Difference between revisions of "CSC231 Homework 5 2015"
(→Problem #1) |
|||
(25 intermediate revisions by the same user not shown) | |||
Line 1: | Line 1: | ||
--[[User:Thiebaut|D. Thiebaut]] ([[User talk:Thiebaut|talk]]) 15:31, 21 October 2015 (EDT) | --[[User:Thiebaut|D. Thiebaut]] ([[User talk:Thiebaut|talk]]) 15:31, 21 October 2015 (EDT) | ||
---- | ---- | ||
− | |||
− | |||
− | |||
− | |||
− | |||
− | |||
+ | <br /> | ||
+ | __TOC__ | ||
+ | <br /> | ||
+ | <bluebox>This assignment is due on 11/04/15, at 11:55 p.m.</bluebox> | ||
+ | <br /> | ||
=Problem #1= | =Problem #1= | ||
<br /> | <br /> | ||
* Ssh to aurora.smith.edu using your 231a-xx account. | * Ssh to aurora.smith.edu using your 231a-xx account. | ||
* Go into your account's public_html directory. | * Go into your account's public_html directory. | ||
− | * Create a file with emacs, and call it '''javascriptTest.html | + | * Create a file with emacs, and call it '''javascriptTest.html'''. |
* Store the following code into it: | * Store the following code into it: | ||
<br /> | <br /> | ||
− | ::<source lang=" | + | ::<source lang="javascript"> |
<html> | <html> | ||
<body> | <body> | ||
Line 43: | Line 42: | ||
</html> | </html> | ||
</source> | </source> | ||
+ | <br /> | ||
+ | * Make the file readable: | ||
+ | |||
+ | chmod a+r javascriptTest.html | ||
+ | |||
+ | * In your favorite browser, go to this URL: http://cs.smith.edu/~231a-xx/javascriptTest.html and replace '''xx''' by your 2-letter Id. | ||
+ | * You should see a page similar to the one shown below. | ||
+ | <br /> | ||
+ | <center>[[Image:CSC231JavascriptTest.png|500px]]</center> | ||
+ | <br /> | ||
+ | ;Question: | ||
+ | : When performing arithmetic, how does Javascript score in terms of speed of execution, compared to Java and Python, as illustrated in the [[Speed_of_Instructions:_Nasm,_Java,_C%2B%2B_and_Python_comparison| page on Nasm, Java, Python]] that we discussed in class? | ||
+ | <br /> | ||
+ | : Submit your answers as a pdf file called '''hw5_1.pdf'''. Include supporting data in the file. Submit it as a pdf file in the HW 5 PB 1 section on Moodle. | ||
+ | <br /> | ||
+ | |||
+ | =Problem #2= | ||
+ | <br /> | ||
+ | This question is similar to Problem 1, but you have to measure the speed of java performing arithmetic with BigIntegers, as compared to performing arithmetic with '''int'''s. | ||
+ | |||
+ | ;Question | ||
+ | : Is using BigIntegers slower, or faster than using '''int'''s, and how much faster or slower? Please include supporting evidence in your answer. Submit a pdf of your answer called '''hw5_2.pdf''' in the HW 5 PB 2 section on Moodle. | ||
+ | <br /> | ||
+ | =Problem #3= | ||
+ | <br /> | ||
+ | * Write a program called '''hw5_3.asm''', and make sure it contains a function called _printHex() that will take the contents of eax in hexadecimal. | ||
+ | * <font color="magenta">Make sure your code is well documented and formatted nicely in columns</font>. | ||
+ | * Make your program use the 231Lib library. | ||
+ | * The main function of your program should be the following: | ||
+ | <br /> | ||
+ | <source lang="asm"> | ||
+ | |||
+ | _start: mov eax, 0x12345678 | ||
+ | call _printHex | ||
+ | call _println | ||
+ | |||
+ | mov eax, 0xABCDEF00 | ||
+ | call _printHex | ||
+ | call _println | ||
+ | |||
+ | mov eax, 0x1234ABCD | ||
+ | call _printHex | ||
+ | call _println | ||
+ | exit: mov eax, 1 | ||
+ | mov ebx, 0 | ||
+ | int 0x80 | ||
+ | |||
+ | </source> | ||
<br /> | <br /> | ||
+ | * Its output should be | ||
+ | |||
+ | 12345678 | ||
+ | ABCDEF00 | ||
+ | 1234ABCD | ||
+ | |||
+ | * Submit your program in the HW 5 PB 3 section, on Moodle. | ||
+ | <br /> | ||
+ | <showafterdate after="20151107 23:59" before="20151231 00:00"> | ||
+ | =Solution for Problems 1 & 2= | ||
+ | <br /> | ||
+ | * See [[Media:CSC231_hw5_1_solution.pdf | Kaitlyn]]'s solution for Problem 1 | ||
+ | * See [[Media:CSC231_hw5_2_solution.pdf | Kaitlyn]]'s solution for Problem 2 | ||
+ | <br /> | ||
+ | =Solution _printHex Function= | ||
+ | <br /> | ||
+ | ::<source lang="asm"> | ||
+ | section .data | ||
+ | table db "0123456789ABCDEF" | ||
+ | hexString db "xxxxxxxx" | ||
+ | tempEax dd 0 | ||
+ | |||
+ | section .text | ||
+ | _printHex: | ||
+ | pushad ; save all registers | ||
+ | mov [tempEax], eax ; save eax, as we are going to need it | ||
+ | ; several times, for all its digits | ||
+ | mov ecx, 8 ; get ready to loop 8 times | ||
+ | |||
+ | ;; get char equivalent to lower nybble of eax | ||
+ | for: and eax, 0xF ; isolate lower nybble of eax | ||
+ | add eax, table ; and translate it into ascii hex char | ||
+ | mov bl, byte[eax] ; bl <- ascii | ||
− | + | ;; make eax point to place where to put this digit in hexString | |
+ | mov eax, hexString ; beginning of table | ||
+ | add eax, ecx ; add ecx to it, since ecx counts | ||
+ | dec eax ; but eax 1 too big, decrement it | ||
+ | mov byte[eax], bl ; store ascii char at right index in hexString | ||
+ | |||
+ | ;; shift eax down by 4 bits by dividing it by 16 | ||
+ | mov ebx, 16 ; ready to shift down by 4 bits | ||
+ | mov edx, 0 | ||
+ | mov eax, [tempEax] ; get eax back | ||
+ | div ebx ; shift down by 4 | ||
+ | mov [tempEax], eax ; save again | ||
+ | |||
+ | loop for ; and repeat, 8 times! | ||
+ | |||
+ | ;; print the pattern in hexString, which contains 8 chars | ||
+ | |||
+ | mov ecx, hexString ; | ||
+ | mov edx, 8 | ||
+ | call _printString | ||
+ | |||
+ | popad | ||
+ | ret | ||
+ | |||
+ | </source> | ||
+ | </showafterdate> | ||
+ | <br /> | ||
+ | <br /> | ||
<br /> | <br /> | ||
− | |||
<br /> | <br /> | ||
+ | [[Category:CSC231]][[Category:Nasm]] |
Latest revision as of 22:07, 17 November 2015
--D. Thiebaut (talk) 15:31, 21 October 2015 (EDT)
Contents
This assignment is due on 11/04/15, at 11:55 p.m.
