Tutorial: Moodle VPL -- A Multi-File Java Program with Data File
--D. Thiebaut (talk) 15:23, 13 June 2014 (EDT)
Contents |
This tutorial builds on the first one in the series, which should be done first, as the present tutorial skips some steps. This tutorial generates a VPL activity that allows automatic evaluation of a Java program with 2 classes stored in 2 files. The application gets its input from a data file. The evaluation tests the program with 3 different data files.
Setup
- Moodle Version 2.7 + (Build: 20140529)
- VPL Version 3.0.1
- For details on how Moodle and VPL were installed, go to this page.
VPL Activity Description
Java Test 2: Two Classes and One Data File
Due date: Tuesday, 21 June 2016, 8:00 PM Requested files: Main.java, SortedList.java, data.txt (Download) Type of work: Individual work Grade settings: Maximum grade: 100 Run: Yes Evaluate: Yes Automatic grade: Yes
Requested files
data.txt
3 4 5 1 10 11 11 -1 100 3 9 110
Execution files
vpl_run.sh
#! /bin/bash cat > vpl_execution << 'EOF' #! /bin/bash javac -J-Xmx128m *.java java Main EOF chmod +x vpl_execution
vpl_debug.sh
File not needed
vpl_evaluate.sh
File not needed
vpl_evaluate.cases
case = Test 1
input = data.txt
output = "File name? min = -1
max = 110
"
case = Test 2
input = data1.txt
output = "File name? min = 1
max = 1
"
case = Test 3
input = data2.txt
output = "File name? Empty array
min undefined
max undefined
"
case = Test 4
input = data3.txt
output = "File name? min = 10
max = 10
"
data1.txt
1 1 1 1 1
data2.txt
Empty file (does not contain any number)
data3.txt
10
Advanced Settings/Files to Keep
- Make sure to click on all the data files you are adding to the module, so that they will be sent to the jail server for testing the student program.
Testing
- Enter the following code in the Edit windows of the Test activity area. Pick the appropriate file name for the given code.
// Main.java import java.io.BufferedReader; import java.io.File; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.Collections; import java.util.Scanner; public class Main { public static void main(String[] args) { BufferedReader br = null; String sCurrentLine = null; SortedList array = new SortedList(); Scanner keyboard = new Scanner( System.in ); System.out.print("File name? "); String fileName = keyboard.next().trim(); try { br = new BufferedReader( new FileReader( fileName ) ); while ((sCurrentLine = br.readLine()) != null) { int x = Integer.parseInt( sCurrentLine.trim() ); array.add( x ); } } catch (IOException e) { //e.printStackTrace(); System.err.println( "Could not find file" ); return; } finally { try { if (br != null) br.close(); } catch (IOException ex) { //ex.printStackTrace(); return; } } if ( array.isEmpty() ) { System.out.println( "Empty array" ); System.out.println( "min undefined" ); System.out.println( "max undefined" ); return; } int max = array.getLast(); int min = array.getFirst(); System.out.println( "min = " + min ); System.out.println( "max = " + max ); } }
- Second program file:
import java.util.ArrayList;
import java.util.Collections;
public class SortedList {
ArrayList<Integer> array = null;
SortedList() {
array = new ArrayList<Integer>();
}
public void add( int x ) {
array.add( x );
Collections.sort( array );
}
public boolean isEmpty() {
return array.isEmpty();
}
public int getFirst( ) {
return array.get( 0 );
}
public int getLast( ) {
return array.get( array.size() - 1 );
}
}
- Click on Save, then Run and verify that you get a correct output in the terminal window.
- Click on Evaluate and verify that you get 100/100 for the 4 tests.
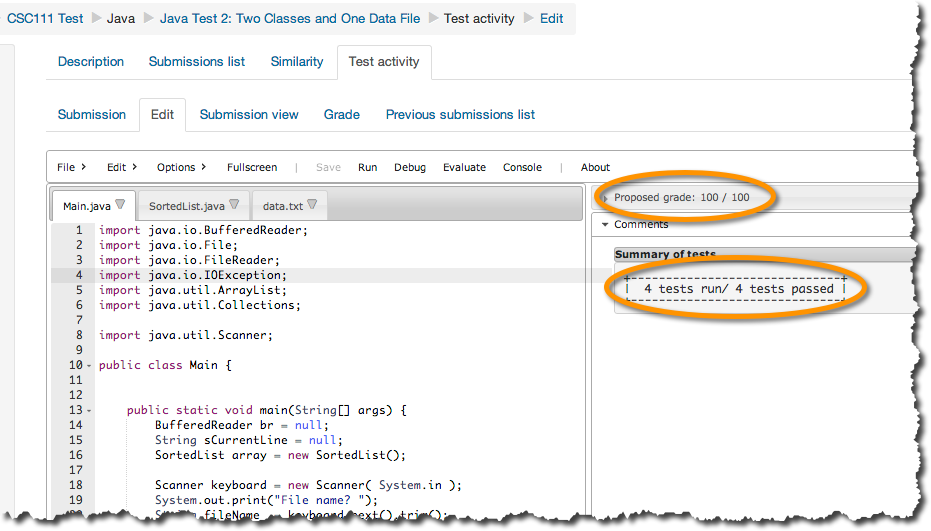
This concludes this tutorial