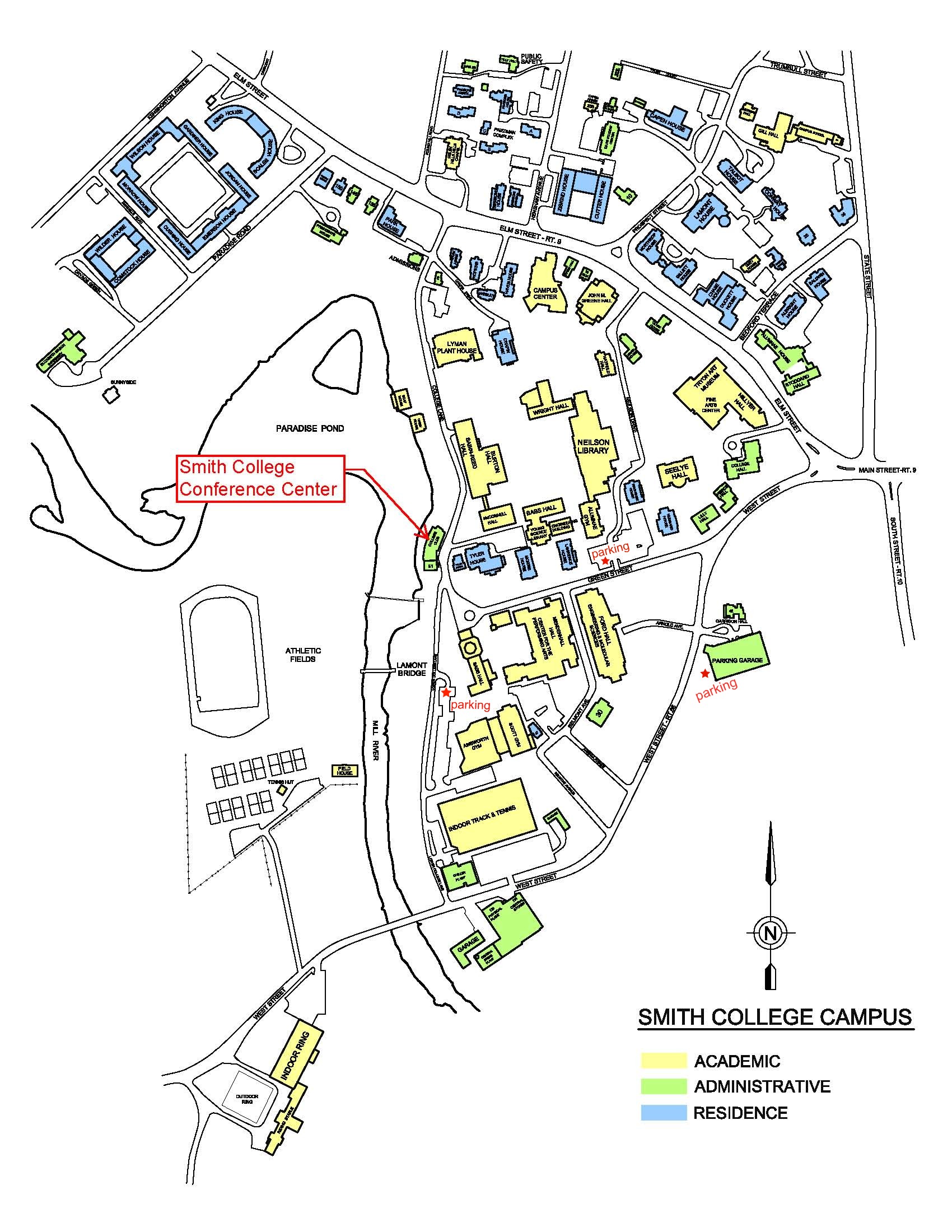
Graphics Library used in Textbook
- It is available for download here. Once downloaded, unzip it and move it to the directory where you are developing your programs.