Problem #1
- Ssh to aurora.smith.edu using your 231a-xx account.
- Go into your account's public_html directory.
- Create a file with emacs, and call it javascriptTest.html.
- Store the following code into it:
<html> <body> <script type="text/javascript"> <!-- var a = 33; var b = 10; var c = "Test"; var i = 0; var linebreak = "<br />"; document.write("sum_i=0^100 = "); sum = 0; for ( i=0; i<=100; i++ ) { sum = sum + i; } document.write( sum ); document.write(linebreak); //--> </script> Example of Javascript arithmetic. <br /> </body> </html>
- Make the file readable:
chmod a+r javascriptTest.html
- In your favorite browser, go to this URL: http://cs.smith.edu/~231a-xx/javascriptTest.html and replace xx by your 2-letter Id.
- You should see a page similar to the one shown below.
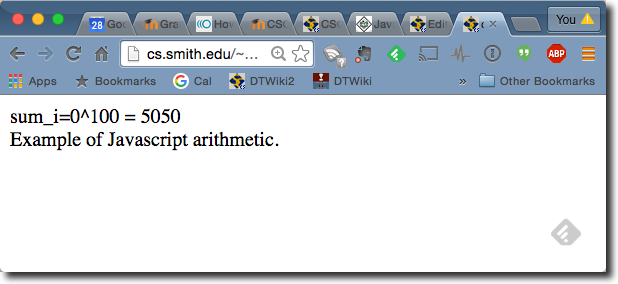
- Question
- When performing arithmetic, how does Javascript score in terms of speed of execution, compared to Java and Python, as illustrated in the page on Nasm, Java, Python that we discussed in class?
- Submit your answers as a pdf file called hw5_1.pdf. Include supporting data in the file. Submit it as a pdf file in the HW 5 PB 1 section on Moodle.
Problem #2
This question is similar to Problem 1, but you have to measure the speed of java performing arithmetic with BigIntegers, as compared to performing arithmetic with ints.
- Question
- Is using BigIntegers slower, or faster than using ints, and how much faster or slower? Please include supporting evidence in your answer. Submit a pdf of your answer called hw5_2.pdf in the HW 5 PB 2 section on Moodle.
Problem #3
- Write a program called hw5_3.asm, and make sure it contains a function called _printHex() that will take the contents of eax in hexadecimal.
- Make sure your code is well documented and formatted nicely in columns.
- Make your program use the 231Lib library.
- The main function of your program should be the following:
_start: mov eax, 0x12345678
call _printHex
call _println
mov eax, 0xABCDEF00
call _printHex
call _println
mov eax, 0x1234ABCD
call _printHex
call _println
exit: mov eax, 1
mov ebx, 0
int 0x80
- Its output should be
12345678 ABCDEF00 1234ABCD
- Submit your program in the HW 5 PB 3 section, on Moodle.
<showafterdate after="20151107 23:59" before="20151231 00:00">
Solution for Problems 1 & 2
Solution _printHex Function
section .data table db "0123456789ABCDEF" hexString db "xxxxxxxx" tempEax dd 0 section .text _printHex: pushad ; save all registers mov [tempEax], eax ; save eax, as we are going to need it ; several times, for all its digits mov ecx, 8 ; get ready to loop 8 times ;; get char equivalent to lower nybble of eax for: and eax, 0xF ; isolate lower nybble of eax add eax, table ; and translate it into ascii hex char mov bl, byte[eax] ; bl <- ascii ;; make eax point to place where to put this digit in hexString mov eax, hexString ; beginning of table add eax, ecx ; add ecx to it, since ecx counts dec eax ; but eax 1 too big, decrement it mov byte[eax], bl ; store ascii char at right index in hexString ;; shift eax down by 4 bits by dividing it by 16 mov ebx, 16 ; ready to shift down by 4 bits mov edx, 0 mov eax, [tempEax] ; get eax back div ebx ; shift down by 4 mov [tempEax], eax ; save again loop for ; and repeat, 8 times! ;; print the pattern in hexString, which contains 8 chars mov ecx, hexString ; mov edx, 8 call _printString popad ret
</showafterdate>