Tutorial: The Basics of a Simple GWT Canvas App
--D. Thiebaut (talk) 20:16, 1 July 2014 (EDT)
This tutorial takes on a very good tutorial by Chad Lung found on giantflyingsaucer.com (you should follow it first), and highlights the intricate relationships that exist between different tags and labels. If you project doesn't follow this set of dependencies, it will not work! The project in question is based on a GWT Web application that makes use of the HTML5 canvas to draw random rectangles on it.
Setup
- Mac OSX 10.8.5 with Java SDK 1.7
- Eclipse Kepler
- Google WebKit gwt-6.2.1.
You can find information on setting up GWT for Eclipse on this site.
Create a New Project
- In Eclipse, create a new Project: File/New/Project
- Pick Web Application Project. Then Next
- Project Name: CanvasExample, Package: xyz (or pick some other path, such as com.mycompany.app), check Use Google Web Toolkit, uncheck Use Google App Engine.
- Finish
- You should by now have a new project, and you need to pay attention to 3 different parts of it, as illustrated below:
Delete Template Files
Google creates a lot of interesting code for us, but for our simple canvas example, we can simplify things a bit. Simply delete
- GreetingService.java
- GreetingServiceAsync.java
- xyz.server and its contents
- xyz.shared and its contents
Add new code in xyz.client, CanvasExample.java
Here we simply copy the code from giantflyingsaucer.com and adapt it to our environment (basically the class and package names get modified):
package xyz.client;
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.canvas.client.Canvas;
import com.google.gwt.canvas.dom.client.Context2d;
import com.google.gwt.user.client.ui.RootPanel;
import com.google.gwt.user.client.Timer;
import com.google.gwt.user.client.ui.Label;
import com.google.gwt.canvas.dom.client.CssColor;
import com.google.gwt.user.client.Random;
public class CanvasExample implements EntryPoint {
Canvas canvas;
Context2d context;
static final int canvasHeight = 300;
static final int canvasWidth = 300;
static final String divTagId = "canvasExample"; // must match div tag in html file
public void onModuleLoad() {
canvas = Canvas.createIfSupported();
if (canvas == null) {
RootPanel.get().add(new Label("Sorry, your browser doesn't support the HTML5 Canvas element"));
return;
}
canvas.setStyleName("mainCanvas"); // *** must match the div tag in CanvasExample.html ***
canvas.setWidth(canvasWidth + "px");
canvas.setCoordinateSpaceWidth(canvasWidth);
canvas.setHeight(canvasHeight + "px");
canvas.setCoordinateSpaceHeight(canvasHeight);
RootPanel.get( divTagId ).add(canvas);
context = canvas.getContext2d();
final Timer timer = new Timer() {
@Override
public void run() {
drawSomethingNew();
}
};
timer.scheduleRepeating(1500);
}
public void drawSomethingNew() {
// Get random coordinates and sizing
int rndX = Random.nextInt(canvasWidth);
int rndY = Random.nextInt(canvasHeight);
int rndWidth = Random.nextInt(canvasWidth);
int rndHeight = Random.nextInt(canvasHeight);
// Get a random color and alpha transparency
int rndRedColor = Random.nextInt(255);
int rndGreenColor = Random.nextInt(255);
int rndBlueColor = Random.nextInt(255);
double rndAlpha = Random.nextDouble();
CssColor randomColor = CssColor.make("rgba(" + rndRedColor + ", "
+ rndGreenColor + "," + rndBlueColor + ", " + rndAlpha + ")");
context.setFillStyle(randomColor);
context.fillRect( rndX, rndY, rndWidth, rndHeight);
context.fill();
}
}
Edits
CanvasExample.gwt.xml
This file can be simplified. Make sure you remove the reference to the xyz.shared folder.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE module PUBLIC "-//Google Inc.//DTD Google Web Toolkit 2.6.1//EN"
"file:////Applications/Utilities/gwt-2.6.1/gwt-module.dtd">
<module rename-to='canvasexample'>
<inherits name='com.google.gwt.user.User'/>
<inherits name='com.google.gwt.user.theme.clean.Clean'/>
<entry-point class='xyz.client.CanvasExample'/>
<source path='client'/>
<!-- allow Super Dev Mode -->
<add-linker name="xsiframe"/>
</module>
web.xml
Remove all the servlets statements
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
version="2.5"
xmlns="http://java.sun.com/xml/ns/javaee">
<welcome-file-list>
<welcome-file>CanvasExample.html</welcome-file>
</welcome-file-list>
</web-app>
CanvasExample.html
Here we get rid of unnecessary code and create a div tag with an Id that must match the divTag string in our java application.
<!doctype html>
<html>
<head>
<meta http-equiv="content-type" content="text/html; charset=UTF-8">
<link type="text/css" rel="stylesheet" href="CanvasExample.css">
<title>Canvas Example</title>
<script type="text/javascript" language="javascript"
src="canvasexample/canvasexample.nocache.js"></script>
</head>
<body>
<!-- OPTIONAL: include this if you want history support -->
<iframe src="javascript:''" id="__gwt_historyFrame" tabIndex='-1'
style="position:absolute;width:0;height:0;border:0"></iframe>
<h1>Web Application Starter Project</h1>
<div id="canvasExample"> </div>
<h1>The End!</h1>
</body>
</html>
Run the Application
- Select the file CanvasExample.java and run it as a Web Application.
- At some point a URL will appear in the Development Mode tab (http://127.0.0.1:8888/Z_canvas3.html?gwt.codesvr=127.0.0.1:9997). Double click on the URL or copy/paste it in your favorite browser (here we use Chrome).
- If everything goes well you should see random colorful rectangles appearing in a Web page (this may take a surprising long time--10 to 20 seconds--to take place):
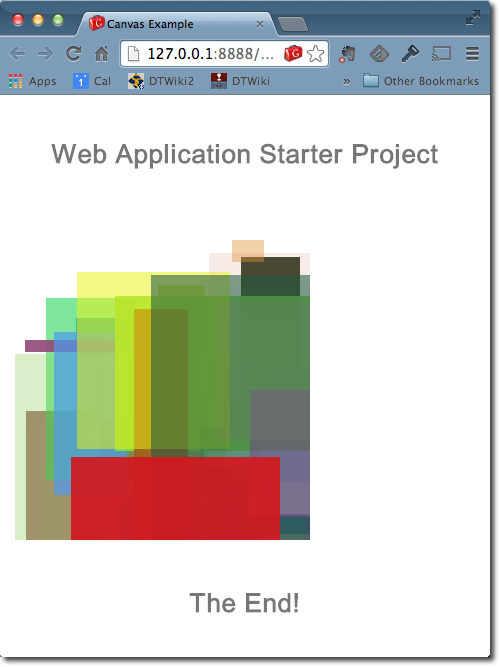