Graphics Library
- Updated by DT: Horstmann's Library
Source
Code W/O Objects
# maps.py
# D. Thiebaut
from graphics2 import GraphicsWindow
MAXWIDTH = 800
MAXHEIGHT = 600
buildings = [
1030, # 0) offsetX
1064, # 1) offsetY
1.0, # 2) scaleX
1.0, # 3) scaleY
[ # 4) the list of list
( "Ford Hall",
(1030, 1064, 1073, 1050, 1122, 1201,1071, 1215 )),
( "Mendenhall Center for the Arts",
(1000, 1067, 1034, 1194, 907, 1223, 899, 1192,
952, 1177, 936, 1115, 924, 1119, 918, 1094 ) )
]
]
offsetX = None
offsetY = None
scaleX = None
scaleY = None
def resetOrigin( buildings ):
for building in buildings[4]:
name, list = building
minX = min( list[0::2] )
minY = min( list[1::2] )
buildings[0] = minX - 20
buildings[1] = minY - 20
def scale( x, y ):
global offsetX, offsetY, scaleX, scaleY
x = (x-offsetX) * scaleX
y = (y-offsetY) * scaleY
return x, y
def main():
global offsetX, offsetY, scaleX, scaleY
win = GraphicsWindow(MAXWIDTH, MAXHEIGHT)
canvas = win.canvas()
resetOrigin( buildings )
offsetX = buildings[0]
offsetY = buildings[1]
scaleX = buildings[2]
scaleY = buildings[3]
bList = buildings[4]
canvas.drawText( 1122, 1201, "MAPS" )
for bName, bCoords in bList:
scaledCoords = []
minX = min( bCoords[0::2] )
minY = min( bCoords[1::2] )
maxX = max( bCoords[0::2] )
maxY = max( bCoords[1::2] )
for i in range( 0, len( bCoords ), 2 ):
x, y = scale( bCoords[i], bCoords[i+1] )
scaledCoords.append( x )
scaledCoords.append( y )
print( "scaledCoords = ", scaledCoords )
canvas.drawPolygon( scaledCoords )
#canvas.setColor( "black" )
x, y = scale( (maxX+minX)//2, (maxY+minY)//2 )
canvas.drawText( x, y, bName )
win.wait()
main()
Source with Objects
from graphics2 import GraphicsWindow
MAXWIDTH = 800
MAXHEIGHT = 600
text = """0 # 0) offsetX
0 # 1) offsetY
1.0 # 2) scaleX
1.0 # 3) scaleY
Ford Hall
1030, 1064, 1073, 1050, 1122, 1201,1071, 1215
Mendenhall Center for the Arts
1000, 1067, 1034, 1194, 907, 1223, 899, 1192, 952, 1177, 936, 1115, 924, 1119, 918, 1094
"""
class Building:
def __init__( self, name, coords ):
self._name = name
self._coords = coords
self._minX = min( coords[0::2] )
self._minY = min( coords[1::2] )
self._maxX = max( coords[0::2] )
self._maxY = max( coords[1::2] )
self._halfX = ( self._minX + self._maxX )//2
self._halfY = ( self._minY + self._maxY )//2
def __str__( self ):
st = self._name + "\n"
st += "--> " + ", ".join( [str(k) for k in self._coords]) + "\n"
return st
def getMinX( self ):
return self._minX
def getMinY( self ):
return self._minY
def getMaxX( self ):
return self._maxX
def getMaxY( self ):
return self._maxY
def draw( self, canvas, offsetX, offsetY ):
xs = [ k-offsetX for k in self._coords[0::2] ]
ys = [ k-offsetY for k in self._coords[1::2] ]
coords = []
for i in range( len( xs ) ):
coords.append( xs[i] )
coords.append( ys[i] )
canvas.drawPolygon( coords )
canvas.drawText( self._halfX-offsetX, self._halfY-offsetY, self._name )
class BuildingList:
def __init__( self, text ):
# parse the text into lines
lines = text.split( "\n" )
#print( "lines = ", lines )
self._offsetX = int( lines[0].split( "#" )[0].strip() )
self._offsetY = int( lines[1].split( "#" )[0].strip() )
self._scaleX = float( lines[2].split( "#" )[0].strip() )
self._scaleY = float( lines[3].split( "#" )[0].strip() )
# concentrate on lines 4 till the end (building names and coords)
lines = lines[4:]
if len( lines[-1] )==0:
lines.pop( -1 )
# create a list of buildings
self._buildingList = []
print( "lines = ", lines )
for i in range( 0, len( lines ), 2 ):
name = lines[i].strip()
print( "name = ", name )
list = lines[i+1].strip().split( "," )
coords = [ int( k.strip() ) for k in list ]
self._buildingList.append( Building( name, coords ) )
# compute the bounding box
minX = self._buildingList[0].getMinX()
minY = self._buildingList[0].getMinY()
maxX = self._buildingList[0].getMaxX()
maxY = self._buildingList[0].getMaxY()
for building in self._buildingList[1:]:
minX = min( minX, building.getMinX() )
minY = min( minY, building.getMinY() )
maxX = max( maxX, building.getMaxX() )
maxY = max( maxY, building.getMaxY() )
# set the offset
self._offsetX = minX - 10
self._offsetY = minY - 10
# set canvas variable. Will be set later.
self._canvas = None
def setCanvas( self, canvas ):
self._canvas = canvas
def __str__( self ):
st = "Buildings\n"
st += "offsetX = %4d\n" % self._offsetX
st += "offsetY = %4d\n" % self._offsetY
st += "scaleX = %4.2f\n" % self._scaleX
st += "scaleY = %4.2f\n" % self._scaleY
for building in self._buildingList:
st += str( building )
return st
def getBuildingLength( self ):
return len( self._buildingList )
def getBuildings( self ):
return self._buildingList
def rescaleAll( self ):
return
def draw( self, canvas ):
self._canvas = canvas
for building in self._buildingList:
building.draw( canvas, self._offsetX, self._offsetY )
def main():
buildings = BuildingList( text )
print( buildings )
win = GraphicsWindow(MAXWIDTH, MAXHEIGHT)
canvas = win.canvas()
buildings.setCanvas( canvas )
buildings.draw( canvas )
win.wait()
main()